The Multiple Methods to Find Absolute Value in Java
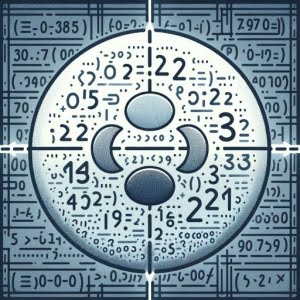
Are you struggling to find the absolute value in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling absolute values in Java, but we’re here to help.
Think of Java’s Math.abs() function as a compass – guiding you to the true north in the world of numbers. It’s a versatile and handy tool for various mathematical tasks.
In this guide, we’ll walk you through the process of finding the absolute value in Java, from the basics to more advanced techniques. We’ll cover everything from the basic usage of Math.abs() function to handling edge cases and alternative approaches.
Let’s get started and master the absolute value in Java!
TL;DR: How Do I Get the Absolute Value in Java?
Ther are multiple methods to get the absolute value in Java. One of them is the Math.abs() function used with the syntax,
int newVariable = Math.abs(variabletoGetAbsFrom);
. The goal of this function, and others, will be to find the absolute value of a variable.
Here’s a quick example:
int a = -10;
int b = Math.abs(a);
System.out.println(b);
// Output:
// 10
In this example, we have a negative integer a
with a value of -10
. We use the Math.abs()
function to get the absolute value of a
, which is 10
, and store it in the variable b
. We then print b
to the console, and as expected, the output is 10
.
This is a basic way to get the absolute value in Java, but there’s much more to learn about the Math.abs() function and its applications. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Understanding the Math.abs() Function
- Handling Edge Cases in Java Absolute Value
- Exploring Alternative Approaches to Java Absolute Value
- Troubleshooting Java Absolute Value Issues
- Understanding Java’s Number Data Types
- The Concept of Absolute Value
- The Practicality of Absolute Value in Java
- Further Resources for Java Absolute Value Mastery
- Wrapping Up: Absolute Value in Java
Understanding the Math.abs() Function
In Java, the Math.abs()
function is a part of the Math class, and it’s used to return the absolute value of the argument it receives. This function can handle different data types, such as int, float, double, and long.
Let’s look at a simple example to understand how it works:
int a = -15;
int b = Math.abs(a);
System.out.println(b);
// Output:
// 15
In this code block, we have a negative integer a
with a value of -15
. We use the Math.abs()
function to get the absolute value of a
, which is 15
, and store it in the variable b
. We then print b
to the console, and as expected, the output is 15
.
Advantages and Potential Pitfalls
The Math.abs()
function is straightforward and easy to use, making it an excellent tool for beginners. It can handle different data types and always returns a positive value or zero.
However, there are some potential pitfalls to be aware of. For instance, if the argument passed to Math.abs()
is equal to the value of Integer.MIN_VALUE
, the result is Integer.MIN_VALUE
itself, which is negative. This is because the absolute value of Integer.MIN_VALUE
is greater than Integer.MAX_VALUE
, causing an overflow.
int a = Integer.MIN_VALUE;
int b = Math.abs(a);
System.out.println(b);
// Output:
// -2147483648
In the above example, the output is -2147483648
, which is the same as Integer.MIN_VALUE
. This may be surprising as we expected a positive value. In the next section, we’ll discuss how to handle such edge cases.
Handling Edge Cases in Java Absolute Value
As we’ve seen, using the Math.abs()
function is straightforward in most cases. But what happens when we encounter edge cases, such as Integer.MIN_VALUE
? Let’s explore how to handle such scenarios.
Dealing with Integer.MIN_VALUE
As we discussed, if the argument passed to Math.abs()
is equal to Integer.MIN_VALUE
, the result is negative due to an overflow. This is because the absolute value of Integer.MIN_VALUE
is greater than Integer.MAX_VALUE
.
int a = Integer.MIN_VALUE;
int b = Math.abs(a);
System.out.println(b);
// Output:
// -2147483648
In the above example, the output is -2147483648
, which is the same as Integer.MIN_VALUE
. This can be surprising as we expected a positive value.
A Solution to the Problem
So, how do we handle this situation? One way is to use the long
data type instead of int
. This way, we can accommodate larger values without encountering an overflow.
long a = Integer.MIN_VALUE;
long b = Math.abs(a);
System.out.println(b);
// Output:
// 2147483648
In this example, we use long
instead of int
for the variables a
and b
. This allows us to handle the absolute value of Integer.MIN_VALUE
without causing an overflow, and the output is the expected positive value 2147483648
.
Best Practices
When working with the Math.abs()
function, it’s crucial to be mindful of the data types you’re using and the values you’re dealing with. If you expect to handle large values that could potentially cause an overflow, consider using a larger data type like long
.
Remember, understanding how to handle edge cases is an essential part of mastering Java absolute value.
Exploring Alternative Approaches to Java Absolute Value
While the Math.abs()
function is the most commonly used approach to get the absolute value in Java, there are alternative methods that you can use. These include using ternary operators or bitwise operators. Let’s explore these techniques and discuss their pros and cons.
Using Ternary Operators
The ternary operator is a shortcut for the if-else
statement and can be used to calculate the absolute value in Java. Here’s an example:
int a = -10;
int b = (a < 0) ? -a : a;
System.out.println(b);
// Output:
// 10
In this code block, we use a ternary operator to check if a
is less than 0
. If a
is negative, we negate it; otherwise, we use a
as it is. The result is the absolute value of a
.
This approach provides more control over the calculation process, but it may be less readable for some people compared to the Math.abs()
function.
Using Bitwise Operators
For those who are comfortable with bitwise operations, the following method can be used to find the absolute value:
int a = -10;
int b = (a >> 31 ^ a) - (a >> 31);
System.out.println(b);
// Output:
// 10
This approach uses bitwise shift and bitwise XOR operators to calculate the absolute value. While this method can be faster than using Math.abs()
, it’s more complex and can be difficult to understand for beginners.
Recommendations
While these alternative methods can be useful in certain scenarios, they are generally more complex and less readable than using the Math.abs()
function. Unless you have a specific reason to use these alternatives, it’s generally recommended to stick with Math.abs()
for simplicity and readability.
Troubleshooting Java Absolute Value Issues
While finding the absolute value in Java using the Math.abs()
function is generally straightforward, you may encounter some issues along the way. Let’s discuss some of the common problems and their solutions.
Dealing with Overflow Errors
One of the most common issues when finding the absolute value in Java is the overflow error. This occurs when the absolute value of a number is larger than the maximum value that the data type can handle.
For instance, if you try to find the absolute value of Integer.MIN_VALUE
using the Math.abs()
function, you’ll encounter an overflow error because the absolute value of Integer.MIN_VALUE
is greater than Integer.MAX_VALUE
.
int a = Integer.MIN_VALUE;
int b = Math.abs(a);
System.out.println(b);
// Output:
// -2147483648
In this example, instead of getting a positive value, we get a negative value due to the overflow error.
Solutions to Overflow Errors
To prevent overflow errors when finding the absolute value in Java, you can use a larger data type such as long
to handle larger values.
long a = Integer.MIN_VALUE;
long b = Math.abs(a);
System.out.println(b);
// Output:
// 2147483648
In this example, we use long
instead of int
for the variables a
and b
. This allows us to handle the absolute value of Integer.MIN_VALUE
without causing an overflow, and the output is the expected positive value 2147483648
.
Key Takeaways
When dealing with absolute values in Java, it’s important to be aware of potential issues like overflow errors. By understanding these problems and knowing how to solve them, you can write more robust and reliable code.
Understanding Java’s Number Data Types
Before we dive deeper into the concept of absolute value in Java, it’s important to understand the different number data types in Java. These include byte
, short
, int
, long
, float
, and double
.
Each of these data types has a different range of values they can represent. For instance, an int
can represent values from -2147483648
to 2147483647
, while a long
can represent much larger values, from -9223372036854775808
to 9223372036854775807
.
int a = 2147483647;
long b = 9223372036854775807L;
System.out.println(a);
System.out.println(b);
// Output:
// 2147483647
// 9223372036854775807
In this example, we declare an int
variable a
and a long
variable b
with their maximum values. When we print these variables, we get the expected values.
The Concept of Absolute Value
The absolute value of a number is its distance from zero on the number line, regardless of the direction. In other words, the absolute value of a number is always positive or zero, never negative.
For instance, the absolute value of -10
is 10
, and the absolute value of 10
is also 10
. This is because both -10
and 10
are 10
units away from zero on the number line.
int a = -10;
int b = Math.abs(a);
System.out.println(b);
// Output:
// 10
In this code block, we use the Math.abs()
function to find the absolute value of a
, which is -10
. The output is 10
, which is the distance of -10
from zero on the number line.
By understanding Java’s number data types and the concept of absolute value, you’ll be better equipped to handle the process of finding the absolute value in Java.
The Practicality of Absolute Value in Java
Finding the absolute value in Java is not just a theoretical concept. It has practical applications in various fields, particularly in data analysis and game development.
Absolute Value in Data Analysis
In data analysis, absolute values are often used to calculate the distance between data points or to measure the magnitude of a quantity regardless of its direction. For instance, when calculating the mean absolute deviation, the absolute value of the deviation from the mean is used.
Absolute Value in Game Development
In game development, the absolute value can be used to calculate the distance between two objects or characters, regardless of their direction. This can be useful in collision detection, pathfinding algorithms, and more.
Expanding Your Java Mathematical Operations Knowledge
Mastering the absolute value is just the beginning. There’s a whole world of mathematical operations in Java waiting to be explored. From basic arithmetic operations to more complex mathematical functions, Java provides a rich set of tools for mathematical computations.
Further Resources for Java Absolute Value Mastery
To deepen your understanding of absolute value and other mathematical operations in Java, consider checking out the following resources:
- Java Math Class: A Quick Overview – Learn how to leverage Java’s Math class for statistical computations.
Exploring BigDecimal in Java – Learn about the BigDecimal class’s utility for handling decimal numbers with accuracy.
Math.random() Method in Java explains the Math.random() function for generating random doubles values between 0.0 and 1.0.
Java Math Class Documentation – The official Oracle documentation for the Math class in Java.
Java Programming Tutorials by GeeksforGeeks includes numerous articles on Java’s mathematical operations.
Java Courses by Codecademy are online courses that teach Java programming lessons on mathematical operations.
Wrapping Up: Absolute Value in Java
In this comprehensive guide, we’ve journeyed through the process of finding the absolute value in Java. We’ve explored the usage of the Math.abs()
function, delved into its application with different data types, and addressed common issues you might encounter along the way.
We began with the basics, learning how to use the Math.abs()
function to find the absolute value of a number in Java. We then ventured into more advanced territory, exploring how to handle edge cases, such as Integer.MIN_VALUE
, and providing solutions to these challenges.
We also looked at alternative approaches to finding the absolute value in Java, such as using ternary operators or bitwise operators. These techniques provide more control and can be faster than using Math.abs()
, but they’re also more complex and may be less readable for some.
Here’s a quick comparison of the methods we discussed:
Method | Complexity | Speed | Readability |
---|---|---|---|
Math.abs() | Low | Fast | High |
Ternary Operators | Medium | Moderate | Medium |
Bitwise Operators | High | Fast | Low |
Whether you’re just starting out with Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to find the absolute value in Java.
Mastering the absolute value is a fundamental part of Java programming. With this knowledge, you’re well-equipped to handle numerical computations in your future projects. Happy coding!