Creating Python Docker Images: Complete Tutorial
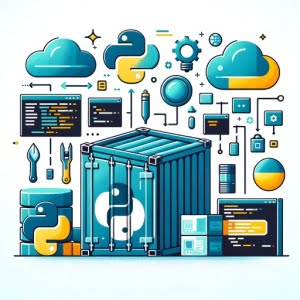
Are you finding it challenging to create a Python Docker image? You’re not alone. Many developers find themselves puzzled when it comes to packaging their Python applications into neat Docker containers.
Think of Docker as a master chef – it can help you prepare your Python application into a gourmet dish, ready to be served anywhere.
Whether you’re dealing with a simple script or a complex web application, understanding how to create a Python Docker image can significantly streamline your deployment process.
In this guide, we’ll walk you through the process of creating a Python Docker image, from the basics to more advanced techniques. We’ll cover everything from writing a Dockerfile, optimizing it, to alternative approaches for creating Python Docker images.
Let’s get started!
TL;DR: How Do I Create a Python Docker Image?
To create a Python Docker image, you need to write a Dockerfile that specifies the Python base image and your application dependencies. Then, you build the Docker image with the
docker build
command.
Here’s a simple example:
FROM python:3.7
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
CMD ["python", "./your-daemon-or-script.py"]
You can build the Docker image with the following command:
docker build -t my-python-app .
This command builds the Docker image and tags (-t) it as my-python-app
. The .
specifies that Docker should look for the Dockerfile in the current directory.
This is a basic way to create a Python Docker image, but there’s much more to learn about Docker and Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Crafting Your First Python Dockerfile
Creating a Dockerfile for a Python application is the first step towards packaging your application into a Docker image. The Dockerfile is essentially a set of instructions that Docker follows to build your image. Let’s dive into the basics of writing a Dockerfile for a Python application.
Here’s an example of a simple Dockerfile:
FROM python:3.7
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
CMD ["python", "./your-daemon-or-script.py"]
Let’s break down what each line does:
FROM python:3.7
: This line specifies the Python base image. It’s the starting point for our Docker image. Thepython:3.7
image contains Python 3.7 and all the dependencies required to run a Python application.WORKDIR /app
: This line sets the working directory inside the Docker container to/app
. All subsequent commands will be run in this directory.COPY . /app
: This line copies the current directory (.
) from the host machine to the/app
directory in the Docker container.RUN pip install -r requirements.txt
: This line installs the Python dependencies specified in therequirements.txt
file. It’s crucial to include all your application’s dependencies in therequirements.txt
file.CMD ["python", "./your-daemon-or-script.py"]
: This line is the command that the Docker container will run when it starts up. It’s running our Python script.
Now, to build the Docker image, you would run the following command in your terminal:
docker build -t my-python-app .
This command tells Docker to build an image based on the Dockerfile in the current directory (.
) and tag it (-t
) as my-python-app
.
After running this command, you should see output indicating that Docker is building the image. If successful, you’ll have a Python Docker image ready to run!
While this basic Dockerfile will work for many Python applications, there are potential pitfalls to be aware of. For example, if your application has many dependencies, the Docker image could become large and slow to build. Additionally, if your application requires specific versions of dependencies, you’ll need to specify these in your requirements.txt
file to ensure your application runs correctly. In the next section, we’ll discuss how to optimize your Dockerfile and manage dependencies more effectively.
Optimizing Your Dockerfile and Managing Dependencies
As your Python application grows and evolves, you may find that the basic Dockerfile we discussed earlier needs some enhancements. Let’s talk about optimizing your Dockerfile, managing dependencies, handling data persistence, and leveraging Docker Compose for multi-container applications.
Dockerfile Optimization and Dependency Management
One common issue with Docker images is their size. Large images take longer to build and push to a Docker registry. They can also consume significant disk space. To mitigate this, we can leverage a few strategies.
Firstly, consider using a slim version of the Python base image, like python:3.7-slim
. These slim images have fewer packages installed, which reduces the image size.
Secondly, be mindful of the order of commands in your Dockerfile. Docker builds images in layers, and it caches these layers. If a layer changes, Docker will rebuild it and all the layers that come after it.
For example, if you change a line of code in your Python application, Docker will invalidate the cache and rebuild the COPY . /app
layer and all subsequent layers. To leverage Docker’s caching effectively, put the commands that change most frequently towards the end of your Dockerfile.
Here’s an optimized version of our previous Dockerfile:
FROM python:3.7-slim
WORKDIR /app
COPY requirements.txt ./
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "./your-daemon-or-script.py"]
In this Dockerfile, we’ve made a few changes:
- We’re using the
python:3.7-slim
base image to reduce the image size. - We’re copying the
requirements.txt
file and installing the Python dependencies before copying the rest of the application code. This allows Docker to cache the image layer with the installed dependencies, which can save time when building the image if your application code changes but your dependencies do not.
Handling Data Persistence
If your application needs to persist data, you’ll need to use Docker volumes. Docker volumes are the preferred mechanism for persisting data generated by and used by Docker containers.
Here’s how you can run your Python Docker image with a Docker volume:
docker run -v /path/on/host:/app/data my-python-app
This command tells Docker to run the my-python-app
image and mount the /path/on/host
directory from the host machine to the /app/data
directory in the Docker container. Your application can read from and write to the /app/data
directory to persist data.
Using Docker Compose for Multi-Container Applications
If your application consists of multiple services that run in separate containers, Docker Compose can be a useful tool. Docker Compose allows you to define and run multi-container Docker applications.
With Docker Compose, you define your application’s services, networks, and volumes in a docker-compose.yml
file. Then, you can start all the services with a single command: docker-compose up
.
Here’s an example docker-compose.yml
file for a Python web application that uses a Redis database:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
redis:
image: "redis:alpine"
In this file:
- The
web
service is built from the Dockerfile in the current directory. It’s accessible on port 5000. - The
redis
service uses theredis:alpine
image.
With this docker-compose.yml
file, you can start your Python web application and the Redis database with the docker-compose up
command.
In this section, we’ve explored some advanced techniques for creating Python Docker images. By optimizing your Dockerfile, managing dependencies effectively, handling data persistence, and using Docker Compose, you can create efficient, scalable Docker images for your Python applications.
Exploring Alternative Methods for Python Docker Images
While the traditional Dockerfile approach works well for many Python applications, there are alternative methods for creating Python Docker images that you might find beneficial. Let’s explore multi-stage builds, third-party tools like Buildpacks, and cloud-based build services.
Multi-Stage Builds
Multi-stage builds are a powerful feature of Docker that allow you to use multiple FROM
instructions in your Dockerfile. Each FROM
instruction can use a different base image and starts a new stage of the build. You can copy artifacts from one stage to another, leaving behind everything you don’t want in the final image.
Here’s an example of a multi-stage Dockerfile for a Python application:
# First stage: build the application
FROM python:3.7-slim AS build
WORKDIR /app
COPY requirements.txt ./
RUN pip install -r requirements.txt
COPY . .
# Second stage: create the final Docker image
FROM python:3.7-slim
WORKDIR /app
COPY --from=build /app .
CMD ["python", "./your-daemon-or-script.py"]
In this Dockerfile:
- The first stage uses the
python:3.7-slim
base image and builds the Python application. It installs the Python dependencies and copies the application code. - The second stage creates the final Docker image. It copies only the application code from the first stage, leaving behind the dependencies and build tools. This results in a smaller final Docker image.
Third-Party Tools: Buildpacks
Buildpacks are a third-party tool that can automate the process of creating Docker images for your Python applications. They detect your application’s language and dependencies, and then build a Docker image without the need for a Dockerfile.
Here’s how you can use the Pack CLI to create a Python Docker image with Buildpacks:
pack build my-python-app --path . --builder heroku/buildpacks:18
This command tells Pack to build a Docker image named my-python-app
using the application code in the current directory (.
) and the Heroku Buildpacks (heroku/buildpacks:18
).
Creating Python Docker images can sometimes present challenges. You might encounter issues related to image size, build time, security, and compatibility. In this section, we’ll discuss these common issues and provide solutions and workarounds.
Image Size
A common issue is the Docker image size. Large images take longer to build and push to a Docker registry, and they consume significant disk space. To reduce the image size, consider using a slim version of the Python base image, like python:3.7-slim
. These slim images have fewer packages installed, which reduces the image size.
Build Time
Long build times can slow down your development process. To speed up build times, leverage Docker’s caching mechanism. Docker caches the layers of your Docker image, and if you change a layer, Docker only needs to rebuild that layer and the ones after it. Therefore, put the commands that change most frequently towards the end of your Dockerfile.
Security
Docker images can have security vulnerabilities. To mitigate this, regularly update the base images in your Dockerfiles. Also, consider using a tool like Docker Bench or Clair to scan your Docker images for vulnerabilities.
Compatibility Issues
Your Python application might work on your machine but fail when running in a Docker container. This could be due to differences in the operating system, system libraries, or Python version. To avoid compatibility issues, ensure that the environment in your Docker container matches the environment where your Python application will run.
Here’s an example of how you can specify a Python version in your Dockerfile to avoid compatibility issues:
FROM python:3.7-slim
In this Dockerfile, we’re using the python:3.7-slim
base image, which includes Python 3.7. This ensures that our Python application will run with Python 3.7, both on our machine and in the Docker container.
In this section, we’ve discussed common issues you might encounter when creating Python Docker images and how to overcome them. By being aware of these potential pitfalls and knowing how to navigate them, you can create efficient, secure, and compatible Python Docker images.
Docker: A Brief Overview
Before we dive deeper into creating Python Docker images, let’s take a step back and understand the fundamentals of Docker, Docker images, and containers. This will help us appreciate why Docker is such a powerful tool for Python developers.
Docker and Its Importance
Docker is an open-source platform designed to automate the deployment, scaling, and management of applications. It does this by packaging applications into containers. A Docker container is a standalone, executable package that includes everything needed to run an application – the code, runtime, libraries, environment variables, and config files.
One of the key benefits of Docker is its ability to provide a consistent environment. This means that if your Python application works in a Docker container on your machine, it will also work in a Docker container on any other machine. This eliminates the infamous ‘it works on my machine’ problem.
Understanding Docker Images
A Docker image is a lightweight, standalone, executable software package that includes everything needed to run a piece of software, including the code, a runtime, libraries, environment variables, and config files.
Docker images are read-only templates used to create Docker containers. When you run a Docker image, Docker creates a Docker container from that image.
Docker and Python: A Powerful Combination
When it comes to Python applications, Docker offers several advantages. First, Docker allows you to manage Python dependencies in an isolated environment. This means you can have different versions of Python and its libraries for different applications, without any conflicts.
Second, Docker makes it easy to deploy Python applications. You can package your application and its dependencies into a Docker image, and then run that image on any machine with Docker installed. This is much easier than manually installing Python and the necessary dependencies on each machine.
Here’s an example of how you can run a Python script using Docker:
docker run -v $(pwd):/app -w /app python:3.7 python your-script.py
This command tells Docker to run the python:3.7
image with the current directory ($(pwd)
) mounted as /app
in the Docker container, and the working directory (-w
) set to /app
. The python your-script.py
command is executed inside the Docker container.
# Output:
# [Expected output from your-script.py]
In this command, Docker takes care of setting up Python 3.7 and executing your script. You don’t need to worry about installing Python or any dependencies on your machine.
In summary, Docker offers a consistent, isolated environment for running Python applications, making it an invaluable tool for Python developers.
Docker’s Role in Modern Python Development
Docker has become an integral part of modern Python development, playing a crucial role in areas such as Continuous Integration/Continuous Deployment (CI/CD), microservices, and cloud deployment. Let’s explore these areas in more detail.
Docker in CI/CD Pipelines
In CI/CD pipelines, Docker can be used to create reproducible build environments. This ensures that the application is built and tested in the same environment every time, eliminating the ‘it works on my machine’ problem.
Here’s an example of how you can use Docker in a CI/CD pipeline:
docker build -t my-python-app .
docker run my-python-app pytest
In this example, we’re building a Docker image for our Python application and then running our tests inside a Docker container.
# Output:
# [Expected output from pytest]
Docker and Microservices
Docker is also a key player in the world of microservices. Microservices are a design pattern where an application is split into smaller, independent services. Each microservice runs in its own Docker container, making it easy to scale and update each service independently.
Docker and Cloud Deployment
When it comes to deploying Python applications to the cloud, Docker can simplify the process. Most cloud providers support Docker, so you can package your application into a Docker image and run it on any cloud.
Exploring Related Concepts: Kubernetes and Docker Swarm
If you’re interested in Docker, you might also want to explore related technologies like Kubernetes and Docker Swarm. Both are container orchestration tools that can manage and scale your Docker containers.
Kubernetes is a powerful, open-source container orchestration system that can manage your Docker containers and services. Docker Swarm is Docker’s own native container orchestration platform. Both have their strengths and weaknesses, and the choice between the two often depends on your specific needs.
Further Resources for Docker and Python Mastery
To deepen your understanding of Docker and Python, consider exploring the following resources:
- This blog post explains how to install Python 3 in Ubuntu. Learn how to check your Python installation and troubleshoot any issues.
Managing Python Versions with pyenv – A tutorial that explores the power of pyenv for managing multiple Python versions on your system.
Exploring Interactive Python (IPython) – A complete guide about the enhanced interactive Python shell, IPython, and its advanced features.
Docker’s official documentation is a comprehensive resource for learning Docker.
The Docker Handbook from freeCodeCamp is a great guide for beginners.
Real Python has several tutorials on using Docker with Python.
These resources can help you master the art of creating Python Docker images and using Docker in your Python development workflow.
Wrapping Up: Mastering Python Docker Images
In this comprehensive guide, we’ve delved into the process of creating Python Docker images, a fundamental skill in modern software development.
We began with the basics, understanding the structure of a Dockerfile and how to write one for a Python application. We then delved into more advanced territory, optimizing our Dockerfile, managing dependencies, handling data persistence, and using Docker Compose for multi-container applications.
We also discussed common challenges you might encounter when creating Python Docker images, such as image size, build time, security, and compatibility issues, providing you with solutions and workarounds for each issue.
We didn’t stop there. We explored alternative methods for creating Python Docker images, such as multi-stage builds, third-party tools like Buildpacks, and cloud-based build services. These alternative methods can offer additional benefits, depending on your specific needs and the complexity of your Python application.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Traditional Dockerfile | Simple, direct control over image | Can result in large images if not carefully managed |
Multi-Stage Builds | Reduces image size | Slightly more complex Dockerfile |
Buildpacks | Automates image creation | Less control over image |
Cloud-Based Build Services | Integrates with CI/CD pipelines | Requires cloud provider account |
Whether you’re a beginner just starting out with Docker or an experienced developer looking to level up your Docker skills, we hope this guide has given you a deeper understanding of how to create Python Docker images. With this knowledge in hand, you’re well-equipped to package your Python applications into Docker images, ready for deployment anywhere. Happy coding!