Python Float: Guide to Decimal Numbers
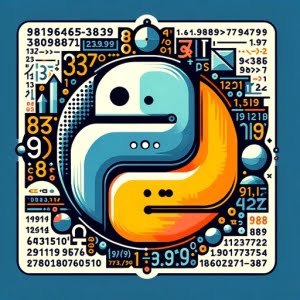
Are you finding it challenging to understand what a float is in Python? You’re not alone. Many developers find themselves puzzled when it comes to working with real numbers in Python.
Think of Python’s float data type as a precise measuring scale – it allows you to work with real numbers with decimal points, providing you with the precision you need.
In this guide, we’ll walk you through the process of working with floats in Python, from the basics to more advanced techniques. We’ll cover everything from declaring and using floats, precision control, to alternative approaches for handling real numbers.
Let’s dive in and start mastering Python floats!
TL;DR: What is a Float in Python?
A float in Python is a numeric data type that is used to represent real numbers. It allows you to work with numbers that have a decimal point, providing a way to handle real numbers in your code.
Here’s a simple example:
num = 3.14
print(type(num))
# Output:
# <class 'float'>
In this example, we’ve declared a variable num
and assigned it the value 3.14
, which is a decimal number. When we print the type of num
, Python tells us it’s a float. This is the basic way to use floats in Python.
But there’s much more to learn about floats in Python, including how to use them in mathematical operations, control their precision, and handle large floats. Continue reading for a more detailed explanation and advanced usage of floats in Python.
Table of Contents
Getting Started with Python Floats
In Python, declaring and using floats is straightforward. You simply assign a decimal number to a variable. Here’s an example:
# declaring a float
pi = 3.14
print(pi)
# Output:
# 3.14
In this example, we’ve declared a float variable pi
and assigned it the value 3.14
. When we print pi
, Python outputs 3.14
.
Advantages of Using Floats
One of the main advantages of using floats is that they allow you to work with real numbers, which are essential in mathematical computations and data analysis. For example, if you’re calculating the area of a circle, you’ll need the value of pi, which is a decimal number.
Pitfalls to Watch Out For
However, it’s important to be aware of some potential pitfalls when working with floats. For instance, due to the way floating-point numbers are represented in computer memory, you might encounter precision errors. Here’s an example:
# precision error with floats
print(0.1 + 0.2)
# Output:
# 0.30000000000000004
In this example, you’d expect the output to be 0.3
, but Python outputs 0.30000000000000004
. This is due to the inherent imprecision of floating-point numbers in computer memory. In most cases, this won’t affect your programs, but it’s something to be aware of when working with floats in Python.
Advanced Python Float Operations
As you become more comfortable with floats in Python, you can start exploring more complex uses. These include mathematical operations, precision control, and handling large floats.
Mathematical Operations with Floats
Python allows you to perform all basic mathematical operations with floats. Here’s an example:
# Mathematical operations with floats
num1 = 10.5
num2 = 2.5
# Addition
print(num1 + num2) # Output: 13.0
# Subtraction
print(num1 - num2) # Output: 8.0
# Multiplication
print(num1 * num2) # Output: 26.25
# Division
print(num1 / num2) # Output: 4.2
In this example, we’ve performed addition, subtraction, multiplication, and division with the float variables num1
and num2
. Python outputs the results as floats.
Precision Control in Floats
You can control the precision of floats in Python using the round()
function. This function takes two arguments: the float you want to round and the number of decimal places. Here’s an example:
# Precision control with round()
num = 3.14159
print(round(num, 2)) # Output: 3.14
In this example, we’ve rounded the float num
to two decimal places using the round()
function. Python outputs 3.14
.
Handling Large Floats
Python can handle very large floats. However, when a float is too large to be represented accurately, Python uses scientific notation. Here’s an example:
# Handling large floats
num = 1e30
print(num) # Output: 1e+30
In this example, we’ve assigned a very large float to the variable num
. When we print num
, Python outputs 1e+30
, which is the scientific notation for the float.
Exploring Alternative Methods for Handling Real Numbers
While floats are a common way to handle real numbers in Python, there are alternative methods you can use. These include the decimal
and fractions
modules, which offer more precision and functionality for certain tasks.
Using the Decimal Module
The decimal
module in Python provides support for fast correctly rounded decimal floating point arithmetic. It’s ideal for financial and monetary calculations. Here’s an example of how to use it:
# Using the decimal module
from decimal import Decimal
num = Decimal('0.1') + Decimal('0.2')
print(num) # Output: 0.3
In this example, we’ve used the Decimal
class from the decimal
module to add 0.1
and 0.2
. Unlike with floats, the result is exactly 0.3
, which demonstrates the precision of the decimal
module.
Working with the Fractions Module
The fractions
module in Python provides support for rational number arithmetic. It can be used when you need to work with fractions. Here’s an example:
# Using the fractions module
from fractions import Fraction
num = Fraction(1, 3) # represents 1/3
print(num) # Output: 1/3
In this example, we’ve used the Fraction
class from the fractions
module to represent the fraction 1/3
. When we print num
, Python outputs 1/3
.
Both the decimal
and fractions
modules offer advantages over using floats, such as more precision and the ability to represent certain numbers exactly. However, they also come with disadvantages, such as being slower and more complex to use. It’s recommended to use these modules when their advantages outweigh their disadvantages for your specific task.
Troubleshooting Common Python Float Issues
While working with floats in Python, you may encounter certain issues, such as precision errors and overflow. Let’s discuss these problems in detail and provide solutions and workarounds.
Overcoming Precision Errors
As we’ve seen earlier, precision errors can occur due to the inherent imprecision of floating-point numbers in computer memory. Here’s an example:
# Precision error with floats
print(0.1 + 0.2) # Output: 0.30000000000000004
In this example, you’d expect the output to be 0.3
, but Python outputs 0.30000000000000004
. This is a precision error.
One way to overcome this issue is to use the round()
function to round the result to the desired number of decimal places. Here’s how to do it:
# Overcoming precision error with round()
result = 0.1 + 0.2
print(round(result, 1)) # Output: 0.3
In this example, we’ve rounded the result of 0.1 + 0.2
to one decimal place using the round()
function. Python outputs 0.3
, which is the expected result.
Handling Overflow Errors
When a float is too large to be represented accurately, Python uses scientific notation. However, if a float is too large even for scientific notation, an overflow error occurs. Here’s an example:
# Overflow error with floats
num = 1e308 * 1.1 # This causes an overflow error
In this example, multiplying 1e308
by 1.1
causes an overflow error because the result is too large to be represented as a float.
One way to handle this issue is to use the decimal
module, which can represent larger numbers. Here’s how to do it:
# Handling overflow error with decimal module
from decimal import Decimal
num = Decimal('1e308') * 1.1
print(num) # This doesn't cause an overflow error
In this example, we’ve used the Decimal
class from the decimal
module to represent the large number. Multiplying Decimal('1e308')
by 1.1
doesn’t cause an overflow error.
Understanding Floating-Point Numbers in Python
Before we delve deeper into the use of floats, it’s crucial to understand what floating-point numbers are and how Python represents them.
The Concept of Floating-Point Numbers
Floating-point numbers, or floats, are real numbers that have a decimal point. They can be positive, negative, or zero. In Python, floats are one of the basic types used to represent numerical values.
# Example of floating-point numbers in Python
positive_float = 3.14
negative_float = -0.01
zero_float = 0.0
print(positive_float, negative_float, zero_float)
# Output:
# 3.14 -0.01 0.0
In this example, we’ve declared three float variables: positive_float
, negative_float
, and zero_float
. When we print these variables, Python outputs their values as floating-point numbers.
Representation of Floats in Python
Python uses the IEEE 754 floating-point standard to represent floats. This standard provides a way to maintain precision and handle very large or very small numbers. However, it can lead to precision errors due to rounding, as we discussed earlier.
Floats vs Other Numeric Types
Python supports several numeric types besides floats, including integers and complex numbers. Each type has its uses and characteristics:
- Integers: These are whole numbers without a decimal point. They’re used when you need to count items or perform calculations that don’t require fractions.
Complex numbers: These are numbers with a real part and an imaginary part. They’re used in fields such as engineering and physics.
Floats: These are real numbers with a decimal point. They’re used when you need to represent fractions or perform calculations that require precision.
While floats are essential for representing real numbers, remember that Python also provides other numeric types for different needs. Choosing the right type can make your code more efficient and easier to understand.
Relevance of Python Floats in Various Fields
Python floats are not only limited to basic arithmetic operations. They hold significant relevance in various advanced fields like data analysis, machine learning, and more.
Python Floats in Data Analysis
In data analysis, floats are often used to represent numerical data, which is crucial for statistical analysis, predictive modeling, and visualization. For instance, when analyzing a dataset of house prices, the prices would typically be represented as floats.
# Example of using floats in data analysis
import pandas as pd
# Create a DataFrame with house prices
data = {'House': ['House1', 'House2', 'House3'], 'Price': [234.5, 456.7, 789.1]}
df = pd.DataFrame(data)
print(df)
# Output:
# House Price
# 0 House1 234.5
# 1 House2 456.7
# 2 House3 789.1
In this example, we’ve created a pandas DataFrame with house prices. The prices are represented as floats.
Python Floats in Machine Learning
In machine learning, floats are used to represent features, labels, and predictions. They’re also used in the internal workings of many machine learning algorithms, such as gradient descent and backpropagation.
Exploring Related Concepts
If you’re interested in delving deeper into Python’s numeric types, you might want to explore related concepts like complex numbers and decimal precision handling. These topics can provide you with a more comprehensive understanding of how Python handles numerical data.
Further Resources for Mastering Python Floats
If you want to learn more about Python floats, here are some resources that you might find helpful:
- Python Data Types Simplified: Quick Reference to Python’s built-in data types for data manipulation and analysis.
Exploring Variables in Python – Master variable usage in Python to write clear and concise code.
Understanding Stacks in Python – Discover Python stack operations and their role in algorithmic problem-solving.
Python’s Official Documentation on Floating Point Arithmetic dives into Python’s handling of floating point numbers.
Python for Data Analysis – Essential guide to python data analysis by industry expert Wes McKinney.
Python Machine Learning – Master Machine Learning with Python with this comprehensive guide.
These resources provide in-depth explanations and practical examples that can help you master the use of Python floats.
Wrapping Up: Mastering Python Floats
In this comprehensive guide, we’ve delved deep into the world of Python floats, exploring their use from basic to advanced levels.
We began with understanding the basic usage of floats in Python, from declaring a float to performing simple arithmetic operations. We then moved on to more advanced techniques, such as controlling the precision of floats and handling large floats. We also highlighted the importance of being aware of precision errors and provided solutions to overcome these issues.
We’ve also explored alternative approaches to handle real numbers in Python, such as the decimal
and fractions
modules, and discussed their advantages and disadvantages. We’ve provided practical code examples to illustrate the usage and effectiveness of these alternatives.
Here’s a quick comparison of the methods we’ve discussed:
Method | Precision | Complexity | Use Case |
---|---|---|---|
Float | Moderate | Low | General purpose |
Decimal | High | Moderate | Financial calculations |
Fraction | Exact | High | Fractional arithmetic |
Whether you’re a beginner just starting out with Python or an experienced developer looking to brush up your skills, we hope this guide has given you a deeper understanding of Python floats and their usage.
Remember, the right method to use depends on your specific task and its requirements. Happy coding!