Numpy’s np.linspace: Evenly Spaced Sequences in Python
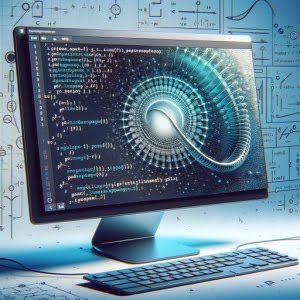
Ever wondered how to generate a sequence of evenly spaced numbers in Python? Just like a skilled mathematician, np.linspace can do this for you. This function is a part of the Numpy library, a powerful tool used by data scientists, researchers, and Python enthusiasts around the globe.
In this guide, we will walk you through the use of np.linspace, from basic usage to advanced techniques. Whether you are a beginner just starting out with Python or a seasoned programmer looking to refine your skills, this article is designed to help you understand and effectively use np.linspace in your projects.
Let’s dive right in and start exploring the power of np.linspace!
TL;DR: How Do I Use np.linspace in Python?
np.linspace in Python is a function that allows you to generate a sequence of evenly spaced numbers over a specified range. You simply specify the start and end of the range and the number of steps you want in your sequence.
Here’s a simple example:
import numpy as np
sequence = np.linspace(0, 1, 5)
print(sequence)
# Output:
# array([0. , 0.25, 0.5 , 0.75, 1. ])
In the above example, we used np.linspace to generate a sequence of 5 numbers evenly spaced between 0 and 1. The function returns a numpy array containing the sequence.
If you want to dive deeper into np.linspace and explore its advanced usage scenarios, continue reading. We have a lot more in store for you!
Table of Contents
Getting Started with np.linspace in Python
np.linspace is a function in the Numpy library in Python. It’s used to generate an array of evenly spaced numbers over a specified range. Let’s break down the parameters it takes and what they mean.
Parameters of np.linspace
np.linspace takes three main parameters:
start
– This is the starting value of the sequence.stop
– This is the end value of the sequence.num
– This is the number of evenly spaced samples to be generated. The default is 50.
Now, let’s look at a basic example of how to use np.linspace:
import numpy as np
sequence = np.linspace(0, 10, 5)
print(sequence)
# Output:
# array([ 0. , 2.5, 5. , 7.5, 10. ])
In this example, we’re asking Python to generate a sequence of 5 numbers, starting at 0 and ending at 10. The output is an array of 5 numbers: 0, 2.5, 5, 7.5, and 10. These numbers are evenly spaced over the range of 0 to 10. This is the basic usage of np.linspace in Python.
Exploring Advanced Features of np.linspace
np.linspace has a few more parameters that can be used to customize the sequence it generates. Let’s dive into them.
The Endpoint Parameter
One of these parameters is endpoint
. By default, endpoint
is set to True, meaning that the stop value is included in the sequence. However, if we set endpoint=False
, the stop value will be excluded. Let’s see it in action:
import numpy as np
sequence = np.linspace(0, 10, 5, endpoint=False)
print(sequence)
# Output:
# array([0., 2., 4., 6., 8.])
In this example, we asked Python to generate a sequence of 5 numbers, starting at 0 and ending before 10. As a result, the sequence does not include the stop value 10.
Different Data Types
np.linspace can also handle different data types. By default, it generates a sequence of float numbers. However, you can specify a different data type using the dtype
parameter. Here’s how you can generate a sequence of integers:
import numpy as np
sequence = np.linspace(0, 10, 5, dtype=int)
print(sequence)
# Output:
# array([ 0, 2, 5, 7, 10])
In this example, we asked np.linspace to generate a sequence of integers. Note that the values in the sequence are rounded down to the nearest integer. This is an important consideration when working with different data types.
These advanced features make np.linspace a flexible and powerful tool for generating sequences in Python.
Exploring Alternatives to np.linspace
While np.linspace is a powerful tool for generating sequences of numbers, Numpy offers other functions that can be used for similar purposes. Let’s take a look at two of them: np.arange and np.logspace.
Using np.arange
np.arange is another Numpy function that generates a sequence of numbers. Unlike np.linspace, np.arange creates sequences based on a step size instead of the total number of samples. Here’s an example:
import numpy as np
sequence = np.arange(0, 10, 2)
print(sequence)
# Output:
# array([0, 2, 4, 6, 8])
In this example, we asked Python to generate a sequence of numbers starting at 0 and up to (but not including) 10, with a step size of 2. As a result, we get an array of numbers from 0 to 8, incremented by 2.
Using np.logspace
np.logspace is another alternative to np.linspace. This function generates a sequence of numbers that are evenly spaced on a log scale. Here’s how you can use np.logspace:
import numpy as np
sequence = np.logspace(0, 1, 5)
print(sequence)
# Output:
# array([ 1. , 1.77827941, 3.16227766, 5.62341325, 10. ])
In this example, we asked Python to generate a sequence of 5 numbers between 10^0 (which is 1) and 10^1 (which is 10) on a log scale. The output is an array of numbers that increase by a factor of approximately 1.78, which is the base of the natural logarithm.
While np.linspace, np.arange, and np.logspace all generate sequences of numbers, they do so in different ways. Understanding these differences can help you choose the right function for your specific needs.
Addressing Common np.linspace Pitfalls
While np.linspace is a powerful tool, it’s not without its quirks. Let’s discuss some common issues that you might encounter when using np.linspace and how to address them.
Floating Point Precision Errors
One common issue is dealing with floating point precision errors. This happens because computers can’t perfectly represent some decimal numbers, leading to minor inaccuracies in the generated sequence. Here’s an example:
import numpy as np
sequence = np.linspace(0, 1, 3)
print(sequence)
# Output:
# array([0. , 0.5, 1. ])
At first glance, this output might seem perfect. But if you look closer, you might find that the actual values are slightly off due to floating point precision errors.
One way to address this issue is by rounding the values to the desired precision using the np.around function:
import numpy as np
sequence = np.linspace(0, 1, 3)
rounded_sequence = np.around(sequence, 2)
print(rounded_sequence)
# Output:
# array([0. , 0.5, 1. ])
In this example, we used np.around to round the values in the sequence to two decimal places. This can help mitigate the effects of floating point precision errors.
Remember, np.linspace is a powerful tool, but it’s important to understand its quirks and how to address them. By being aware of these issues and knowing how to handle them, you can use np.linspace effectively in your Python projects.
Understanding Numpy Arrays: The Backbone of np.linspace
To fully understand how np.linspace works, it’s crucial to understand the basics of Numpy arrays, as np.linspace returns a Numpy array.
What is a Numpy Array?
A Numpy array, or ndarray, is a grid of values, all of the same type. It provides a high-performance multidimensional array object, and tools for working with these arrays. It’s a core part of the Numpy library, which is a powerful tool for numerical computations in Python.
import numpy as np
array = np.array([1, 2, 3, 4, 5])
print(array)
# Output:
# array([1, 2, 3, 4, 5])
In this example, we created a one-dimensional Numpy array with the values 1, 2, 3, 4, and 5. You can think of this one-dimensional array as a list of numbers.
How Does np.linspace Use Numpy Arrays?
When you use np.linspace, the function generates a sequence of numbers and returns them in a Numpy array. This is why understanding Numpy arrays is crucial for understanding np.linspace.
import numpy as np
sequence = np.linspace(0, 10, 5)
print(sequence)
# Output:
# array([ 0., 2.5, 5., 7.5, 10.])
In this example, np.linspace generated a sequence of 5 numbers, starting at 0 and ending at 10. The function then returned this sequence in a Numpy array.
By understanding Numpy arrays and how they work, you can gain a deeper understanding of np.linspace and how to use it effectively in your Python projects.
Leveraging np.linspace in Larger Projects
np.linspace is not just for generating simple sequences. It can be a powerful tool when incorporated into larger scripts or projects. Let’s explore how np.linspace can be used in data visualization and simulations.
Generating Data for Plots
One common use of np.linspace is to generate data for plots. For instance, you can use np.linspace to create the x-values for a line plot. Here’s an example:
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.show()
# Output: A sine wave plot
In this example, we used np.linspace to generate 100 x-values between 0 and 10. We then calculated the sine of these values to get the corresponding y-values. Finally, we plotted the x and y values using Matplotlib, a popular plotting library in Python.
Simulating Numerical Experiments
np.linspace can also be used in simulations. For example, you might use np.linspace to generate a range of initial conditions for a physics simulation or a set of parameters for a machine learning model.
Related Numpy Functions
When working on larger projects, you might find other Numpy functions useful. To find out more, consider checking out the following resources:
- Numpy and Data Visualization – Learn how to create stunning plots in Python and gain insights into NumPy’s role in data preprocessing.
Stacking Arrays Vertically with numpy.vstack() – Explore the “numpy vstack” function and its role in vertical stacking of arrays in Python.
Generating Zero-Filled Arrays with np.zeros() – Discover the “np.zeros” function for creating NumPy arrays filled with zeros in Python.
Official Numpy Documentation – The official documentation of Numpy offering a detailed overview of the module and its functionalities.
Numpy Tutorial on TutorialsPoint – A comprehensive tutorial from TutorialsPoint covering various aspects of Numpy and its practical applications in Python.
Numpy Tutorial Video on YouTube – A YouTube video tutorial providing visual instruction for understanding and using Numpy.
By understanding how np.linspace and related methods can be used in larger projects, you can leverage Numpy’s full potential and make your Python scripts more powerful and efficient.
Wrapping Up: Mastering np.linspace in Python
In this comprehensive guide, we’ve taken a deep dive into the np.linspace function in Python. We’ve explored how to generate a sequence of evenly spaced numbers, from the basics to more advanced techniques.
We’ve learned that np.linspace takes three main parameters: start
, stop
, and num
. We’ve seen how to use these parameters to control the range and number of values in our sequence. We’ve also delved into the endpoint
and dtype
parameters, which offer additional control over the generated sequence.
We’ve addressed common issues, such as dealing with floating point precision errors, and offered solutions to mitigate these issues. We’ve also explored alternative functions like np.arange and np.logspace, which offer different ways to generate sequences of numbers.
Finally, we’ve seen how np.linspace can be used in larger projects, from generating data for plots to simulating numerical experiments. We’ve suggested related Numpy functions that might be useful in these contexts.
Here’s a quick comparison of the numpy functions discussed:
Function | Description |
---|---|
np.linspace | Generates a sequence of evenly spaced values over a specified range. |
np.arange | Generates a sequence of values with a specified step size. |
np.logspace | Generates a sequence of evenly spaced values on a log scale. |
Remember, np.linspace is more than just a function for generating sequences. It’s a powerful tool that can enhance your Python projects. Keep exploring, keep learning, and keep coding!