Bash Associative Arrays | How to Declare and Use Cases
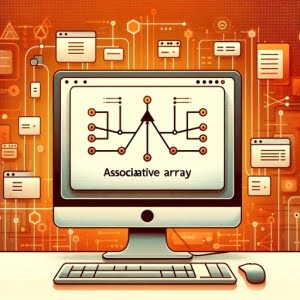
Are you finding it challenging to work with associative arrays in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling associative arrays in Bash, but we’re here to help.
Think of Bash’s associative arrays as a well-organized library – allowing us to store and retrieve data in an efficient manner, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with associative arrays in Bash, from their declaration, manipulation, to advanced usage. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
Let’s get started and master Bash associative arrays!
TL;DR: How Do I Use Associative Arrays in Bash?
To declare and use an associative array in Bash, you use the
declare -A
command followed by the array name, for exampledeclare -A sample_array
. You can then assign values to specific keys in the array. Here’s a simple example:
declare -A my_array
my_array["key"]="value"
echo ${my_array["key"]}
# Output:
# 'value'
In this example, we declare an associative array named my_array
. We then assign the value ‘value’ to the key ‘key’ in the array. When we echo the value of the key ‘key’, we get the output ‘value’.
This is just a basic way to use associative arrays in Bash, but there’s much more to learn about creating and manipulating associative arrays in Bash. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Bash Associative Arrays: Declaration and Basic Usage
- Advanced Bash Associative Arrays: Looping and Manipulation
- Exploring Alternatives to Bash Associative Arrays
- Troubleshooting Common Issues with Bash Associative Arrays
- Understanding Bash Arrays: Indexed vs Associative
- Applying Bash Associative Arrays in Larger Projects
- Wrapping Up: Mastering Bash Associative Arrays
Bash Associative Arrays: Declaration and Basic Usage
Bash’s associative arrays provide a powerful way to organize and access data. Let’s start by understanding how to declare, assign, and access values in an associative array in Bash.
Declaring an Associative Array
You declare an associative array using the declare -A
command followed by the array name. Here’s an example:
declare -A fruits
In this example, we have declared an associative array named fruits
.
Assigning Values to an Associative Array
Once you’ve declared an associative array, you can assign values to specific keys. Here’s how you can do it:
fruits["apple"]="red"
fruits["banana"]="yellow"
fruits["grape"]="purple"
In the above example, we’re assigning colors to different fruits in the fruits
associative array.
Accessing Values in an Associative Array
To access the value of a specific key in an associative array, you use the following syntax:
echo ${fruits["apple"]}
# Output:
# 'red'
In this example, we’re accessing the value of the key ‘apple’ in the fruits
associative array. The output is ‘red’, which is the color we assigned to ‘apple’.
Associative arrays in Bash are a powerful tool for organizing and accessing data. However, they can be a bit tricky to work with if you’re not familiar with their syntax. It’s important to remember to use the correct syntax when declaring, assigning, and accessing values in an associative array to avoid any potential pitfalls.
Advanced Bash Associative Arrays: Looping and Manipulation
Once you’ve got the hang of declaring, assigning, and accessing values in an associative array, it’s time to dive into more complex operations. These operations include looping over an array, accessing keys and values, and manipulating the array.
Looping Over an Associative Array
Bash allows you to loop over an associative array, which can be very useful when you need to perform an operation on each element in the array. Here’s how you can do it:
for key in ${!fruits[@]}; do
echo "The color of $key is ${fruits[$key]}"
done
# Output:
# 'The color of apple is red'
# 'The color of banana is yellow'
# 'The color of grape is purple'
In this example, we’re looping over the fruits
associative array and printing out the color of each fruit. The !fruits[@]
syntax is used to access all keys in the fruits
associative array.
Accessing Keys and Values
In addition to accessing individual keys and values, Bash allows you to access all keys or values in an associative array at once. Here’s how you can do it:
echo ${!fruits[@]}
# Output:
# 'apple banana grape'
In this example, we’re accessing all keys in the fruits
associative array at once. The output is ‘apple banana grape’.
Manipulating an Associative Array
Bash provides several ways to manipulate an associative array. For instance, you can remove a key-value pair from an associative array using the unset
command. Here’s an example:
unset fruits["apple"]
echo ${!fruits[@]}
# Output:
# 'banana grape'
In the above example, we’re removing the key-value pair for ‘apple’ from the fruits
associative array. When we echo all keys in the fruits
associative array, ‘apple’ is no longer present.
By understanding these advanced uses of associative arrays in Bash, you can handle more complex data structures and make your scripts more efficient and versatile.
Exploring Alternatives to Bash Associative Arrays
While associative arrays are a powerful tool in Bash, they are not the only way to handle complex data structures. There are alternative methods, like using indexed arrays or external tools like awk. Let’s explore these alternatives and discuss their pros and cons.
Indexed Arrays in Bash
Indexed arrays are a simpler form of arrays in Bash. They store a list of items, accessed by their numerical index. Here’s an example of how to use indexed arrays:
declare -a colors=('red' 'yellow' 'purple')
echo ${colors[0]}
# Output:
# 'red'
In this example, we declare an indexed array named colors
and assign it three values. We then access the first value in the array using its index (0). The output is ‘red’.
While indexed arrays are simpler and easier to use than associative arrays, they are also less flexible. You can only access values by their numerical index, not by a custom key.
Using awk for Data Manipulation
Awk is a powerful text-processing tool that can be used as an alternative to Bash associative arrays. Here’s an example of how you can use awk to process data:
echo -e 'apple red
banana yellow
grape purple' | awk '{print $1 " is " $2}'
# Output:
# 'apple is red'
# 'banana is yellow'
# 'grape is purple'
In this example, we’re using awk to process a tab-separated list of fruits and their colors. The output is a list of sentences stating the color of each fruit.
While awk is a powerful tool for data manipulation, it can be more complex and less intuitive than Bash associative arrays, especially for beginners.
In conclusion, while Bash associative arrays are a versatile tool for handling complex data structures, there are alternative methods available. The best method to use depends on your specific needs and your comfort level with each tool.
Troubleshooting Common Issues with Bash Associative Arrays
Working with associative arrays in Bash can sometimes lead to unexpected issues. Let’s discuss some common problems and their solutions, along with best practices for optimization.
Issue: Incorrect Declaration or Value Assignment
One common issue is incorrectly declaring an associative array or assigning a value to it. It’s crucial to use the correct syntax for these operations. For example, forgetting the -A
option when declaring an associative array can lead to issues.
declare my_array
my_array["key"]="value"
echo ${my_array["key"]}
# Output:
# ''
In this example, we forgot to include the -A
option when declaring the associative array. As a result, the value assignment doesn’t work as expected, and the output is an empty string.
Issue: Trying to Access Non-Existent Keys
Another common issue is trying to access a key that doesn’t exist in the associative array. Bash doesn’t give an error when you do this, but it can lead to unexpected behavior in your scripts.
declare -A my_array
my_array["key"]="value"
echo ${my_array["non_existent_key"]}
# Output:
# ''
In this example, we’re trying to access a key that doesn’t exist in the my_array
associative array. The output is an empty string, which might not be the expected behavior.
Best Practices and Tips for Optimization
When working with associative arrays in Bash, here are some best practices and tips for optimization:
- Always use the
-A
option when declaring an associative array. - Check whether a key exists in the associative array before trying to access its value.
- Use the
!
operator to access keys in an associative array. - Use the
unset
command to remove a key-value pair from an associative array.
By understanding these common issues and best practices, you can avoid potential pitfalls and make your Bash scripts more robust and efficient.
Understanding Bash Arrays: Indexed vs Associative
Before we delve deeper into associative arrays, it’s essential to understand the basic concept of arrays in Bash and the difference between indexed and associative arrays.
What are Arrays in Bash?
In Bash, an array is a variable that can hold multiple values. Each value is assigned a unique index that you can use to access the value. Here’s an example of an indexed array in Bash:
declare -a colors=('red' 'yellow' 'purple')
echo ${colors[1]}
# Output:
# 'yellow'
In this example, we declare an indexed array named colors
and assign it three values. We then access the second value in the array using its index (1). The output is ‘yellow’.
Indexed Arrays vs Associative Arrays
In an indexed array, the indices are numbers, whereas, in an associative array, the indices (or keys) are strings. This key-value pair structure allows for more flexibility in accessing and manipulating data.
declare -A fruits
fruits["apple"]="red"
echo ${fruits["apple"]}
# Output:
# 'red'
In this example, we declare an associative array named fruits
and assign a value to the key ‘apple’. We then access the value of ‘apple’ using its key. The output is ‘red’.
Why are Associative Arrays Useful?
Associative arrays are particularly useful when you need a more flexible data structure than indexed arrays can provide. They allow you to map keys to values, which can be especially helpful when the data you’re working with is more complex or when the relationships between elements are important.
By understanding the fundamentals of Bash arrays and the differences between indexed and associative arrays, you’ll be better equipped to use the right type of array for your specific needs.
Applying Bash Associative Arrays in Larger Projects
So far, we’ve explored the basics and advanced usage of associative arrays in Bash. But how do these concepts apply to larger scripts or projects? Let’s discuss that, along with related topics like file handling and data processing in Bash.
Integrating Associative Arrays in Scripts
Associative arrays can be incredibly useful in larger Bash scripts. They allow you to manage complex data structures and relationships, making your scripts more efficient and readable.
#!/bin/bash
declare -A user
user["name"]="John Doe"
user["email"]="[email protected]"
echo "User Details:"
echo "Name: ${user["name"]}"
echo "Email: ${user["email"]}"
# Output:
# User Details:
# Name: John Doe
# Email: [email protected]
In this script, we’re using an associative array to store and display user details. This approach can be scaled up for scripts that handle more complex data.
Associative Arrays in Data Processing
Associative arrays can also be used for data processing tasks in Bash. For example, you could use an associative array to count the frequency of words in a text.
declare -A word_count
while read -a words; do
for word in "${words[@]}"; do
((word_count["$word"]++))
done
done < file.txt
for word in "${!word_count[@]}"; do
echo "$word: ${word_count[$word]}"
done
# Output:
# word1: count1
# word2: count2
# ...
In this script, we’re reading words from a file and using an associative array to count their occurrences.
Further Resources for Bash Associative Array Mastery
To deepen your understanding of Bash associative arrays and their application in larger projects, here are some resources you might find helpful:
- GNU Bash Manual: The official GNU Bash manual is a comprehensive resource that covers all aspects of Bash scripting, including associative arrays.
- Bash Guide for Beginners: This guide provides a beginner-friendly introduction to Bash scripting, with a section dedicated to arrays.
- Advanced Bash-Scripting Guide: This guide goes into more depth on Bash scripting, including advanced topics like associative arrays.
Wrapping Up: Mastering Bash Associative Arrays
In this comprehensive guide, we’ve navigated the landscape of Bash associative arrays, an efficient and versatile tool for handling complex data structures in Bash.
We began with the basics, exploring how to declare, assign, and access values in an associative array. We then delved into more advanced usage, such as looping over an array, accessing keys and values, and manipulating the array. Along the way, we tackled common challenges you might face when working with associative arrays in Bash, offering solutions to help you overcome these hurdles.
We also examined alternatives to associative arrays, like indexed arrays and external tools like awk, giving you a broader view of the options available for handling data in Bash. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity |
---|---|---|
Associative Arrays | High | Moderate |
Indexed Arrays | Low | Low |
awk | High | High |
Whether you’re just starting out with Bash associative arrays or you’re looking to enhance your skills, we hope this guide has enriched your understanding and confidence in using associative arrays.
The ability to efficiently handle complex data structures is a powerful tool in Bash scripting. Now, you’re well equipped to harness the power of Bash associative arrays in your projects. Happy scripting!