NPM Packages Explained | A Node.js Essentials Guide
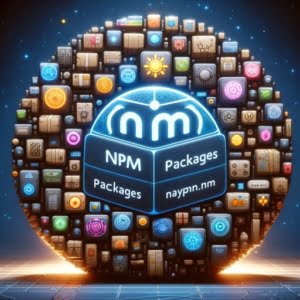
Ever felt lost when it comes to using npm packages in your projects? At IOFLOOD, we’ve encountered this challenge more than you would expect. That’s why we’ve put together a practical guide on npm packages. By following our straightforward instructions, you’ll learn how to easily incorporate powerful functionality into your projects, enhancing your development experience.
This guide will explore the essentials of npm packages, from finding and installing them to managing dependencies in your projects. With npm acting as the backbone for package management in the Node.js ecosystem, mastering its use is not just beneficial; it’s essential for any developer looking to harness the full power of Node.js. Whether you’re a beginner or looking to refine your package management skills, this article is your starting point.
Let’s dive into the world of npm packages together and empower your coding journey!
TL;DR: What Are npm Packages and How Do I Use Them?
npm packages are reusable code modules that can be integrated into Node.js projects to add functionality. To use an npm package, you first need to install it using the command
npm install [package]
.
Here’s a quick example:
npm install express
This command installs the Express package, a fast, unopinionated, minimalist web framework for Node.js. After installation, you can require it in your project like so:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Application running on port 3000');
});
# Output:
# Application running on port 3000
In this example, we’ve installed Express and used it to create a simple web server that responds with ‘Hello World!’ when you access the root URL. This demonstrates how npm packages can be quickly installed and utilized to add powerful features to your Node.js projects.
Eager to dive deeper into npm packages and unlock more advanced features? Keep reading for a comprehensive guide on mastering npm packages, from basic usage to expert-level package management.
Table of Contents
Getting Started with npm Packages
Embarking on your journey with Node.js, one of the first tools you’ll encounter is npm, the Node Package Manager. It’s the largest ecosystem of open source libraries in the world, and mastering its use is a key skill for any Node.js developer. Let’s break down the basics: finding, installing, and requiring npm packages in your Node.js projects.
Finding npm Packages
Before you can install a package, you need to find one that suits your needs. The npm website (npmjs.com) offers an extensive library of packages. Searching for a package often involves filtering through options and reading documentation to ensure it fits your project requirements.
Installing npm Packages
Once you’ve found a package, installing it is straightforward. Open your terminal, navigate to your project directory, and run:
npm install moment
This command installs the ‘moment’ package, a popular library for handling dates and times in JavaScript. The package is downloaded and saved into the ‘node_modules’ directory of your project, and an entry is added to the ‘package.json’ file under dependencies.
Requiring npm Packages
After installation, you can use the package in your project by requiring it. Here’s how you can incorporate the ‘moment’ package:
const moment = require('moment');
console.log(moment().format('MMMM Do YYYY, h:mm:ss a'));
# Output:
# October 3rd 2023, 4:50:32 pm
In this example, we require the ‘moment’ package and use it to format the current date and time. This demonstrates the power of npm packages in adding functionality to your Node.js applications with just a few lines of code.
Understanding package.json
The package.json
file is the heart of any Node.js project, managing project dependencies and scripts. When you install a package, npm adds it to this file, ensuring that anyone else working on the project can install the same dependencies with the npm install
command.
Managing Package Versions
npm packages follow semantic versioning, or semver, a system for versioning that helps you manage dependencies more effectively. It’s important to understand how to specify package versions in your package.json
file to avoid potential conflicts and ensure compatibility.
By mastering these basic steps, you’re well on your way to leveraging the vast ecosystem of npm packages to enhance your Node.js projects. Each package installed brings new possibilities, pushing the boundaries of what you can create.
Elevating Your npm Skills
As you become more comfortable with npm packages, it’s time to delve into more advanced features and commands that can significantly enhance your workflow and project efficiency. This section will guide you through managing global vs. local packages, understanding semantic versioning, and keeping your packages up-to-date with npm update
.
Global vs. Local Packages
npm allows you to install packages either globally (available across all projects) or locally (restricted to the project you’re working on). Understanding when and why to use each option is key.
Installing Globally
Global installations are ideal for tools you’ll use across multiple projects. For example, to install the nodemon
tool globally, you’d use:
npm install -g nodemon
Installing Locally
Local installations are project-specific. Here’s how you’d install axios
, a promise-based HTTP client, locally:
npm install axios
After installation, axios
can be required in your project files:
const axios = require('axios');
axios.get('https://api.example.com').then(response => {
console.log(response.data);
});
# Output:
# {"message": "API response data"}
In this code block, we’re making a GET request using axios
to fetch data from an example API. This illustrates the power of local packages in enabling specific functionalities within your projects.
Semantic Versioning Explained
npm packages adhere to semantic versioning, or semver, a 3-number system (major.minor.patch) that helps you manage package updates. Understanding semver is crucial for managing dependencies without breaking your project.
Keeping Packages Up-to-Date
Keeping your packages updated is essential for security and functionality. The npm update
command updates all packages within the constraints of your package.json
file’s semver ranges.
npm update
This command will check for available updates within your specified version ranges and apply them, ensuring your project stays current and secure.
By mastering these intermediate-level npm commands and concepts, you’re not only enhancing your Node.js projects but also ensuring they remain robust, secure, and efficient. The journey through npm’s vast ecosystem is ongoing, and these skills are vital for navigating it successfully.
Exploring Beyond npm
While npm is the cornerstone of managing Node.js packages, the JavaScript ecosystem is vibrant and constantly evolving. This means there are alternative tools and advanced npm features that can offer different advantages or better fit certain workflows. Let’s dive into the world of alternative package managers like Yarn, and uncover how to leverage advanced npm functionalities such as creating and publishing your own npm packages.
Yarn: A Popular Alternative
Yarn emerged as a strong competitor to npm, focusing on speed and security. It caches every package it downloads, so it never needs to download it again. It also parallelizes operations to maximize resource utilization. Here’s how you can start a new project with Yarn:
yarn init
Yarn and npm are largely compatible, but Yarn can sometimes handle dependencies more predictably, thanks to its ‘lockfile’.
Creating Your npm Package
Creating and publishing your own npm package can seem daunting, but it’s a rewarding way to contribute to the community. Here’s a basic rundown:
- Create a new directory for your package and navigate into it.
- Run
npm init
to start the process of creating yourpackage.json
file. - Fill out the information as prompted.
Once your package is ready, publishing it is as simple as running:
npm publish
This command publishes your package to the npm registry, making it available for others to install. Remember, you’ll need to create an npm account and log in via the CLI if you haven’t already.
Enhancing Package Security
Security is paramount when working with packages, whether they’re your own or others’. npm includes tools like npm audit
to scan your project for vulnerabilities:
npm audit
This command produces a report detailing known vulnerabilities in your dependencies and, when possible, provides guidance on how to address them.
Optimizing Package Installation Times
For large projects, installation times can become a bottleneck. Using npm’s ci
command instead of install
can significantly reduce installation times in continuous integration environments:
npm ci
Unlike npm install
, npm ci
installs dependencies directly from package-lock.json
, skipping certain user-oriented features of install
and focusing on speed and consistency.
By embracing these expert-level approaches and tools, you can enhance your Node.js development workflow, contribute to the npm ecosystem, and ensure your projects are secure and efficient. The journey through npm packages is vast and full of opportunities for growth and exploration.
Even with the vast capabilities and efficiencies npm packages bring to Node.js development, you may encounter challenges such as version conflicts, deprecated packages, and installation errors. Understanding how to troubleshoot these issues is crucial for maintaining a healthy project.
Resolving Version Conflicts
Version conflicts occur when different parts of your project require different versions of the same package. This can lead to unexpected behavior or errors. The npm ls
command helps identify the dependencies causing conflicts:
npm ls <package-name>
For example, if you’re facing issues with the lodash
package, you would run:
npm ls lodash
This command displays the dependency tree for lodash
, helping you pinpoint where the version conflict arises. From there, you can decide whether to upgrade or downgrade certain packages to resolve the conflict.
Dealing with Deprecated Packages
npm warns you when installing or using deprecated packages. While it’s tempting to ignore these warnings, deprecated packages can introduce security vulnerabilities or compatibility issues. To check for deprecated packages, use:
npm outdated
This command lists all outdated packages, including any that are deprecated. It’s a best practice to replace deprecated packages with supported alternatives or update them to a more recent, non-deprecated version.
Troubleshooting Installation Errors
Installation errors can stem from a variety of issues, from network problems to corrupted node_modules
directories. If you encounter an installation error, try clearing the npm cache and reinstalling:
npm cache clean --force
npm install
This process removes any corrupted package data from your cache and attempts a fresh installation. It’s a simple yet effective way to resolve most installation errors.
Maintaining a Healthy Dependency Tree
Keeping your dependency tree healthy involves regular audits and updates. The npm audit
command scans your project for vulnerabilities and suggests fixes:
npm audit
Following the audit’s recommendations helps ensure your project’s dependencies are secure and up-to-date. Additionally, using npm update
regularly keeps your packages at their latest compatible versions, minimizing the risk of conflicts and vulnerabilities.
Navigating the intricacies of npm packages is a continuous learning process. By mastering these troubleshooting techniques and considerations, you can maintain a robust, efficient, and secure Node.js project, leveraging the full power of npm packages.
The Roots of npm
The npm ecosystem, standing for Node Package Manager, has become synonymous with Node.js development. Since its inception in 2010, npm has evolved into the largest software registry in the world. Developers use npm to share and borrow packages, and many organizations use it to manage private development as well. But how did npm come to play such a pivotal role in the JavaScript ecosystem?
npm and JavaScript Development
npm packages are essentially building blocks for JavaScript applications. These packages can range from small utilities to entire frameworks, drastically reducing development time and complexity. The npm registry acts as a public database of these packages, where developers can publish their work and find packages created by others.
Understanding npm Packages
An npm package contains all the files needed to implement a set of functionality. Each package comes with a package.json
file, which includes metadata about the package such as its version, dependencies, scripts, and more. Here’s an example of what a package.json
might look like:
{
"name": "my-sample-package",
"version": "1.0.0",
"description": "A sample npm package",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
In this example, the package.json
file outlines basic information about the package, including a script to run tests. This file is crucial as it tells npm how to handle the package.
The npm Registry: A Hub of Innovation
The npm registry plays a critical role in the npm ecosystem. It’s where packages are published, stored, and managed. Developers can search the registry to find packages that fit their needs, contributing to a culture of code reuse and collaboration within the JavaScript community.
npm’s Impact on Software Development
npm has fundamentally changed how developers build applications. By simplifying the process of sharing and reusing code, npm has accelerated the development of JavaScript applications. It’s not just about the code; it’s about the community that npm has fostered, encouraging open-source contributions and collaboration.
By understanding the history and fundamentals of npm, developers can appreciate the depth and breadth of the npm ecosystem. It’s a testament to the power of open-source software and the collective effort of millions of developers worldwide.
Web Development and npm Packages
The role of npm packages in the development of modern web applications cannot be overstated. From enhancing build tools to providing the backbone for powerful frameworks and server-side applications, npm packages are indispensable. Let’s explore how npm packages integrate with various aspects of web development, and where you can go to deepen your understanding and skills.
npm and Build Tools
Build tools like Webpack and Gulp rely heavily on npm packages to function. For example, installing and setting up Webpack in your project involves several npm packages:
npm install --save-dev webpack webpack-cli
This command installs Webpack and its command line interface as development dependencies, preparing your project for bundling and optimization tasks.
Enhancing Frameworks with npm
Frameworks such as React, Angular, and Vue.js are installed and updated via npm. Here’s how you would start a new React project:
npx create-react-app my-app
This command utilizes npx
, an npm package runner, to scaffold a new React application. It showcases the seamless integration of npm in setting up and managing project frameworks.
Server-Side Applications with npm
Node.js server-side applications leverage npm packages for everything from setting up the server to connecting with databases. Express.js, a minimal and flexible Node.js web application framework, can be added to your project with:
npm install express
After installation, integrating Express into your application allows for easy routing and server setup, demonstrating the critical role of npm packages in server-side development.
Further Resources for npm Package Exploration
To continue your journey with npm packages and fully leverage their potential in your projects, consider exploring the following resources:
- NPM Documentation – Visit the official NPM docs for comprehensive guides on usage and package management.
Node.js Guides – Discover tutorials and guides on Node.js from the official Node.js website.
JavaScript Weekly – Stay updated with JavaScript related articles and tutorials, often featuring NPM packages.
These resources offer a wealth of information and practical advice for both beginners and experienced developers looking to deepen their understanding of npm packages and their applications in modern web development.
Wrapping Up: Using npm Packages
In this comprehensive guide, we’ve explored the vast world of npm packages, an essential component for Node.js development. From the fundamentals of finding, installing, and requiring packages in your projects to advanced techniques for managing dependencies, npm packages offer a plethora of functionalities to enhance and streamline your development process.
We began with the basics, illustrating how to navigate the npm ecosystem, find the right packages for your needs, and integrate them into your Node.js applications. We delved into the importance of the package.json
file and how to manage package versions to maintain project stability.
Moving on to more advanced topics, we explored managing global vs. local packages, understanding semantic versioning, and keeping your packages up-to-date with npm update
. These skills are crucial for any developer looking to maintain a robust and secure Node.js environment.
We also ventured into alternative package managers like Yarn and discussed creating and publishing your own npm packages, highlighting the importance of package security and optimization for installation times. These expert-level insights open new avenues for developers to contribute to and benefit from the npm ecosystem.
Aspect | Basic Use | Advanced Use | Expert Insights |
---|---|---|---|
Package Management | Finding and installing packages | Semantic versioning and npm update | Publishing packages and security |
Skill Level | Beginner | Intermediate | Expert |
As we wrap up, it’s clear that understanding npm packages is crucial for efficient Node.js development. The journey from basic usage to advanced package management is filled with opportunities for growth and exploration. We encourage you to continue delving into npm’s capabilities, contributing to the ecosystem, and leveraging the power of npm packages in your projects. With the tools and knowledge shared in this guide, you’re well-equipped to take your Node.js projects to the next level. Happy coding!