Python Substring Quick Reference
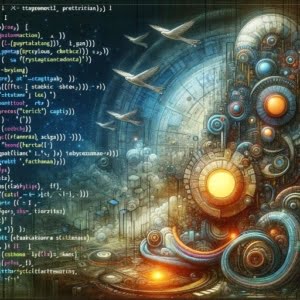
Has Python’s string manipulation ever left you feeling like you’re navigating a maze? Have you wished for an easier way to extract specific character sequences? If so, you’re in the right place. This blog post will demystify Python’s string manipulation, focusing particularly on substring extraction.
Whether you’re a Python novice or a seasoned coder looking for a refresher, this comprehensive guide will equip you with the skills to extract substrings in Python effectively. By the end of this post, you’ll be slicing through strings with the precision of a master chef.
TL;DR: How do I extract a substring in Python?
To extract a substring in Python, you typically use the slicing method. You can also use Python’s built-in string methods like
find()
,index()
,split()
, etc, depending on what you wish to achieve.
Here is how you do it with slicing:
# given string s
s = "Hello, World!"
# get the substring from index 2 to index 5 (exclusive)
sub_str = s[2:5]
print(sub_str)
# Output: llo
Remember, Python indexing starts at 0, so index 2 is the third character and slicing is exclusive of the end index.
Table of Contents
Introduction to Substrings
A substring is a portion of a string. It can be as short as a single character or as long as the entire string itself. Substring extraction is a key skill in string manipulation, and Python offers several built-in methods to do this effectively.
Python offers a variety of ways to extract substrings from a string. One of the most intuitive methods is through slicing.
Python String Slicing
In Python, slicing allows you to extract a section of a sequence, such as a string. It’s akin to taking a slice from a cake. You specify where your slice starts and ends, and Python hands you the piece.
The syntax for slicing in Python is sequence[start:stop:step]
. Here’s how it works:
start
: The index where the slice starts. If omitted, it defaults to 0, which is the beginning of the string.stop
: The index where the slice ends. This index is not included in the slice. If omitted, it defaults to the length of the string.step
: The amount by which the index increases. If omitted, it defaults to 1. If the step value is negative, the slicing goes from right to left.
Here’s a basic example:
string = "Hello, Python!"
substring = string[0:5]
print(substring) # Outputs: Hello
This code extracts the first five characters from the string, forming the substring ‘Hello’.
Example of slicing with a step value:
string = 'Hello, Python!'
substring = string[::2]
print(substring) # Outputs: 'Hlo yhn'
The “2” in the step above means it will increment forward by 2 characters each time it extracts a character — in effect, skipping every other character.
Examples of Substring Extraction
Let’s explore a few more examples of substring extraction in different scenarios.
Extracting the first n characters from a string:
string = "Hello, Python!"
substring = string[:5]
print(substring) # Outputs: Hello
Extracting the last n characters from a string:
string = "Hello, Python!"
substring = string[-1:]
print(substring) # Outputs: !
Extracting a substring between two known substrings:
string = "Hello, Python!"
start = string.find('Hello') + len('Hello')
end = string.find('Python')
substring = string[start:end].strip()
print(substring) # Outputs: ,
Substring Extraction: Important Considerations
While working with substrings, remember that Python string indices start at 0. Also, in Python, strings are immutable, meaning they can’t be changed after they’re created. So, any operation that manipulates a string will actually create a new string.
Example of string immutability:
string = 'Hello, Python!'
string[0] = 'h' # Raises a TypeError
Other Methods for Substring Extraction
Beyond slicing, Python also offers several built-in methods for substring extraction, such as find()
, index()
, and split()
.
Find()
The find()
method returns the index of the first occurrence of the specified substring. If the substring is not found, it returns -1.
string = "Hello, Python!"
index = string.find('Python')
print(index) # Outputs: 7
Index()
The index()
method is similar to find()
, but raises an exception if the substring is not found.
Example of using the index()
method:
string = 'Hello, Python!'
index = string.index('Python')
print(index) # Outputs: 7
Split()
The split()
method splits a string into a list where each word is a list item. You can specify the separator; the default separator is any whitespace.
string = "Hello, Python!"
words = string.split()
print(words) # Outputs: ['Hello,', 'Python!']
Efficiency of Substring Extractions
The efficiency of a substring extraction method depends on the specific requirements of your task. Slicing is a fast and efficient method for extracting substrings when you know the start and end indices. But if you need to find the position of a substring within a string, methods like find()
and index()
are more suitable.
Example of comparing the performance of slicing and the find()
method:
import time
# The '*' operator concatenates the string 'Hello, Python!' 10,000 times
string = 'Hello, Python!' * 10000
# We'll run each command 1,000 times in a loop
repeat_count = 1000
start = time.time()
for _ in range(repeat_count):
substring = string[0:5]
end = time.time()
print('Slicing:', end - start)
start = time.time()
for _ in range(repeat_count):
index = string.find('Python')
end = time.time()
print('find():', end - start)
If you run the above code, you can get an idea for the time it takes for each method.
In terms of performance, Python’s built-in string methods are implemented in C, making them highly efficient.
Python String Manipulation Basics
Now that you’ve learned about Substrings, it may be helpful to get a broader view on String operations in Python more generally.
In Python, a string is a series of characters enclosed in single ('Hello'
) or double quotes ("Hello"
). Strings are one of the most frequently used data types in Python, boasting a multitude of built-in methods for text data manipulation.
example_string = "Hello, Python!"
print(example_string)
Executing the above code will output: Hello, Python!
Python strings are not just sequences of characters; they’re versatile tools that can be manipulated in numerous ways. Here are some basic operations you can perform on strings:
Operation | Description |
---|---|
Concatenation | Joins two or more strings together |
Repetition | Repeats a string a specified number of times |
Indexing | Accesses a character at a specific position in the string |
Regular Expressions | Also known as “regex”, these allow for flexible pattern matching and substitution with strings |
Slicing
This operation extracts a part of the string.
Here’s an example of how you can extract substrings from a Python string using slicing:
string = "Hello, Python"
# Slicing from 3rd to 7th character
substring = string[2:7]
print(substring) # Outputs: llo,
Concatenation
This operation joins two or more strings together.
string1 = "Hello"
string2 = "Python"
print(string1 + string2) # Outputs: HelloPython
Repetition
This operation repeats a string a specified number of times.
string = "Hello"
print(string * 3) # Outputs: HelloHelloHello
Indexing
This operation accesses a character at a specific position in the string.
string = "Hello"
print(string[1]) # Outputs: e
Inserting Variables into Strings: String Formatting
Python provides several ways to format strings, enabling you to insert variables into strings and format them in various ways. The format()
method is a versatile tool for string formatting. It replaces placeholders – denoted by {}
– in the string with its arguments.
Example of string formatting using f-strings with the format()
command:
name = 'Python'
string = 'Hello, {}!'.format(name)
print(string) # Outputs: Hello, Python!
Python 3.6 introduced f-strings, a new way of formatting strings that’s more concise and readable than the format()
method.
name = 'Python'
string = f'Hello, {name}!'
print(string) # Outputs: Hello, Python!
Regular Expressions for Powerful String Manipulation
Regular expressions, or regex, are a potent tool for string manipulation. They offer a flexible and efficient way to search, replace, and extract information from strings. Python’s re
module supports regular expressions, providing a robust platform for complex string manipulations.
When it comes to substring extraction, regular expressions come into their own when you want to extract a substring that matches a specific pattern.
For instance, you may need to extract all email addresses from a text or all dates in a particular format. Let’s look at an example of using regular expressions to extract substrings in Python:
import re
string = "The rain in Spain"
substring = re.findall("ai", string)
print(substring) # Outputs: ['ai', 'ai']
In this example, the re.findall()
method returns all occurrences of the pattern ‘ai’ in the string.
Regular expressions excel in complex substring extraction scenarios. For example, you might need to extract all the URLs from a webpage or all the hashtags from a social media post. Regular expressions make these tasks straightforward. Here’s an example of how to extract all the hashtags from a text:
import re
text = "#Python is awesome. #coding"
hashtags = re.findall(r"#(w+)", text)
print(hashtags) # Outputs: ['Python', 'coding']
In this example, the regular expression r"#(w+)"
matches any sequence of alphanumeric characters preceded by a hashtag.
Python’s In-Built String Methods
Python’s built-in string methods offer a robust set of tools for string manipulation. These include lower()
, upper()
, split()
, replace()
, find()
, among others. See below for examples of the more commonly used built-in methods:
Strip()
strip()
( and rstrip() ) Removes leading and trailing whitespace from a string.
string = ' Hello, Python! '
print(string.strip()) # Outputs: 'Hello, Python!'
Upper()
upper()
Converts a string to uppercase.
string = 'Hello, Python!'
print(string.upper()) # Outputs: 'HELLO, PYTHON!'
Lower()
lower()
Converts a string to lowercase.
string = 'Hello, Python!'
print(string.lower()) # Outputs: 'hello, python!'
StartsWith()
startswith()
Checks if a string starts with a specified substring.
string = 'Hello, Python!'
print(string.startswith('Hello')) # Outputs: True
EndsWith()
endswith()
Checks if a string ends with a specified substring.
string = 'Hello, Python!'
print(string.endswith('Python!')) # Outputs: True
Count()
count()
Counts the number of occurrences of a substring in a string.
string = 'Hello, Python!'
print(string.count('o')) # Outputs: 2
Split()
split()
breaks up a string at the specified separator and returns a list of substrings.
string = 'Hello, Python!'
# Split string at every space
print(string.split(' ')) # Outputs: ['Hello,', 'Python!']
Replace()
replace()
replaces all occurrences of a substring in a string with another substring.
string = 'Hello, Python!'
# Replace 'Hello' with 'Hi'
print(string.replace('Hello', 'Hi')) # Outputs: 'Hi, Python!'
Find()
find()
searches for a substring in a string and returns the index of the first occurrence. If the substring is not found, it returns -1.
string = 'Hello, Python!'
# Find the first occurrence of 'o'
print(string.find('o')) # Outputs: 4
These are just a few examples of Python’s built-in string methods. By mastering these methods, you can perform a wide array of string manipulation tasks in Python with ease and efficiency.
Examples with Other Languages
While Python string manipulation, particularly substring extraction, has been our main focus, it’s essential to note that string manipulation is a core aspect of almost all programming languages.
However, the implementation of string manipulation, especially substring extraction, can significantly differ from one language to another. Let’s briefly examine how substring extraction is accomplished in some other popular programming languages.
Java
In Java, the substring()
method is utilized to extract a substring from a string. It can accept one or two parameters: the start index, and optionally, the end index.
String string = "Hello, Java!";
String substring = string.substring(0, 5);
System.out.println(substring); // Outputs: Hello
JavaScript
JavaScript also employs a substring()
method for substring extraction. As in Java, you can specify the start and end indices.
let string = "Hello, JavaScript!";
let substring = string.substring(0, 5);
console.log(substring); // Outputs: Hello
C++
In C++, the substr()
function is used to extract a substring. You specify the start index and the length of the substring.
#include <iostream>
#include <string>
int main() {
std::string string = "Hello, C++!";
std::string substring = string.substr(0, 5);
std::cout << substring << std::endl; // Outputs: Hello
return 0;
}
Ruby
Ruby employs the slice()
method for substring extraction. You can specify the start index and the length of the substring, or provide a range.
string = "Hello, Ruby!"
substring = string.slice(0, 5)
puts substring # Outputs: Hello
As evident, while the method names and syntax may vary, the concept of substring extraction is a common thread across all these languages.
Python’s approach, with its powerful slicing syntax and rich set of string methods, offers a particularly flexible and intuitive way to manipulate strings.
Further Resources for Python Strings
If you’re interested in learning more ways to handle strings in Python, here are a few resources that you might find helpful:
- Revolutionizing Text Processing in Python with Strings: Revolutionize your text processing skills in Python by mastering string methods and best practices outlined in this.
Python Strip(): Removing Leading and Trailing Characters from a String: Learn how to use the strip() method in Python to remove leading and trailing characters from a string, with examples and explanations of different use cases.
Python Match Case Statement: New Pattern Matching Syntax: This article introduces the new match case statement in Python.
How to Substring a String in Python: An article on freeCodeCamp that explains different ways to extract substrings from a string in Python, with examples and explanations.
Python String Substring: Tutorial and Examples: DigitalOcean provides a tutorial on Python string substring operations, covering various techniques to extract substrings from a string.
Python substring() Method: Simplilearn Tutorial: Simplilearn offers a tutorial on the substring() method in Python, explaining how to extract substrings from a string using this method.
Wrapping Up
In this comprehensive guide, we’ve journeyed through the world of Python string manipulation, with a spotlight on substring extraction. We’ve untangled the different techniques to extract substrings in Python, from the straightforward slicing technique to the potent regular expressions, and the built-in string methods like find()
, index()
, and split()
.
Each method comes with its unique strengths and weaknesses. Slicing is quick and efficient for simple substring extraction tasks, but it demands knowledge of the start and end indices of the substring. Python’s built-in string methods provide a handy way to perform a variety of string manipulation tasks, including substring extraction. However, for complex substring extraction tasks that involve pattern matching, regular expressions are the go-to tool, despite their potential pitfalls and performance implications.
But substring extraction is just one facet of string manipulation in Python. Python’s string manipulation capabilities extend far beyond that, encompassing a wide array of operations like concatenation, formatting, and various string methods. By mastering these capabilities, you can unlock the full potential of Python strings and significantly enhance your Python programming skills.
We also took a swift detour to explore how substring extraction is handled in other popular programming languages, underscoring the flexibility and intuitiveness of Python’s approach to string manipulation.
So, keep honing your string manipulation skills and happy Pythoning! Remember, extracting substrings in Python is like finding a specific book in a library. It might seem overwhelming at first, but once you know the system, it becomes a walk in the park.