Python Multiline Comments / Block Comments Guide
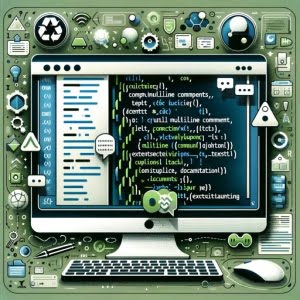
Ever been puzzled about how to write multiline comments in Python? Think of them as hidden notes in a book, spanning multiple lines, and providing detailed explanations within your code.
In this comprehensive guide, we’ll unravel the mystery of writing multiline comments in Python, starting from the fundamental basics to exploring more advanced techniques.
TL;DR: How Do I Write Multiline Comments in Python?
Multiline comments in Python are enclosed within two sets of triple quotes:
'''comment'''
. Let’s look at a simple example:
'''
This is a multiline
comment in Python
'''
# Output:
# This is a comment and will not affect the execution of your code.
In this example, everything within the triple quotes is considered a comment by Python and is ignored during code execution. It’s a handy way to add detailed explanations or deactivate specific lines of code.
Dive in deeper with us as we explore more detailed information and advanced usage scenarios of multiline comments in Python.
Table of Contents
- Python Multiline Comments: The Basics
- Multiline Comments for Function Documentation
- Exploring Alternative Commenting Techniques
- Navigating Common Multiline Comment Issues
- Understanding Comments in Python
- The Bigger Picture: Multiline Comments in Larger Projects
- Further Resources for Python Programming
- Python Multiline Comments: A Recap
Python Multiline Comments: The Basics
Python multiline comments are a way to write explanations or notes that span multiple lines. This feature is especially useful when you need to provide detailed explanations or deactivate specific lines of code.
To write a multiline comment in Python, you simply need to enclose your text within triple quotes. Here’s an example:
'''
This is a multiline comment
It spans multiple lines
Python will ignore these lines when executing the code
'''
print('Hello, World!')
# Output:
# Hello, World!
In this example, the lines within the triple quotes are the multiline comment. Python ignores these lines when executing the code, so the only output is from the print statement.
The advantage of using multiline comments is that it allows for detailed explanations without disrupting the flow of your code. However, it’s worth noting that Python doesn’t have a specific syntax for multiline comments. The triple quotes are actually a way to write multiline strings, and Python simply ignores these strings if they’re not assigned to a variable. This can potentially lead to confusion if not used properly, so it’s always a good idea to use clear and concise language in your comments.
Multiline Comments for Function Documentation
Python multiline comments can also be used to create documentation for functions or classes. This is commonly done using docstrings, which are a type of multiline comment enclosed in triple quotes. They’re placed just below the function or class definition, providing an explanation of what the function or class does.
Here’s an example of how you would use a multiline comment to document a function:
def add_numbers(a, b):
'''
This function adds two numbers
Args:
a (int): The first number
b (int): The second number
Returns:
The sum of a and b
'''
return a + b
print(add_numbers(3, 2))
# Output:
# 5
In this code block, the multiline comment (docstring) provides a detailed explanation of the add_numbers
function. It explains the purpose of the function, the arguments it takes, and what it returns.
This type of documentation is not only helpful for other developers who might use your function, but it’s also beneficial for you. It serves as a reminder of how your function works, especially when dealing with complex codebases or returning to your code after some time. This is an advanced use of multiline comments in Python, showcasing their utility beyond simple code explanations.
Exploring Alternative Commenting Techniques
Apart from the conventional method of using triple quotes for multiline comments, Python also allows the use of single-line comments to create a multiline effect. This technique involves using the hash (#
) symbol, which is Python’s syntax for single-line comments.
Here’s how you can use single-line comments to create a multiline comment effect:
# This is the first line of the comment
# This is the second line of the comment
print('Hello, World!')
# Output:
# Hello, World!
In this example, each line starting with the #
symbol is a single-line comment. Python ignores these lines during execution, resulting in the same effect as a multiline comment.
The benefit of this approach is that it’s clear to the reader that these lines are meant to be comments, as the #
symbol is the official syntax for comments in Python. However, this method can be more time-consuming if you have a lot of text, as you need to add the #
symbol to each line.
When deciding between using triple quotes or single-line comments for multiline comments, consider the length of your comment and the clarity of your code. If you’re writing a short comment, single-line comments might be more straightforward. For longer comments or function documentation, using triple quotes can be more efficient.
Like any coding practice, writing multiline comments in Python can sometimes lead to unexpected issues. Let’s explore some common problems and their solutions.
Unintentional String Creation
One common issue is unintentionally creating a string instead of a comment. Remember, Python doesn’t have a specific syntax for multiline comments. When you use triple quotes, you’re actually creating a multiline string. Python treats this as a comment only if it’s not assigned to a variable.
Here’s an example of this issue:
comment = '''
This was meant to be a comment
But it is now a string
'''
print(comment)
# Output:
# This was meant to be a comment
# But it is now a string
In this case, the text within the triple quotes is assigned to the variable comment
, and Python treats it as a string, not a comment.
Indentation Errors
Another common issue is indentation errors. In Python, indentation is crucial. If your comment isn’t indented correctly, it can lead to an IndentationError
.
Here’s an example:
def add_numbers(a, b):
'''This function adds two numbers'''
return a + b
# Output:
# IndentationError: expected an indented block
In this case, the comment isn’t indented correctly, leading to an IndentationError
. To avoid this, ensure your comments align with the code they’re associated with.
Understanding these common issues can help you write more effective multiline comments in Python, enhancing your code’s readability and maintainability.
Understanding Comments in Python
Before delving deeper into the realm of multiline comments, it’s crucial to understand what comments are and why they’re used in Python programming.
In Python, comments are snippets of text that explain the functionality of code. They’re written for humans to read, and Python’s interpreter ignores them when executing the code. This means you can write notes, explanations, or reminders without affecting the code’s functionality.
Here’s an example of a single-line comment in Python:
# This is a comment
print('Hello, World!')
# Output:
# Hello, World!
In this example, the line starting with the #
symbol is a comment. Python ignores this line when executing the code.
Comments play a pivotal role in code readability and maintenance. They make your code easier to understand, not only for others but also for yourself, especially when revisiting your code after some time. They can explain the purpose of specific code sections, provide information about the code’s author and creation date, and even help debug the code.
In the context of Python multiline comments, they serve the same purpose but over multiple lines. This is particularly useful when you need to provide a detailed explanation or temporarily deactivate a block of code. Understanding this fundamental concept is the first step towards mastering Python multiline comments.
The Bigger Picture: Multiline Comments in Larger Projects
Multiline comments are not just for simple scripts; they hold significant relevance in larger projects too. When working on a complex project, it’s common to have multiple scripts, functions, and classes. Having detailed multiline comments can greatly enhance the readability and maintainability of such projects.
Consider this example:
def complex_function(a, b, c):
'''
This function performs a complex operation
Args:
a (int): The first parameter
b (int): The second parameter
c (int): The third parameter
Returns:
The result of the complex operation
'''
# Complex code goes here
return result
# Output:
# This is a comment and will not affect the execution of your code.
In this example, the multiline comment (docstring) provides a detailed explanation of the complex_function
. This is incredibly useful for anyone reading the code, as they can understand the purpose of the function, its inputs, and its outputs without having to decipher the complex code.
Exploring Python Docstrings and Comment Best Practices
If you’re interested in learning more about Python multiline comments, you might want to explore Python docstrings. Docstrings are a type of multiline comment used for documentation. They’re written in triple quotes and placed just below the definition of a function, class, or module.
Along with docstrings, it’s also worth learning about comment best practices. This includes writing clear and concise comments, avoiding unnecessary comments, and updating your comments as your code changes. Remember, the goal of comments is to improve your code’s readability, so always keep the reader in mind when writing your comments.
Further Resources for Python Programming
For a full reference guide on Python Keywords, Click Here. Understanding essential Python keywords is the first step to enhacning your Python skills.
And for information on related Python Programmnig topics, we have gathered an additional list of resources:
- Improving Code Clarity with Python Comments – Learn about Python’s comment usage for adding context and explanation to your code.
Simplifying Membership Testing in Python – Master the versatile “in” keyword in Python for efficient iteration and membership testing.
Multiline Comments in Python – Linux Handbook – Learn how to use multiline comments in Python effectively.
How to Comment Multiple Lines in Python – Altcademy – A practical guide on commenting multiple lines in Python.
Python Multiline Comments – GeeksforGeeks – Understand multiline comments in Python with this detailed explanation.
Incorporating this knowledge about Python Keywords into your daily programming can make a difference in your proficiency as a Python developer. Make the most of these resources to unlock new levels of mastery with Python Keywords.
Python Multiline Comments: A Recap
In our journey to understand Python multiline comments, we’ve covered a lot of ground. From the basics of writing multiline comments using triple quotes, to documenting functions with docstrings, and even exploring alternative approaches using single-line comments. We’ve seen how these ‘hidden notes’ in our code can enhance readability and maintainability, especially in larger projects.
Let’s revisit an example of a Python multiline comment:
'''
This is a multiline comment
Python will ignore these lines during execution
'''
print('Hello, World!')
# Output:
# Hello, World!
In this example, the lines within the triple quotes are the multiline comment. Python skips over these lines during execution, making them an ideal place for explanations or notes.
We also discussed common issues that you might encounter when writing multiline comments, such as unintentional string creation and indentation errors. By understanding these potential pitfalls, you can write more effective comments and avoid confusion.
Finally, we touched on the importance of comments in larger projects and the role of docstrings for function documentation. As you continue your Python journey, remember that comments are your friends. They can make your code more understandable for others and for your future self. So don’t shy away from using them, and always aim for clear and concise language.