Learn Python: Pass By Reference
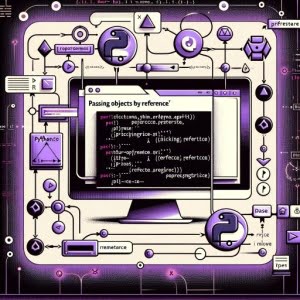
Ever found yourself puzzled over the concept of ‘pass by reference’ in Python? Imagine lending a book to a friend. Any notes or highlights your friend makes in the book will be visible to you when you get it back.
This is similar to how ‘pass by reference’ works in Python — changes made to an object within a function are reflected outside the function as well.
In this comprehensive guide, we’ll demystify the concept of ‘pass by reference’ in Python and provide you with practical examples and useful tips to help you understand and use it effectively.
TL;DR: How Does Python Handle Pass by Reference?
In Python, objects like lists and dictionaries are passed by reference. This means any modifications made inside a function are also visible outside it. Let’s look at a simple example:
def change_list(my_list):
my_list.append(1)
list = []
change_list(list)
print(list)
# Output:
# [1]
In the above example, we defined a function change_list
that appends 1
to the passed list. We then created an empty list, passed it to the function, and printed it. Despite the change being made inside the function, the output shows the list with the added 1
, demonstrating Python’s pass by reference.
For a more in-depth understanding of pass by reference in Python, including how it handles different types of objects and common issues you might encounter, keep reading.
Table of Contents
Pass by Reference with Mutable Objects
Python’s approach to pass by reference particularly comes into play with mutable objects, such as lists and dictionaries. When these objects are passed to a function, any changes made within the function are also reflected outside of it. Let’s look at a simple example using a list:
def modify_list(my_list):
my_list.append('new_item')
my_list = ['item1', 'item2']
modify_list(my_list)
print(my_list)
# Output:
# ['item1', 'item2', 'new_item']
In this example, we defined a function modify_list
that appends a string 'new_item'
to the given list. We then created a list my_list
with two items, passed it to the function and printed it. The output shows the list with the added 'new_item'
, despite this addition being made inside the function. This demonstrates Python’s pass by reference with mutable objects.
This feature can be quite useful, as it allows functions to directly modify data structures. However, it’s crucial to be mindful of this behavior as it can lead to unexpected results if not handled properly. For example, if you’re not intending to modify the original list, you might be surprised to find it changed after calling a function on it.
Pass by Reference with Immutable Objects
When dealing with immutable objects such as integers and strings, Python’s handling of pass by reference behaves slightly differently. Let’s explore this with an example:
def modify_value(my_val):
my_val += 10
my_val = 20
modify_value(my_val)
print(my_val)
# Output:
# 20
In this example, we defined a function modify_value
that adds 10
to the given value. We then assigned the value 20
to my_val
, passed it to the function, and printed it. Interestingly, the output shows the original value of my_val
, not the incremented value.
This is because integers are immutable in Python. When my_val
is passed to the function, a new local my_val
is created within the function scope. Any changes made to this local my_val
do not affect the original my_val
outside the function.
This is a key difference between Python’s handling of pass by reference with mutable and immutable objects. With mutable objects like lists and dictionaries, modifications inside a function are reflected outside. But with immutable objects like integers and strings, changes inside a function are local to the function and do not affect the original object.
Understanding this difference is crucial for effective Python programming. It helps prevent bugs and ensures your functions behave as expected.
Exploring Alternative Approaches
While Python’s pass by reference behavior is fundamental to its operation, there are alternative methods to achieve similar results. One of these methods involves using the copy
module. Let’s explore this with an example:
import copy
def modify_list_copy(my_list):
my_list_copy = copy.deepcopy(my_list)
my_list_copy.append('new_item')
return my_list_copy
my_list = ['item1', 'item2']
new_list = modify_list_copy(my_list)
print('Original list:', my_list)
print('New list:', new_list)
# Output:
# Original list: ['item1', 'item2']
# New list: ['item1', 'item2', 'new_item']
In this example, we used the copy.deepcopy()
function to create a copy of my_list
inside the function. We then appended 'new_item'
to the copy, not the original list. This way, the original list remains unchanged, and the function returns a new list with the modifications.
This method can be particularly useful when you want a function to modify a data structure without affecting the original. However, it does come with a downside: making a copy of a large data structure can be memory-intensive and slow down your program.
Troubleshooting Python’s Pass by Reference
While Python’s pass by reference behavior is generally predictable, you may encounter some issues or unexpected behavior, particularly when dealing with immutable objects. Let’s discuss some common issues and their solutions.
Unexpected Behavior with Immutable Objects
One common issue arises when trying to modify an immutable object inside a function, expecting it to reflect outside the function. As we’ve seen earlier, this doesn’t happen due to Python’s handling of immutable objects. Here’s an example:
def modify_string(my_str):
my_str += ' world'
my_str = 'Hello'
modify_string(my_str)
print(my_str)
# Output:
# 'Hello'
In this example, we tried to append ' world'
to my_str
inside the function. However, the output shows the original my_str
, without the appended text. This is because strings are immutable in Python, and any changes made inside the function are local to the function and do not affect the original string.
Solutions and Workarounds
One solution to this issue is to have the function return the modified object and assign it to the original variable. Here’s how you can do it:
def modify_string(my_str):
my_str += ' world'
return my_str
my_str = 'Hello'
my_str = modify_string(my_str)
print(my_str)
# Output:
# 'Hello world'
In this revised example, the function modify_string
returns the modified string, which is then assigned to my_str
. The output now shows the expected 'Hello world'
.
Python’s Object Model and Pass by Reference
To fully grasp Python’s pass by reference behavior, it’s essential to understand its object model and the difference between mutable and immutable objects.
Python’s Object Model
In Python, everything is an object, whether it’s a number, a string, a list, or a custom class you’ve created. Each object has an identity (its address in memory), a type (e.g., int, str, list), and a value. The identity and type of an object are immutable, but the value can be mutable or immutable, depending on the object’s type.
Mutable vs Immutable Objects
Mutable objects, like lists and dictionaries, can have their value changed after they’re created. This means you can add, remove, or modify elements in a list or dictionary.
On the other hand, immutable objects, like integers and strings, cannot have their value changed after they’re created. If you try to change the value of an immutable object, Python creates a new object with the new value.
# Mutable object example
list1 = [1, 2, 3]
list1.append(4) # This changes the value of list1
print(list1)
# Output: [1, 2, 3, 4]
# Immutable object example
num = 10
num += 5 # This creates a new object with the value 15
print(num)
# Output: 15
In the mutable object example, we appended 4
to list1
, changing its value. In the immutable object example, we added 5
to num
. However, instead of changing the value of num
, Python created a new object with the value 15
.
Understanding Python’s object model and the difference between mutable and immutable objects is crucial for understanding its pass by reference behavior.
Extending Python’s Pass by Reference to Real-world Applications
Understanding Python’s pass by reference behavior isn’t just an academic exercise, it has real-world implications in various Python applications, especially in data manipulation with libraries like pandas and numpy.
Data Manipulation in Pandas and Numpy
When working with these libraries, understanding how Python handles object references can be crucial. For instance, when you pass a pandas DataFrame or a numpy array to a function, any changes made inside the function will be reflected in the original DataFrame or array, much like how lists and dictionaries behave.
import numpy as np
def modify_array(arr):
arr[0] = 100
arr = np.array([1, 2, 3])
modify_array(arr)
print(arr)
# Output:
# array([100, 2, 3])
In this example, we passed a numpy array to a function and changed its first element. The change is reflected in the original array, demonstrating Python’s pass by reference behavior with numpy arrays.
Delving Deeper: Memory Management in Python
To extend your understanding of Python’s pass by reference, you might want to explore related concepts like memory management in Python. Understanding how Python allocates and manages memory can give you deeper insights into why it behaves the way it does with object references.
There are many resources available online for learning about Python’s memory management, including official Python documentation and various tutorials and blog posts. Diving into these resources can help you become a more proficient and effective Python programmer.
Python’s Pass by Reference: A Recap
In our journey through Python’s pass by reference, we’ve seen how Python handles it with different types of objects. With mutable objects like lists and dictionaries, Python reflects changes made inside a function outside of it. However, with immutable objects like integers and strings, changes inside a function are local to the function and do not affect the original object.
We’ve also discussed some common issues you might encounter with Python’s pass by reference, particularly with immutable objects. We’ve suggested solutions and workarounds for these issues, such as having the function return the modified object and assigning it to the original variable.
In addition to Python’s built-in pass by reference behavior, we’ve explored alternative approaches like using the copy
module to create a copy of an object and modify it without affecting the original object. However, we’ve noted that this approach can be memory-intensive and slow for large data structures.
Understanding Python’s pass by reference and these alternative approaches can give you more flexibility in your programming. It allows you to choose the most appropriate method for your specific needs and constraints, and helps you write more effective and bug-free Python code.