Spring Boot Starters | Simplify Your Maven Configurations
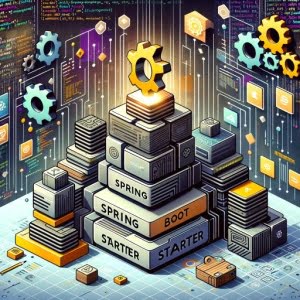
Are you wrestling with managing dependencies in your Spring Boot application? You’re not alone. Many developers find themselves in a similar struggle, but there’s a tool that can make this process a breeze.
Think of Spring Boot Starters as a dependable assistant that can simplify your Maven configuration and streamline your project setup. These starters are like a key that unlocks a smoother, more efficient development process.
In this guide, we’ll provide a comprehensive walkthrough of understanding and using Spring Boot Starters. We’ll explore everything from basic usage to advanced techniques, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Spring Boot Starters!
TL;DR: What is a Spring Boot Starter?
Spring Boot Starters
are a set of convenient dependency descriptors that you can include in your Spring Boot application. They must be included in an XML file, and specified by<groupId></groupId>
and<artifactId></artifactId>
blocks. Their use is to simplify your Maven configuration and streamline your project setup.
Here’s a simple example of how to include a Spring Boot Starter in your Maven configuration:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
In this example, we’ve included the spring-boot-starter-test
in our Maven configuration. This starter is designed for simple endpoint unit testing, by allowing you to write code that mocks the servlet request and response through your endpoint.
This is just a basic way to use Spring Boot Starters, but there’s much more to learn about managing dependencies in Spring Boot. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Spring Boot Starters
Spring Boot Starters are designed to be incredibly straightforward to use. Let’s start by understanding how to include them in your Maven configuration.
Including Spring Boot Starters in Maven Configuration
To include a Spring Boot Starter in your Maven configuration, you need to add a dependency in your pom.xml
file. Let’s consider an example where we want to build a web application using Spring Boot. In this case, we will use the spring-boot-starter-web
Starter.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
In this example, the spring-boot-starter-web
Starter is added as a dependency. This starter is designed for building web applications, including RESTful applications using Spring MVC. It simplifies the setup by providing the necessary dependencies, so you don’t have to manually specify each one.
Advantages of Using Spring Boot Starters
Spring Boot Starters offer several advantages. They simplify your Maven configuration by bundling together related dependencies. This means you spend less time managing individual dependencies and more time writing code. They also ensure that you are using compatible versions of libraries, reducing the risk of version conflicts.
Potential Pitfalls of Using Spring Boot Starters
While Spring Boot Starters are extremely helpful, they are not without their potential pitfalls. One common issue is over-reliance on Starters, which can lead to unused dependencies being included in your project, thus increasing the size of your application. It’s important to only include the Starters you need to avoid unnecessary bloat.
Another potential pitfall is version conflicts. While Starters generally manage versions well, conflicts can still occur if you are using other libraries not included in the Starter. Always be mindful of the libraries you are using and their compatibility with your chosen Starter.
Exploring Different Spring Boot Starters
Spring Boot offers a variety of Starters tailored for different purposes. Let’s delve into some of these specialized Starters and their usage.
Spring Boot Starter for Web Applications
The spring-boot-starter-web
is designed for building web applications, including RESTful applications using Spring MVC. It simplifies the setup by providing the necessary dependencies, so you don’t have to manually specify each one.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Spring Boot Starter for Data JPA
The spring-boot-starter-data-jpa
is a Starter for using Spring Data JPA with Hibernate. It simplifies database operations and reduces the amount of boilerplate code.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
Spring Boot Starter for Security
The spring-boot-starter-security
Starter provides a range of security features for your application. It includes authentication, authorization, and protection against common vulnerabilities.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Each of these Starters brings its own set of dependencies and autoconfigurations, simplifying the development process. By choosing the right Starter for your project, you can ensure that you have all the necessary dependencies without having to add them manually.
Remember, each Starter is designed for a specific purpose, so choose the one that best fits your application’s needs.
Alternative Approaches to Dependency Management
While Spring Boot Starters offer a convenient way to manage dependencies, they’re not the only game in town. Let’s explore an alternative method: manually specifying all dependencies.
Manually Specifying Dependencies
Instead of relying on a Spring Boot Starter, you can manually specify each dependency in your pom.xml
file. This gives you complete control over the versions of each library you’re using and ensures you only include the dependencies you need.
Here’s an example of manually specifying dependencies for a web application:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.8</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.8</version>
</dependency>
<!-- additional dependencies as needed -->
</dependencies>
In this example, we’ve manually added the spring-core
and spring-webmvc
dependencies for our web application. We’ve specified the version for each dependency, ensuring we’re using the versions we want.
Advantages and Disadvantages of Manually Specifying Dependencies
Manually specifying dependencies gives you complete control and ensures you’re only including the dependencies you need. This can lead to a smaller application size and fewer potential version conflicts.
However, this approach also has its downsides. It requires a deep understanding of each library and its dependencies. It also involves more work as you need to manually add each dependency and ensure they’re compatible with each other.
Recommendations
If you’re new to Spring Boot or working on a small to medium-sized project, Spring Boot Starters can simplify your dependency management and let you focus on writing code. However, for large, complex projects or if you need precise control over your dependencies, manually specifying your dependencies can be a viable alternative.
Like any tool, Spring Boot Starters can occasionally present challenges. Let’s discuss some common issues you might encounter and how to resolve them.
Resolving Version Conflicts
One common issue is version conflicts. While Spring Boot Starters usually manage versions well, conflicts can occur if you’re using other libraries not included in the Starter.
For example, let’s say you’re using a library that requires a specific version of Spring MVC, but the spring-boot-starter-web
Starter includes a different version. This might cause a version conflict.
To resolve this, you can exclude the conflicting dependency from the Starter and manually specify the correct version. Here’s how you can do this in your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.8</version>
</dependency>
In this example, we’ve excluded spring-webmvc
from spring-boot-starter-web
and manually added it with the version we want.
Handling Missing Dependencies
Another common issue is missing dependencies. If your application fails to start or behaves unexpectedly, it might be due to a missing dependency.
You can usually identify missing dependencies by examining the error messages in your application’s logs. Once you’ve identified the missing dependency, you can add it to your pom.xml
file.
<dependency>
<groupId>com.example</groupId>
<artifactId>missing-dependency</artifactId>
<version>1.0.0</version>
</dependency>
In this example, we’ve added the missing-dependency
that our application needs to function correctly.
Remember, while Spring Boot Starters simplify dependency management, they’re not infallible. Always be vigilant and ready to troubleshoot issues as they arise.
Understanding Dependencies in Java Projects
In Java projects, dependencies are external libraries or modules that your application needs to function correctly. These can range from utility libraries like Apache Commons to full-fledged frameworks like Spring MVC.
Managing these dependencies can be a challenging task. You need to ensure that you have the right versions of each dependency and that they are compatible with each other. This is where Maven comes in.
Maven: A Lifesaver for Dependency Management
Maven is a build automation tool used primarily for Java projects. One of its key features is dependency management. You specify the dependencies for your project in a pom.xml
file, and Maven takes care of downloading them and including them in your build.
Here’s an example of how you might specify a dependency in a pom.xml
file:
<dependency>
<groupId>com.example</groupId>
<artifactId>example-library</artifactId>
<version>1.0.0</version>
</dependency>
In this example, we’re telling Maven that our project depends on version 1.0.0 of example-library
from com.example
.
Simplifying Dependency Management with Spring Boot Starters
While Maven simplifies dependency management, it can still be challenging to manage dependencies for complex applications. This is especially true for Spring applications, which can have many dependencies.
Spring Boot Starters aim to simplify this process. A Starter is a pre-defined set of dependencies that are commonly used together. By including a Starter in your pom.xml
file, you’re telling Maven to include all of the dependencies in that Starter in your project.
Let’s go back to our spring-boot-starter-web
example:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
By including this Starter, we’re telling Maven to include all of the dependencies necessary for building a web application with Spring MVC. This includes libraries for handling web requests, running an embedded server, and many others.
In short, Spring Boot Starters make it easy to get up and running with Spring Boot by simplifying your Maven configuration and ensuring you have all the dependencies you need.
Scaling Up with Spring Boot Starters
As your Spring Boot projects grow in size and complexity, you’ll find Spring Boot Starters increasingly invaluable. They not only simplify the initial setup but also ease the maintenance of these larger projects.
Streamlining Large Projects with Spring Boot Starters
In larger projects, managing dependencies can become a monumental task. You might have dozens or even hundreds of dependencies, each with their version to manage. Spring Boot Starters streamline this process by bundling related dependencies together. This means you can add a single Starter to your pom.xml
file instead of dozens of individual dependencies.
Moreover, Starters ensure that you’re using compatible versions of each dependency. This significantly reduces the risk of version conflicts, which can be a major headache in large projects.
Exploring Related Concepts
Once you’ve mastered Spring Boot Starters, there are several related concepts worth exploring. These include Spring Boot AutoConfiguration and Spring Boot Actuator.
- Spring Boot AutoConfiguration is a feature that automatically configures your Spring application based on the dependencies you have added. For example, if you add
spring-boot-starter-web
, Spring Boot AutoConfiguration automatically configures your application to support web functionality. Spring Boot Actuator provides production-ready features for your Spring Boot application. It includes features like health checks, metrics collection, HTTP tracing, and more. These features can be incredibly useful in monitoring and managing your application in a production environment.
Further Resources for Spring Boot Starters
If you’re interested in learning more about Spring Boot Starters and related concepts, here are a few resources to get you started:
- IOFlood’s Java Spring Boot Article – Leverage Spring Boot starters for quick project setup.
Java Spring Framework: Overview – Understand Spring fundamentals for Java apps.
Spring Boot Actuator: Basics – Discover Spring Boot Actuator for monitoring and management.
Spring Boot’s official documentation is an excellent resource for understanding Spring Boot’s features, including Starters.
Baeldung’s Spring Boot tutorials offers in-depth guides on various Spring Boot topics, including Starters, AutoConfiguration, and Actuator.
JavaBrains’ Spring Boot YouTube series provides video tutorials on Spring Boot, making complex concepts easier to grasp.
Wrapping Up: Spring Boot Starters
In this comprehensive guide, we’ve navigated the landscape of Spring Boot Starters, a crucial tool for simplifying Maven configuration and streamlining your project setup in Spring Boot.
We began with the basics, learning how to include Spring Boot Starters in your Maven configuration, and understanding their advantages and potential pitfalls. We then moved on to more advanced usage, exploring different Spring Boot Starters for various purposes such as web, data JPA, and security.
Along the journey, we addressed common issues you might encounter when using Spring Boot Starters, such as version conflicts and missing dependencies, and provided solutions and workarounds for each issue. We also explored alternative approaches to dependency management in Spring Boot, such as manually specifying all dependencies, and weighed their pros and cons.
Here’s a quick comparison of the methods we’ve discussed:
Method | Control | Complexity | Flexibility |
---|---|---|---|
Spring Boot Starters | Moderate | Low | High |
Manually Specifying Dependencies | High | High | High |
Whether you’re just starting out with Spring Boot or you’re looking to level up your Spring Boot knowledge, we hope this guide has given you a deeper understanding of Spring Boot Starters and their role in managing dependencies.
The ability to efficiently manage dependencies is a powerful tool in your Spring Boot toolkit, and now you’re well equipped to make the most of it. Happy coding!