Java replaceAll() Method: Usage Cases and Examples
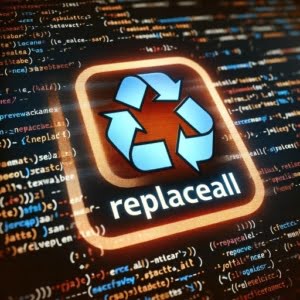
Are you finding it challenging to replace strings in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling string replacements in Java, but we’re here to help.
Think of Java’s replaceAll method as a skilled craftsman – it can seamlessly mold your strings with precision, replacing all occurrences of a specific pattern with another. It’s a powerful tool in your Java toolkit, particularly when dealing with large strings or complex replacements.
In this guide, we’ll walk you through the process of using the replaceAll method in Java, from its basic usage to more advanced techniques. We’ll cover everything from simple string replacements to dealing with regular expressions (regex), as well as alternative approaches and common pitfalls.
Let’s get started and master the replaceAll method in Java!
TL;DR: How Do I Use the replaceAll Method in Java?
The
replaceAll
method in Java is used to replace all occurrences of a specified regex with a replacement string, for example to replace all instances of ‘with’ to ‘without’, you would use the syntax,String newStr = str.replaceAll("with", "without");
. It’s a powerful tool for string manipulation in Java.
Here’s a simple example:
String str = "Hello, World!";
String newStr = str.replaceAll("World", "Java");
System.out.println(newStr);
// Output:
// 'Hello, Java!'
In this example, we’ve used the replaceAll
method to replace all occurrences of ‘World’ with ‘Java’ in the string. The result is a new string: ‘Hello, Java!’.
This is a basic way to use the
replaceAll
method in Java, but there’s much more to learn about string manipulation and handling regular expressions. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Understanding Java’s replaceAll Method
- Tackling Complex Replacements with Regex
- Exploring Alternative Methods for String Replacement in Java
- Troubleshooting Common Issues with Java replaceAll
- The Building Blocks: Java’s String Class and Regular Expressions
- Expanding Your Horizons: The Power of String Replacement
- Wrapping Up: Mastering Java’s replaceAll Method
Understanding Java’s replaceAll Method
The replaceAll
method in Java is a part of the String class. It’s used to replace each substring of this string that matches the given regular expression with the given replacement.
Here’s the method signature:
public String replaceAll(String regex, String replacement)
In this method, regex
is the regular expression to which this string is to be matched, and replacement
is the string to be substituted for each match.
Let’s take a look at a simple example:
String str = "I love apples, apples are my favorite fruit.";
String newStr = str.replaceAll("apples", "oranges");
System.out.println(newStr);
// Output:
// 'I love oranges, oranges are my favorite fruit.'
In this example, all occurrences of the word ‘apples’ are replaced with ‘oranges’. The replaceAll
method scans the string from the beginning to the end, replacing each matching substring with the replacement string.
The replaceAll
method is case-sensitive, so it won’t replace a string if the case doesn’t match. For example, ‘apples’ and ‘Apples’ are considered different strings.
Advantages and Potential Pitfalls
The replaceAll
method is a powerful tool for string manipulation, but it’s not without its potential pitfalls. Here are a few things to keep in mind:
- Advantage: It’s a straightforward and efficient way to replace all occurrences of a string.
- Advantage: It supports regular expressions, allowing for complex replacements.
- Potential Pitfall: It might not behave as expected with certain special characters, as they may be interpreted as regex.
- Potential Pitfall: It’s case-sensitive, which can lead to missed replacements if not handled properly.
In the next section, we’ll delve into more complex replacements using regular expressions with the replaceAll
method.
Tackling Complex Replacements with Regex
As you become more comfortable with the replaceAll
method, you might find yourself needing to handle more complex replacements. This is where regular expressions (regex) come into play.
Regex is a sequence of characters that forms a search pattern. It’s incredibly useful for string manipulation, and Java’s replaceAll
method fully supports it.
Let’s take a look at an example where we use regex with the replaceAll
method:
String str = "I am learning Java 101, 102, 103";
String newStr = str.replaceAll("\d+", "XXX");
System.out.println(newStr);
// Output:
// 'I am learning Java XXX, XXX, XXX'
In this example, \d+
is a regex that matches one or more digits. We’re using it with the replaceAll
method to replace all sequences of digits in the string with ‘XXX’.
Understanding the Differences
When using regex with the replaceAll
method, it’s important to understand that the method will interpret the first parameter as a regular expression. This can lead to unexpected results if you’re not familiar with regex syntax.
For instance, the dot character (‘.’) in regex is a special character that matches any character. If you’re trying to replace all dots in a string using replaceAll
, you might run into problems:
String str = "www.example.com";
String newStr = str.replaceAll(".", "-");
System.out.println(newStr);
// Output:
// '-----------------'
To correctly replace the dots, you would need to escape them using two backslashes (\.
):
String str = "www.example.com";
String newStr = str.replaceAll("\\.", "-");
System.out.println(newStr);
// Output:
// 'www-example-com'
Best Practices
When using replaceAll
with regex, keep these best practices in mind:
- Be aware of special characters in regex and escape them if necessary.
- Use precompiled patterns with
Pattern
andMatcher
classes for better performance when dealing with complex regex or large texts. - Always test your regex to ensure it’s working as expected before using it with
replaceAll
.
Exploring Alternative Methods for String Replacement in Java
While replaceAll
is a powerful tool for string manipulation, Java provides other methods for replacing strings, such as replace
and StringBuilder
. These methods can sometimes be more efficient or easier to use, depending on the scenario.
Using the replace Method
The replace
method is similar to replaceAll
, but it doesn’t support regular expressions. It’s simpler and can be faster when dealing with straightforward replacements.
Here’s an example of using replace
:
String str = "I love apples, apples are my favorite fruit.";
String newStr = str.replace("apples", "oranges");
System.out.println(newStr);
// Output:
// 'I love oranges, oranges are my favorite fruit.'
As you can see, the replace
method works similarly to replaceAll
for simple replacements. However, it won’t work for regex patterns.
Using StringBuilder for String Replacement
StringBuilder
is another alternative for string replacement in Java. It’s particularly useful when you’re dealing with large strings or performing numerous replacements, as it’s more efficient than replaceAll
or replace
.
Here’s an example of using StringBuilder
for string replacement:
StringBuilder str = new StringBuilder("I love apples, apples are my favorite fruit.");
int index = str.indexOf("apples");
while (index != -1) {
str.replace(index, index + "apples".length(), "oranges");
index = str.indexOf("apples", index + "oranges".length());
}
System.out.println(str.toString());
// Output:
// 'I love oranges, oranges are my favorite fruit.'
In this example, we’re using a loop to find and replace all occurrences of ‘apples’ with ‘oranges’. StringBuilder
allows us to modify the string in place, which can be more efficient than creating a new string with each replacement.
Comparing the Methods
Method | Supports Regex | Performance | Complexity |
---|---|---|---|
replaceAll | Yes | Moderate | Moderate |
replace | No | High | Low |
StringBuilder | No | Very High | High |
As you can see, each method has its advantages and disadvantages. replaceAll
is versatile and supports regular expressions, but it can be slower than the alternatives. replace
is faster and simpler, but it doesn’t support regex. StringBuilder
offers the best performance, but it’s more complex and doesn’t support regex.
Choosing the right method depends on your specific needs. If you need to handle complex replacements with regex, replaceAll
is your best bet. For simple replacements, replace
can be faster and easier to use. If performance is a concern, particularly with large strings or many replacements, StringBuilder
can be an efficient alternative.
Troubleshooting Common Issues with Java replaceAll
While using the replaceAll
method in Java, you may encounter a few common issues. These can range from regex syntax errors to null pointer exceptions. Let’s discuss some of these problems and their solutions.
Dealing with Regex Syntax Errors
Regex syntax errors are common when using replaceAll
. These errors occur when the regex pattern is not correctly formatted.
Here’s an example that causes a regex syntax error:
String str = "Hello, World!";
try {
String newStr = str.replaceAll("Hello(", "Hi");
System.out.println(newStr);
} catch (PatternSyntaxException e) {
System.out.println("Invalid regex pattern");
}
// Output:
// 'Invalid regex pattern'
In this example, the opening parenthesis in the regex pattern is not closed, causing a PatternSyntaxException
. To avoid this, make sure your regex pattern is correctly formatted.
Handling Null Pointer Exceptions
A null pointer exception can occur if you try to call replaceAll
on a null string. Here’s an example:
String str = null;
try {
String newStr = str.replaceAll("Hello", "Hi");
System.out.println(newStr);
} catch (NullPointerException e) {
System.out.println("Cannot call replaceAll on a null string");
}
// Output:
// 'Cannot call replaceAll on a null string'
In this example, we’re trying to call replaceAll
on a null string, causing a NullPointerException
. To avoid this, always check if your string is null before calling replaceAll
.
Other Considerations
- Performance: If you’re dealing with large strings or performing many replacements,
replaceAll
can be slow. Consider usingStringBuilder
for better performance. - Special Characters: Remember that some characters have special meanings in regex. If your replacement string contains dollar signs (
$
) or backslashes (\
), you’ll need to escape them with a backslash.
By understanding these common issues and their solutions, you can use the replaceAll
method more effectively in your Java programs.
The Building Blocks: Java’s String Class and Regular Expressions
To fully grasp the replaceAll
method, it’s important to understand the fundamentals of Java’s String class and the concept of regular expressions.
The Java String Class
In Java, strings are objects that represent sequences of characters. The java.lang.String
class is used to create and manipulate strings.
String str = "Hello, World!";
System.out.println(str);
// Output:
// 'Hello, World!'
In this example, we’re creating a string object str
and printing it to the console. The String
class offers many methods for manipulating strings, such as replaceAll
.
Understanding Regular Expressions
Regular expressions, or regex, are a powerful tool for matching patterns in strings. They’re used in many programming languages, including Java.
Here’s an example of using regex to match any digit in a string:
String str = "I have 2 apples";
boolean hasDigit = str.matches(".*\d+.*");
System.out.println(hasDigit);
// Output:
// true
In this example, .*\d+.*
is a regex that matches any string containing one or more digits. We’re using it with the matches
method to check if our string contains a digit.
Understanding the String
class and regular expressions is crucial for mastering the replaceAll
method. With these fundamentals, you can create complex replacements and efficiently manipulate strings in Java.
Expanding Your Horizons: The Power of String Replacement
String replacement, especially with the replaceAll
method, is an essential skill in Java programming. It has wide-ranging applications beyond simple text manipulation.
String Replacement in File Handling
In file handling, you often need to read text files and manipulate their content. This could involve replacing certain patterns or cleaning up the data. The replaceAll
method can be a handy tool for such tasks.
String fileContent = "Hello, World! This is a text file.";
String newContent = fileContent.replaceAll("World", "Java");
// Output:
// 'Hello, Java! This is a text file.'
In this example, we’re replacing ‘World’ with ‘Java’ in the content of a text file.
String Replacement in Data Processing
Data processing often involves cleaning and transforming data. String replacement is a common operation in data cleaning. You might need to remove unwanted characters, replace abbreviations, or standardize the data format.
String rawData = "Date: 2022/01/01, Temp: 30C";
String cleanedData = rawData.replaceAll("/", "-").replaceAll("C", " Celsius");
// Output:
// 'Date: 2022-01-01, Temp: 30 Celsius'
In this example, we’re cleaning raw data by replacing slashes with dashes in the date format and replacing ‘C’ with ‘ Celsius’ for clarity.
Diving Deeper: String Formatting and Regular Expressions
If you want to further enhance your string manipulation skills, consider exploring related concepts like string formatting and regular expressions. String formatting allows you to create formatted strings with placeholders, while regular expressions enable you to perform complex pattern matching and replacements.
Further Resources for Mastering Java String Manipulation
To deepen your understanding of Java string manipulation, consider checking out the following resources:
- Tips and Techniques for Using the String Class in Java – Learn to handle special characters in Java Strings.
Java String Concatenation Techniques – Master string concatenation techniques for combining strings efficiently in Java.
Exploring String.join Functionality in Java – Learn about specifying delimiter strings and providing iterable collections of strings.
Oracle’s Java Documentation – A guide on strings in Java, including string manipulation methods.
Regular-Expressions.info – A tutorial on using regular expressions in Java.
Baeldung’s Guide to Java Strings covers various aspects of Java strings, including creation, manipulation, and comparison.
These resources offer in-depth tutorials and examples to help you master Java string manipulation and the replaceAll
method.
Wrapping Up: Mastering Java’s replaceAll Method
In this comprehensive guide, we’ve journeyed through the world of Java’s replaceAll
method, a powerful tool for string manipulation.
We began with the basics, learning how to use replaceAll
to replace all occurrences of a specific pattern with another in a string. We then ventured into more advanced territory, exploring complex replacements using regular expressions. Along the way, we tackled common challenges you might face when using replaceAll
, such as regex syntax errors and null pointer exceptions, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to string replacement in Java, comparing replaceAll
with other methods like replace
and StringBuilder
. Here’s a quick comparison of these methods:
Method | Supports Regex | Performance | Complexity |
---|---|---|---|
replaceAll | Yes | Moderate | Moderate |
replace | No | High | Low |
StringBuilder | No | Very High | High |
Whether you’re just starting out with replaceAll
or you’re looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of replaceAll
and its capabilities.
With its versatility and support for regular expressions, replaceAll
is a powerful tool for string manipulation in Java. Happy coding!