Python Set() | Data Type Usage Guide (With Examples)
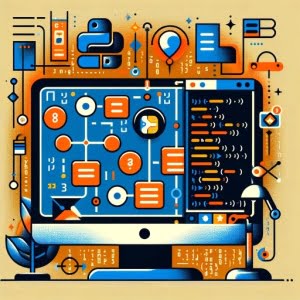
Ever felt muddled while working with sets in Python? Imagine a basket filled with distinct items; each one is unique and serves a purpose. Similarly, a set in Python is a collection of unique elements. In this guide, we will unravel the mystery of Python sets, helping you understand how to create and manipulate them – from basic to advanced usage.
Whether you’re a novice just starting out or a seasoned programmer looking to brush up on your Python skills, this guide will serve as a comprehensive resource for all things related to Python sets.
TL;DR: How Do I Create and Use a Python Set?
You can create a Python set by using the
set()
function or the{}
syntax. Here’s a simple example of creating a set:
fruits = {'apple', 'banana', 'cherry'}
print(fruits)
# Output:
# {'apple', 'banana', 'cherry'}
In this example, we created a set named ‘fruits’ containing three unique elements. We then printed the set, and as expected, it displayed all the elements in the set. However, it’s noteworthy that the order of elements in a Python set is not preserved, as sets are inherently unordered.
If you’re eager to delve deeper into Python sets, including their manipulation and advanced usage, keep reading!
Table of Contents
- Getting Started with Python Sets
- Exploring Set Operations in Python
- Alternative Ways to Handle Collections in Python
- Troubleshooting Common Python Set Issues
- Understanding the Concept of Sets in Computer Science
- Python Sets in Data Analysis and Machine Learning
- Expanding Your Knowledge
- Python Sets: A Recap and Beyond
Getting Started with Python Sets
Python sets are incredibly versatile and easy to use. Let’s break down the basics of creating a set, adding elements to it, and removing elements from it.
Creating a Python Set
The most straightforward way to create a set in Python is using the {}
syntax or the set()
function. Here’s how you can do it:
# Using {} syntax
fruits = {'apple', 'banana', 'cherry'}
print(fruits)
# Output:
# {'apple', 'banana', 'cherry'}
# Using set() function
vegetables = set(['carrot', 'peas', 'spinach'])
print(vegetables)
# Output:
# {'carrot', 'peas', 'spinach'}
In the first example, we used the {}
syntax to create a set named ‘fruits’. In the second example, we used the set()
function to create a set named ‘vegetables’. Both methods produced the same result: a set of unique elements.
However, keep in mind that when creating an empty set, you must use the set() syntax because {} by itself is used to create an empty dictionary.
Adding and Removing Elements
To add an element to a set, you can use the add()
function. To remove an element, you can use the remove()
function. Let’s look at an example:
# Adding an element
fruits.add('mango')
print(fruits)
# Output:
# {'apple', 'banana', 'cherry', 'mango'}
# Removing an element
fruits.remove('apple')
print(fruits)
# Output:
# {'banana', 'cherry', 'mango'}
In this example, we first added ‘mango’ to the ‘fruits’ set and then removed ‘apple’. As you can see, manipulating elements in a Python set is as simple as that.
Benefits and Pitfalls of Using Sets
Python sets are an efficient way to store unique elements and perform operations like union, intersection, and difference. However, it’s important to note that sets are unordered, meaning they don’t maintain the order in which elements are added. This can be a pitfall if your application requires maintaining an order of elements.
Exploring Set Operations in Python
Now that you’re familiar with the basics of Python sets, let’s dive into some advanced operations you can perform on them. Python sets support several powerful operations such as union, intersection, and difference.
Union Operation
The union operation combines the elements of two sets. In Python, you can perform a union operation using the union()
function or the |
operator. Here’s an example:
fruits = {'apple', 'banana', 'cherry'}
more_fruits = {'orange', 'pineapple', 'banana'}
# Using union() function
all_fruits = fruits.union(more_fruits)
print(all_fruits)
# Output:
# {'apple', 'banana', 'cherry', 'orange', 'pineapple'}
# Using | operator
all_fruits = fruits | more_fruits
print(all_fruits)
# Output:
# {'apple', 'banana', 'cherry', 'orange', 'pineapple'}
In both cases, we combined the ‘fruits’ and ‘more_fruits’ sets into a new set named ‘all_fruits’. Notice that ‘banana’, which was present in both original sets, only appears once in the union set. This is because sets only contain unique elements.
Intersection Operation
The intersection operation finds the common elements between two sets. You can perform this operation using the intersection()
function or the &
operator. Here’s how:
# Using intersection() function
common_fruits = fruits.intersection(more_fruits)
print(common_fruits)
# Output:
# {'banana'}
# Using & operator
common_fruits = fruits & more_fruits
print(common_fruits)
# Output:
# {'banana'}
In both examples, we found the common element ‘banana’ between the ‘fruits’ and ‘more_fruits’ sets.
Difference Operation
The difference operation finds the elements present in one set but not in another. You can perform this operation using the difference()
function or the -
operator. Let’s see it in action:
# Using difference() function
unique_fruits = fruits.difference(more_fruits)
print(unique_fruits)
# Output:
# {'apple', 'cherry'}
# Using - operator
unique_fruits = fruits - more_fruits
print(unique_fruits)
# Output:
# {'apple', 'cherry'}
In both cases, we found the elements ‘apple’ and ‘cherry’ that are present in the ‘fruits’ set but not in the ‘more_fruits’ set.
These operations provide a powerful way to manipulate and compare sets in Python, making them a versatile tool in your programming toolkit.
Alternative Ways to Handle Collections in Python
While sets are a powerful tool, Python offers other ways to handle collections, such as lists and dictionaries. Each has its own strengths and weaknesses, and understanding these can help you choose the best tool for your specific task.
Python Lists
A Python list is a collection of items that are ordered and changeable. Unlike sets, lists allow duplicate elements. Here’s a simple example of a list:
fruits_list = ['apple', 'banana', 'cherry', 'apple']
print(fruits_list)
# Output:
# ['apple', 'banana', 'cherry', 'apple']
In this example, we created a list named ‘fruits_list’ with four elements, including a duplicate ‘apple’. As you can see, lists preserve the order of elements and allow duplicates. However, this flexibility comes at a cost: operations like checking whether an item exists in the list can be slower than in a set, especially for large lists.
Python Dictionaries
A Python dictionary is a collection of key-value pairs that are unordered, changeable, and indexed. Dictionaries are incredibly useful when you want to associate items with a specific identifier or property. Here’s a simple example of a dictionary:
fruits_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
print(fruits_dict)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3}
In this example, we created a dictionary named ‘fruits_dict’ where each fruit (the key) is associated with a number (the value). Like sets, dictionaries in Python are implemented using hash tables, which provide fast access to items. However, dictionaries use more memory than sets or lists, and the keys in a dictionary must be immutable.
In conclusion, while sets are a great tool for handling collections of unique items, lists and dictionaries offer additional flexibility and functionality. Understanding these alternatives can help you write more efficient and effective Python code.
Troubleshooting Common Python Set Issues
Working with Python sets is generally straightforward, but there are some common issues that can trip up even experienced programmers. Let’s explore these pitfalls and how to avoid them.
Attempting to Add Mutable Elements
One common issue is trying to add mutable (changeable) elements, such as lists, to a set. This will result in a TypeError
, because set elements must be immutable (unchangeable). Here’s an example:
fruits = {'apple', 'banana', 'cherry'}
try:
fruits.add(['kiwi', 'melon'])
except TypeError as e:
print(e)
# Output:
# unhashable type: 'list'
In this example, we attempted to add a list of fruits to the ‘fruits’ set. However, this resulted in a TypeError
with the message ‘unhashable type: ‘list”. This is because lists are mutable and cannot be hashed, a requirement for set elements.
To avoid this issue, you can convert mutable elements to an immutable type, such as a tuple, before adding them to the set. Here’s how you can do it:
fruits = {'apple', 'banana', 'cherry'}
fruits.add(('kiwi', 'melon'))
print(fruits)
# Output:
# {'apple', 'banana', 'cherry', ('kiwi', 'melon')}
In this example, we first converted the list to a tuple, which is an immutable type, and then added it to the ‘fruits’ set. This time, the operation succeeded without any errors.
Understanding these common issues and their solutions can help you avoid potential pitfalls and make the most of Python sets.
Understanding the Concept of Sets in Computer Science
Before diving deeper into Python sets, it’s important to grasp the fundamental concept of sets in computer science. A set is a collection of distinct elements, often used to perform operations like union, intersection, and difference.
Sets in Computer Science
A set, in computer science, is an abstract data type that can store unique values, without any particular order. It is a concept derived from mathematics, where a set is a collection of distinct objects.
# A simple mathematical set
math_set = {1, 2, 3, 4, 5}
print(math_set)
# Output:
# {1, 2, 3, 4, 5}
In this example, we created a simple set of numbers. Notice that each number is unique and the set does not maintain any particular order.
Sets in Python: Unordered Collections of Unique Elements
In Python, sets are implemented in a way that mimics the mathematical concept of sets. They are collections of unique elements and are unordered. This means that, unlike lists or tuples, sets do not record element position or order of insertion, and so do not support indexing, slicing, or other sequence-like behavior.
# A Python set
python_set = {'apple', 'banana', 'cherry'}
print(python_set)
# Output:
# {'apple', 'banana', 'cherry'}
In this example, we created a Python set of fruits. Each fruit is a unique element, and the set does not maintain any specific order.
Understanding the fundamentals of sets in computer science and their implementation in Python is key to effectively using Python sets and leveraging their unique properties in your code.
Python Sets in Data Analysis and Machine Learning
Python sets are not just limited to simple operations; they play a significant role in advanced fields like data analysis and machine learning.
Sets in Data Analysis
In data analysis, Python sets are used for tasks like identifying unique items in a dataset or comparing different datasets. For example, if you have a list of user IDs and want to find out how many unique users there are, you can simply convert the list to a set and get its length.
user_ids = [1, 2, 2, 3, 4, 4, 4, 5, 5, 5]
unique_users = set(user_ids)
print(len(unique_users))
# Output:
# 5
In this example, we have a list of user IDs with some duplicates. By converting the list to a set, we can easily find out the number of unique user IDs.
Sets in Machine Learning
In machine learning, Python sets can be used to handle categorical data. For instance, if you have a dataset with a categorical feature like ‘color’ that takes values like ‘red’, ‘green’, and ‘blue’, you can use a set to find out all the unique colors.
colors = ['red', 'green', 'blue', 'red', 'blue', 'blue']
unique_colors = set(colors)
print(unique_colors)
# Output:
# {'red', 'green', 'blue'}
In this example, we have a list of colors with some duplicates. By converting the list to a set, we can easily find out all the unique colors.
Expanding Your Knowledge
To further enhance your understanding of Python sets, consider the following articles on related concepts:
- Python Data Types: Understanding Basics – Explore dictionary manipulation techniques for your data handling processes.
Simplifying Queue and Stack Implementation with deque – Learn how to use deque in Python for thread-safe data manipulation.
Understanding Array Length in Python – Master Python array length computation to optimize your code for performance.
Python 2 Sets Official Documentation – Deep dive into sets in Python 2 from the official resources.
Python Sets YouTube Tutorial – Watch and learn about Python sets.
W3Schools’ Python Sets Tutorial is a great guide to using sets in Python.
Remember, the best way to learn is by doing, so don’t hesitate to get your hands dirty and start coding!
Python Sets: A Recap and Beyond
In this comprehensive guide, we’ve explored Python sets, a powerful tool for handling collections of unique elements. We started with the basics of creating a set, adding elements to it, and removing elements. We saw how easy it is to manipulate Python sets and discussed the benefits and potential pitfalls of using them.
# Recap of basic set operations
fruits = {'apple', 'banana', 'cherry'}
fruits.add('mango')
fruits.remove('apple')
print(fruits)
# Output:
# {'banana', 'cherry', 'mango'}
In this code block, we recap the basic operations of adding and removing elements in a Python set.
We then delved into advanced set operations such as union, intersection, and difference. These operations provide a powerful way to manipulate and compare sets in Python.
# Recap of advanced set operations
all_fruits = fruits.union({'orange', 'pineapple'})
common_fruits = fruits.intersection({'banana', 'pineapple'})
unique_fruits = fruits.difference({'banana', 'pineapple'})
print(all_fruits, common_fruits, unique_fruits)
# Output:
# {'banana', 'cherry', 'mango', 'orange', 'pineapple'} {'banana'} {'cherry', 'mango'}
In this code block, we recap the advanced operations of union, intersection, and difference in a Python set.
We also discussed common issues when working with sets, such as attempting to add mutable elements, and provided solutions to these problems. Moreover, we looked at alternative ways to handle collections in Python, such as using lists or dictionaries, and compared their advantages and disadvantages.
Finally, we touched upon the relevance of Python sets in data analysis and machine learning, and suggested exploring related concepts for a deeper understanding.
In conclusion, Python sets are a versatile tool in your programming toolkit, whether you’re handling unique items in a collection, comparing datasets, or dealing with categorical data in machine learning. Happy coding!