Python Comments Guide (With Examples)
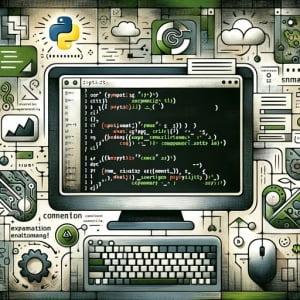
Have you ever felt overwhelmed while trying to understand a piece of Python code? Or maybe you’ve written a complex function and came back to it weeks later, only to find yourself lost in your own logic. This is where Python comments come into play.
Think of comments as secret notes or breadcrumbs in your code, guiding you and others towards understanding what’s happening under the hood.
In this comprehensive guide, we’ll walk you through the basics of writing comments in Python, their crucial role in code readability, and the best practices to follow. By the end of this guide, ‘Python comment’ will no longer be a confusing term for you, but a powerful tool in your coding arsenal.
TL;DR: How Do I Write a Comment in Python?
In Python, you write a comment using the ‘#’ symbol. Comments are the programmer’s notes that provide clarity about the code but are ignored by the Python interpreter.
Here’s a simple example:
# This is a comment
print('Hello, World!')
# Output:
# Hello, World!
In this example, ‘# This is a comment’ is a comment. It’s a note for anyone reading the code, explaining what the code does. The Python interpreter ignores this line when running the code. The next line, ‘print(‘Hello, World!’)’, is a Python command that prints the text ‘Hello, World!’ to the console.
Intrigued? Keep reading! This guide will provide a detailed understanding of Python comments, their advanced usage scenarios, and best practices.
Table of Contents
- The Basics of Python Comments
- Harnessing Docstrings for Documentation
- Exploring Advanced Documentation Techniques
- Navigating Common Pitfalls with Python Comments
- Understanding the Role of Comments in Programming
- The Impact of Comments in Larger Projects
- Expanding Your Knowledge
- Wrapping Up: The Power of Python Comments
The Basics of Python Comments
In Python, there are two types of comments: single-line and multi-line. Single-line comments are great for short explanations, while multi-line comments are used for longer descriptions or disabling multiple lines of code.
Single-line Comments
Single-line comments start with the ‘#’ symbol. Everything after ‘#’ on that line is part of the comment.
Here’s an example:
# This is a single-line comment
print('Hello, World!') # You can also place comments after code
# Output:
# Hello, World!
In this example, ‘This is a single-line comment’ and ‘You can also place comments after code’ are both comments. They provide context for the code but don’t affect the code’s execution.
Multi-line Comments
Python doesn’t have a specific syntax for multi-line comments, but there’s a workaround. You can use a series of single-line comments, or you can use triple quotes (‘”””‘ or “”’) which are typically used for docstrings.
Here’s an example of using multiple single-line comments:
# This is a multi-line comment
# Each line is a separate comment
print('Hello, World!')
# Output:
# Hello, World!
And here’s an example of using triple quotes:
"""
This is a multi-line comment
using triple quotes
"""
print('Hello, World!')
# Output:
# Hello, World!
While both methods work, keep in mind that using triple quotes for multi-line comments is not a standard practice. Triple quotes are typically reserved for docstrings, used to document modules, classes, and functions.
When and Why to Use Comments
Comments are essential for providing context to your code. They help others (and future you) understand the purpose and functionality of your code. However, it’s crucial to strike a balance. Over-commenting can make your code messy and hard to read, while under-commenting can leave readers confused. Use comments to explain complex logic, assumptions, and important decisions in your code.
Remember, good comments don’t describe the code, they explain why the code is written the way it is.
Harnessing Docstrings for Documentation
As you delve deeper into Python, comments evolve into a more structured form called ‘docstrings’. Docstrings, short for documentation strings, are a type of comment used to explain what a function, module, or class does. They’re more formal and are used by Python’s help() function and automatic documentation tools.
Crafting Docstrings
Docstrings are written between triple quotes, allowing them to span multiple lines. They’re placed right after the definition of a function, module, or class.
Here’s an example:
def greet(name):
"""
This function greets the person passed into the name parameter
:param name: The name of the person to greet
:return: A greeting message
"""
return f'Hello, {name}!'
print(greet('World'))
# Output:
# Hello, World!
In this example, the docstring explains what the function ‘greet’ does, its parameters, and what it returns. This information can be accessed using Python’s built-in help() function:
help(greet)
# Output:
# Help on function greet in module __main__:
#
# greet(name)
# This function greets the person passed into the name parameter
# :param name: The name of the person to greet
# :return: A greeting message
The Power of Docstrings
Docstrings serve as an accessible reference for your code, making it easier for others (and future you) to understand the purpose and behavior of your functions, modules, and classes. They’re especially useful in larger codebases and open-source projects where multiple people might work on the same code.
However, just like with comments, it’s important to find a balance. A good docstring should provide useful information, but it shouldn’t be so long that it becomes a chore to read. Aim for concise and clear descriptions that explain the ‘why’ and ‘how’ of your code, not just the ‘what’.
Exploring Advanced Documentation Techniques
Python provides several other ways to document your code beyond comments and docstrings. One such method is ‘Type Hints’. Introduced in Python 3.5, type hints are a new syntax for declaring the expected data type of a variable, a function’s arguments, and return value.
Understanding Type Hints
Type hints improve code readability by making the data types explicit. They are especially useful in large projects or when working with others, as they provide a clear understanding of a function’s expected input and output.
Here’s an example:
def greet(name: str) -> str:
return f'Hello, {name}!'
print(greet('World'))
# Output:
# Hello, World!
In this example, ‘name: str’ is a type hint indicating that the function ‘greet’ expects a string as an argument. ‘-> str’ is another type hint indicating that the function returns a string.
The Power of Type Hints
Type hints don’t affect the execution of your code; Python still remains a dynamically-typed language. They’re just hints and can be ignored without causing any errors. However, they can be used by tools like Mypy to catch potential bugs before running the code.
While type hints add another layer of clarity to your code, they can also make your code look busier. It’s important to use them judiciously, where they add value. As with comments and docstrings, the key is to find a balance.
In the end, whether you choose to use comments, docstrings, type hints, or a combination of these, the goal is to make your code as understandable and maintainable as possible. Remember, good code isn’t just about ‘what’ it does, but also about ‘how’ and ‘why’ it does it.
While comments and docstrings are powerful tools to improve code readability, they come with their own set of potential issues. Let’s discuss some common pitfalls and how to avoid them.
Over-Commenting
It might be tempting to comment every line of your code, but it often leads to cluttered and hard-to-read code. Comments should add value and clarity. If a line of code is self-explanatory, it probably doesn’t need a comment.
For instance, consider this code:
# This code prints 'Hello, World!' to the console
print('Hello, World!')
# Output:
# Hello, World!
The comment in this example is unnecessary. The print statement is straightforward and doesn’t need extra explanation.
Writing Vague Comments
Vague comments are as bad as no comments. A comment like ‘# Fix the bug’ doesn’t provide any useful information. Instead, explain what the bug was and how the code fixes it.
Consider this example:
# Fix the bug
x = 10
y = 0
if y != 0:
z = x / y
A more useful comment might be:
# Check if y is zero to prevent a ZeroDivisionError
x = 10
y = 0
if y != 0:
z = x / y
Ignoring Docstrings
Docstrings are an essential part of Python programming, especially for larger projects. Ignoring them can lead to confusion and difficulties in maintaining the code. Always document your functions, classes, and modules with clear and concise docstrings.
Remember, the goal of comments and docstrings is to make your code more understandable. Use them wisely and your code will be much easier to read, maintain, and debug.
Understanding the Role of Comments in Programming
In the realm of programming, comments serve as indispensable tools that foster understanding and maintainability of code. But what exactly are comments, and why are they so important?
What are Comments?
In Python, comments are lines that exist in your code but are ignored by the Python interpreter. They’re written for humans to read and not for machines. They start with the hash symbol (#), and everything following it on that line is considered a comment.
Here’s an example:
# This line is a comment
print('This line is code')
# Output:
# This line is code
In this example, ‘This line is a comment’ is a comment and doesn’t affect the execution of the code. ‘print(‘This line is code’)’ is a line of code that prints a message to the console.
The Importance of Comments
Comments are like the secret sauce of good programming. They provide context, explain the logic behind the code, and make it easier for others (and future you) to understand what’s going on. This is especially important in complex projects or when working in a team setting.
A well-placed comment can save hours of debugging and confusion. It can explain why a certain approach was chosen, what a complex piece of code does, or remind you of any assumptions or important decisions made during coding.
Improving Readability and Maintenance
Comments significantly improve the readability of your code. They provide a high-level overview of what the code does, making it easier to understand the code’s flow without getting lost in the details.
Furthermore, comments make your code more maintainable. They serve as a guide when you need to update or debug your code, helping you understand the original intent and logic. This is particularly useful in large projects or when your code will be handled by others.
In conclusion, comments, when used wisely, can be a powerful tool in your Python programming toolkit. They enhance the readability and maintainability of your code, making it easier to work with in the long run.
The Impact of Comments in Larger Projects
As your Python projects grow in size and complexity, the importance of comments and docstrings becomes even more apparent. In large-scale projects or open-source contributions, comments serve as a roadmap, guiding other developers through your code.
Consider an open-source project where multiple developers, possibly from around the world, contribute to the code. In such a scenario, comments and docstrings are vital to convey the purpose and functionality of your code. They help maintain a consistent understanding of the project among all contributors.
Here’s a simple example of a function with a descriptive docstring in a hypothetical open-source project:
def calculate_distance(point_a: tuple, point_b: tuple) -> float:
"""
Calculate the Euclidean distance between two points in a 2D space.
:param point_a: A tuple representing the coordinates (x, y) of the first point
:param point_b: A tuple representing the coordinates (x, y) of the second point
:return: The Euclidean distance between point_a and point_b
"""
return ((point_b[0] - point_a[0])**2 + (point_b[1] - point_a[1])**2)**0.5
print(calculate_distance((1, 2), (4, 6)))
# Output:
# 5.0
In this example, the docstring clearly explains the purpose of the function, its parameters, and its return value. Any contributor can understand what this function does and how to use it, even without any context.
Expanding Your Knowledge
While comments and docstrings are essential tools for writing clean and maintainable code, they’re just the tip of the iceberg. There’s a whole world of best practices and techniques to explore, such as code documentation, clean code practices, code reviews, and more. Each of these topics can greatly enhance your coding skills and make you a better programmer.
Further Resources on Python Programming
The journey to understanding Python Keywords doesn’t stop here – dive deeper with these carefully selected resources designed to build on what you’ve learned:
- Beginner’s Guide to Python Keywords – Master Python programming with knowledge of crucial keywords.
Python nonlocal: Accessing Variables in Nested Functions – Understand the role of “nonlocal” in Python.
Writing Multiline Comments in Python – Explore Python’s multiline comment syntax for adding extensive annotations.
Python Introduction – Official Documentation – Get started with Python by understanding the basics.
Python Comments Guide – Understand the role and best practices of using comments in Python coding.
Comments in Python Tutorial – A step-by-step guide on how and why to write comments in Python.
To deepen your understanding of these topics, consider exploring resources like the official Python documentation, online programming courses, coding bootcamps, and programming books. Remember, the journey to becoming a great programmer is a continuous learning process, and every step you take in expanding your knowledge is a step in the right direction.
Wrapping Up: The Power of Python Comments
Throughout this guide, we’ve explored the essential role of comments in Python programming. From simple single-line comments to more advanced docstrings and type hints, we’ve seen how these tools can greatly enhance the readability and maintainability of your code.
In Python, comments are lines that start with a ‘#’ symbol and are ignored by the Python interpreter. They serve as notes to the programmer, providing clarity and context to the code. However, it’s important to avoid over-commenting and writing vague comments, as these can lead to confusion and cluttered code.
Docstrings, written between triple quotes, provide a more structured way to document your code. They explain the purpose and behavior of functions, modules, and classes, making your code easier to understand and maintain. Remember, a good docstring is concise and clear, explaining the ‘why’ and ‘how’ of your code, not just the ‘what’.
For an even more advanced approach, we discussed type hints. Introduced in Python 3.5, type hints declare the expected data type of a variable or a function’s arguments and return value. They make your code more explicit and can be used by tools like Mypy to catch potential bugs before running the code.
Finally, we discussed the importance of comments in larger projects and open-source contributions. In such scenarios, comments serve as a roadmap, guiding other developers through your code.
To summarize, comments, docstrings, and type hints are powerful tools in your Python programming toolkit. When used wisely, they can make your code more understandable, easier to debug, and more enjoyable to work with. So the next time you find yourself writing Python code, remember the power of a well-placed comment!