Pip Install Requirements.txt | Python Package Manager
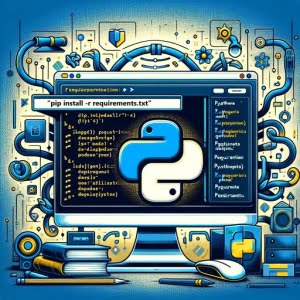
Are you finding it challenging to install multiple Python packages using a requirements.txt file? Stop the struggle and let pip be your personal assistant, swiftly handling package installations from a simple text file.
This comprehensive guide will walk you through the process, from basic use to advanced techniques.
In the next sections, we’ll dive deeper into the workings of pip and requirements.txt, and how you can leverage them to manage your Python packages efficiently.
TL;DR: How Do I Use pip to Install Packages from a requirements.txt File?
Simply use the command
pip install -r requirements.txt
in your terminal. This will install all the packages listed in your requirements.txt file.
Consider this basic example:
# Let's say your requirements.txt file contains the following:
numpy==1.21.2
pandas==1.3.3
# To install these packages, run the following command in your terminal:
pip install -r requirements.txt
# Output:
# Collecting numpy==1.21.2
# Downloading numpy-1.21.2-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.3 MB)
# Collecting pandas==1.3.3
# Downloading pandas-1.3.3-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (11.3 MB)
# Successfully installed numpy-1.21.2 pandas-1.3.3
In this example, pip reads the requirements.txt file and installs the numpy and pandas packages as specified. It’s as simple as that!
This guide will delve into more details, including advanced usage scenarios. So, keep reading to become a pip and requirements.txt maestro!
Table of Contents
Basic Usage of requirements.txt
If you’re new to Python or have never used a requirements.txt file before, this section is for you. We’ll go over a step-by-step guide on using the pip install -r requirements.txt
command, including how to navigate to the correct directory and what to expect during the installation process.
Step 1: Navigate to the Correct Directory
Firstly, you need to navigate to the directory containing your requirements.txt file. You can do this using the ‘cd’ command. Let’s say your requirements.txt file is in a folder named ‘my_project’ on your desktop. You would navigate to it like so:
cd Desktop/my_project
# Output:
# You won't see any output from this command if it's successful. If the directory does not exist, you'll see an error message.
Step 2: Inspect Your requirements.txt File
Your requirements.txt file should list the Python packages you want to install, each on a new line. Here’s a simple example:
# requirements.txt
numpy==1.21.2
pandas==1.3.3
In this example, we’re specifying that we want to install numpy version 1.21.2 and pandas version 1.3.3.
Step 3: Run the pip Install Command
With your terminal pointed to the correct directory, you can now run the pip install -r requirements.txt
command:
pip install -r requirements.txt
# Output:
# Collecting numpy==1.21.2
# Downloading numpy-1.21.2-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.3 MB)
# Collecting pandas==1.3.3
# Downloading pandas-1.3.3-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (11.3 MB)
# Successfully installed numpy-1.21.2 pandas-1.3.3
This command tells pip to install all the packages listed in your requirements.txt file. In this case, it installs numpy 1.21.2 and pandas 1.3.3.
And there you have it! You’ve just installed multiple Python packages using pip install -r requirements.txt
. In the next section, we’ll explore more complex usage scenarios.
Advanced Uses and Pip Installs
As you become more comfortable with using pip and requirements.txt, you can start to leverage more advanced use cases. This includes using pip with different types of requirements.txt files, such as those specifying certain package versions or even installing directly from URLs.
Specifying Package Versions
In a requirements.txt file, you can specify the version of the package you want to install. This is useful when you need to ensure compatibility with your existing code. Here’s an example of a requirements.txt file with specific versions:
# requirements.txt
numpy==1.21.2
pandas==1.3.3
When you run pip install -r requirements.txt
, pip will install the exact versions you specified:
pip install -r requirements.txt
# Output:
# Collecting numpy==1.21.2
# Downloading numpy-1.21.2-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.3 MB)
# Collecting pandas==1.3.3
# Downloading pandas-1.3.3-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (11.3 MB)
# Successfully installed numpy-1.21.2 pandas-1.3.3
Installing Packages from URLs
You can also install packages directly from URLs. This is especially useful when you want to install a version of a package that’s not available on PyPI, or if you want to install a package from a private repository. Here’s an example:
# requirements.txt
https://github.com/username/repository/archive/branch.zip
When you run pip install -r requirements.txt
, pip will download the package from the URL and install it:
pip install -r requirements.txt
# Output:
# Collecting https://github.com/username/repository/archive/branch.zip
# Downloading https://github.com/username/repository/archive/branch.zip
# Requirement already satisfied (use --upgrade to upgrade): package==version from https://github.com/username/repository/archive/branch.zip in /usr/local/lib/python3.7/site-packages
In this example, pip downloads the package from the given URL and installs it. Note that you need to replace ‘username’, ‘repository’, and ‘branch.zip’ with the actual values.
With these advanced techniques, you can make pip and requirements.txt work for any Python package installation scenario. In the next section, we’ll look at some alternative approaches to package management.
Alternative Tools: Pipenv and Docker
While pip install -r requirements.txt
serves as a powerful tool for managing Python packages, it’s not the only game in town. Two other popular alternatives are Pipenv and Docker. Let’s delve into these alternatives and weigh their pros and cons against using pip and requirements.txt.
Embracing Pipenv for Package Management
Pipenv is a packaging tool for Python that solves some common problems associated with the typical workflow using pip, virtualenv, and the good old requirements.txt.
# To install a package using Pipenv, you would use the following command:
pipenv install numpy
# Output:
# Creating a virtualenv for this project...
# Pipfile: /path/to/Pipfile
# Installing numpy...
# Adding numpy to Pipfile's [packages]...
# Installation Succeeded
In this example, Pipenv creates a virtual environment for your project, installs numpy, and adds it to the Pipfile, which is the equivalent of the requirements.txt file in the pip world.
Pipenv’s main advantages are its automatic virtual environment management and its use of the Pipfile, which is easier to read and manage than requirements.txt. However, it’s a bit slower than pip, and some users find its automatic virtual environment management to be more of a hindrance than a help.
Leveraging Docker for Isolated Environments
Docker is another alternative for managing Python packages. It allows you to create isolated environments, called containers, where you can run your Python applications with their dependencies.
# Dockerfile
FROM python:3.7
WORKDIR /app
COPY requirements.txt ./
RUN pip install -r requirements.txt
COPY . .
CMD [ "python", "./your_script.py" ]
In this Dockerfile, Docker creates a new container with Python 3.7, sets the working directory to /app, copies your requirements.txt file and your application into the container, and runs pip install -r requirements.txt
to install the packages. It then runs your_script.py when the container starts.
Docker’s main advantage is its ability to create completely isolated environments, which ensures that your application runs the same way regardless of where the Docker container is deployed. However, Docker has a steeper learning curve than pip or Pipenv, and it can be overkill for simple Python applications.
In conclusion, while pip install -r requirements.txt
is a powerful tool for managing Python packages, alternatives like Pipenv and Docker offer their own unique advantages and could be a better fit depending on your specific needs.
Troubleshooting Common Issues
While pip install -r requirements.txt
is a straightforward way to install Python packages, you may encounter some common issues. Let’s discuss these problems and provide solutions and workarounds.
Dealing with Missing Packages
One of the most common issues is that pip can’t find a package listed in your requirements.txt file. This usually means the package doesn’t exist, or there’s a typo in its name.
pip install -r requirements.txt
# Output:
# ERROR: Could not find a version that satisfies the requirement non_existent_package (from versions: none)
# ERROR: No matching distribution found for non_existent_package
In this example, pip can’t find a package named ‘non_existent_package’. To resolve this issue, check your requirements.txt file for typos or outdated package names.
Resolving Incompatible Versions
Another common issue is incompatible package versions. This can occur when one package requires a certain version of another package, but a different version is listed in your requirements.txt file.
pip install -r requirements.txt
# Output:
# ERROR: Cannot install numpy==1.21.2 and numpy==1.18.5 because these package versions have conflicting dependencies.
In this example, pip cannot install two conflicting versions of numpy. To fix this, you need to determine which version of numpy your project actually needs and update your requirements.txt file accordingly.
Handling Connection Errors
Sometimes, pip might fail to download a package due to a network error.
pip install -r requirements.txt
# Output:
# ERROR: Could not find a version that satisfies the requirement numpy (from versions: none)
# ERROR: No matching distribution found for numpy
In this example, pip fails to find numpy, but it’s likely due to a network issue rather than the package not existing. To resolve this, check your internet connection and try again.
By understanding these common issues and their solutions, you can troubleshoot problems with pip install -r requirements.txt
effectively and keep your Python project on track.
Purpose of Requirements.txt in pip
To fully leverage pip install -r requirements.txt
, it’s crucial to understand the purpose and structure of a requirements.txt file and how pip interacts with it. This knowledge will also shed light on the importance of package management in Python development.
The Purpose and Structure of a Requirements.txt File
A requirements.txt file is a simple text file that lists the Python packages your project depends on. Each line of the file contains the name of a package and optionally the desired version.
# requirements.txt
numpy==1.21.2
pandas==1.3.3
In this example, the requirements.txt file specifies that the project depends on numpy version 1.21.2 and pandas version 1.3.3.
How pip Interacts with Requirements.txt
pip is a package manager for Python. It can install packages from the Python Package Index (PyPI) and other sources. When you run pip install -r requirements.txt
, pip reads the list of packages from the requirements.txt file and installs each one.
pip install -r requirements.txt
# Output:
# Collecting numpy==1.21.2
# Downloading numpy-1.21.2-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.3 MB)
# Collecting pandas==1.3.3
# Downloading pandas-1.3.3-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (11.3 MB)
# Successfully installed numpy-1.21.2 pandas-1.3.3
In this example, pip installs the exact versions of numpy and pandas specified in the requirements.txt file.
The Importance of Package Management in Python Development
Package management is crucial in Python development. It ensures all developers working on a project use the same packages and versions, leading to consistent behavior across different environments. A requirements.txt file, combined with pip, provides a simple yet effective solution for package management in Python.
Management in Large Python Projects
As your Python projects grow in size and complexity, the role of package management becomes increasingly critical. It ensures consistency across different environments and development stages, from local development to production deployment.
Consider a scenario where you’re working on a large project with multiple developers. Without a clear list of required packages (like a requirements.txt file), each developer might end up using different versions of the same package. This can lead to inconsistent behavior and hard-to-debug errors.
By using pip install -r requirements.txt
, you ensure everyone is on the same page. This command installs the same packages with the same versions for every developer, leading to a consistent development environment.
# In a large project, your requirements.txt file might look like this:
numpy==1.21.2
pandas==1.3.3
scikit-learn==1.0
matplotlib==3.5.1
# Installing these packages is as simple as running:
pip install -r requirements.txt
# Output:
# Collecting numpy==1.21.2
# Downloading numpy-1.21.2-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.3 MB)
# Collecting pandas==1.3.3
# Downloading pandas-1.3.3-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (11.3 MB)
# Collecting scikit-learn==1.0
# Downloading scikit_learn-1.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (23.1 MB)
# Collecting matplotlib==3.5.1
# Downloading matplotlib-3.5.1-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (10.7 MB)
# Successfully installed numpy-1.21.2 pandas-1.3.3 scikit-learn-1.0 matplotlib-3.5.1
In this example, pip installs numpy, pandas, scikit-learn, and matplotlib as specified in the requirements.txt file. This ensures that every developer working on the project uses the same versions of these packages.
Improving Development Workflow
While pip install -r requirements.txt
is a great start, there’s much more to learn about Python package management and development workflows. Here are some related topics for further reading:
- Virtual Environments: These create isolated spaces for your Python projects, ensuring that packages installed in one project don’t interfere with another. Tools like venv and virtualenv can help with this.
Continuous Integration (CI): CI is a practice where developers integrate their code into a shared repository frequently, usually multiple times per day. Each integration can then be verified by an automated build and tests. Tools like Jenkins, Travis CI, and CircleCI can help set up CI for your Python projects.
Deployment Strategies: Once your code is ready to move from your local machine to a production environment, you’ll need a deployment strategy. This could involve containerization (like Docker), cloud platforms (like AWS or Google Cloud), or server management tools (like Ansible).
By understanding and implementing these concepts, you can create robust, scalable, and efficient development workflows for your Python projects.
Further Resources for Pip
To learn more about pip and Python package management, check out the following resources:
- A Closer Look at Pip: Installation and Commands: Master the installation and configuration of “pip” on different operating systems.
How-To Install a Specific Python Package Version with pip: Explore the ins and outs of “pip install” to control package versions in Python.
Installing pip for Python: A Beginner’s Guide: Learn how to install “pip” for Python and kickstart your package management journey.
Inedo’s Blog Post on Managing Python Packages walks through the necessary steps to effectively manage Python packages.
Poetry Website: The official Poetry site provides an extensive guide on how this tool helps in Python package and dependency management.
Real Python’s Article on Pip: This in-depth guide covers the ins and outs of pip, Python’s primary package installer.
Recap of pip install requirements.txt
Throughout this guide, we’ve explored how to use pip install -r requirements.txt
to manage Python packages. We’ve seen how it can install packages from a simple text file, making it a powerful tool for Python developers.
We’ve also discussed common issues that can occur when using pip install -r requirements.txt
, such as missing packages, incompatible versions, and connection errors. By understanding these issues and their solutions, you can troubleshoot problems effectively.
Finally, we’ve looked at alternative approaches to package management, such as Pipenv and Docker. Each tool has its own strengths and weaknesses, and the best choice depends on your specific needs.
Tool | Strengths | Weaknesses |
---|---|---|
pip | Simple, easy to use | Can lead to dependency conflicts |
Pipenv | Manages virtual environments, uses the easy-to-read Pipfile | Slower than pip, automatic virtual environment management can be a hindrance |
Docker | Creates completely isolated environments | Steeper learning curve, can be overkill for simple applications |
By understanding these tools and their trade-offs, you can choose the best package management solution for your Python projects.