Python List .extend() Method Guide | Uses and Examples
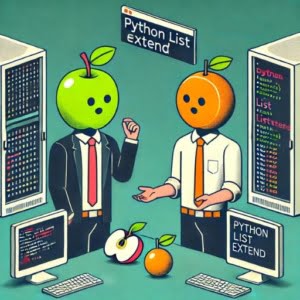
When we develop automated processes for data management, we at IOFLOOD like to use Python’s list.extend() method to append multiple elements to lists. This method offers significant advantages by allowing bulk additions to lists in a single operation. Today’s article delves into what the list.extend() method is and how to use it effectively, providing practical examples to assist our dedicated cloud service customers in enhancing their list manipulation practices.
This guide will explore list.extend()
syntax, parameters, return values, and practical usage examples. We’ll also highlight how it differs from the append()
method.
So, buckle up and get ready to unravel the power of Python’s list.extend()
method!
TL;DR: What is Python’s list.extend() method?
Python’s
list.extend()
method is a built-in function that allows you to add elements from another iterable (like a list, tuple, string, etc.) to the end of an existing list. It’s a powerful tool for list manipulation, especially when you need to merge two lists or add multiple elements to a list in one operation. To use it, you simply calllist.extend(iterable)
Example of using list.extend()
where ‘list’ is your existing list and ‘iterable’ is the iterable whose elements you want to add:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Table of Contents
Understanding list.extend() Method
In the Python programming language, list.extend()
is a built-in method that facilitates the addition of elements from an iterable (like a list, tuple, string, etc.) to the end of an existing list.
It is especially useful when you need to combine two lists or infuse multiple elements into a list in a single operation.
Syntax and Parameters
The syntax for the list.extend()
method is straightforward:
list.extend(iterable)
In this syntax, ‘list’ denotes your existing list to which you want to add elements. ‘Iterable’ represents the iterable (e.g., list, tuple, string) whose elements you wish to incorporate into the list.
Return Value
A unique characteristic of the list.extend()
method is its lack of a return value. Instead of producing a result, it modifies the original list.
If you attempt to print the result of a
list.extend()
operation, it will yieldNone
because the method doesn’t generate a result.
Example of list.extend()
returning None
:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
result = list1.extend(list2)
print(result) # Output: None
print(list1) # Output: [1, 2, 3, 4, 5, 6]
The changes are applied directly to the list.
Understanding Iterables
An iterable in Python is an object that can return its elements one at a time. Lists, tuples, strings, dictionaries, and sets are all examples of iterables.
The list.extend()
method can work with any of these, demonstrating its versatility in list manipulation.
Iterable | Description |
---|---|
List | Ordered collection of items |
Tuple | Immutable ordered collection of items |
String | Sequence of characters |
Dictionary | Collection of key-value pairs |
Set | Unordered collection of unique items |
Basic Example of extend()
The list.extend()
method traverses the iterable passed as an argument and appends each element to the end of the list. For instance, if you have a list [1, 2, 3]
and you aim to incorporate elements from another list [4, 5, 6]
, list.extend()
enables you to accomplish this in a single operation.
Example of merging elements with list.extend()
:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Separating Iterable Elements
When you employ the list.extend()
method with an iterable, it dissects the iterable and adds each element separately. For example, if you use extend()
with a string, it will decompose the string into individual characters and append each character to the list.
Example of separating iterable elements with list.extend()
:
list1 = ['a', 'b', 'c']
str1 = 'def'
list1.extend(str1)
print(list1) # Output: ['a', 'b', 'c', 'd', 'e', 'f']
Practical Usage of list.extend() Method
Having grasped the basic understanding of the list.extend()
method, it’s time to delve into its practical usage with different types of iterables.
As a versatile method, list.extend()
can handle a variety of iterables. Let’s examine its workings with a list, tuple, string, set, and dictionary.
Adding Elements from Another List
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Incorporating Elements from a Tuple
list1 = [1, 2, 3]
tuple1 = (4, 5, 6)
list1.extend(tuple1)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Infusing Elements from a String
list1 = ['a', 'b', 'c']
str1 = 'def'
list1.extend(str1)
print(list1) # Output: ['a', 'b', 'c', 'd', 'e', 'f']
Adding Elements from a Set
list1 = [1, 2, 3]
set1 = {4, 5, 6}
list1.extend(set1)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Merging Elements from a Dictionary
list1 = ['a', 'b', 'c']
dict1 = {'d': 1, 'e': 2, 'f': 3}
list1.extend(dict1)
print(list1) # Output: ['a', 'b', 'c', 'd', 'e', 'f']
In the scenario with a dictionary, the list.extend()
method incorporates the dictionary’s keys into the list. To include the dictionary’s values or key-value pairs, you can employ dict.values()
or dict.items()
, respectively.
# Example for dict.values()
list1 = ['a', 'b', 'c']
dict1 = {'d': 1, 'e': 2, 'f': 3}
list1.extend(dict1.values())
print(list1) # Output: ['a', 'b', 'c', 1, 2, 3]
# Example for dict.items()
list2 = ['x', 'y', 'z']
dict2 = {'p': 10, 'q': 20, 'r': 30}
list2.extend(dict2.items())
print(list2) # Output: ['x', 'y', 'z', ('p', 10), ('q', 20), ('r', 30)]
In the first code block, list1.extend(dict1.values())
appends the values of dict1
to list1
.
In the second code block, list2.extend(dict2.items())
appends the key-value pairs of dict2
to list2
in the form of tuples.
Extending a List Using List-Slicing
For more complex list manipulations, list.extend()
can be used alongside list-slicing. If you need to insert elements from another list at a specific position in the original list, you can slice the original list and use extend()
to add the new elements.
list1 = [1, 2, 6, 7]
list2 = [3, 4, 5]
list1[2:2] = list2
print(list1) # Output: [1, 2, 3, 4, 5, 6, 7]
Warning When Extending a List with a Set or Dictionary
When extending a list with a set or dictionary, the order of the new elements is not guaranteed due to the unordered nature of sets and dictionaries in Python. If the order of elements is crucial, it’s advisable to use a list or tuple instead.
Comparing to Python list.append()
Both the list.extend()
and list.append()
methods play a vital role in adding elements to a list in Python. However, they function differently and are utilized in distinct scenarios.
Understanding the list.append() Method
The list.append()
method in Python is designed to add a single element at the end of the list. In contrast to list.extend()
, which decomposes the iterable and integrates each of its elements separately, list.append()
incorporates the iterable as a single entity.
Let’s clarify this with an example:
list1 = [1, 2, 3]
list1.append([4, 5, 6])
print(list1) # Output: [1, 2, 3, [4, 5, 6]]
As evident, the second list was incorporated as a single element, resulting in a nested list.
Distinguishing Between extend() and append()
The primary distinction between the extend()
and append()
methods lies in their handling of iterables. While extend()
decomposes the iterable and integrates each of its elements to the list, append()
incorporates the entire iterable as a single entity.
This can be demonstrated with a practical example:
# Using extend()
list1 = ['a', 'b', 'c']
list1.extend('def')
print(list1) # Output: ['a', 'b', 'c', 'd', 'e', 'f']
# Using append()
list1 = ['a', 'b', 'c']
list1.append('def')
print(list1) # Output: ['a', 'b', 'c', 'def']
As observed, extend()
integrated each character of the string separately, while append()
incorporated the entire string as a single entity.
Choosing Between extend() and append()
The list.extend()
and list.append()
methods can be employed strategically based on the argument type, list structure, number of elements to add, and performance considerations.
Generally, extend()
is more efficient than append()
when adding multiple elements to a list. However, for adding a single element, append()
is the preferred choice.
Method | Use Case |
---|---|
extend() | Adding multiple elements to a list |
append() | Adding a single element to a list |
A thorough understanding of the practical usage of the list.extend()
method can enhance your Python coding efficiency. Its capability to handle different types of iterables renders it a versatile tool for list manipulation.
Time Complexity of extend() and append()
Both extend()
and append()
have a time complexity of O(k), where k represents the number of elements to be added.
Method | Time Complexity |
---|---|
extend() | O(k) |
append() | O(k) |
This implies that the execution time of these methods increases linearly with the number of elements.
Evaluating Memory Usage
In terms of memory usage, extend()
can be more memory-efficient than append()
when integrating many elements to a list. This is because extend()
modifies the original list, while append()
may create a new list in memory if it needs to resize the list (which occurs when the list’s capacity is exceeded).
Further Resources for Python
If you’re interested in learning more ways to utilize the Python language, here are a few resources that you might find helpful:
- Mastering List Operations in Python: Take your Python skills to the next level by learning advanced list operations and techniques.
A Comprehensive Guide to Python List Methods: This guide provides an overview of various methods available for Python lists, including manipulation, addition, removal, and searching operations.
Looping Through a List in Python: A Complete Tutorial: This tutorial covers different techniques to loop through a list in Python, demonstrating how to access list elements, iterate with enumerate(), and apply list comprehensions.
Python Syntax Cheat Sheet: Our comprehensive cheat sheet provides an overview of the basic syntax and language constructs in Python, making it a handy reference for both beginners and experienced Python programmers.
Python List extend() Method – w3schools.com: This w3schools.com resource provides information on how to use the extend() method to add elements from one list to another.
list extend() Method – programiz.com: The programiz.com tutorial covers the extend() method in Python, explaining how to extend a list by appending elements from another list.
Python | list extend() – GeeksforGeeks: GeeksforGeeks offers a tutorial on the extend() method in Python, detailing how to add elements from an iterable to a list using this method.
Final Thoughts: Python List Extending
This post has provided an in-depth exploration of Python’s list.extend()
method. We’ve learned that list.extend()
is a powerful built-in function that enables you to add elements from another iterable to the end of a list, making it a valuable tool for list manipulation.
We’ve examined the syntax and parameters of the list.extend()
method and discovered its return values. We’ve also delved into the concept of iterables in Python, and how the list.extend()
method can merge elements from another iterable into a list and separate iterable elements for list addition.
Through a series of practical examples, we’ve demonstrated how to use the list.extend()
method with different types of iterables, such as lists, tuples, strings, sets, and dictionaries. We’ve also drawn a comparison between the list.extend()
and list.append()
methods, emphasizing their key differences and use cases.
In summary, Python’s list.extend()
method is a versatile and efficient tool for adding multiple elements to a list. By understanding its workings and knowing when to use it, you can enhance the cleanliness and efficiency of your Python code, thereby boosting your data manipulation skills.