Working with Java Empty String: A Detailed Guide
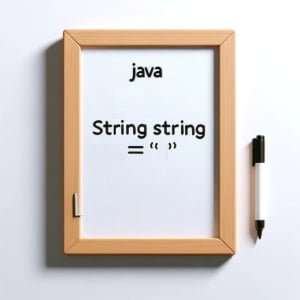
Are you finding it challenging to handle empty strings in Java? You’re not alone. Many developers grapple with this task, but there’s a method to the madness.
Like a blank canvas, an empty string in Java can be the starting point for many operations. It can be used in various scenarios, from validating user input to initializing variables and beyond.
This guide will walk you through everything you need to know about creating, checking, and handling empty strings in Java. We’ll explore Java’s String class, delve into its basic and advanced usage, and even discuss common issues and their solutions.
So, let’s dive in and start mastering empty strings in Java!
TL;DR: How Do I Create and Check an Empty String in Java?
In Java, you can create an empty string like this:
String str = "";
and check if a string is empty usingstr.isEmpty()
. This allows you to initialize a string without any characters and verify its emptiness.
Here’s a simple example:
String str = "";
boolean isEmpty = str.isEmpty();
System.out.println(isEmpty);
// Output:
// true
In this example, we first create an empty string str
. Then, we use the isEmpty()
method to check if str
is empty. The isEmpty()
method returns true
if the string is empty and false
otherwise. In this case, since str
is an empty string, the output is true
.
This is just a basic way to create and check an empty string in Java. But there’s much more to learn about handling and manipulating strings in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Creating and Checking Empty Strings in Java
- Advanced Operations with Empty Strings
- Alternative Methods to Check for Empty Strings
- Handling Common Errors with Empty Strings
- Understanding Java’s String Class and the Role of Empty Strings
- Exploring the Use of Empty Strings in Real-World Scenarios
- Exploring Related Topics: String Manipulation and Regular Expressions
- Wrapping Up: Empty Strings in Java
Creating and Checking Empty Strings in Java
In Java, creating an empty string is a straightforward process. You can initialize a string without any characters, like so:
String str = "";
In this example, str
is an empty string. There are no characters between the quotation marks.
But how can you confirm that a string is indeed empty? Java provides a built-in method called isEmpty()
for this purpose.
Here’s how you use it:
String str = "";
boolean isEmpty = str.isEmpty();
System.out.println(isEmpty);
// Output:
// true
In this code block, we first initialize str
as an empty string. We then use isEmpty()
to check whether str
is empty. The isEmpty()
method returns true
when the string is empty and false
otherwise. In our case, since str
is an empty string, the output is true
.
Implications of Using Empty Strings
Empty strings in Java are not just placeholders. They can serve various purposes in your code. For instance, they can represent the absence of input or be used as a default value for string variables.
However, it’s important to note that an empty string is not the same as a null string. An empty string is a string with no characters, while a null string is a string variable that has not been given a value. This distinction is crucial when dealing with string operations in Java.
Advanced Operations with Empty Strings
Once you’re comfortable with creating and checking empty strings, you can move on to more complex operations, such as concatenation and comparison.
String Concatenation
In Java, you can concatenate (or join) strings using the +
operator. An empty string can be a useful tool in concatenation operations. For example:
String str1 = "Hello";
String str2 = "";
String str3 = str1 + str2;
System.out.println(str3);
// Output:
// Hello
In this code block, we concatenate str1
(which contains “Hello”) and str2
(which is an empty string). The result is “Hello”, stored in str3
. As you can see, concatenating an empty string doesn’t affect the original string.
String Comparison
You can also compare an empty string with other strings. Java provides the equals()
method for string comparison. Here’s how you can use it with an empty string:
String str1 = "";
String str2 = "";
boolean isEqual = str1.equals(str2);
System.out.println(isEqual);
// Output:
// true
In this example, we compare str1
and str2
, both of which are empty strings. The equals()
method returns true
if the strings are equal, and false
otherwise. Since both str1
and str2
are empty, the method returns true
.
These examples illustrate that an empty string in Java is not just a placeholder. It’s a fully-fledged string that you can manipulate and operate on, just like any other string.
Alternative Methods to Check for Empty Strings
While the isEmpty()
method is a straightforward way to check if a string is empty, Java offers other methods that can be used for the same purpose. Two of these methods are str.length() == 0
and str.equals("")
.
Using str.length() == 0
The length()
method returns the length of a string. If a string is empty, its length is 0. Here’s how you can use this method to check if a string is empty:
String str = "";
boolean isEmpty = str.length() == 0;
System.out.println(isEmpty);
// Output:
// true
In this example, str
is an empty string, so its length is 0. Hence, str.length() == 0
returns true
.
Using str.equals("")
The equals()
method checks if two strings are the same. You can use it to check if a string is equal to an empty string (""
). Here’s an example:
String str = "";
boolean isEmpty = str.equals("");
System.out.println(isEmpty);
// Output:
// true
In this code block, str
is an empty string. So, str.equals("")
returns true
, indicating that str
is indeed an empty string.
Which Method Should You Use?
All three methods (isEmpty()
, length() == 0
, and equals("")
) can check if a string is empty. So which one should you use? It really depends on your specific use case and personal preference.
isEmpty()
is the most straightforward and readable method. On the other hand, length() == 0
and equals("")
can be more versatile. For example, length()
can also tell you how many characters are in a string, and equals()
can compare a string to any other string, not just an empty string.
Handling Common Errors with Empty Strings
Working with empty strings in Java can sometimes lead to unexpected issues. Let’s take a look at some common problems and their solutions.
Null vs Empty Strings
A common mistake is confusing an empty string with a null string. An empty string is a string with no characters (""
), while a null string is a string variable that hasn’t been assigned a value. This distinction is important because calling methods on a null string will throw a NullPointerException
.
For example, consider the following code:
String str = null;
boolean isEmpty = str.isEmpty();
System.out.println(isEmpty);
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this code, str
is null, so calling str.isEmpty()
throws a NullPointerException
.
To avoid this, you can check if a string is null before calling any methods on it:
String str = null;
boolean isEmpty = str == null || str.isEmpty();
System.out.println(isEmpty);
// Output:
// true
In this revised code, str == null || str.isEmpty()
checks if str
is either null or empty. This prevents the NullPointerException
.
Empty Strings and Whitespace
Another common issue is confusing an empty string with a string that contains only whitespace. The isEmpty()
method returns false
for a string that contains whitespace because whitespace is considered a character.
Here’s an example:
String str = " ";
boolean isEmpty = str.isEmpty();
System.out.println(isEmpty);
// Output:
// false
In this code, str
contains a space, so str.isEmpty()
returns false
.
If you want to treat strings that contain only whitespace as empty, you can use the trim()
method to remove whitespace from the start and end of the string before checking if it’s empty:
String str = " ";
boolean isEmpty = str.trim().isEmpty();
System.out.println(isEmpty);
// Output:
// true
In this revised code, str.trim().isEmpty()
returns true
because trim()
removes the space from str
, making it an empty string.
Understanding Java’s String Class and the Role of Empty Strings
The Java String class is one of the most commonly used classes in Java. It represents a sequence of characters (or a string of text). An instance of the String class can be created in two ways:
// Using a string literal
String str1 = "Hello";
// Using the new keyword
String str2 = new String("Hello");
// Output:
// No output, but str1 and str2 are both initialized with the value "Hello"
In the above example, str1
and str2
are both strings with the value “Hello”. The first method is more common because it’s simpler and more efficient.
But what happens if you don’t provide any characters between the quotes? You get an empty string:
String str = "";
// Output:
// No output, but str is initialized as an empty string
An empty string in Java is a string that contains no characters. It’s not the same as a null string, which is a string variable that hasn’t been assigned a value.
Empty strings are useful in several scenarios. For example, they can represent the absence of input or serve as a default value for string variables. They can also be used in operations like string concatenation and comparison. Understanding how to create, check, and manipulate empty strings is an essential skill for any Java programmer.
Exploring the Use of Empty Strings in Real-World Scenarios
Empty strings in Java aren’t just theoretical constructs, they have practical applications in real-world programming scenarios. Let’s explore a couple of these.
Parsing Data with Empty Strings
When parsing data from external sources such as files or databases, you may encounter empty strings. These could represent missing or optional data. In such cases, knowing how to handle empty strings becomes crucial.
For example, if you’re reading a CSV file where some fields might be empty, those fields will appear as empty strings in your Java program. You’ll need to check for and handle these empty strings appropriately to avoid errors or incorrect data.
Handling User Input
Another common scenario is handling user input. Users might submit a form without filling in all fields, leading to empty strings in your program. Again, you’ll need to check for and handle these empty strings to ensure your program behaves correctly.
Exploring Related Topics: String Manipulation and Regular Expressions
Once you’ve mastered empty strings, there are many related topics you could explore to further improve your Java skills. Two such topics are string manipulation and regular expressions.
String manipulation involves changing, splitting, joining, or otherwise manipulating strings. Regular expressions are a powerful tool for pattern matching and text processing, and they can be used in conjunction with strings for tasks like validation, searching, and replacing.
Further Resources for Java Strings
To help you delve deeper into these topics, here are some resources you might find useful:
- IOFlood’s Java String Class Article – Explore Java String comparison methods.
Exploring StringBuilder in Java – Learn about StringBuilder’s mutable nature and its advantages over String.
Java String Split: Usage Guide – Explore how to split strings into substrings using the split method in Java.
Java String Documentation provides detailed information about all its methods.
Java Regular Expressions Tutorial – A comprehensive tutorial on using regular expressions in Java.
Java String Manipulation Guide – An in-depth guide on string manipulation in Java, covering topics like string formatting, comparison and more.
Wrapping Up: Empty Strings in Java
In this comprehensive guide, we’ve delved into the world of empty strings in Java, a fundamental aspect of string manipulation in the language.
We started with the basics, learning how to create and check for empty strings. We then explored more advanced operations with empty strings, such as concatenation and comparison. We also examined alternative methods to check for empty strings, including str.length() == 0
and str.equals("")
.
Along the way, we tackled common challenges you might encounter when working with empty strings, such as distinguishing between null and empty strings, and handling strings that contain only whitespace. We provided solutions and best practices for each of these issues.
Here’s a quick comparison of the methods we’ve discussed for checking if a string is empty:
Method | Readability | Versatility |
---|---|---|
isEmpty() | High | Moderate |
length() == 0 | Moderate | High |
equals("") | Moderate | High |
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up on your skills, we hope this guide has given you a deeper understanding of empty strings in Java and their practical applications.
Understanding how to create, check, and manipulate empty strings is a key part of mastering Java’s String class. With this knowledge, you’re well-equipped to handle a wide range of string manipulation tasks in Java. Happy coding!