Understanding Loops in Java: Your Complete Guide
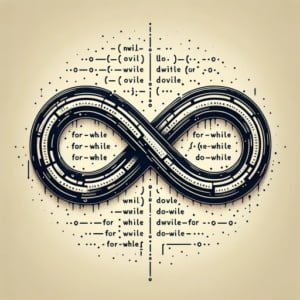
Are you finding it challenging to master loops in Java? You’re not alone. Many developers, especially beginners, find themselves puzzled when it comes to understanding and implementing loops in Java. Think of Java loops as a repeating chorus in a song, allowing you to repeat a block of code until a certain condition is met.
This guide will walk you through the ins and outs of using loops in Java, from the basics to advanced techniques. We’ll cover everything from understanding the basic use of loops, exploring advanced use cases, to discussing alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering loops in Java!
TL;DR: How Do I Use Loops in Java?
Java provides three types of loops:
for, while, and do-while
. Each of these loops can be used to repeat a block of code, but they each have their own unique use cases and syntax.
Here’s a simple example of a for loop:
for(int i = 5; i > 0; i--) {
System.out.println(i);
}
# Output:
# 5
# 4
# 3
# 2
# 1
In this example, we’ve created a for loop that prints the numbers 5 through 1. The loop starts with i
at 5 and continues as long as i
is greater than 0, decrementing i
by 1 after each iteration. The System.out.println(i);
line is the code that gets repeated in each loop, printing the current value of i
.
This is just a basic way to use loops in Java, but there’s much more to learn about using loops effectively and efficiently. Continue reading for more detailed explanations, examples, and advanced techniques.
Table of Contents
Exploring the Basics of Java Loops
In Java, there are three primary types of loops that you’ll encounter: for
, while
, and do-while
loops. Let’s break down each type and understand how they work, their advantages, and potential pitfalls.
The For Loop in Java
The for
loop is perhaps the most commonly used loop in Java. It’s perfect for situations where you know exactly how many times you want to loop through a block of code.
Here’s the basic syntax of a for
loop:
for(initialization; condition; increment/decrement){
// code to be executed
}
Let’s see an example:
for(int i = 0; i < 5; i++) {
System.out.println(i);
}
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the loop starts with i
at 0 (initialization
), continues as long as i
is less than 5 (condition
), and increments i
by 1 after each loop (increment
). The System.out.println(i);
line is the code that gets executed in each iteration, printing the current value of i
.
The While Loop in Java
The while
loop in Java is used when you want to repeat a part of your program several times, but you do not know in advance how many times the repetition should occur.
Here’s the basic syntax of a while
loop:
while(condition){
// code to be executed
}
Let’s see an example:
int i = 0;
while(i < 5) {
System.out.println(i);
i++;
}
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the while
loop continues as long as i
is less than 5, and increments i
by 1 after each loop. The System.out.println(i);
line is the code that gets executed in each iteration, printing the current value of i
.
The Do-While Loop in Java
The do-while
loop is similar to the while
loop, but with a key difference: the do-while
loop will execute the block of code once before checking the condition, then it will repeat the loop as long as the condition is true.
Here’s the basic syntax of a do-while
loop:
do{
// code to be executed
}
while(condition);
Let’s see an example:
int i = 0;
do {
System.out.println(i);
i++;
}
while(i < 5);
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the do-while
loop executes the block of code once, then continues as long as i
is less than 5, incrementing i
by 1 after each loop. The System.out.println(i);
line is the code that gets executed in each iteration, printing the current value of i
.
Each of these loops has its unique use cases and advantages. However, it’s also crucial to be aware of potential pitfalls like infinite loops and understand how to avoid them. We’ll explore these topics and more in the following sections.
Delving Deeper: Advanced Uses of Java Loops
Now that we’ve covered the basics, let’s explore some more complex uses of loops in Java. Specifically, we’ll look at nested loops and loops with break
or continue
statements.
Nested Loops in Java
A nested loop is a loop inside another loop. The inner loop will be executed one time for each iteration of the outer loop. This is particularly useful when you need to work with multi-dimensional arrays or when you need to repeat a sequence of instructions in a loop a certain number of times.
Here’s an example of a nested for
loop that prints a 5×5 matrix:
for(int i = 0; i < 5; i++) {
for(int j = 0; j < 5; j++) {
System.out.print("*");
}
System.out.println();
}
# Output:
# *****
# *****
# *****
# *****
# *****
In this example, the outer loop runs five times, and for each iteration of the outer loop, the inner loop also runs five times, printing a star. After the inner loop completes, the outer loop prints a newline to start a new row.
Break and Continue Statements
Java provides two essential keywords – break
and continue
, which can be used to control the flow of loops.
The break
statement is used to terminate the loop immediately when a certain condition is met.
Here’s an example of a for
loop that uses a break
statement:
for(int i = 0; i < 10; i++) {
if(i == 5) {
break;
}
System.out.println(i);
}
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the for
loop breaks as soon as i
equals 5, so it only prints the numbers 0 through 4.
The continue
statement skips the current iteration of the loop and continues with the next iteration.
Here’s an example of a for
loop that uses a continue
statement:
for(int i = 0; i < 10; i++) {
if(i == 5) {
continue;
}
System.out.println(i);
}
# Output:
# 0
# 1
# 2
# 3
# 4
# 6
# 7
# 8
# 9
In this example, the for
loop skips the iteration where i
equals 5, so it prints all the numbers from 0 to 9, except 5.
Understanding how to use nested loops and control flow statements like break
and continue
can greatly enhance your ability to write efficient and effective Java code. In the next section, we’ll explore some alternative approaches to creating loops in Java.
Exploring Alternative Looping Techniques in Java
While for
, while
, and do-while
loops are the most common looping structures in Java, there are other ways to create loops, especially as you dive into more advanced Java programming. Two such techniques are using the Stream API and recursion.
Looping with Java Stream API
Java’s Stream API, introduced in Java 8, provides a more declarative approach to iterating over collections. It’s a powerful tool that can simplify your code and make it easier to parallelize your operations.
Here’s an example of using the Stream API to iterate over a list:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream().forEach(System.out::println);
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we create a Stream from the list and use the forEach
method to print each element. The System.out::println
part is a method reference, a shorthand notation for a lambda expression that calls a single method.
Recursion as an Alternative to Looping
Recursion, the process in which a method calls itself to solve a problem, can often be used as an alternative to traditional looping structures. It’s particularly useful when you need to traverse data structures like trees or graphs.
Here’s an example of a recursive method that calculates the factorial of a number:
public static int factorial(int n) {
if(n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
System.out.println(factorial(5));
# Output:
# 120
In this example, the factorial
method calls itself with a smaller value until it reaches the base case where n
equals 0. At that point, it starts returning the results back up the call stack, multiplying them together to get the final result.
While these alternative approaches can be powerful, they also have their drawbacks. The Stream API can be less efficient for simple loops, and recursion can lead to stack overflow errors for large inputs. As always, it’s crucial to understand the tools at your disposal and make the right decision based on the specific requirements of your project.
Troubleshooting Common Loop-Related Issues in Java
Like any programming concept, loops in Java can sometimes present challenges or obstacles. However, understanding these common issues and their solutions can help you write more robust and efficient code. Let’s take a look at some of these problems and how to solve them.
Dealing with Infinite Loops
An infinite loop occurs when the condition of the loop is always true. This can happen due to a programming error and can cause your program to hang or consume excessive resources.
Here’s an example of an infinite loop:
// WARNING: This is an infinite loop. Do not run this code.
while(true) {
System.out.println("This is an infinite loop.");
}
In this example, the condition of the while
loop is always true
, so it will keep printing the message indefinitely.
To avoid infinite loops, make sure that the loop condition will eventually become false. Always double-check your conditions and the variables involved in them.
Looping Over Collections: Concurrent Modification Exception
When looping over a collection in Java, you might encounter a ConcurrentModificationException
if you try to modify the collection while iterating over it.
Here’s an example that throws a ConcurrentModificationException
:
List<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5));
for(Integer number : numbers) {
if(number == 3) {
numbers.remove(number);
}
}
# Output:
# Exception in thread "main" java.util.ConcurrentModificationException
In this example, we’re trying to remove an element from the list while we’re iterating over it, which causes a ConcurrentModificationException
.
To avoid this issue, you can use an iterator to safely remove elements during iteration:
Iterator<Integer> iterator = numbers.iterator();
while(iterator.hasNext()) {
Integer number = iterator.next();
if(number == 3) {
iterator.remove();
}
}
In this example, we’re using an Iterator
to loop over the list. When we find the number 3, we call the remove
method on the iterator, which safely removes the current element.
Optimization Considerations
When working with loops in Java, it’s important to consider performance. Unoptimized loops can significantly slow down your program, especially when dealing with large data sets.
For example, when looping over a list, using the get
method to access elements by their index in a LinkedList
can lead to poor performance because each get
operation takes linear time. Instead, use an iterator or a for-each loop, which can access elements in constant time.
Remember, understanding the common issues and best practices related to loops can help you write better, more efficient Java code.
Understanding Control Flow in Java
Control flow is a fundamental concept in programming that refers to the order in which the instructions, or statements, in a program are executed. Loops, as we’ve been discussing, are a critical part of control flow, allowing us to repeat blocks of code.
The Role of Loops in Control Flow
In the context of control flow, loops provide a way to execute a block of code multiple times, which is useful when you need to run a particular operation on each item in a collection, or simply repeat an operation a certain number of times. This is a significant improvement over having to write out each operation individually, and it provides the flexibility to handle dynamic data where the exact number of operations might not be known ahead of time.
Related Control Flow Constructs
While loops are an essential part of control flow in Java, they are just one piece of the puzzle. Other control flow constructs in Java include if-else
statements and switch
statements.
if-else
statements allow your program to choose between two or more blocks of code to execute based on whether certain conditions are true. For example:
int number = 10;
if(number > 0) {
System.out.println("The number is positive.");
} else {
System.out.println("The number is not positive.");
}
# Output:
# The number is positive.
In this example, the program checks if the number is greater than 0. If the condition is true, it prints “The number is positive.” Otherwise, it prints “The number is not positive.”
switch
statements, on the other hand, allow your program to choose between multiple blocks of code to execute based on the value of a variable or expression. For example:
int dayOfWeek = 2;
switch(dayOfWeek) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
// ... other cases ...
}
# Output:
# Tuesday
In this example, the program prints the name of the day of the week based on the value of dayOfWeek
. If dayOfWeek
is 1, it prints “Monday”. If dayOfWeek
is 2, it prints “Tuesday”, and so on.
Understanding how loops, if-else
statements, and switch
statements work together to control the flow of your program is key to writing effective and efficient Java code.
Applying Java Loops in Real-World Projects
Loops in Java, while simple in concept, are incredibly powerful tools when applied in larger programs or projects. They form the backbone of many algorithms and are used extensively in data processing, user interaction, and more.
Java Loops and Data Structures
One common use case for loops is when working with data structures like arrays or lists. For example, you might use a for
loop to iterate over an array and perform some operation on each element:
int[] numbers = {1, 2, 3, 4, 5};
for(int number : numbers) {
System.out.println(number * 2);
}
# Output:
# 2
# 4
# 6
# 8
# 10
In this example, we’re using a for-each loop to double each element in the array and print the result. This is a simple example, but in a real-world application, you might be performing more complex operations or working with larger and more complex data structures.
Looping in Larger Programs
In larger programs, you might find yourself using loops to control the flow of your application, such as in a main event loop in a game or an application server. In these cases, understanding how to control and manage loops effectively is crucial to ensuring that your program runs smoothly and efficiently.
Further Resources for Mastering Java Loops
While this guide provides a comprehensive overview of loops in Java, there’s always more to learn. Here are a few resources that offer more in-depth information about loops and related topics:
- Java Fundamentals – Build a strong foundation in core Java and Control Flow concepts.
While Loop in Java – Learn about the while loop in Java for iterative execution based on a condition
Using If Statement in Java – Master the if statement for implementing branching logic in Java programs.
Oracle’s Java Tutorials: Oracle, the maintainer of Java, provides a wealth of tutorials and documentation on all aspects of Java, including loops.
GeeksforGeeks’ Java Programming Language section offers guides on Java programming, including loops and data structures.
Baeldung’s Guide to Java Loops goes into even more depth on Java loops, including advanced topics and best practices.
Wrapping Up: Mastering Loops in Java
In this comprehensive guide, we’ve navigated through the concept of loops in Java, an essential tool in any programmer’s toolkit. From basic for
, while
, and do-while
loops to more advanced usage, alternative approaches, and troubleshooting common issues, we’ve covered a wide spectrum of knowledge about Java loops.
We began with the basics, learning how to use the three types of loops in Java, each with its unique use cases and syntax. We then delved deeper into more complex uses of loops, including nested loops and loops with break
or continue
statements. We also explored alternative ways to create loops in Java, such as using the Stream API or recursion.
Along the way, we tackled common issues that you might encounter when using loops, such as infinite loops and concurrent modification exceptions, and provided solutions to help you overcome these challenges. We also discussed optimization considerations to ensure that your loops run as efficiently as possible.
Here’s a quick comparison of the loop methods we’ve discussed:
Loop Method | Use Case | Pros | Cons |
---|---|---|---|
for loop | When the number of iterations is known | Simple and straightforward | Not suitable when the number of iterations is unknown |
while loop | When the number of iterations is unknown | Flexible condition checking | Risk of infinite loops if not handled correctly |
do-while loop | When the loop should execute at least once | Executes the loop body before checking the condition | Less commonly used than for and while |
Stream API | Iterating over collections | More declarative and can simplify code | Can be less efficient for simple loops |
Recursion | When a problem can be divided into smaller subproblems | Can simplify complex problems | Risk of stack overflow for large inputs |
Whether you’re a beginner just getting started with Java or an experienced developer looking to deepen your understanding of loops, we hope this guide has been a valuable resource. Loops are a fundamental part of Java programming, and mastering them is key to writing efficient and effective code. Happy coding!