Unraveling Java String Class : From Basics to Advanced
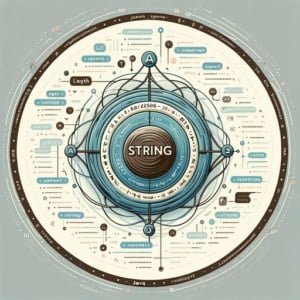
Are you finding it challenging to understand the String class in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling strings in Java, but we’re here to help.
Think of the String class in Java as a Swiss Army Knife – a versatile tool with a multitude of uses. It provides a wide range of methods for manipulating strings, making it a crucial part of Java programming.
This guide will provide a comprehensive overview of the String class, its methods, and how to effectively use it in your Java programs. We’ll cover everything from the basics of creating and manipulating strings to more advanced techniques, as well as alternative approaches.
Let’s get started and master the String class in Java!
TL;DR: What is the String Class in Java?
The String class in Java is a built-in class that represents a sequence of characters, created with the
String
keyword before a variable, such as:String sample = "Sample String";
. It also provides numerous methods for manipulating strings, such aslength(), charAt(), and substring().
It is a powerful tool in any Java programmer’s toolkit.
Here’s a simple example of creating a string in Java:
String str = "Hello, World!";
System.out.println(str);
# Output:
# Hello, World!
In this example, we’ve created a string str
and assigned it the value Hello, World!
. We then print the string to the console, resulting in the output Hello, World!
.
This is just a basic introduction to the String class in Java. There’s much more to learn about creating and manipulating strings, including advanced methods and alternative approaches. Continue reading for a deeper understanding of the String class and its methods.
Table of Contents
Diving Into Basic String Methods in Java
Let’s start our exploration of the String class by understanding how to create strings and use basic methods like length()
, charAt()
, and substring()
.
Creating Strings in Java
In Java, we can create a string simply by using the =
operator. Here’s an example:
String greeting = "Hello, Java!";
System.out.println(greeting);
# Output:
# Hello, Java!
In this code snippet, we’ve created a string named greeting
and assigned it the value Hello, Java!
. We then print the string to the console, resulting in the output Hello, Java!
.
Using the length() Method
The length()
method returns the length of a string. It’s useful when you need to know how many characters are in a string. Here’s how you can use it:
String greeting = "Hello, Java!";
int length = greeting.length();
System.out.println(length);
# Output:
# 11
In the example above, the length()
method returns 11
, which is the number of characters in the string Hello, Java!
.
Using the charAt() Method
The charAt()
method returns the character at a specific index in a string. Remember, string indices in Java start at 0
. Let’s see this method in action:
String greeting = "Hello, Java!";
char letter = greeting.charAt(7);
System.out.println(letter);
# Output:
# J
In this example, charAt(7)
returns J
, which is the character at the 7th index of the string Hello, Java!
.
Using the substring() Method
The substring()
method extracts a portion of a string. You can specify the start index, and optionally, the end index. Here’s an example:
String greeting = "Hello, Java!";
String sub = greeting.substring(7, 11);
System.out.println(sub);
# Output:
# Java
In the above code, substring(7, 11)
extracts the portion of the string from index 7
to 11
, giving us Java
.
These are just a few examples of the basic methods provided by the String class in Java. Understanding these methods is the first step in mastering string manipulation in Java.
Advanced String Manipulation in Java
Now that we’ve covered the basics, let’s delve into more advanced methods of the String class: compareTo()
, concat()
, and replace()
.
The compareTo() Method
The compareTo()
method compares two strings lexicographically (based on the Unicode value of the characters). It returns a negative integer, zero, or a positive integer if the invoking string is less than, equal to, or greater than the provided string. Here’s an example:
String str1 = "Apple";
String str2 = "Banana";
int result = str1.compareTo(str2);
System.out.println(result);
# Output:
# -1
In the above code, compareTo()
returns -1
, indicating that str1
(Apple) is lexicographically less than str2
(Banana).
The concat() Method
The concat()
method appends one string to the end of another. It’s useful when you want to combine two strings. Here’s how you can use it:
String str1 = "Hello";
String str2 = "Java!";
String result = str1.concat(str2);
System.out.println(result);
# Output:
# HelloJava!
In this example, concat()
combines str1
and str2
to give us HelloJava!
.
The replace() Method
The replace()
method replaces all occurrences of a specified character or substring with a new character or substring. Here’s an example:
String str = "Hello, Java!";
String replaced = str.replace('a', 'A');
System.out.println(replaced);
# Output:
# Hello, JAvA!
In the above code, replace('a', 'A')
replaces all occurrences of a
with A
, resulting in Hello, JAvA!
.
These methods offer more advanced string manipulation capabilities, which can be extremely useful in a variety of programming scenarios.
Exploring Alternatives to String Class in Java
While the String class is a powerful tool for string manipulation in Java, there are alternative classes that can be more efficient in certain scenarios. Let’s explore the StringBuilder
and StringBuffer
classes.
The StringBuilder Class
StringBuilder
is a mutable sequence of characters. This means you can change the string without creating a new object, making it more efficient when you need to perform repeated string modifications.
Here’s an example of using StringBuilder
:
StringBuilder sb = new StringBuilder("Hello");
sb.append(" Java!");
System.out.println(sb.toString());
# Output:
# Hello Java!
In the above code, we create a StringBuilder
object sb
and append Java!
to it. The append()
method modifies the StringBuilder
object in place, without creating a new object.
The StringBuffer Class
StringBuffer
is similar to StringBuilder
in that it’s also a mutable sequence of characters. The key difference is that StringBuffer
is thread-safe, meaning it can be used safely in multithreaded environments.
Here’s an example of using StringBuffer
:
StringBuffer sb = new StringBuffer("Hello");
sb.append(" Java!");
System.out.println(sb.toString());
# Output:
# Hello Java!
Like StringBuilder
, StringBuffer
also allows in-place modifications, but it provides thread safety at the cost of performance.
Choosing the Right Tool
While String
is an excellent choice for storing and manipulating strings, StringBuilder
and StringBuffer
offer more efficiency for repeated modifications. If thread safety is a concern, StringBuffer
is the way to go. Otherwise, StringBuilder
offers the best performance.
Troubleshooting Common Issues with String Class in Java
As with any programming tool, there can be challenges and considerations to keep in mind when using the String class in Java. One of the most common issues relates to memory usage and string immutability.
Understanding String Immutability
One of the key characteristics of the String class in Java is that strings are immutable. This means that once a string is created, it cannot be changed. Instead, every time you modify a string, a new object is created in memory.
String str = "Hello";
str = str + " Java!";
System.out.println(str);
# Output:
# Hello Java!
In this code, when we append Java!
to str
, a new string object is created to hold the result. The original string Hello
remains unchanged in memory.
Memory Issues with String Immutability
The immutability of strings can lead to memory issues in programs that perform extensive string manipulation. Each time a string is modified, a new object is created, which can quickly consume memory.
To avoid this, consider using StringBuilder
or StringBuffer
for operations that modify strings repeatedly, as these classes allow in-place modification of strings.
StringBuilder sb = new StringBuilder("Hello");
sb.append(" Java!");
System.out.println(sb.toString());
# Output:
# Hello Java!
In this example, StringBuilder
allows us to append Java!
to the original string without creating a new object, thus saving memory.
Remember, understanding the tools and their implications is key to writing efficient and effective code. The String class in Java is a powerful tool, but it’s important to use it wisely to avoid potential issues.
Unpacking Immutability and Memory in Java
To fully grasp the workings of the String class in Java, it’s crucial to understand two fundamental concepts: Immutability and the Java memory model.
What is Immutability in Java?
In Java, immutability refers to the unchangeable nature of an object once it’s created. In the context of the String class, it means that once a String object is created, it cannot be changed. Any modification to a String object results in a new object in memory.
String original = "Java";
String modified = original.concat(" String");
System.out.println(original);
System.out.println(modified);
# Output:
# Java
# Java String
In this example, the concat()
method doesn’t change the original
string. Instead, it creates a new string modified
that contains the result of the concatenation.
Understanding the Java Memory Model
The Java memory model specifies how and when different threads can see values written to shared variables by other threads. It plays a crucial role in determining how a Java program is executed in memory.
When a String object is created, it’s stored in a special area of the heap known as the String Constant Pool. This is due to the immutability of strings in Java. If a new string is created with the same value as an existing string, Java simply refers to the existing string in the pool, saving memory.
String str1 = "Hello";
String str2 = "Hello";
System.out.println(str1 == str2);
# Output:
# true
In the above code, str1
and str2
both refer to the same object in the String Constant Pool. The ==
operator returns true
, indicating that they’re indeed the same object.
Understanding these fundamental concepts is key to mastering the String class in Java and writing efficient and effective code.
String Class in Java: Beyond the Basics
Now that we’ve covered the fundamentals and advanced uses of the String class in Java, it’s time to explore how this knowledge applies to larger applications, such as web applications and databases, and delve into related concepts like regular expressions and string encoding and decoding.
String Class in Web Applications
In web applications, the String class in Java plays a crucial role in handling and manipulating text data. Whether it’s processing form inputs or generating dynamic HTML content, the methods provided by the String class are invaluable tools for web developers.
String Class in Databases
When working with databases, the String class is used extensively to construct SQL queries, process database results, and handle any text data stored in the database. Understanding how to effectively use the String class can greatly simplify your database operations.
Exploring Regular Expressions in Java
Regular expressions provide a powerful way to search, replace, and pattern match strings. Java supports regular expressions through the Pattern
and Matcher
classes, which can be used in conjunction with the String class for more complex string operations.
Understanding String Encoding and Decoding
In Java, strings are encoded as Unicode characters. Understanding how strings are encoded and decoded can help prevent common issues like character corruption and data loss, especially when working with non-English text or transferring data between different systems.
Further Resources for Java String Class
Ready to delve deeper into the String class in Java? Here are some resources that can help you further your understanding and mastery:
- Java Fundamentals Explained – Master core Java skills for building robust and scalable applications
Exploring Substring in Java – Learn about the substring method’s syntax and usage.
Java String Concatenation: Guide – Explore different approaches to concatenate strings in Java.
Java String Class Documentation provides detailed descriptions of all the methods and properties of the class.
Java Tutorials by Oracle covers a wide range of topics, including strings.
Baeldung’s Guide on Java Strings covers everything from basic string operations to more advanced topics like string encoding and decoding.
By exploring these resources and practicing with real-world examples, you’ll be well on your way to mastering the String class in Java.
Wrapping Up: Java String Class
In this comprehensive guide, we’ve journeyed through the vast world of the String class in Java, a fundamental and powerful tool for handling and manipulating text data in your Java programs.
We started with the basics, learning how to create strings and use basic methods like length()
, charAt()
, and substring()
. We then delved into more advanced methods like compareTo()
, concat()
, and replace()
, providing you with a deeper understanding of the String class and its capabilities.
Along the way, we tackled common issues you might face when using the String class, such as memory issues due to string immutability, and provided solutions to help you overcome these challenges. We also explored alternative approaches to string handling in Java, introducing you to the StringBuilder
and StringBuffer
classes.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
String | Immutable, thread-safe | Can lead to memory issues for extensive string manipulation |
StringBuilder | Mutable, better performance for repeated modifications | Not thread-safe |
StringBuffer | Mutable, thread-safe | Slightly slower performance due to thread safety |
Whether you’re just starting out with Java or you’re looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of the String class and its capabilities.
With its balance of versatility and power, the String class is a fundamental tool for any Java programmer. Now, you’re well-equipped to handle and manipulate strings in your Java programs. Happy coding!