How to Set and Use Environment Variables in Bash Script
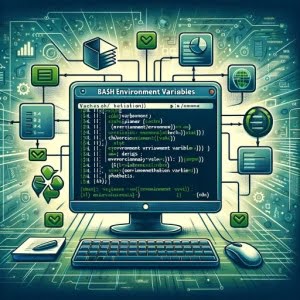
Ever found yourself puzzled about bash environment variables? You’re not the only one. Many developers find bash environment variables a bit mystifying. Think of bash environment variables as a backstage pass – they give you special access to certain system properties, allowing your scripts to interact with the system in powerful ways.
Bash environment variables are like the secret sauce that can add flavor to your bash scripts, making them more flexible and powerful. They can be used to store data that can be accessed by commands and scripts, making your scripts more dynamic and adaptable.
In this guide, we’ll walk you through the process of using and managing bash environment variables, from the basics to more advanced techniques. We’ll cover everything from setting and using variables, making them permanent, to using them in scripts and even troubleshooting common issues.
So, let’s dive in and start mastering bash environment variables!
TL;DR: How Do I Set and Use Bash Environment Variables?
To set a bash environment variable, you use the
export
command. You can then access this variable using the$
symbol. Here’s a simple example:
export VARNAME="Hello, World!"
echo $VARNAME
# Output:
# Hello, World!
In this example, we’ve used the export
command to set a bash environment variable named VARNAME
with the value ‘Hello, World!’. We then use the echo
command along with the $
symbol to access and print the value of VARNAME
, which outputs ‘Hello, World!’.
This is a basic way to set and use bash environment variables, but there’s much more to learn about managing and using them effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Bash Environment Variables: The Basics
- Setting Bash Environment Variables Permanently
- Using Environment Variables in Scripts
- Exploring Alternative Methods to Manage Bash Environment Variables
- Troubleshooting Common Issues with Bash Environment Variables
- Understanding the Fundamentals of Bash Environment Variables
- Bash Environment Variables: The Bigger Picture
- Wrapping Up: Mastering Bash Environment Variables
Understanding Bash Environment Variables: The Basics
Bash environment variables are key-value pairs that can be created and used in your shell sessions. They are essentially a way to store information that can be used by commands and scripts.
Let’s start with setting a simple bash environment variable. This can be done using the export
command followed by the variable name and its value. Here’s an example:
export GREETING="Hello, Bash!"
echo $GREETING
# Output:
# Hello, Bash!
In this example, we’ve set a variable named GREETING
with the value ‘Hello, Bash!’. The echo
command is then used to print the value of the variable. The $
symbol is used to access the value of the variable.
Setting and using bash environment variables like this can be very useful. For instance, they can be used to store data that you want to reuse throughout your script, like paths to important directories or configuration settings.
However, it’s important to note that variables set this way are only available in the current shell session. If you open a new terminal window or start a new session, the variable won’t be available. We’ll discuss how to make environment variables permanent in the next section.
Another potential pitfall to be aware of is that variable names are case-sensitive. So, GREETING
, Greeting
, and greeting
would be three different variables. Always ensure you’re using the correct case when setting and accessing your variables.
Setting Bash Environment Variables Permanently
While it’s useful to set environment variables for the current shell session, there are times when you might want a variable to persist across sessions or even after a system reboot. To make an environment variable permanent, you can add the export
command to the .bashrc
or .bash_profile
file in your home directory.
Here’s an example of how to add an environment variable to your .bashrc
file:
echo 'export GREETING="Hello, Bash!"' >> ~/.bashrc
source ~/.bashrc
echo $GREETING
# Output:
# Hello, Bash!
In this example, we’re using the echo
command to append our export
command to the .bashrc
file. The source
command is then used to reload the .bashrc
file, making our new environment variable available in the current session. The echo
command is then used to verify that the variable has been set correctly.
Using Environment Variables in Scripts
Bash environment variables can also be extremely useful in scripts. They can be used to store data that your script needs to function, such as configuration settings or file paths.
Here’s an example of a simple script that uses an environment variable:
#!/bin/bash
echo $GREETING
When you run this script, it will print the value of the GREETING
environment variable. If you’ve followed the previous steps and made the GREETING
variable permanent, this script will work in any new shell session.
Remember, the power of bash environment variables lies in their ability to make your scripts more dynamic and adaptable. By understanding and utilizing these concepts, you can write more efficient and flexible bash scripts.
Exploring Alternative Methods to Manage Bash Environment Variables
While using export
in your shell or scripts is a common approach, there are other methods to manage environment variables that can be more suitable depending on your specific needs.
Using Different Shell Environments
Different shell environments, like Zsh or Fish, have their own ways of setting environment variables. If you’re using Zsh, you can set environment variables in your .zshrc
file, similar to how you would do it in Bash.
echo 'export ZSH_VAR="Hello, Zsh!"' >> ~/.zshrc
source ~/.zshrc
echo $ZSH_VAR
# Output:
# Hello, Zsh!
In this example, we’re adding an environment variable to the .zshrc
file and then sourcing it. The variable is then available in your Zsh sessions.
Using Third-Party Tools
There are also third-party tools available that can help manage environment variables, such as direnv
or dotenv
. These tools can be particularly useful when working with projects that require different environment variables.
Here’s an example of how you might use direnv
:
First, install direnv
:
sudo apt install direnv
Then, in your project directory, create a .envrc
file with your environment variables:
echo 'export PROJ_VAR="Hello, Project!"' > .envrc
Finally, allow direnv
to load this file:
direnv allow .
# Output:
# direnv: loading .envrc
# direnv: export +PROJ_VAR
Now, PROJ_VAR
will be automatically set whenever you enter this directory, and unset when you leave.
Each of these methods has its own advantages and disadvantages. Using different shell environments can provide more powerful features, but requires learning a new shell. Third-party tools can make managing project-specific environment variables easier, but add an extra dependency to your project. It’s important to choose the method that best fits your needs.
Troubleshooting Common Issues with Bash Environment Variables
Working with bash environment variables can sometimes lead to unexpected results. Here are a few common issues that you may encounter and some tips on how to resolve them.
Variable is not Available in a New Shell Session
If you find that a variable you’ve set is not available in a new shell session, it’s likely because the variable was not set permanently. Variables set with the export
command are only available in the current shell session. To make a variable permanent, you can add the export
command to your .bashrc
or .bash_profile
file.
Here’s an example of how to add a variable to your .bashrc
file:
echo 'export NEW_VAR="Hello, New Session!"' >> ~/.bashrc
source ~/.bashrc
echo $NEW_VAR
# Output:
# Hello, New Session!
In this example, we’re adding a new environment variable to the .bashrc
file and sourcing it. The variable is then available in the current and future shell sessions.
Variable Name is Case-Sensitive
Bash environment variables are case-sensitive. This means that VAR
, Var
, and var
are three different variables. Always ensure that you’re using the correct case when setting and accessing your variables.
Here’s an example to illustrate this:
export Var="Hello, Bash!"
echo $Var
echo $VAR
echo $var
# Output:
# Hello, Bash!
#
#
In this example, we’ve set a variable named Var
and tried to access it using different cases. As you can see, only the correct case returns the value of the variable.
Remember, understanding how bash environment variables work can help you troubleshoot any issues you might encounter. With the right knowledge, you can use environment variables effectively to enhance your bash scripts.
Understanding the Fundamentals of Bash Environment Variables
At their core, environment variables are a type of dynamic-named value that can affect the way running processes behave on a computer. They exist in every operating system, and are used to simplify complex command inputs, store temporary values for shell scripts, and customize the shell environment.
In the context of bash, environment variables hold information about the shell environment, the user’s preferences, and the system. They are stored in a part of the system’s memory that holds key-value pairs. These pairs are called ‘variables’ and the name of the variable is the ‘key’.
A simple example of this is the PATH
environment variable. This variable holds a list of directories where executables are located:
echo $PATH
# Output:
# /usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
In this example, the echo
command is used to print the value of the PATH
variable. The output is a colon-separated list of directories that the system will search when looking for executables.
Understanding environment variables is crucial for bash scripting and command line usage. They allow you to customize your shell environment, store important data, and make your scripts more flexible and efficient. Mastering their usage can greatly enhance your abilities as a developer or system administrator.
Bash Environment Variables: The Bigger Picture
Bash environment variables are not just standalone elements; they play a significant role in larger scripts or projects. Their ability to store and manage information makes them an essential tool in shell scripting and process management.
Relevance in Larger Scripts
In larger scripts, bash environment variables can be used to store configuration settings, paths to important files or directories, and other data that needs to be accessed throughout the script. This can greatly simplify your scripts, making them more readable and maintainable.
For instance, consider a script that needs to work with a specific directory. Instead of hardcoding the directory path in every command that needs it, you can store the path in a bash environment variable at the start of the script:
#!/bin/bash
export DIR_PATH="/path/to/your/directory"
cd $DIR_PATH
ls
# Output:
# file1 file2 file3
In this script, we’ve set the DIR_PATH
variable to the path of the directory we’re interested in. We can then use this variable throughout the script whenever we need to refer to this directory.
Exploring Related Concepts
Understanding bash environment variables is just the tip of the iceberg. There are many related concepts that you might find interesting, such as shell scripting and process management.
Shell scripting is the process of writing scripts for the shell to execute. These scripts can automate tasks, manage files and processes, and perform many other tasks. Bash environment variables are a fundamental part of shell scripting, as they allow scripts to store and manage information.
Process management involves controlling and interacting with running processes on your system. Bash environment variables can be used to control the behavior of these processes, making them an important tool in process management.
Further Resources for Bash Environment Variables Mastery
To deepen your understanding of bash environment variables and related concepts, consider exploring these resources:
- GNU Bash Manual: This is the official manual for Bash. It provides in-depth information about all aspects of Bash, including environment variables.
Bash Scripting Tutorial: This tutorial provides a comprehensive introduction to bash scripting, including the use of environment variables.
Linux Process Management: This article provides an overview of process management in Linux, including how environment variables can be used to control processes.
Wrapping Up: Mastering Bash Environment Variables
In this comprehensive guide, we’ve unraveled the mystery of bash environment variables, shedding light on how to use and manage them effectively in your shell sessions and scripts.
We started with the basics, learning how to set and use bash environment variables. We then advanced to making these variables permanent and using them in scripts. We also explored common pitfalls and how to avoid them, ensuring a smooth journey in the world of bash environment variables.
We didn’t stop there. We ventured into the realm of alternative approaches, discussing different shell environments and third-party tools for managing environment variables. We also tackled common issues you might encounter when working with bash environment variables, providing you with practical solutions and workarounds.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Bash export | Simple, available in all bash sessions | Variables not permanent |
.bashrc or .bash_profile | Makes variables permanent | Requires editing shell configuration files |
Different shell environments | May provide more features | Requires learning a new shell |
Third-party tools | Simplifies managing project-specific variables | Adds an extra dependency |
Whether you’re a beginner just starting out with bash environment variables, or an experienced developer looking to brush up your skills, we hope this guide has enriched your understanding and enhanced your command over bash environment variables.
With bash environment variables, you can make your scripts more dynamic, your shell environment more customized, and your command line usage more efficient. Now, you’re well equipped to harness the power of bash environment variables. Happy scripting!