If-Else Java: Mastering Decision Making in Code
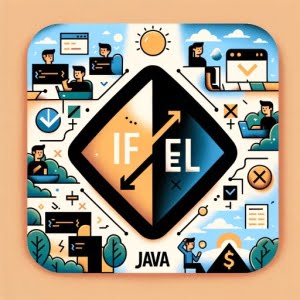
Ever found yourself puzzled on how to make your Java program make decisions? You’re not alone. Many developers find themselves at crossroads when it comes to controlling the flow of their Java programs. Think of the ‘if-else’ statement in Java as a traffic light – it regulates the flow of your program, directing it where to go based on certain conditions.
This guide will take you through the journey of mastering the ‘if-else’ statement in Java, from the basics to more advanced usage. We’ll cover everything from writing your first if-else statement, understanding its flow, to handling more complex scenarios with nested if-else and if-else-if ladder. We’ll also explore alternative approaches to control the flow of a Java program.
So, let’s dive in and start mastering the if-else statement in Java!
TL;DR: How Do I Use the If-Else Statement in Java?
An ‘if-else’ statement is used to perform different actions based on different conditions, defined with the syntax:
if (condition) {// code if condition is true} else {code if condition is false}
It’s like a fork in the road, directing your program based on the conditions you set.
Here’s a simple example:
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
// Output:
// Depends on the condition. If it's true, the first block of code will execute. If it's false, the second block of code (after 'else') will execute.
In this example, the ‘if-else’ statement checks a condition. If the condition is true, it executes the code within the ‘if’ block. If the condition is false, it executes the code within the ‘else’ block. It’s a simple yet powerful way to control the flow of your program.
But there’s so much more to the ‘if-else’ statement in Java. Continue reading for a deeper understanding, more advanced usage scenarios, and alternative approaches.
Table of Contents
Beginner’s Guide to If-Else in Java
The ‘if-else’ statement in Java is a cornerstone of decision-making in your program. It allows you to execute different blocks of code based on specific conditions. Let’s break down how to use it step by step.
Writing Your First If-Else Statement
Here’s a simple example of how to use the ‘if-else’ statement:
int score = 85;
if (score >= 90) {
System.out.println("Excellent!");
} else {
System.out.println("Good job, but there's room for improvement.");
}
// Output:
// 'Good job, but there's room for improvement.'
In this example, we’re checking if the score is greater than or equal to 90. If it is, the program will print ‘Excellent!’, otherwise it will print ‘Good job, but there’s room for improvement.’
Advantages of Using If-Else
The ‘if-else’ statement is a powerful tool for controlling your program’s flow. It allows you to specify different actions for different conditions, making your program more flexible and adaptable.
Pitfalls to Watch Out For
While the ‘if-else’ statement is incredibly useful, it’s important to use it correctly. Be careful not to write conditions that are always true or always false, as this can lead to unexpected behavior. Additionally, remember that the order of your conditions matters. The program will check the conditions in the order they appear, so make sure you’re considering all possible scenarios.
Intermediate-Level If-Else in Java
As you gain more experience with Java, you’ll find situations where a simple ‘if-else’ statement isn’t enough. This is where more complex structures like nested ‘if-else’ and ‘if-else-if’ ladders come in.
Nested If-Else Statements
A nested ‘if-else’ statement is an ‘if-else’ statement within another ‘if-else’ statement. It allows for more complex decision-making in your program.
Here’s an example:
int score = 85;
if (score >= 90) {
System.out.println("Excellent!");
} else {
if (score >= 80) {
System.out.println("Very Good!");
} else {
System.out.println("Good job, but there's room for improvement.");
}
}
// Output:
// 'Very Good!'
In this example, if the score is not greater than or equal to 90, the program checks another condition: if the score is greater than or equal to 80. If it is, it prints ‘Very Good!’. If not, it prints ‘Good job, but there’s room for improvement.’
If-Else-If Ladder
An ‘if-else-if’ ladder is a series of conditions checked one after the other. It’s useful when you have multiple conditions to check.
Here’s an example:
int score = 85;
if (score >= 90) {
System.out.println("Excellent!");
} else if (score >= 80) {
System.out.println("Very Good!");
} else {
System.out.println("Good job, but there's room for improvement.");
}
// Output:
// 'Very Good!'
In this example, the program checks the conditions in order. If the score is not greater than or equal to 90, it checks if the score is greater than or equal to 80. If it is, it prints ‘Very Good!’. If not, it prints ‘Good job, but there’s room for improvement.’
Exploring Alternatives to If-Else in Java
While ‘if-else’ statements are a fundamental part of controlling flow in Java, they aren’t the only tool at your disposal. Another powerful tool is the ‘switch’ statement.
The Power of Switch Statements
The ‘switch’ statement in Java allows you to select one of many code blocks to be executed. It’s a more readable and efficient alternative to the ‘if-else-if’ ladder when dealing with multiple conditions.
Here’s an example:
int day = 3;
String dayOfWeek;
switch (day) {
case 1:
dayOfWeek = "Monday";
break;
case 2:
dayOfWeek = "Tuesday";
break;
case 3:
dayOfWeek = "Wednesday";
break;
// ...and so on for the rest of the week
default:
dayOfWeek = "Invalid day";
}
System.out.println(dayOfWeek);
// Output:
// 'Wednesday'
In this example, the ‘switch’ statement checks the value of ‘day’. Depending on the value, it assigns a different string to ‘dayOfWeek’. If ‘day’ is not a value between 1 and 7, it assigns ‘Invalid day’ to ‘dayOfWeek’.
Advantages and Drawbacks of Switch Statements
Switch statements can make your code cleaner and easier to read when dealing with multiple conditions. However, they are less flexible than ‘if-else’ statements. They can only be used with discrete values and cannot handle ranges or complex conditions.
Making the Right Choice
When deciding whether to use ‘if-else’ or ‘switch’, consider the complexity of your conditions. If you’re dealing with ranges or complex conditions, ‘if-else’ is the way to go. If you’re dealing with multiple discrete values, consider using ‘switch’ for cleaner, more readable code.
Troubleshooting Java If-Else Statements
Like any tool, ‘if-else’ statements in Java can present challenges, especially when used in complex ways. Let’s look at some common issues and their solutions.
Dealing with Syntax Errors
One of the most common issues when using ‘if-else’ statements is syntax errors. These can occur if you forget a bracket, misspell a keyword, or make other typographical errors. For example:
if (score >= 90)
System.out.println("Excellent!"
} else {
System.out.println("Good job, but there's room for improvement.");
}
// Output:
// Syntax error on token "else", delete this token
In this case, the opening bracket after the ‘if’ statement is missing, causing a syntax error. The solution is to correct the syntax:
if (score >= 90) {
System.out.println("Excellent!");
} else {
System.out.println("Good job, but there's room for improvement.");
}
Handling Logical Errors
Another common issue is logical errors, where the code runs without errors, but doesn’t produce the expected result. This usually happens due to incorrect conditions in the ‘if-else’ statement.
For example, consider this code:
int score = 85;
if (score <= 90) {
System.out.println("Excellent!");
} else {
System.out.println("Good job, but there's room for improvement.");
}
// Output:
// 'Excellent!'
Even though the score is 85, the program prints ‘Excellent!’ because the condition checks if the score is less than or equal to 90, not greater than or equal to. The solution is to correct the condition:
if (score >= 90) {
System.out.println("Excellent!");
} else {
System.out.println("Good job, but there's room for improvement.");
}
// Output:
// 'Good job, but there's room for improvement.'
These are just a few examples of the challenges you might face when using ‘if-else’ statements in Java. Remember, the key to effective troubleshooting is understanding the problem, reading error messages carefully, and knowing how ‘if-else’ statements work.
Control Flow: The Heart of Programming
Control flow is a fundamental concept in programming. It’s the order in which your program executes instructions. In Java, control flow is managed using conditional statements like ‘if-else’, loops, and other constructs.
The Role of If-Else in Control Flow
The ‘if-else’ statement is a conditional statement that plays a pivotal role in control flow. It allows your program to choose a path of execution based on whether a condition is true or false.
Here’s a simple ‘if-else’ statement:
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
// Output:
// Depends on the condition. If it's true, the first block of code will execute. If it's false, the second block of code (after 'else') will execute.
In this example, the program checks the condition. If it’s true, it executes the code within the ‘if’ block. If it’s false, it executes the code within the ‘else’ block.
Understanding Conditional Statements
Conditional statements are the building blocks of control flow. They allow your program to make decisions, repeat actions, and handle complex logic. The ‘if-else’ statement is one of the most common conditional statements, but there are others, like ‘switch’ and ‘for’, each with its unique use cases.
By mastering ‘if-else’ and other conditional statements, you’ll be able to write Java programs that can handle complex tasks, making you a more effective and versatile developer.
If-Else in Larger Java Projects
The ‘if-else’ statement isn’t just for simple programs or exercises. It’s a fundamental tool that you’ll use in almost every Java project, no matter how large or complex.
Integrating If-Else with Loops
In larger projects, you’ll often find ‘if-else’ statements used in conjunction with loops. For example, you might use a ‘for’ or ‘while’ loop to iterate over a collection of items, and an ‘if-else’ statement to perform different actions based on each item.
Here’s an example:
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
System.out.println(i + " is even");
} else {
System.out.println(i + " is odd");
}
}
// Output:
// 0 is even
// 1 is odd
// 2 is even
// 3 is odd
// ...and so on up to 9
In this example, the program uses a ‘for’ loop to iterate over the numbers 0 through 9. For each number, it uses an ‘if-else’ statement to check if the number is even or odd, and prints a message accordingly.
If-Else and Exception Handling
‘If-else’ statements are also often used in exception handling, a crucial part of any Java program. You might use an ‘if-else’ statement to check if a certain condition is met before performing an action that could throw an exception.
Here’s an example:
String str = null;
if (str != null) {
System.out.println(str.length());
} else {
System.out.println("Cannot get the length of a null string");
}
// Output:
// 'Cannot get the length of a null string'
In this example, the program uses an ‘if-else’ statement to check if ‘str’ is not null before trying to get its length. If ‘str’ is null, it prints a warning message instead of throwing a NullPointerException.
Further Resources for Mastering If-Else in Java
If you want to dive deeper into the ‘if-else’ statement in Java and related topics, here are some resources to check out:
- IOFlood’s Complete Guide to Java Loops explores nested loops for complex iteration patterns in Java.
Java Switch Statement – Explore the switch statement in Java for multi-branch decision-making.
Using Else-If in Java – Master the else-if construct for complex decision-making in Java programs.
Java if-else statement by Oracle: Oracle’s official Java tutorials offer exhaustive information on if-else statements.
Java if-else by JavaTpoint explains using if-else conditions in Java, with easy-to-understand examples.
Java if-else statement with examples by GeeksforGeeks includes a variety of examples and use cases.
Wrapping Up: Mastering If-Else Statements in Java
In this comprehensive guide, we’ve explored the ins and outs of ‘if-else’ statements in Java, from the basics to more advanced usage scenarios.
We began with the basics, learning how to use ‘if-else’ statements to control the flow of a Java program. We then delved deeper, tackling more complex structures like nested ‘if-else’ and ‘if-else-if’ ladders. Along the way, we also discussed common issues that you might encounter when using ‘if-else’ statements and provided solutions to these problems.
We didn’t stop at ‘if-else’ statements, though. We also explored alternative approaches to control flow in Java, such as using ‘switch’ statements. We compared these methods, giving you a sense of the broader landscape of tools for managing control flow in Java.
Here’s a quick comparison of the methods we’ve discussed:
Method | Complexity | Flexibility |
---|---|---|
If-Else | Moderate | High |
Nested If-Else | High | High |
If-Else-If Ladder | High | High |
Switch | Moderate | Moderate |
Whether you’re just starting out with Java or you’re looking to level up your control flow skills, we hope this guide has given you a deeper understanding of ‘if-else’ statements and their alternatives.
With a solid grasp of ‘if-else’ statements, you’ll be well-equipped to write Java programs that can handle complex decision-making. Happy coding!