Python Tuple | Data Type Usage Guide (With Examples)
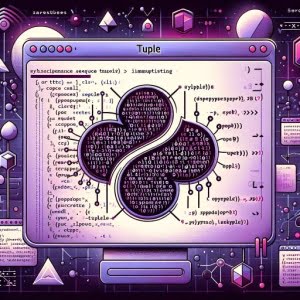
Ever felt like you’re wrestling with a locked suitcase when trying to grasp the concept of tuples in Python? You’re not alone.
Tuples, just like a tightly locked suitcase, are immutable in Python, meaning once you’ve packed them, you can’t change what’s inside. They can hold a collection of items, but once created, they remain constant.
So, why use them, and how? This comprehensive guide will walk you through everything you need to know about Python tuples, from basic use to advanced techniques. So, let’s unlock the suitcase and dive into the fascinating world of Python tuples.
TL;DR: What is a Tuple in Python?
A tuple in Python is an immutable sequence type. It’s like a list that cannot be changed once created. Here’s a simple example of a tuple:
my_tuple = ('apple', 'banana', 'cherry')
print(my_tuple)
# Output:
# ('apple', 'banana', 'cherry')
In the example above, we created a tuple named ‘my_tuple’ with three elements. Once created, you can’t add or remove elements from ‘my_tuple’ or change the existing elements. This immutability makes tuples a useful tool in Python programming, especially when you want to ensure certain data remains constant.
If you’re intrigued and want to unlock more about tuples, including their advanced usage, keep reading!
Table of Contents
Getting Started with Python Tuples
Tuples in Python are incredibly straightforward to create. You simply define your tuple by enclosing your items, separated by commas, within parentheses ()
. Here’s an example of a basic Python tuple:
fruit_tuple = ('apple', 'banana', 'cherry')
print(fruit_tuple)
# Output:
# ('apple', 'banana', 'cherry')
In the above code, we’ve created a tuple named fruit_tuple
that contains three elements. When we print fruit_tuple
, we get the output ('apple', 'banana', 'cherry')
, which is the tuple we defined.
One of the key characteristics of tuples is their immutability, i.e., once a tuple is created, you cannot change its content. This is advantageous when you have a set of data that should not be altered.
However, this immutability can also be a pitfall if you’re not careful. For instance, if you try to change an item in the tuple like this:
fruit_tuple[0] = 'orange'
# Output:
# TypeError: 'tuple' object does not support item assignment
You’ll encounter a TypeError
. Python is telling us that tuples do not support item assignment, which is a fancy way of saying you can’t change their content once they’re created.
Advanced Tuple Techniques
Python tuples offer more than just a static collection of items. They can be used in more complex ways, such as tuple unpacking, nested tuples, and as dictionary keys. Let’s explore these advanced uses.
Tuple Unpacking
Tuple unpacking allows you to assign the elements of a tuple to different variables. This can be handy when you want to extract values from a tuple in a single line. Here’s how it works:
fruit_tuple = ('apple', 'banana', 'cherry')
fruit1, fruit2, fruit3 = fruit_tuple
print(fruit1, fruit2, fruit3)
# Output:
# apple banana cherry
In the above code, we’ve assigned each item in the fruit_tuple
to a separate variable (fruit1
, fruit2
, fruit3
). When we print these variables, we get the individual elements of the tuple.
Nested Tuples
Tuples can hold other tuples within them, creating what we call ‘nested tuples’. This is useful when you want to group related data together. Here’s an example:
nested_tuple = (('apple', 'banana'), ('cherry', 'date'))
print(nested_tuple)
# Output:
# (('apple', 'banana'), ('cherry', 'date'))
In this example, nested_tuple
contains two tuples, each with two elements.
Tuples as Dictionary Keys
Because of their immutability, tuples can be used as keys in Python dictionaries, unlike lists. This is advantageous when you need a composite key, i.e., a key that is made up of multiple parts. Here’s an example:
fruit_colors = {( 'apple', 'banana'): 'yellow', ('cherry', 'date'): 'red'}
print(fruit_colors[('apple', 'banana')])
# Output:
# yellow
Here, we’ve used tuples as keys in our fruit_colors
dictionary. When we print the value associated with the key ('apple', 'banana')
, we get ‘yellow’.
Exploring Alternatives to Tuples
While tuples are powerful and versatile data structures in Python, they aren’t the only game in town. Python offers other data structures such as lists and dictionaries. Understanding these alternatives can help you make informed decisions about which data structure to use based on your specific needs.
Lists: The Mutable Alternative
Lists in Python are similar to tuples, but unlike tuples, they are mutable. This means you can add, remove, or change elements in a list after it’s created. Here’s an example of a Python list:
fruit_list = ['apple', 'banana', 'cherry']
fruit_list[0] = 'orange'
print(fruit_list)
# Output:
# ['orange', 'banana', 'cherry']
In the above code, we created a list and then changed the first element from ‘apple’ to ‘orange’. This would not be possible with a tuple. While this mutability provides flexibility, it may not be ideal when you want to ensure certain data remains constant.
Dictionaries: Key-Value Pairs
Dictionaries in Python are a collection of key-value pairs. They are mutable like lists but provide a way to associate pieces of related information. Here’s an example:
fruit_colors = {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'}
print(fruit_colors['apple'])
# Output:
# red
In the above code, we created a dictionary where each fruit (key) is associated with a color (value). This association is something unique to dictionaries and can’t be achieved with tuples or lists.
While tuples, lists, and dictionaries each have their own advantages, the best choice depends on your specific needs. If you need immutability, go with tuples. If you need to change the data, lists are your best bet. And if you need to associate values, dictionaries are the way to go.
While working with Python tuples, you may encounter some common errors or obstacles. Let’s explore these issues and their solutions, along with some best practices for optimization.
Attempting to Modify a Tuple
One of the most common errors when working with tuples is trying to modify them. Remember, tuples are immutable. Let’s see what happens when we try to change a tuple:
fruit_tuple = ('apple', 'banana', 'cherry')
fruit_tuple[0] = 'orange'
# Output:
# TypeError: 'tuple' object does not support item assignment
The above code returns a TypeError
because we’re trying to change a tuple, which is not allowed in Python. If you need a collection of items that can be modified, consider using a list instead.
Unpacking a Tuple Incorrectly
Another common issue arises when trying to unpack more or fewer variables than the tuple contains. Here’s an example:
fruit_tuple = ('apple', 'banana', 'cherry')
fruit1, fruit2 = fruit_tuple
# Output:
# ValueError: too many values to unpack (expected 2)
In this case, Python throws a ValueError
because we’re trying to unpack a three-item tuple into only two variables. To solve this, ensure the number of variables matches the number of items in the tuple.
Best Practices and Optimization
When working with tuples, it’s good practice to use them for collections of items that are not meant to change. This clearly signals to anyone reading your code that those values should remain constant. For larger collections of items, tuples can be more memory-efficient than lists, making your programs more optimized. Remember, the right data structure can make your code both easier to read and more efficient.
Delving into Python Immutability
Immutability is a fundamental concept in Python that directly impacts how we work with tuples. But what does it really mean for a data structure to be immutable, and why is it so important?
In Python, an immutable object is an object whose state cannot be changed after it’s created. This means that once you’ve defined an immutable object, such as a tuple, you can’t add, remove, or change its elements. Let’s see this in action:
fruit_tuple = ('apple', 'banana', 'cherry')
fruit_tuple[0] = 'orange'
# Output:
# TypeError: 'tuple' object does not support item assignment
In the above code, Python throws a TypeError
when we try to change an element in the tuple. This is Python’s way of enforcing immutability.
The Importance of Immutability
Immutability is important for a couple of reasons. First, it provides a degree of safety. When you define a tuple, you can be sure its data will remain constant throughout the program, preventing accidental modifications.
Second, immutability makes Python tuples hashable, meaning they can be used as dictionary keys, unlike lists. This is a powerful feature when you need a composite key, i.e., a key made up of multiple parts.
Beyond Tuples: Other Immutable Types
Tuples aren’t the only immutable objects in Python. Other immutable types include integers, floats, strings, and frozensets. Like tuples, these types can’t be changed after they’re created, providing the same benefits of safety and hashability.
Expanding Tuple Usage in Larger Projects
Python tuples, with their immutability and ability to hold multiple data types, can be incredibly useful in larger scripts or projects. For instance, they can be used to group related data together, making your code cleaner and more organized.
Consider a scenario where you’re building a script to manage a database of students. Each student could be represented as a tuple, with elements for their name, age, and grade level.
student = ('John Doe', 16, '10th grade')
print(student)
# Output:
# ('John Doe', 16, '10th grade')
In this example, the tuple student
holds related pieces of data together in a logical and organized way.
Complementary Data Structures
Tuples often work hand-in-hand with other data structures. For instance, lists of tuples are a common sight in Python code. This combination allows you to have an ordered collection of immutable data.
students = [('John Doe', 16, '10th grade'), ('Jane Doe', 15, '9th grade')]
print(students)
# Output:
# [('John Doe', 16, '10th grade'), ('Jane Doe', 15, '9th grade')]
In this example, students
is a list of tuples, each representing a different student.
For more advanced usage, you can explore how tuples interact with dictionaries, sets, and other Python data structures.
Further Resources and Readings
For a deeper dive into the previously mentioned topics, and more, check out the following guides:
- Python Data Types Logic: Simplified – Explore boolean data type usage in Python to enhance your logical reasoning skills.
Python Set Intersection: Usage Guide with Examples – Dive into set intersection operations in Python for finding common elements between sets.
Exploring Bisect Module in Python – Learn how to use bisect in Python for binary search and insertion into sorted collections.
Python Sets – Detailed guides on Python sets by Real Python.
Python’s Official Data Structures Documentation – Understanding Python’s data structures from official sources.
Python Dictionaries Tutorial – Comprehensive guide to Python dictionaries by W3Schools.
Python Tuples: A Quick Recap
Python tuples, with their immutability and ability to hold multiple data types, are a powerful tool in Python’s data structure toolkit. They provide safety and efficiency, especially when working with data that should remain constant.
Here’s a quick comparison of tuples with other Python data structures:
Data Structure | Mutable | Use Case |
---|---|---|
Tuple | No | Grouping related, constant data |
List | Yes | Grouping related, changeable data |
Dictionary | Yes | Associating related key-value pairs |
To summarize, tuples are best used when you need to group related data that should remain constant. They can be used in more advanced ways, such as tuple unpacking, nested tuples, and as dictionary keys. However, when you need to change the data or associate values, lists and dictionaries may be more suitable.
Remember, Python will throw a TypeError
if you try to modify a tuple, and a ValueError
if you try to unpack more or fewer variables than the tuple contains. To avoid these issues, ensure you understand the concept of immutability and use the right number of variables when unpacking a tuple.
Tuples often work hand-in-hand with other data structures, particularly in larger scripts or projects. To expand your knowledge, explore how tuples interact with dictionaries, sets, and other Python data structures.