Getter and Setter in Java: Your Ultimate Guide
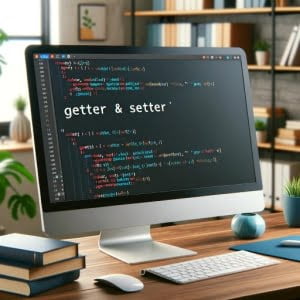
Are you finding it hard to understand getters and setters in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling getters and setters in Java, but we’re here to help.
Think of Java’s getters and setters as gatekeepers – they control access to a class’s properties, ensuring data integrity and encapsulation. They are a fundamental part of Java’s object-oriented programming paradigm.
In this guide, we’ll walk you through the process of using getters and setters in Java, from their creation, manipulation, and usage. We’ll cover everything from the basics of these methods to more advanced techniques, as well as alternative approaches.
Let’s get started and master getters and setters in Java!
TL;DR: What Are Getters and Setters in Java and How Do I Use Them?
Getters and setters in Java are methods used to retrieve and modify the value of a class’s private variable. They are normally defined with the syntax,
public String getValue() {return value;}
while setters are defined with the code,public void setValue(String value) {this.value = value;
They are a fundamental part of Java’s object-oriented programming, providing a way to control access to a class’s properties.
Here’s a simple example:
class Person {
private int age;
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
# Output:
# If the name was set to '42', getName() will return '42'
In this example, we’ve created a Person
class with a private variable age
. The getAge()
method (getter) retrieves the value of age
, and the Age(int age)
method (setter) modifies the value of age
. If we set the name to ’42’, calling getAge()
will return ’42’.
This is a basic way to use getters and setters in Java, but there’s much more to learn about these methods. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Getters and Setters in Java
- Advanced Usage of Getters and Setters in Java
- Exploring Alternative Approaches to Data Access
- Troubleshooting Common Issues with Getters and Setters
- Delving into Encapsulation in Java
- The Relevance of Getters and Setters in Larger Java Projects
- Wrapping Up: Getters and Setters in Java
Getting Started with Getters and Setters in Java
In Java, getters and setters play a crucial role in data encapsulation, a core concept of object-oriented programming. They provide a way to access and modify the data inside a class.
Creating Getters and Setters
To create a getter and setter in Java, you define two methods within your class. The getter method returns the value of the variable, while the setter method sets or updates that value.
Here’s how you can create a getter and setter for a name
variable in a Person
class:
class Person {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, getName()
is the getter method for name
, and setName(String name)
is the setter. The name
variable is private, which means it can’t be accessed directly from outside the Person
class. However, the getter and setter methods are public, allowing controlled access to name
.
Advantages of Using Getters and Setters
Using getters and setters has several advantages:
- Encapsulation: They help to hide the internal implementation of the class and protect the data.
- Control over access: They provide control over how a field is accessed or updated.
- Flexibility: They allow the class to change its internal implementation without affecting other parts of the code that use the class.
Potential Pitfalls
While getters and setters are very helpful, there are some potential pitfalls to be aware of:
- Overuse: Using getters and setters for every property can lead to ‘anemic’ models where the class is only used for storing data and does not contain any business logic.
- Data consistency: If multiple setters modify the same data, it can lead to inconsistent state of the object.
In the next section, we’ll dive into more complex uses of getters and setters in Java.
Advanced Usage of Getters and Setters in Java
As you gain more experience with Java, you’ll encounter scenarios that require a more complex use of getters and setters. They can be employed with different types of variables such as arrays, lists, and even in different contexts like inheritance and interfaces.
Getters and Setters with Arrays and Lists
Consider a Student
class that contains a list of grades. We can use getters and setters to manipulate this list.
class Student {
private List<Integer> grades;
public List<Integer> getGrades() {
return grades;
}
public void setGrades(List<Integer> grades) {
this.grades = grades;
}
}
# Output:
# If the grades were set to [90, 85, 95], getGrades() will return [90, 85, 95]
In this example, getGrades()
returns the list of grades, and setGrades(List grades)
sets the grades. If we set the grades to [90, 85, 95], calling getGrades()
will return [90, 85, 95].
Getters and Setters in Inheritance
In Java, a subclass inherits all the public and protected members of its parent class. So, if a getter or setter is defined in the parent class, it can be used in the subclass.
class Person {
protected String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
class Student extends Person {
private int studentID;
public int getStudentID() {
return studentID;
}
public void setStudentID(int studentID) {
this.studentID = studentID;
}
}
# Output:
# If the name was set to 'John' and studentID was set to 123, getName() will return 'John' and getStudentID() will return 123
In this example, Student
is a subclass of Person
. It inherits the name
variable and its getter and setter from Person
. It also has its own studentID
variable with its corresponding getter and setter. If we set the name to ‘John’ and the studentID to 123, calling getName()
will return ‘John’ and getStudentID()
will return 123.
In the next section, we’ll explore alternative approaches to handle access to a class’s properties.
Exploring Alternative Approaches to Data Access
While getters and setters are a common way to control access to a class’s properties in Java, there are other strategies you can employ as well. These include using the ‘protected’ keyword and creating public methods that perform more complex operations.
Leveraging the ‘Protected’ Keyword
The protected
keyword in Java creates a field that is accessible within the same package and also by the subclasses of its class in any package. This can sometimes be a suitable alternative to using private fields with getters and setters.
class Person {
protected String name;
}
class Employee extends Person {
public void printName() {
System.out.println(name);
}
}
# Output:
# If the name was set to 'John' in the Employee class, printName() will print 'John'
In this example, the name
field in the Person
class is declared as protected
. The Employee
class, which extends Person
, can directly access the name
field without needing a getter or setter.
Using Public Methods for Complex Operations
Sometimes, you might want to perform a more complex operation when getting or setting a property. In such cases, you can create a public method to handle this.
class Circle {
private double radius;
public double getArea() {
return Math.PI * radius * radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
}
# Output:
# If the radius was set to 3, getArea() will return approximately 28.27
In this example, instead of a simple getter for the radius
field, we’ve created a getArea()
method that returns the area of the circle. This method performs a complex operation (calculating the area) using the radius
field.
These alternative approaches offer different benefits and drawbacks. The ‘protected’ keyword can simplify your code by eliminating the need for getters and setters, but it also makes your fields more accessible, which could potentially compromise data integrity. On the other hand, using public methods for complex operations can provide more functionality but might also make your code more complex. The best approach depends on the specific requirements of your project.
Troubleshooting Common Issues with Getters and Setters
Despite their usefulness, getters and setters in Java can sometimes lead to unexpected issues. Let’s discuss some of these problems and how to resolve them.
Null Pointer Exceptions
One common issue is the Null Pointer Exception. This error occurs when you call a method or access a property on a null object. Here’s an example:
class Person {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Person person = null;
String name = person.getName();
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’re trying to call the getName()
method on a null Person
object, which throws a NullPointerException
. To avoid this, always ensure that the object is not null before calling methods on it.
Data Consistency Issues
Another common issue arises when multiple threads access and modify the same data concurrently, leading to data inconsistency. For instance, if two threads simultaneously call a setter method to modify the same field, one update might overwrite the other.
To prevent this, you can synchronize the getter and setter methods:
class Person {
private String name;
public synchronized String getName() {
return name;
}
public synchronized void setName(String name) {
this.name = name;
}
}
In this example, the getName()
and setName(String name)
methods are synchronized
, which means only one thread can access them at a time. This ensures that the name
field can’t be updated by one thread while another is reading it.
Understanding these issues and solutions can help you use getters and setters more effectively in your Java programming. In the next section, we’ll delve into the fundamentals of encapsulation in Java and how getters and setters fit into this concept.
Delving into Encapsulation in Java
Encapsulation is one of the four fundamental principles of object-oriented programming (OOP), along with inheritance, polymorphism, and abstraction. It plays a pivotal role in why we use getters and setters in Java.
Encapsulation: The Pillar of Data Protection
Encapsulation is a mechanism that binds together code and the data it manipulates, and keeps both safe from outside interference and misuse. In Java, encapsulation is achieved by declaring the variables of a class as private and providing public getter and setter methods to manipulate those variables.
class Person {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
# Output:
# If the name was set to 'John', getName() will return 'John'
In this example, the name
variable is encapsulated in the Person
class. It’s marked as private, which means it can’t be accessed directly from outside this class. To access and modify name
, we use the public methods getName()
and setName(String name)
, respectively. If we set the name to ‘John’, calling getName()
will return ‘John’.
Encapsulation and Getters/Setters: A Perfect Match
Getters and setters are instrumental in encapsulation. They provide a way to access a class’s private fields indirectly. Without getters and setters, we would have to make our fields public to allow access, which could compromise data integrity.
By using getters and setters, we can control what gets passed to our fields and how our data is accessed. This is a key aspect of encapsulation and a fundamental part of creating robust, secure Java applications.
The Relevance of Getters and Setters in Larger Java Projects
As your Java projects grow, the importance of getters and setters becomes more evident. They play a critical role in maintaining data integrity and encapsulation, which are key to developing robust, scalable applications.
Getters and Setters in GUI Programming
In GUI programming, getters and setters are often used to control the data displayed on the screen. For example, a setter might be used to update the text of a label, while a getter could be used to retrieve the current value of a text field.
Getters and Setters for Database Access
When working with databases in Java, getters and setters can be used to handle the data that’s being read from or written to the database. You can use setters to prepare the data to be inserted into the database, and getters to retrieve the data from the database and use it in your application.
Exploring Related Concepts
Understanding getters and setters can also pave the way for you to explore related concepts in Java, like inheritance, interfaces, and polymorphism. These are all fundamental aspects of object-oriented programming and can help you write more efficient, maintainable code.
Further Resources for Mastering Getters and Setters in Java
Ready to take your understanding of getters and setters to the next level? Here are some resources that can help:
- Java Object: A Quick Overview – Discover the versatility of objects for modeling complex systems in Java.
Exploring instanceof Operator Usage – Learn type checking and polymorphic behavior with instanceof in Java programs.
Singleton Class in Java Overview – Learn about the Singleton design pattern in Java.
Oracle’s Java Tutorials covers all aspects of Java programming, including getters and setters.
Java Code Geeks offers a wealth of Java programming resources, including articles on getters and setters.
Geeks for Geeks Java Library also offers in-depth articles on various topics, including getters and setters.
Wrapping Up: Getters and Setters in Java
In this comprehensive guide, we’ve delved into the world of getters and setters in Java, exploring their purpose, usage, and the common issues you might encounter.
We began with the basics, understanding the role of getters and setters in Java and how to use them. We then delved into more advanced usage, exploring how getters and setters can be used with different types of variables and in different contexts like inheritance and interfaces. We also discussed alternative approaches to controlling access to a class’s properties, such as using the ‘protected’ keyword and creating public methods that perform more complex operations.
Throughout this journey, we’ve tackled common issues you might face when using getters and setters, such as null pointer exceptions and data consistency issues, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Getters and Setters | Encapsulation, Control over access, Flexibility | Overuse, Data consistency issues |
‘Protected’ Keyword | Simplifies code, Eliminates need for getters and setters | More accessible fields, Potential compromise of data integrity |
Public Methods for Complex Operations | Provides more functionality | Can make code more complex |
Whether you’re just starting out with getters and setters in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of getters and setters and their role in Java programming.
Understanding getters and setters is crucial for maintaining data integrity and encapsulation in your Java applications. Now, you’re well equipped to harness the power of getters and setters in your Java projects. Happy coding!