IPython: Interactive Python Interpreter Guide
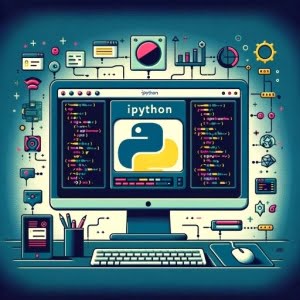
Frustrated with the limitations of the standard Python interpreter? You’re not alone. Many developers find themselves seeking a more powerful tool to enhance their Python coding experience. But, imagine a Python interpreter on steroids – that’s IPython for you.
IPython, an interactive Python interpreter, offers a host of features that can turbo-charge your Python coding workflow. From syntax highlighting to tab completion, IPython is designed to streamline your coding process and make it more efficient.
In this guide, we’ll walk you through the basics to the advanced features of IPython. We’ll explore everything from installation, starting an IPython session, to using its advanced features like the debugger and profiling code. We’ll also discuss common issues and their solutions.
Let’s dive in and start mastering IPython!
TL;DR: What is IPython and How Do I Use It?
IPython is an interactive command-line terminal for Python. It enhances your Python coding experience with features like syntax highlighting, tab completion, and more. Here’s a simple example of using IPython:
$ ipython
In [1]: print('Hello, IPython!')
# Output:
# Hello, IPython!
In this example, we start an IPython session by typing ipython
in the command line. The In [1]:
prompt indicates that IPython is ready for your input. We then use the print()
function to output ‘Hello, IPython!’.
This is just a glimpse of what IPython can do. Continue reading to explore the powerful features of IPython and how to leverage them to turbo-charge your Python coding experience.
Table of Contents
- Getting Started with IPython
- Advanced IPython Features
- Exploring Alternatives to IPython
- IPython Troubleshooting: Common Issues and Solutions
- Understanding Python Interpreters and the REPL Environment
- IPython: Facilitating Larger Projects and Data Analysis
- Wrapping Up: Mastering IPython for Enhanced Python Development
Getting Started with IPython
Before we dive into the powerful features of IPython, let’s start with the basics: installing and starting an IPython session.
Installing IPython
To install IPython, you can use pip, the Python package installer. Open your terminal and type the following command:
pip install ipython
After successful installation, you can start an IPython session.
Starting an IPython Session
To start an IPython session, simply type ipython
in your terminal:
ipython
Your terminal will then display something like this:
Python 3.8.5 (default, Jan 27 2021, 15:41:15)
Type 'copyright', 'credits' or 'license' for more information
IPython 7.20.0 -- An enhanced Interactive Python. Type '?' for help.
In [1]:
The In [1]:
prompt indicates that IPython is ready for your input.
Basic Features of IPython
Now that you’ve started an IPython session, let’s explore its basic features.
Syntax Highlighting
Unlike the standard Python interpreter, IPython supports syntax highlighting. This makes your code easier to read and understand.
Tab Completion
IPython also supports tab completion, which can save you a lot of typing. For instance, if you want to type print
, you can just type pr
and then press Tab
, IPython will complete the rest for you.
Magic Commands
One of the most powerful features of IPython is its magic commands. These are special commands that start with a %
symbol and allow you to perform advanced tasks. For example, the %run
magic command allows you to run a Python script from within IPython.
Here’s an example:
%run my_script.py
# Output:
# [The output of your script]
In this example, we use the %run
magic command to run a Python script named my_script.py
. The output of the script is then displayed in the IPython session.
Advanced IPython Features
After mastering the basics of IPython, it’s time to explore its more advanced features. These include using the debugger, profiling code, and employing IPython notebooks.
Debugging with IPython
IPython has a built-in debugger that can help you track down errors in your code. To use the debugger, you can use the %debug
magic command after an exception has been raised. Here’s an example:
def divide(x, y):
return x / y
divide(1, 0)
# Output:
# ZeroDivisionError: division by zero
%debug
# Output:
# > <ipython-input-2-6f8f47a00332>(2)divide()
# ----> 1 def divide(x, y):
# 2 return x / y
# ipdb>
In this example, we first define a function divide
that divides two numbers. We then call this function with 1
and 0
as arguments, which raises a ZeroDivisionError
. By typing %debug
, we can enter the debugging mode and inspect what caused the error.
Profiling Code
IPython also allows you to profile your code to find bottlenecks. You can use the %timeit
magic command to measure the execution time of your code. Here’s an example:
%timeit [n ** 2 for n in range(1000)]
# Output:
# 1000 loops, best of 5: 277 µs per loop
In this example, we use %timeit
to measure the time it takes to square each number in a range of 1000. The output tells us that it takes about 277 microseconds per loop on average.
Using IPython Notebooks
IPython notebooks, also known as Jupyter notebooks, are a great way to write and share your Python code. They allow you to combine code, text, and multimedia in a single document. You can start a new notebook by typing jupyter notebook
in your terminal.
Here’s an example of how to use a notebook to run some Python code:
# In a Jupyter notebook cell:
print('Hello, IPython notebook!')
# Output:
# Hello, IPython notebook!
In this example, we create a new cell in a Jupyter notebook and use the print()
function to output ‘Hello, IPython notebook!’. The output is then displayed below the cell.
Exploring Alternatives to IPython
While IPython is a powerful tool for Python development, there are other Python interpreters and Integrated Development Environments (IDEs) that you might want to consider. Let’s compare IPython with Jupyter, PyCharm, and the standard Python interpreter.
Jupyter: Interactive Python Notebooks
Jupyter, formerly known as IPython Notebook, is an open-source web application that allows you to create and share documents that contain live code, equations, visualizations, and narrative text. It’s great for data cleaning and transformation, numerical simulation, statistical modeling, data visualization, and machine learning.
# In a Jupyter notebook cell:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4])
plt.ylabel('Numbers')
plt.show()
# Output:
# [A line graph is displayed in the Jupyter notebook]
In this example, we use a Jupyter notebook to create a simple line graph using the matplotlib library. The graph is then displayed directly in the notebook.
PyCharm: A Robust Python IDE
PyCharm is a popular IDE for Python development. It offers features like intelligent code completion, on-the-fly error checking and quick-fixes, easy project navigation, and more.
# In the terminal:
pip install pycharm
# Output:
# Successfully installed pycharm-2021.2.3
In this example, we install PyCharm using pip. After installation, you can start PyCharm and create a new Python project.
Standard Python Interpreter: The Classic Approach
The standard Python interpreter is the original and most straightforward way to run Python code. It doesn’t have the advanced features of IPython, Jupyter, or PyCharm, but it’s simple and reliable.
# In the terminal:
python
# Output:
# Python 3.8.5 (default, Jan 27 2021, 15:41:15)
# >>>
In this example, we start a session with the standard Python interpreter by typing python
in the terminal. The >>>
prompt indicates that the interpreter is ready for your input.
Each of these tools has its pros and cons. IPython offers advanced features like syntax highlighting, tab completion, and magic commands, but it’s a command-line tool and lacks a graphical user interface. Jupyter allows you to combine code, text, and multimedia in a single document, but it’s more suited for data analysis than general Python development. PyCharm offers a full-featured IDE with intelligent code completion and other productivity features, but it might be overkill for simple scripts. The standard Python interpreter is simple and reliable, but it lacks the advanced features of the other tools.
IPython Troubleshooting: Common Issues and Solutions
While IPython is a powerful tool, you may encounter some issues while using it. Let’s discuss some common problems and how to solve them.
Installation Problems
If you’re having trouble installing IPython, make sure you have the latest version of pip installed. You can upgrade pip using the following command:
pip install --upgrade pip
After upgrading pip, you should be able to install IPython without any issues.
Issues with Specific Features
Sometimes, you might encounter issues with specific features of IPython. For example, if the tab completion feature isn’t working, you might need to install the readline library:
pip install readline
After installing readline, the tab completion feature should work as expected.
Solving Common Issues
If you’re facing other issues with IPython, the following tips might help:
- Check your Python version: IPython requires Python 3.7 or later. You can check your Python version using the
python --version
command. Update IPython: If you’re using an old version of IPython, updating it might solve your problem. You can update IPython using the
pip install --upgrade ipython
command.Consult the documentation: The IPython documentation is a great resource for troubleshooting. It provides detailed information about IPython’s features and how to use them.
By understanding these common issues and their solutions, you can ensure a smooth and productive IPython experience.
Understanding Python Interpreters and the REPL Environment
To fully appreciate the power of IPython, we first need to understand what a Python interpreter is and what the REPL environment entails.
The Role of a Python Interpreter
A Python interpreter is a console that allows you to execute Python code line by line. It reads and interprets the Python code into a language that your computer can understand.
# Standard Python Interpreter
python
# Output:
# Python 3.8.5 (default, Jan 27 2021, 15:41:15)
# >>>
In this example, we start a session with the standard Python interpreter by typing python
in the terminal. The >>>
prompt indicates that the interpreter is ready for your input.
The REPL Environment
REPL stands for Read-Eval-Print Loop. It’s an environment that reads the code, evaluates it, prints the result, and then loops back to read the next line of code. This interactive environment is what makes Python a great language for beginners and for experimenting with new ideas.
How IPython Enhances the Standard Python Interpreter
While the standard Python interpreter is powerful, IPython takes it a step further by adding a host of features that make the coding process more interactive and efficient.
- Syntax Highlighting: IPython adds color to your code based on its syntax. This makes your code easier to read and understand.
- Tab Completion: IPython allows you to autocomplete your code by pressing the
Tab
key. This not only saves you typing time but also helps prevent typos. - Magic Commands: IPython introduces magic commands, which are special commands that start with a
%
symbol and allow you to perform advanced tasks.
With these enhancements, IPython becomes a powerful tool that boosts your productivity and makes your Python coding experience more enjoyable.
IPython: Facilitating Larger Projects and Data Analysis
IPython isn’t just a tool for writing Python code more efficiently. It’s also a powerful platform that can facilitate larger projects and complex data analysis tasks.
IPython in Large-Scale Projects
In larger projects, IPython’s advanced features like debugging and profiling can be extremely helpful. They allow you to track down errors and performance bottlenecks, which can be crucial in large-scale projects.
# Debugging in IPython
%debug
# Profiling in IPython
%timeit [n ** 2 for n in range(1000)]
# Output:
# 1000 loops, best of 5: 277 µs per loop
In this example, we use the %debug
magic command to debug our code and the %timeit
magic command to profile a list comprehension operation. These features can help us ensure that our code is correct and efficient.
IPython for Data Analysis and Machine Learning
IPython is also a fantastic tool for data analysis and machine learning. Its support for interactive computing and its integration with data science tools like NumPy, pandas, and matplotlib make it a great choice for these tasks.
# Using pandas in IPython
import pandas as pd
# Create a data frame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df)
# Output:
# A B
# 0 1 4
# 1 2 5
# 2 3 6
In this example, we use pandas, a popular data analysis library, to create a data frame in IPython. This is a simple example of how you can use IPython for data analysis.
Exploring Jupyter Notebooks and Python Libraries
If you’re interested in interactive computing and data analysis, you might want to explore related concepts like Jupyter notebooks and Python libraries for data analysis. Jupyter notebooks allow you to create and share documents that contain live code, equations, visualizations, and narrative text. Python libraries like NumPy, pandas, and matplotlib provide powerful tools for numerical computing and data visualization.
Further Resources for Expanding Your IPython Skills
If you’re interested in learning more about IPython and its applications, here are a few resources that you might find helpful:
- A Comprehensive Tutorial on how to install Python on Ubuntu and simplify your development environment setup.
Dockerizing Your Python Application – Dive into the world of containerization and simplify Python application deployment with Docker.
Making Your Python Environment Active – A quick guide on the art of seamlessly activating virtual environments in Python.
IPython’s Official Documentation – A comprehensive guide to IPython and its features.
Python Data Science Handbook – A book by Jake VanderPlas that covers IPython, NumPy, pandas, matplotlib, and other data science tools.
DataCamp’s IPython Or Jupyter? – A tutorial that introduces IPython and Jupyter notebooks.
By exploring these resources and practicing your skills, you can become a master of IPython and leverage its power in your Python projects.
Wrapping Up: Mastering IPython for Enhanced Python Development
In this comprehensive guide, we’ve delved into the world of IPython, an interactive Python interpreter that turbo-charges your Python coding experience.
We began with the basics, introducing IPython and its installation process. We then explored its basic features, such as syntax highlighting, tab completion, and magic commands. As we ventured into more advanced territory, we uncovered the power of IPython’s debugger, its ability to profile code, and the use of IPython notebooks. We also tackled common issues you might encounter when using IPython, providing solutions and tips to ensure a smooth coding experience.
Alongside IPython, we also discussed alternative Python interpreters and IDEs, such as Jupyter, PyCharm, and the standard Python interpreter. Here’s a quick comparison of these tools:
Tool | Pros | Cons |
---|---|---|
IPython | Advanced features, Interactive | Command-line tool |
Jupyter | Combines code, text, multimedia | Suited for data analysis |
PyCharm | Full-featured IDE, Intelligent code completion | Might be overkill for simple scripts |
Standard Python Interpreter | Simple and reliable | Lacks advanced features |
Whether you’re a Python newbie or a seasoned developer looking to boost your productivity, we hope this guide has given you a deeper understanding of IPython and its capabilities.
With its balance of advanced features and interactivity, IPython is a powerful tool that can enhance your Python development experience. Happy coding!