Python Variable Guide: From Basics to Mastery
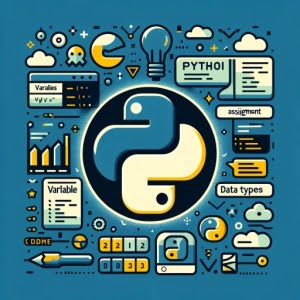
Are you finding it challenging to understand variables in Python? You’re not alone. Many developers, especially beginners, often find themselves puzzled when it comes to understanding and using variables in Python.
Think of Python variables as containers – they store data that your program can use.
In this guide, we’ll walk you through the process of understanding and using variables in Python, from the basics to more advanced techniques. We’ll cover everything from declaring and using variables, different types of variables, to common issues and their solutions.
Let’s dive in and start mastering Python variables!
TL;DR: What is a Variable in Python?
A variable in Python is a reserved memory location to store values. In simpler terms, a variable in a Python program gives data to the computer for processing.
Here’s a simple example:
x = 5
print(x)
# Output:
# 5
In this example, we’ve declared a variable x
and assigned it the value 5
. When we print x
, Python retrieves the value stored in x
and outputs 5
.
This is just the tip of the iceberg when it comes to Python variables. Keep reading to delve deeper into the world of Python variables and learn how to use them effectively in your programs.
Table of Contents
- Declaring and Using Python Variables: A Beginner’s Guide
- Exploring Different Types of Python Variables
- Broadening Your Horizons: Alternative Approaches to Python Variables
- Troubleshooting Common Python Variable Issues
- Understanding Python’s Data Types and Structures
- Memory Management in Python
- Exploring the Relevance of Python Variables
- Wrapping Up: Mastering Python Variables
Declaring and Using Python Variables: A Beginner’s Guide
In Python, declaring a variable is simple. You don’t need to specify the type of variable while declaring it, unlike many other programming languages. Python is smart enough to get the variable type based on the value it is assigned.
Here’s how you can declare and use a Python variable:
# Declare a variable and assign a value
my_variable = 'Hello, Python!'
# Use the variable
print(my_variable)
# Output:
# 'Hello, Python!'
In the code above, we declared a variable called my_variable
and assigned it the value 'Hello, Python!'
. When we print my_variable
, Python retrieves the value stored in my_variable
and outputs 'Hello, Python!'
.
Variables are essential for storing data that your program can use. They allow you to store, retrieve, and manipulate data, making your programs flexible and dynamic.
However, there are a few potential pitfalls to be aware of when using variables in Python:
- Variable Name Rules: Variable names in Python can only contain letters, numbers, or underscores, and they can’t start with a number.
- Case Sensitivity: Python variables are case-sensitive, meaning
my_variable
,My_Variable
, andMY_VARIABLE
would each be different variables. - Reserved Words: Python has some reserved words that can’t be used as variable names, such as
for
,while
,if
, etc.
Understanding these rules and potential pitfalls will help you use variables in Python more effectively.
Exploring Different Types of Python Variables
Python supports various data types, and each type of data can be stored in variables. Let’s delve into the different types of variables you can use in Python and how to work with them.
Integer Variables
An integer variable stores an integer value. Here’s an example:
# Declare an integer variable
num = 10
print(num)
# Output:
# 10
In this example, we declared a variable num
and assigned it the integer value 10
.
String Variables
A string variable stores a string of characters. Here’s an example:
# Declare a string variable
greeting = 'Hello, Python!'
print(greeting)
# Output:
# 'Hello, Python!'
Here, we declared a variable greeting
and assigned it the string 'Hello, Python!'
.
List Variables
A list variable stores a list of items. Here’s an example:
# Declare a list variable
fruits = ['apple', 'banana', 'cherry']
print(fruits)
# Output:
# ['apple', 'banana', 'cherry']
In this example, we declared a variable fruits
and assigned it a list of strings.
Understanding the different types of variables in Python is crucial as it allows you to store different types of data in your programs. It’s best to choose the type of variable that best suits the data you’re working with to make your programs more efficient and easier to understand.
Broadening Your Horizons: Alternative Approaches to Python Variables
As you advance in your Python journey, you’ll find there are several methods to work with variables beyond the basics. These methods involve using different data structures or even third-party libraries. Let’s explore some of these alternative approaches.
Using Tuples
Tuples are similar to lists, but they are immutable, meaning their values can’t be changed once assigned. Here’s an example:
# Declare a tuple
fruits = ('apple', 'banana', 'cherry')
print(fruits)
# Output:
# ('apple', 'banana', 'cherry')
In this example, we declared a tuple fruits
and assigned it a list of strings. Tuples are beneficial when you have a list of items that shouldn’t be changed.
Using Dictionaries
Dictionaries in Python are used to store key-value pairs. Here’s an example:
# Declare a dictionary
person = {'name': 'Alice', 'age': 25}
print(person)
# Output:
# {'name': 'Alice', 'age': 25}
In this example, we declared a dictionary person
and assigned it a set of key-value pairs. Dictionaries are useful when you want to associate values with keys, so you can look them up efficiently.
Using Third-Party Libraries
There are also third-party libraries like NumPy and Pandas that provide additional data structures to work with variables. For instance, the NumPy library provides arrays, and the Pandas library provides dataframes.
# Import NumPy
import numpy as np
# Declare a NumPy array
arr = np.array([1, 2, 3, 4, 5])
print(arr)
# Output:
# [1 2 3 4 5]
In this example, we declared a NumPy array arr
and assigned it a list of integers. NumPy arrays are beneficial when you’re working with large datasets or performing mathematical operations on your data.
Each of these alternative approaches has its advantages and disadvantages, and the best one to use depends on your specific use case. It’s recommended to familiarize yourself with these methods and use them when appropriate to make your Python programs more efficient and versatile.
Troubleshooting Common Python Variable Issues
While working with Python variables, you might encounter a few common issues. Here, we’ll discuss some of these problems, their solutions, and some tips to avoid them in the future.
Type Errors
Type errors occur when you try to perform an operation on a variable that’s not appropriate for its data type. For example:
num = '5'
print(num + 5)
# Output:
# TypeError: can only concatenate str (not "int") to str
In this example, we tried to add an integer to a string, which Python doesn’t allow, resulting in a TypeError
. To fix this, we need to make sure the data types of the variables we’re operating on are compatible.
Name Errors
Name errors occur when you try to use a variable that hasn’t been defined yet. For example:
print(my_var)
# Output:
# NameError: name 'my_var' is not defined
In this example, we tried to print a variable my_var
that we hadn’t defined, resulting in a NameError
. To fix this, we need to ensure that we’ve defined a variable before we use it.
Unassigned Variable Errors
Unassigned variable errors occur when you try to use a variable that has been declared but not assigned a value. For example:
# Declare a variable without assigning a value
var =
print(var)
# Output:
# SyntaxError: invalid syntax
In this example, we declared a variable var
but didn’t assign it a value, resulting in a SyntaxError
. To fix this, we need to ensure that we assign a value to a variable when we declare it.
Understanding these common issues and their solutions will help you troubleshoot problems when working with Python variables. Remember, the key to effective programming is practice and persistence, so don’t be discouraged by these issues. Instead, use them as learning opportunities to improve your Python skills.
Understanding Python’s Data Types and Structures
To fully grasp the concept of Python variables, it’s essential to understand Python’s data types and data structures. These form the foundation upon which variables are built.
Python supports several basic data types, such as:
- Integers: These are whole numbers, like
5
,10
, or-1
. - Floats: These are decimal numbers, like
3.14
,0.99
, or-0.5
. - Strings: These are sequences of characters, like
'Hello, Python!'
. - Booleans: These are truth values, either
True
orFalse
.
Python also supports complex data structures, like:
- Lists: These are ordered collections of items, like
['apple', 'banana', 'cherry']
. - Tuples: These are ordered, immutable collections of items, like
('apple', 'banana', 'cherry')
. - Dictionaries: These are unordered collections of key-value pairs, like
{'name': 'Alice', 'age': 25}
.
Each of these data types and structures can be stored in variables, allowing your Python programs to handle a wide variety of data.
# Integer variable
num = 10
# Float variable
pi = 3.14
# String variable
greeting = 'Hello, Python!'
# Boolean variable
is_true = True
# List variable
fruits = ['apple', 'banana', 'cherry']
# Tuple variable
colors = ('red', 'green', 'blue')
# Dictionary variable
person = {'name': 'Alice', 'age': 25}
Memory Management in Python
When you declare a variable in Python, it reserves a space in memory to store the variable’s value. This memory management is handled automatically by Python, so you don’t need to worry about it in most cases.
However, it’s good to know that each variable in Python holds a reference to the object (i.e., the value) stored in memory. When the reference count of an object drops to zero, Python’s garbage collector automatically frees the memory.
Understanding these fundamental concepts can help you better understand how Python variables work and how to use them effectively in your programs.
Exploring the Relevance of Python Variables
Python variables play a crucial role in various aspects of Python programming. From function definitions to control flow structures like loops, variables are at the heart of it all.
Variables in Function Definitions
In function definitions, variables are used to hold the values of arguments passed to the function. These variables are local to the function and cease to exist once the function execution is over.
# Define a function with a variable
def greet(name):
print(f'Hello, {name}!')
# Call the function
greet('Alice')
# Output:
# 'Hello, Alice!'
In this example, name
is a variable that holds the value of the argument passed to the greet
function.
Variables in Loops
In loops, variables are used as counters or to hold the current item in a sequence.
# Loop through a list using a variable
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, fruit
is a variable that holds the current item in the fruits
list for each iteration of the loop.
Further Resources for Python Variable Mastery
To delve deeper into Python variables and related concepts, here are some resources you might find helpful:
- Beginner’s Guide to Python Data Types – Master Python’s numeric data types for arithmetic operations and computations.
Understanding 2D Arrays in Python – Learn how to work with Python 2D arrays to represent matrices and grids.
Exploring Floats in Python covers Python float operations and avoiding floating-point arithmetic errors.
Official Python Documentation: Using Python as a Calculator – Learn Python’s calculator capabilities from the official guide.
Real Python’s Deep Dive Into Python Variables – Comprehensive coverage of Python variables.
Geeks for Geeks Guide on Python Variables and Data Types explores Python variables and data types.
Remember, mastering Python variables is just the beginning. There’s a whole world of Python programming waiting for you to explore. Happy coding!
Wrapping Up: Mastering Python Variables
In this comprehensive guide, we’ve embarked on a journey to understand the world of Python variables. From their basic use to more advanced techniques, we’ve covered every aspect to provide you with a solid foundation in Python variables.
We began with the basics, exploring how to declare and use variables in Python. We then delved into the different types of variables – integers, strings, and lists – and how to work with them effectively. We also ventured into alternative approaches, such as using tuples, dictionaries, and third-party libraries, to broaden your horizons beyond the basics.
Along the way, we tackled common issues that you might encounter when working with Python variables, such as type errors, name errors, and unassigned variable errors, providing you with solutions and workarounds for each issue.
We also took a step back to understand the fundamentals, discussing Python’s data types and structures and memory management, to give you a deeper understanding of the concepts underlying Python variables.
Here’s a quick comparison of the different ways to handle variables we’ve discussed:
Method | Pros | Cons |
---|---|---|
Basic Use | Simple, easy to understand | Limited functionality |
Advanced Use | More functionality, can handle different data types | More complex, requires understanding of data types |
Alternative Approaches | Broadens possibilities, can handle complex data structures | Requires knowledge of additional libraries |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python variables and their importance in Python programming. Happy coding!