Java String Concatenation | Complete Method Guide
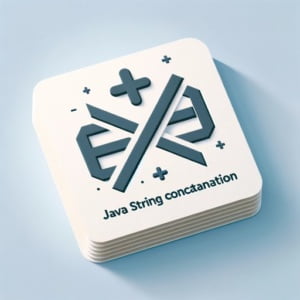
Java string concatenation is a common operation we perform to construct dynamic messages and logs at IOFLOOD. String concatenation allows us to combine multiple strings performed with various methods. Today’s article will cover all methods of how to concatenate strings in Java, to assist our dedicated cloud service customers in enhancing their code.
In this guide, we’ll walk you through string concatenation Java processes, from the basics to more advanced techniques. We’ll cover everything from using the ‘+’ operator, to more efficient methods like StringBuilder and StringBuffer, and even alternative approaches.
Let’s dive in and start mastering how to add to a string in Java!
TL;DR: How To Concatenate Strings in Java?
The simplest way to concatenate strings in Java is using the ‘+’ operator. For instance, you can concatenate two strings,
str1
andstr2
, with syntax:String result = str1 + str2;
.
Here’s a simple example:
String str1 = "Hello, ";
String str2 = "World!";
String result = str1 + str2;
System.out.println(result);
// Output:
// 'Hello, World!'
In this example, we’ve used the ‘+’ operator to concatenate two strings, str1
and str2
. The result is a new string that combines the contents of str1
and str2
, which is then printed to the console.
This is a basic way to concatenate strings in Java, but there are more efficient methods, especially for larger projects. Continue reading for a comprehensive guide on Java string concatenation, including more advanced techniques and alternative approaches.
Table of Contents
Using ‘+’: Java String Concatenation
The ‘+’ is one of the built-in Java operators and the most basic tool for string concatenation. It’s simple to use and understand, making it a great starting point for beginners.
Here’s how it works: When you use the ‘+’ operator between two strings, Java combines them into a single string. This operation is known as string concatenation.
Let’s take a look at an example:
String str1 = "Java ";
String str2 = "String Concatenation";
String result = str1 + str2;
System.out.println(result);
// Output:
// 'Java String Concatenation'
In this example, we’ve used the ‘+’ operator to concatenate the strings str1
and str2
. The result is a new string that combines the contents of str1
and str2
, which is then printed to the console.
Advantages of the ‘+’ Operator
The main advantage of the ‘+’ operator is its simplicity. It’s straightforward to use and easy to understand, making it an excellent tool for beginners or for simple string concatenation tasks.
Pitfalls of ‘+’ String Append Java Operator
However, the ‘+’ operator has its drawbacks. For one, it can be inefficient, especially in loops or when concatenating a large number of strings. This is because each use of the ‘+’ operator creates a new string object, which can slow down your program and use up more memory. This can be a drawback for especially long strings in java.
In the next sections, we’ll explore more efficient methods of string concatenation in Java, suitable for larger projects or more complex scenarios.
Basic Methods: String Append Java
As you dive deeper into Java string concatenation, you’ll encounter more efficient methods, particularly when dealing with larger projects. Two such methods involve using the StringBuilder
and StringBuffer
classes.
Understanding StringBuilder and StringBuffer
StringBuilder
and StringBuffer
are mutable sequences of characters, meaning they can be modified after they are created. This feature makes them more efficient than the ‘+’ operator when concatenating multiple strings.
Let’s explore an example of using StringBuilder
:
StringBuilder sb = new StringBuilder();
sb.append("Hello, ");
sb.append("World!");
System.out.println(sb.toString());
// Output:
// 'Hello, World!'
In this example, we create a StringBuilder
object and use the append
method to add strings. The result is a single string that combines the appended strings, which is then printed to the console.
StringBuffer
works in a similar way, but it is thread-safe, meaning it is safe to use in multithreaded programs. However, this thread-safety comes with a slight performance cost compared to StringBuilder
.
Advantages of StringBuilder and StringBuffer
The main advantage of StringBuilder
and StringBuffer
is their efficiency. They are particularly useful when concatenating a large number of strings or when concatenating strings in a loop. Unlike the ‘+’ operator, they do not create a new string object with each concatenation, which saves memory and improves performance.
Best Practices for Using StringBuilder and StringBuffer
While StringBuilder
and StringBuffer
are more efficient than the ‘+’ operator, it’s important to use them correctly. Here are a few best practices:
- Use
StringBuilder
when you don’t need thread-safety, as it is faster thanStringBuffer
. - Use
StringBuffer
when you need to concatenate strings in a multithreaded program. - Avoid converting a
StringBuilder
orStringBuffer
to a string frequently, as this can reduce performance. - When using
StringBuilder
orStringBuffer
in a loop, create theStringBuilder
orStringBuffer
object outside the loop to avoid creating a new object with each iteration.
Advanced Java String Concatenation
As you gain more experience with Java string concatenation, you might find yourself looking for more advanced or specialized methods. Two such methods include using the concat()
method and the StringUtils
class from Apache Commons Lang.
The concat() Method
The concat()
method is a built-in method in the String
class that concatenates the specified string to the end of the current string.
Here’s an example:
String str1 = "Hello, ";
String str2 = "World!";
String result = str1.concat(str2);
System.out.println(result);
// Output:
// 'Hello, World!'
In this example, we use the concat()
method to concatenate str2
to the end of str1
. The result is a new string that combines str1
and str2
.
However, like the ‘+’ operator, the concat()
method creates a new string object, which can be inefficient for large numbers of strings or in a loop.
StringUtils Class from Apache Commons Lang
The StringUtils
class in Apache Commons Lang provides several methods for manipulating strings, including a join()
method for string concatenation.
Here’s an example:
import org.apache.commons.lang3.StringUtils;
String str1 = "Hello, ";
String str2 = "World!";
String result = StringUtils.join(str1, str2);
System.out.println(result);
// Output:
// 'Hello, World!'
In this example, we use the join()
method from StringUtils
to concatenate str1
and str2
. This method is particularly useful when concatenating a large number of strings, as it is more efficient than the ‘+’ operator or the concat()
method.
Choosing the Right Method for Java String Concatenation
Each of these methods has its advantages and disadvantages, and the best one to use depends on your specific situation. If you’re working with a small number of strings, the ‘+’ operator or the concat()
method may be sufficient. For larger numbers of strings or in a loop, StringBuilder
, StringBuffer
, or StringUtils.join()
may be more efficient. And if you’re working in a multithreaded program, StringBuffer
is a safe choice.
Tips for String Concatenation Java
As with any programming task, you may encounter certain issues or considerations when concatenating strings in Java. Let’s discuss some of the most common ones and provide solutions and workarounds.
Memory Inefficiency with the ‘+’ Operator
One common issue is memory inefficiency when using the ‘+’ operator in a loop. As we’ve discussed, the ‘+’ operator creates a new string object each time it’s used. This can lead to excessive memory usage and slow performance in a loop.
Here’s an example:
String result = "";
for (int i = 0; i < 1000; i++) {
result += "Hello, World! ";
}
System.out.println(result);
// Output:
// 'Hello, World! Hello, World! Hello, World! ...'
In this example, a new string object is created in each iteration of the loop, which can be inefficient.
A more efficient approach would be to use StringBuilder
or StringBuffer
in a loop, as they modify the existing string object rather than creating a new one.
Thread-Safety Considerations with StringBuilder and StringBuffer
Another consideration is thread-safety when using StringBuilder
and StringBuffer
. StringBuilder
is not thread-safe, meaning it can lead to issues in a multithreaded program. On the other hand, StringBuffer
is thread-safe, but this comes with a slight performance cost.
Here’s an example of a thread-safe string concatenation using StringBuffer
:
StringBuffer sb = new StringBuffer();
sb.append("Hello, ");
sb.append("World!");
System.out.println(sb.toString());
// Output:
// 'Hello, World!'
In this example, we use StringBuffer
to safely concatenate strings in a multithreaded program.
In conclusion, when concatenating strings in Java, it’s important to consider the specific requirements and constraints of your program. Whether it’s memory efficiency, performance, or thread-safety, there’s a suitable method for every scenario.
Understanding How to add to a string
To fully grasp Java string concatenation, it’s crucial to understand the fundamentals of the String
class and its primary characteristic: immutability.
What is the String Class in Java?
In Java, String
is a final class, meaning it cannot be inherited or subclassed. It represents a sequence of characters, like a sentence or a word. The String
class provides numerous methods to perform operations on strings, including concatenation.
The Immutability of Strings in Java
A key feature of strings in Java is their immutability. Once a String
object is created, it cannot be changed. This might seem counterintuitive when we talk about string concatenation, but here’s what actually happens:
When you concatenate two strings, Java doesn’t modify either of the original strings. Instead, it creates a new String
object that contains the result of the concatenation.
Here’s an example:
String str1 = "Hello, ";
String str2 = "World!";
String result = str1 + str2;
System.out.println(str1); // 'Hello, '
System.out.println(str2); // 'World!'
System.out.println(result); // 'Hello, World!'
In this example, str1
and str2
remain unchanged after the concatenation. The result of the concatenation is a new string, stored in result
.
Why Does Immutability Matter in String Concatenation?
The immutability of strings has significant implications for string concatenation. Because each concatenation operation creates a new String
object, concatenating strings using the ‘+’ operator can be inefficient, especially in loops or when dealing with a large number of strings. This is why alternative methods like StringBuilder
, StringBuffer
, or StringUtils.join()
can be more efficient for these scenarios.
Understanding the String
class and its immutability is key to mastering string concatenation in Java. With this knowledge, you can make informed decisions about which concatenation method to use in different situations.
Practical Uses of String Concatenation
Java string concatenation is not just a fundamental concept—it’s also a powerful tool in larger projects. For instance, you might use string concatenation to build SQL queries or generate dynamic HTML content in a web application.
Building SQL Queries with Java String Concatenation
When interacting with a database in Java, you often need to build SQL queries dynamically. String concatenation is a common way to construct these queries. However, always remember to sanitize inputs to prevent SQL injection attacks.
Generating Dynamic HTML Content
In web development, you might use Java string concatenation to generate dynamic HTML content. For example, you might concatenate strings to create a custom HTML template, or to insert dynamic data into an HTML page.
Exploring Related Concepts in Java
Java string concatenation is just one aspect of string manipulation in Java. If you’re interested in going beyond concatenation, you might also explore related concepts like string formatting, string comparison, and string conversion in Java.
Further Resources for Mastering Java String Operations
To deepen your understanding of Java string operations, here are a few resources that you might find helpful:
- Fundamentals Covered: The String Class in Java – Learn to handle whitespace in Java Strings.
Exploring ReplaceAll Functionality in Java – Master replaceAll usage for complex string transformations in Java.
Trimming Strings in Java – Learn about trimming whitespace characters such as spaces, tabs, and newlines.
Oracle’s Java Documentation on String provides a detailed overview of all the methods available in the
String
class.Java String Tutorial by DigitalOcean tutorial offers a guide on various operations you can perform with strings in Java.
Baeldung’s Guide on Java Strings covers a wide range of topics related to strings in Java, including concatenation.
Recap: String Concatenation Java
In this comprehensive guide, we’ve journeyed through the world of Java string concatenation, exploring its various methods, their usage, and the common issues you might encounter.
We started with the basics, learning how to use the ‘+’ operator for simple string concatenation. We then delved into more advanced techniques, such as using the StringBuilder
and StringBuffer
classes for more efficient string concatenation. Along the way, we tackled common challenges like memory inefficiency and thread safety, providing you with solutions and workarounds for each issue.
We also explored alternative approaches to string concatenation, such as using the concat()
method and the StringUtils
class from Apache Commons Lang. These methods offer their own advantages and trade-offs, giving you a range of options to choose from based on your specific needs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Simplicity | Efficiency | Thread-Safety |
---|---|---|---|
‘+’ Operator | High | Low | High |
StringBuilder | Moderate | High | Low |
StringBuffer | Moderate | High | High |
concat() Method | High | Moderate | High |
StringUtils.join() | Low | High | High |
Whether you’re just starting out with Java string concatenation or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the different methods and their nuances.
With this knowledge in hand, you’re now well-equipped to handle any string concatenation task in Java. Happy coding!