Python Get Index of Item In List | 3 Simple Methods
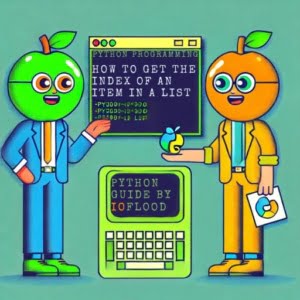
While automating our data retrieval processes at IOFLOOD, we needed to decide how to get the index of an item in a list in a simple and consistent manner. Through practice, we have discovered various methods like list.index()
, and more. Today, we aim to share our personal tips to benefit our dedicated hosting customers also looking to improve their data management projects.
This straightforward guide will quickly walk you through the built-in functions in Python to get index of item in list. Whether you’re a beginner just getting started or an intermediate programmer looking to brush up on your skills, this guide has got you covered.
So let’s dive and and learn how to get index of elements in lists for Python!
TL;DR: How Do I Find Index of Item in List with Python?
In Python, get index of item in list by using the built-in function
list.index()
with the syntax,index = my_list.index([item])
.
Here’s a simple example:
my_list = ['apple', 'banana', 'cherry']
index = my_list.index('banana')
print(index)
# Output:
# 1
In the above example, we have a list called my_list
containing three items. We want to find the index of the item ‘banana’. The list.index()
function does just that, returning the position of ‘banana’ in the list (remember, Python list indices start from 0). So, when we print the index, it outputs ‘1’ because ‘banana’ is the second item in the list.
Intrigued? Keep reading for a more detailed explanation, including handling advanced scenarios and alternative approaches.
Table of Contents
Intro to Python Get Index of Item in List
Python’s list.index()
function is a built-in method that returns the index of the first occurrence of an item in a list. It’s a simple and straightforward way to locate an item in a list.
Here’s how it works:
my_list = ['apple', 'banana', 'cherry']
index = my_list.index('banana')
print(index)
# Output:
# 1
In the above snippet, my_list.index('banana')
returns ‘1’, which is the index of ‘banana’ in the list my_list
. Python list indices start from 0, so ‘apple’ is at index 0, ‘banana’ at index 1, and ‘cherry’ at index 2.
Understanding the list.index()
Function
The list.index()
function takes one mandatory argument, which is the item you’re looking for. It searches the list from the beginning, and once it finds the item, it immediately returns the index and stops searching.
Advantages and Pitfalls
The list.index()
function is straightforward and easy to use. It’s efficient for small lists or when you’re sure the item is in the list.
However, if the item isn’t in the list, Python will raise a ValueError
.
Here’s an example:
my_list = ['apple', 'banana', 'cherry']
index = my_list.index('orange')
print(index)
# Output:
# ValueError: 'orange' is not in list
In the above code, we tried to find ‘orange’ in my_list
, but since ‘orange’ is not in the list, Python raises a ValueError
. In the next section, we’ll discuss how to handle such scenarios and more advanced use cases.
Other Methods: Get Python List Index
While Python’s list.index()
function is a handy tool for finding the index of an item in a list, it’s not the only way.
Let’s delve into some alternative methods, such as using a for loop or list comprehension, and understand their usage and effectiveness.
Using a For Loop
A for loop can be used to iterate over the list and check each item. Here’s an example:
my_list = ['apple', 'banana', 'cherry']
for i in range(len(my_list)):
if my_list[i] == 'banana':
print(i)
# Output:
# 1
In the above code, the for loop iterates over the indices of my_list
. If the item at the current index is ‘banana’, it prints the index.
This method gives you more control over the iteration process compared to
list.index()
. However, it’s more verbose and might be overkill for simple scenarios.
Leveraging List Comprehension
List comprehension is a compact way of creating a new list by iterating over an existing one. It can be combined with the enumerate()
function to find the indices of an item. Here’s how:
my_list = ['grape', 'banana', 'orange', 'blueberry', 'grape']
indices = [i for i, x in enumerate(my_list) if x == 'banana']
print(indices)
# Output:
# [1]
In the above code, the list comprehension iterates over my_list
with enumerate()
, which returns each item along with its index. It filters out the indices where the item is ‘banana’.
This method is concise and powerful, especially when dealing with multiple occurrences of an item. However, it might be less readable for beginners compared to the for loop or
list.index()
method.
In conclusion, each method has its strengths and weaknesses. The list.index()
function is simple and straightforward, the for loop provides more control, and list comprehension is compact and powerful. Choose the one that best fits your situation.
Errors with Python Index of Item in List
Finding the index of an item in a Python list is generally straightforward, but like any programming task, it can present some challenges. Let’s discuss common issues you might encounter and how to resolve them.
Navigating Plython list index ValueError
The most common issue you might need to handle is a ValueError
. As we mentioned earlier, Python’s list.index()
function will raise a ValueError
if it can’t find the item in the list.
To handle this, you can use a try-except block. Here’s an example:
my_list = ['apple', 'banana', 'cherry']
try:
index = my_list.index('orange')
print(index)
except ValueError:
print('Item not in list')
# Output:
# Item not in list
In the above code, we try to find ‘orange’ in my_list
. Since ‘orange’ is not in my_list
, Python raises a ValueError
, which we catch in the except block and print a friendly message.
Dealing with Non-Existence of an Item
If you’re not sure whether an item is in the list and don’t want to use a try-except block, you can check if the item is in the list before calling list.index()
. Here’s how:
my_list = ['apple', 'banana', 'cherry']
item = 'orange'
if item in my_list:
print(my_list.index(item))
else:
print('Item not in list')
# Output:
# Item not in list
In the above code, we first check if ‘orange’ is in my_list
with the in
keyword. If it is, we print its index. If it’s not, we print a friendly message.
Handling Multiple Occurrences of an Item
The list.index()
function only returns the index of the first occurrence of an item. If there are multiple occurrences of the item, and you want to find their indices, you can use a for loop along with the enumerate()
function. Here’s how:
my_list = ['apple', 'banana', 'cherry', 'apple', 'cherry']
indices = [i for i, x in enumerate(my_list) if x == 'apple']
print(indices)
# Output:
# [0, 3]
In the above code, we use a list comprehension with enumerate()
, which returns each item in my_list
along with its index. The list comprehension filters out the indices where the item is ‘apple’. So, the output is a list of all indices where ‘apple’ appears in my_list
.
By considering these common issues and their solutions, you can write robust code that finds the index of an item in a Python list reliably and efficiently.
Decoded: Find Index of Item in List
Before we dive deeper into finding an item’s index in a Python list, let’s take a moment to understand Python lists and their properties.
A list in Python is an ordered collection of items which can be of any type. Lists are mutable, meaning you can add, remove, and change items after the list is created. Here’s an example of a Python list:
my_list = ['apple', 'banana', 'cherry']
print(my_list)
# Output:
# ['apple', 'banana', 'cherry']
In the above code, my_list
is a list of three strings. We print my_list
, and it outputs the entire list.
List Indices
Each item in a Python list has an index, which is its position in the list. Indices in Python lists start from 0, so the first item is at index 0, the second item is at index 1, and so on. Here’s an illustration:
my_list = ['apple', 'banana', 'cherry']
print(my_list[0]) # prints 'apple'
print(my_list[1]) # prints 'banana'
print(my_list[2]) # prints 'cherry'
In the above code, we access each item in my_list
by its index. So, my_list[0]
returns ‘apple’, my_list[1]
returns ‘banana’, and my_list[2]
returns ‘cherry’.
Understanding Python lists and their indices is fundamental to finding the index of an item, as we’ve been discussing. With this foundation, you can better appreciate the
list.index()
function and other methods to find an item’s index in a Python list.
Use Cases of Python List Item Index
Finding the index of an item in a Python list is not just an isolated task. It often forms a part of larger scripts or projects. For instance, on a data analysis project you might have a list of data points. Finding the data point’s index can help you access corresponding data in other lists or arrays.
Exploring Related Concepts
Understanding how to find an item’s index in a list is a stepping stone to exploring more advanced concepts in Python. List manipulation, such as adding, removing, or changing items, and list iteration, such as looping over a list or using list comprehensions, are areas worth exploring further.
# List Manipulation
my_list = ['apple', 'banana', 'cherry']
my_list.append('orange')
print(my_list)
# Output:
# ['apple', 'banana', 'cherry', 'orange']
# List Iteration
for i, item in enumerate(my_list):
print(f'Item {i} is {item}')
# Output:
# Item 0 is apple
# Item 1 is banana
# Item 2 is cherry
# Item 3 is orange
In the first code block, we add an item to my_list
with the append()
function, demonstrating list manipulation. In the second code block, we iterate over my_list
with a for loop and the enumerate()
function, demonstrating list iteration.
For more information on Python list manipoulation, check out our complete Python List Methods guide, here!
Further Resources for Python
To deepen your understanding of Python lists and related concepts, consider exploring Python’s official documentation, online programming courses, or Python-focused blogs and forums. The more you explore, the more proficient you’ll become at handling Python lists and tackling more complex tasks.
Here are a few resources from our blog that you might find helpful:
- Python Lists and Their Impact on Data Analysis: Gain insights into the crucial role of Python lists in data analysis and discover effective methods for applying them in your analyses.
Appending Elements to a List in Python: A guide demonstrating different approaches to appending elements to a list in Python, including the append() method and list concatenation.
Creating a Unique List in Python: A tutorial that explores various methods to create a unique list in Python by removing duplicate elements.
Python list index() Method: The official documentation on the index() method for Python lists from Programiz, explaining how to get the index of an element in a list.
Python List index(): An article on GeeksforGeeks that demonstrates the usage of the index() method to find the index of an element in a list.
List Index Method in Python: A DataCamp tutorial that covers the index() method in Python and includes examples to illustrate its usage.
End: Get Index of Item in List Python
Throughout this guide, we’ve explored various methods to find the index of an item in a Python list, a common task that forms the basis for more complex operations in Python programming.
We’ve seen how to use Python’s built-in list.index()
function, handled scenarios where the item is not in the list or when there are multiple occurrences of the item, and delved into alternative approaches using a for loop and list comprehension.
Each method has its advantages and potential pitfalls. The list.index()
function is simple and straightforward, but it can raise a ValueError
if the item is not in the list. The for loop gives you more control over the iteration process, while list comprehension offers a compact and powerful way to handle multiple occurrences of an item.
In conclusion, understanding how to find the index of an item in a Python list is a fundamental skill in Python programming. It’s not just about locating an item—it’s about understanding Python lists, their properties, and how to manipulate them. So, keep exploring, keep coding, and you’ll master Python lists in no time!