Python Unique List: How To Remove Duplicates from Lists
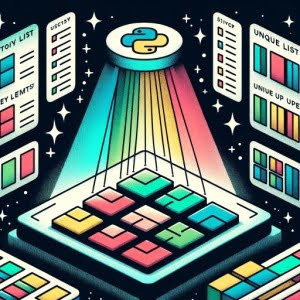
Unique lists have helped us maintain accuracy in our data sets while working to improve our internal scripts at IOFLOOD. As our use cases do not require recording duplicate entries, it has been necessary to consistently filter them out. In this article, we explore different techniques for creating unique lists in Python, sharing our best practices and examples to aid our cloud server hosting customers.
This guide will walk you through the process of creating a unique list in Python, from the basics to more advanced techniques. We’ll cover everything from using the set data type to more sophisticated methods like list comprehension and the unique() function from the numpy library. We’ll also discuss alternative approaches and common issues you may encounter along the way.
So, let’s dive in and start mastering the creation of unique lists in Python!
TL;DR: How Do I Create a Unique List in Python?
The simplest way to create a unique list in Python is by converting the list to a set and then back to a list, like
unique_list = list(set(my_list))
. This is because sets, by definition, cannot have duplicate elements. Here’s a quick example:
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(set(my_list))
print(unique_list)
# Output:
# [1, 2, 3, 4, 5]
In this example, we first convert the list to a set, which automatically removes any duplicate elements. Then, we convert the set back to a list. The result is a list with the same elements as the original, but with all duplicates removed.
This is a basic way to create a unique list in Python, but there’s much more to learn about handling lists and removing duplicates. Continue reading for more detailed explanations and advanced techniques.
Table of Contents
Basic Use: The Set Method
If you’re new to Python or just want a quick and easy way to create a unique list, the set method is your go-to solution. This method works by converting your list into a set.
In Python, a set is a built-in data type that automatically removes all duplicates. Once your list is converted into a set, you can convert it back into a list, and voila, you have a list with no duplicate elements.
Here’s an example:
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(set(my_list))
print(unique_list)
# Output:
# [1, 2, 3, 4, 5]
In this example, we start with a list that has duplicate elements. We then convert that list into a set using the set()
function, which automatically removes any duplicates. Finally, we convert the set back into a list using the list()
function.
The advantage of this method is its simplicity. It’s a quick and easy way to remove duplicates from a list. However, one potential pitfall to keep in mind is that sets, unlike lists, are unordered.
When you convert a list into a set, you may lose the original order of your elements. If the order of elements is important for your use case, you may need to explore other methods of creating a unique list in Python, which we’ll cover in the next sections.
Advanced Techniques for Unique Lists
For those who are more comfortable with Python, there are more advanced methods to create a unique list. These methods offer more control and can preserve the order of elements, unlike the set method.
Using List Comprehension
List comprehension is a Pythonic way to perform operations on lists. It’s like a compact for-loop. Here’s how you can use list comprehension to create a unique list:
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = []
[unique_list.append(i) for i in my_list if i not in unique_list]
print(unique_list)
# Output:
# [1, 2, 3, 4, 5]
In this example, we start with an empty list called unique_list
. We then use list comprehension to iterate over my_list
. For each element in my_list
, we check if it’s already in unique_list
. If it’s not, we append it to unique_list
. The result is a list with the same elements as my_list
, but with all duplicates removed.
Using NumPy’s Unique Function
If you’re working with numerical data, the NumPy library offers a handy function called unique()
. This function returns a sorted, unique array from the input array.
Here’s an example:
import numpy as np
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = np.unique(my_list)
print(unique_list)
# Output:
# array([1, 2, 3, 4, 5])
In this example, we import the NumPy library and use the unique()
function to create a unique list from my_list
. Note that unique()
returns an array, not a list. If you need a list, you can convert the array back to a list using the tolist()
function.
Both of these methods offer more control than the set method and can preserve the order of elements. However, they may be a bit more complex, especially for beginners. As always, the best method to use depends on your specific needs and the nature of your data.
Alternate Tools for Duplicate Removal
While the set method, list comprehension, and NumPy’s unique function are popular ways to create a unique list in Python, they are by no means the only ones. In this section, we’ll explore two alternative methods: using the pandas library and creating a custom function.
Using Pandas’ drop_duplicates Method
The pandas library is a powerful tool for data manipulation in Python. It provides a method called drop_duplicates()
that can be used to remove duplicate elements from a list.
Here’s an example:
import pandas as pd
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = pd.Series(my_list).drop_duplicates().tolist()
print(unique_list)
# Output:
# [1, 2, 3, 4, 5]
In this example, we first convert my_list
into a pandas Series. We then use the drop_duplicates()
method to remove any duplicates. Finally, we convert the Series back into a list.
Creating a Custom Function
If you need more control over how duplicates are removed, you can create a custom function. This function can be tailored to your specific needs.
Here’s an example of a custom function that removes duplicates while preserving the order of elements:
def unique_list(input_list):
seen = set()
result = []
for item in input_list:
if item not in seen:
seen.add(item)
result.append(item)
return result
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = unique_list(my_list)
print(unique_list)
# Output:
# [1, 2, 3, 4, 5]
In this function, we use a set to keep track of elements we’ve already seen. For each element in input_list
, we check if it’s already in seen
. If it’s not, we add it to seen
and append it to result
. The result is a list with the same elements as input_list
, but with all duplicates removed.
Both of these methods offer more control than the set method and can preserve the order of elements. However, they may require more coding knowledge, especially for beginners. As always, the best method to use depends on your specific needs and the nature of your data.
Handling Errors with Python Lists
While creating a unique list in Python is generally straightforward, you may encounter some issues along the way. Let’s discuss some of these common issues and how to solve them.
Dealing with Unhashable Types
One issue you might come across is dealing with unhashable types. A hashable object has a hash value which never changes during its lifetime (see the hash()
function), and can be compared to other objects. In Python, mutable objects like lists or sets are unhashable, while immutable objects like integers, floats, strings, and tuples are hashable.
This becomes a problem when you try to create a set from a list that contains unhashable types, like another list or a set. Here’s an example:
my_list = [1, 2, [3, 4], 5]
try:
unique_list = list(set(my_list))
except TypeError as e:
print(e)
# Output:
# unhashable type: 'list'
In this example, my_list
contains another list, [3, 4]
. When we try to convert my_list
into a set, Python throws a TypeError
because lists are unhashable.
One solution to this problem is to convert the inner lists into tuples, which are hashable:
my_list = [1, 2, tuple([3, 4]), 5]
unique_list = list(set(my_list))
print(unique_list)
# Output:
# [1, 2, (3, 4), 5]
In this example, we first convert the inner list into a tuple using the tuple()
function. We can then successfully create a set from my_list
and convert it back into a list.
Remember, creating a unique list in Python can be a straightforward task, but it’s not without its quirks. Understanding these common issues and their solutions can save you a lot of time and frustration.
Hashability Explained in Python Lists
Before we delve deeper into creating unique lists in Python, it’s essential to understand Python’s list data type and the concept of hashability.
Understanding Python Lists
In Python, a list is a built-in data type that can store a collection of items. These items can be of any type and can be mixed. Lists are mutable, meaning you can change their content without changing their identity. You can create a list by placing items, separated by commas, inside square brackets []
.
Here’s an example of a Python list:
my_list = [1, 'two', 3.0, [4, 5]]
print(my_list)
# Output:
# [1, 'two', 3.0, [4, 5]]
In this example, my_list
is a list that contains an integer, a string, a float, and another list.
What is Hashability in Python?
The concept of hashability is central to understanding how Python creates unique lists. An object is hashable if it has a hash value that remains constant during its lifetime. You can retrieve an object’s hash value using the hash()
function:
my_int = 1
print(hash(my_int))
# Output:
# 1
In this example, we retrieve the hash value of an integer, which is the integer itself.
Python’s built-in set type uses hash values to quickly compare its elements. This is why sets automatically remove any duplicates: they simply refuse to add an element if another element with the same hash value is already present.
This also explains why mutable objects like lists or sets are unhashable: their content can change, so their hash value would change as well. Immutable objects like integers, floats, strings, and tuples, on the other hand, are hashable.
Understanding Python’s list data type and the concept of hashability is crucial when trying to create a unique list. As we’ve seen, creating a unique list is essentially a matter of removing duplicates, and Python uses hash values to identify duplicates.
Data Manipulation and Unique Lists
Creating a unique list is not just a coding exercise. It has practical applications in many areas, particularly in data manipulation in Python. When working with large datasets, you’ll often need to remove duplicates to get accurate results. This is where the techniques we’ve discussed come in handy.
Exploring Related Concepts
Creating a unique list is just one aspect of data manipulation in Python. There are many related concepts worth exploring, such as data cleaning and data analysis.
Data cleaning involves preparing your data for analysis by removing or modifying data that is incorrect, incomplete, irrelevant, duplicated, or improperly formatted. This is where creating a unique list often comes into play.
Data analysis, on the other hand, involves inspecting, cleansing, transforming, and modeling data with the goal of discovering useful information, informing conclusions, and supporting decision-making. It’s the next step after data cleaning and also benefits from having a unique list.
Further Resources for Mastering Python Lists
To deepen your understanding of Python lists and data manipulation, here are some resources you might find helpful:
- Python Lists: Techniques for Efficient Coding: Explore techniques for writing efficient code using Python lists, empowering you to improve your programming efficiency and proficiency.
Getting the Index of an Item in a List in Python: This guide explores different ways to retrieve the index of an item in a list in Python.
Joining a List into a String in Python: This tutorial discusses various methods for joining the elements of a list into a string in Python.
Python.org’s Python List Tutorial offers a comprehensive guide to Python’s list data type.
Python for Data Analysis by Wes McKinney, creator of the pandas library, is a great resource for anyone interested in data manipulation in Python.
Python Data Science Handbook by Jake VanderPlas covers a range of topics related to data science in Python, including data manipulation.
Remember, creating a unique list in Python is a valuable skill, but it’s also part of a larger context. By exploring related concepts and resources, you can become not just a better Python programmer, but a better data scientist.
Recap: Duplicate Items in Python Lists
In this comprehensive guide, we’ve delved into the process of creating unique lists in Python, from the basic to more advanced techniques. We’ve explored the importance of unique lists in Python and how mastering this task can significantly improve your data manipulation skills.
We began with the basics, learning how to create a unique list using the set method. We then progressed to more advanced techniques, such as list comprehension and NumPy’s unique function. We also explored alternative methods, including using the pandas library and creating a custom function.
Along the way, we tackled common issues you might encounter, such as dealing with unhashable types, and offered solutions to help you navigate these challenges. Additionally, we took a deep dive into the background and fundamentals of Python’s list data type and the concept of hashability.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Set Method | Simple, Quick | Unordered, Only for Hashable Types |
List Comprehension | Preserves Order, Flexible | More Complex |
NumPy’s Unique Function | Fast, Sorted Output | Requires NumPy, Output is an Array |
Pandas’ drop_duplicates Method | Handles DataFrames, Preserves Order | Requires Pandas |
Custom Function | Highly Customizable | Requires More Coding Knowledge |
Whether you’re a Python beginner or an experienced developer, we hope this guide has equipped you with the knowledge and skills to create unique lists in Python efficiently and effectively.
Creating a unique list is a fundamental task in Python and a crucial step in many data manipulation and data analysis tasks. With this guide, you’re now well-prepared to tackle these tasks head-on. Happy coding!