Java Switch Statement: Guide to Multiple Conditions
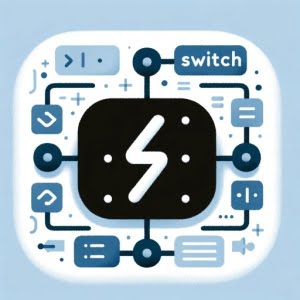
Ever felt like you’re wrestling with understanding the switch statement in Java? You’re not alone. Many developers find the concept of switch statements a bit daunting. Think of the switch statement in Java as a traffic controller – directing the flow of your code based on specific conditions.
Switch statements are a powerful way to control the flow of your code, making them extremely popular for handling multiple conditions in a more readable and efficient way compared to if-else statements.
In this guide, we’ll walk you through the process of using switch statements in Java, from the basics to more advanced techniques. We’ll cover everything from understanding the basic syntax, handling different types of switch cases, to troubleshooting common issues.
Let’s dive in and start mastering the Java switch statement!
TL;DR: How Do I Use the Switch Statement in Java?
The switch statement in Java is used to select one of many code blocks to be executed:
switch (variableToBeSwitched)
. It’s a powerful tool for controlling the flow of your code, especially when you have multiple conditions to handle.
Here’s a simple example:
int day = 3;
switch (day) {
case 1:
System.out.println('Monday');
break;
case 2:
System.out.println('Tuesday');
break;
// ...
}
# Output:
# 'Tuesday'
In this example, we have a switch statement that checks the value of the variable day
. Depending on the value, it executes a different code block. If day
is 1, it prints ‘Monday’. If day
is 2, it prints ‘Tuesday’. In our case, day
is 3, so none of the cases match and nothing is printed.
This is just a basic way to use the switch statement in Java, but there’s much more to learn about controlling the flow of your code. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Decoding the Basic Syntax of Java Switch Statement
- Leveraging Switch Statements: Beyond the Basics
- Exploring Alternatives to Java Switch Statement
- Common Pitfalls and Best Practices with Java Switch Statement
- Understanding Control Flow Statements in Java
- Applying Java Switch Statement in Real-World Projects
- Wrapping Up: Mastering Java Switch Statement
Decoding the Basic Syntax of Java Switch Statement
The switch statement in Java is a multiway branch statement. It provides an easy way to dispatch execution to different parts of your code based on the value of an expression. Here’s the basic syntax:
switch (expression) {
case value1:
// code to be executed if expression equals value1;
break;
case value2:
// code to be executed if expression equals value2;
break;
// you can have any number of case statements.
default:
// code to be executed if expression doesn't match any cases;
}
The switch
keyword is followed by an expression in parentheses (this can be a variable or a calculated value). The result of this expression is then compared with the values for each case
in the structure. If there’s a match, the block of code associated with that case
is executed.
The break
keyword is used after each case to terminate the statement sequence. If break
is not used, all cases after the correct one (including the default
case) are executed until a break
is encountered.
Let’s look at a practical example:
int num = 2;
switch (num) {
case 1:
System.out.println('One');
break;
case 2:
System.out.println('Two');
break;
default:
System.out.println('Not one or two');
}
# Output:
# 'Two'
In this example, the switch expression is num
, which has a value of 2. The code block under case 2
is executed, and ‘Two’ is printed. If num
were not 1 or 2, the default
case would be executed, and ‘Not one or two’ would be printed.
Leveraging Switch Statements: Beyond the Basics
As you become more comfortable with the basic usage of the switch statement in Java, you can start to explore some of its more advanced applications. Two such applications include nested switch statements and using switch with string variables. Let’s dive into these topics.
Nested Switch Statements
Just like you can nest if-else statements, you can also nest switch statements. This means you can have a switch statement inside another switch statement. Here’s how you can do it:
char outer = 'A';
char inner = 'B';
switch(outer) {
case 'A':
System.out.println('Outer is A');
switch(inner) {
case 'B':
System.out.println('Inner is B');
break;
}
break;
case 'B':
System.out.println('Outer is B');
break;
}
# Output:
# 'Outer is A'
# 'Inner is B'
In this example, we have an outer switch statement that checks the value of outer
. Inside the case where outer
is ‘A’, we have another switch statement that checks the value of inner
. This allows us to handle more complex conditions.
Using Switch with String Variables
Starting from Java 7, you can use switch statements with string variables. This can be very useful when you want to perform different actions based on the value of a string. Here’s an example:
String day = 'Monday';
switch(day) {
case 'Monday':
System.out.println('Start of the work week');
break;
case 'Friday':
System.out.println('End of the work week');
break;
default:
System.out.println('Middle of the work week');
}
# Output:
# 'Start of the work week'
In this example, the switch statement checks the value of the string day
. Depending on the value, it prints a different message. This is a powerful feature that can greatly simplify your code when dealing with string conditions.
Exploring Alternatives to Java Switch Statement
While the switch statement is a powerful tool for controlling the flow of your code, Java provides other control flow statements that can be used as alternatives. Two of these are if-else statements and the ternary operator. Let’s explore these alternatives and compare their benefits and drawbacks to the switch statement.
If-Else Statements
The if-else statement is the most basic way to control the flow of your code. It’s more verbose than the switch statement, but it can handle more complex conditions. Here’s an example:
int num = 2;
if (num == 1) {
System.out.println('One');
} else if (num == 2) {
System.out.println('Two');
} else {
System.out.println('Not one or two');
}
# Output:
# 'Two'
This code does the same thing as our previous switch statement example, but it uses if-else statements instead. If-else statements are more flexible than switch statements because they can handle conditions that are not just equality checks. However, they can become unwieldy when there are many conditions to check.
Ternary Operator
The ternary operator is a shorthand way of writing an if-else statement. It’s less readable than both if-else and switch statements, but it can be very concise. Here’s an example:
int num = 2;
String result = (num == 1) ? 'One' : (num == 2) ? 'Two' : 'Not one or two';
System.out.println(result);
# Output:
# 'Two'
In this example, we use the ternary operator to check the value of num
and assign a different string to result
based on that value. The ternary operator can be very useful for simple conditions, but it can become difficult to read with more complex conditions.
In conclusion, while the switch statement is a powerful tool for controlling the flow of your code, there are other options available in Java. Depending on your specific needs, you might find that if-else statements or the ternary operator are more suitable.
Common Pitfalls and Best Practices with Java Switch Statement
While the switch statement in Java is a powerful tool, it can also be a source of bugs if not used correctly. Let’s discuss some of the common issues you might encounter when using the switch statement and how to solve them.
Forgetting the Break Keyword
One of the most common mistakes when using the switch statement is forgetting to include the break
keyword at the end of each case. When this happens, Java will continue executing the next case(s) until it encounters a break
statement. This is known as ‘fall through’.
Here’s an example:
int num = 1;
switch (num) {
case 1:
System.out.println('One');
case 2:
System.out.println('Two');
break;
default:
System.out.println('Not one or two');
}
# Output:
# 'One'
# 'Two'
In this example, we forgot to include a break
statement after the first case. As a result, both ‘One’ and ‘Two’ are printed, even though num
is 1. To avoid this issue, always remember to include a break
statement at the end of each case.
No Default Case
Another common issue is forgetting to include a default case in your switch statement. The default case is executed when none of the other cases match the switch expression. If you don’t include a default case, your switch statement might not do anything if none of the cases match.
Here’s an example:
int num = 3;
switch (num) {
case 1:
System.out.println('One');
break;
case 2:
System.out.println('Two');
break;
}
# Output:
# (nothing)
In this example, num
is 3, which doesn’t match any of the cases in our switch statement. Because we didn’t include a default case, nothing is printed. To avoid this issue, always include a default case in your switch statement.
In conclusion, while the switch statement in Java is a powerful tool, it’s important to use it correctly. By being aware of these common issues and following best practices, you can avoid bugs and write more robust code.
Understanding Control Flow Statements in Java
Before we delve deeper into the specifics of the switch statement, it’s essential to grasp the concept of control flow statements in Java. Control flow statements, as the name suggests, control the order in which the code statements are executed. These are the building blocks that allow Java (and indeed, any programming language) to make decisions and carry out repetitive tasks.
In Java, control flow statements are divided into three categories: decision-making statements (like if, if-else, and switch), looping statements (like for, while, and do-while), and branching statements (like break, continue, and return).
The switch statement falls under decision-making statements, allowing your program to choose different paths of execution based on an expression’s outcome.
Grasping the Concept of ‘Fall Through’ in Switch Statements
A unique characteristic of the switch statement in Java is the ‘fall through’ behavior. When a match is found in the case values, and there’s no break statement to terminate the current case, Java will execute all subsequent cases until it encounters a break statement or reaches the end of the switch block.
Here’s an example to illustrate this:
int num = 1;
switch (num) {
case 1:
System.out.println('One');
case 2:
System.out.println('Two');
break;
default:
System.out.println('Not one or two');
}
# Output:
# 'One'
# 'Two'
In this example, num
is 1, which matches the first case. However, because there’s no break statement after the first case, Java ‘falls through’ to the second case and executes that as well, resulting in both ‘One’ and ‘Two’ being printed.
Understanding this ‘fall through’ behavior is crucial when working with switch statements in Java, as it can lead to unexpected results if not handled correctly.
Applying Java Switch Statement in Real-World Projects
As we’ve seen, the switch statement is a powerful tool for controlling the flow of your code. But how does it apply to larger, real-world projects? One common use case is creating a simple menu system.
Building a Simple Menu System with Java Switch
Let’s say you’re building a text-based game, and you want to present the player with a list of options. You could use a switch statement to handle the player’s input and execute different code based on their choice. Here’s a simple example:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println('Choose an option:
1. Start game
2. Load game
3. Exit');
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println('Starting game...');
// code to start a new game
break;
case 2:
System.out.println('Loading game...');
// code to load a saved game
break;
case 3:
System.out.println('Exiting...');
// code to exit the game
break;
default:
System.out.println('Invalid option');
}
}
}
# Output (assuming the user inputs '1'):
# 'Choose an option:
# 1. Start game
# 2. Load game
# 3. Exit'
# 'Starting game...'
In this example, we present the user with a menu of options and use a switch statement to handle their input. This is a simple but powerful way to use the switch statement in a real-world project.
Further Resources for Mastering Java Switch
Statement are an integral part of Java Control flow. Their usefulness can be enhanced when used along with loops. To learn more on how to utilize loops for controlling the flow of code, you can Click Here.
If you would like to further enhance your understanding and usage of other statements in Java, consider exploring these additional resources:
- Conditions in Java – Learn about conditional statements and expressions in Java for decision-making.
Using If-Else in Java – Master the if-else statement for implementing conditional logic and flow control in Java.
Java Tutorials by Oracle cover all aspects of the language, including control flow statements like the switch statement.
Java switch statement by W3Schools provides a clear and easy-to-digest guide on how to use the ‘switch’ statement in Java.
Java Control Flow: Oracle’s Java Documentation provides a more in-depth look at control flow in Java, including a detailed section on switch statements.
In addition to these resources, consider exploring related topics such as loops in Java and exception handling to broaden your Java programming skills.
Wrapping Up: Mastering Java Switch Statement
In this comprehensive guide, we’ve navigated through the intricacies of the switch statement in Java, shedding light on its syntax, usage, and application in various scenarios.
We began with the basics, understanding the fundamental syntax and structure of the switch statement. We then delved deeper, exploring its advanced usage, including nested switch statements and the use of switch with string variables. Along the journey, we also tackled common pitfalls such as forgetting the break keyword and not including a default case, and provided effective solutions to these challenges.
Moreover, we took a detour to discuss alternative approaches to control flow in Java, such as if-else statements and the ternary operator, and compared their benefits and drawbacks to the switch statement. Here’s a quick comparison of these methods:
Method | Flexibility | Readability | Complexity |
---|---|---|---|
Switch Statement | Moderate | High | Low |
If-Else Statements | High | Moderate | Moderate |
Ternary Operator | Low | Low | High |
Whether you’re a beginner just starting out with Java or an intermediate developer looking to refine your skills, we hope this guide has given you a deeper understanding of the switch statement and its usage in Java.
The switch statement, with its ability to simplify complex condition handling, is a powerful tool in your Java toolkit. Now, with the knowledge acquired from this guide, you’re well equipped to use it effectively in your Java programming journey. Happy coding!