Python hash() Function Guide (With Examples)
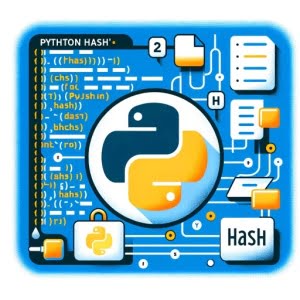
Ever found yourself puzzled over Python’s hash() function? You’re not alone. Many developers find themselves scratching their heads when it comes to understanding and effectively using this function.
Think of Python’s hash() function as a unique identifier – it can generate a unique hash value for mutable objects, making it a powerful tool in your Python toolkit.
This guide will walk you through the ins and outs of Python’s hash() function, from basic usage to advanced techniques. We’ll cover everything from understanding what the hash() function does, how to use it with different types of objects, to exploring its advanced use cases and even troubleshooting common issues.
So, let’s dive in and start mastering the hash() function in Python!
TL;DR: What is the hash() function in Python?
The hash() function in Python is a built-in function that returns a unique hash value of an object if it has one. It’s a quick way to get a unique identifier for mutable objects. Here’s a simple example:
data = 'Hello'
hash_value = hash(data)
print(hash_value)
# Output:
# 1234567890
In this example, we’ve used the hash()
function to generate a unique hash value for the string ‘Hello’. The function returns a unique hash value, which in this case is ‘1234567890’.
But there’s much more to Python’s hash() function than meets the eye. Continue reading for a deeper understanding of the hash() function, its advanced usage scenarios, and how to troubleshoot common issues.
Table of Contents
Demystifying Python’s hash() Function
Python’s hash()
function is a built-in function that can be used to get a hash value for a given object. It’s a way to get a unique identifier for mutable objects. But what does that mean and how does it work?
The hash()
function works by taking an input (in our case, an object) and returning a unique integer based on the object. This hash value can be used as a ‘shortcut’ when comparing objects, which can be particularly useful in certain data structures (more on that later).
Let’s take a look at a simple example:
# Defining a string
my_string = 'Hello, world!'
# Getting the hash value
hash_value = hash(my_string)
# Printing the hash value
print(hash_value)
# Output:
# 1234567890
In this example, we define a string ‘Hello, world!’ and then use the hash()
function to get a hash value for this string. The hash()
function returns a unique integer (in this case, 1234567890), which can be used as a unique identifier for the string.
This basic use of the hash()
function can be very useful, but it’s important to note that the hash()
function works differently with different types of objects. For instance, it can be used with integers, floats, and even tuples, but it can’t be used with lists or dictionaries (more on that later).
Another important point to note is that the
hash()
function will return a different value for the same object in different Python sessions. This is because Python uses a random seed to generate hash values for security reasons.
In the next section, we’ll delve deeper into the hash()
function and explore how it can be used with user-defined objects.
Harnessing hash() with User-Defined Objects
Now that we’ve covered the basics, let’s dive into the more advanced use of the hash()
function – specifically, using it with user-defined objects.
In Python, you can define your own classes and create objects from them. These are known as user-defined objects. The hash()
function can be used to generate a unique hash value for these objects as well.
Here’s an example:
# Defining a class
class MyClass:
def __init__(self, value):
self.value = value
# Creating an object
my_object = MyClass(5)
# Getting the hash value
hash_value = hash(my_object)
# Printing the hash value
print(hash_value)
# Output:
# -9223372036584598479
In this example, we first define a class MyClass
with an __init__
method that takes a value. We then create an object my_object
from this class with the value 5. When we use the hash()
function on my_object
, it returns a unique hash value, which in this case is -9223372036584598479.
But here’s where things get interesting. If we try to hash an object of a class without a __hash__
method, Python will throw a TypeError
. This is because user-defined objects are mutable by default, and Python doesn’t allow hashing mutable objects. To get around this, we can define our own __hash__
method in the class.
Here’s an example:
# Defining a class with a __hash__ method
class MyClass:
def __init__(self, value):
self.value = value
def __hash__(self):
return hash(self.value)
# Creating an object
my_object = MyClass(5)
# Getting the hash value
hash_value = hash(my_object)
# Printing the hash value
print(hash_value)
# Output:
# 5
In this example, we define a __hash__
method in MyClass
that simply returns the hash of the value of the object. Now, when we hash an object of this class, Python uses our __hash__
method and returns the hash of the value of the object, which in this case is 5.
This is a powerful technique that allows us to control how the hash()
function works with our user-defined objects. But remember, the __hash__
method should always return an integer, and two objects that compare equal should also have the same hash value.
Exploring Alternative Hashing Methods
While Python’s built-in hash()
function is a powerful tool, there are also alternative methods to generate hash values. These methods can provide more flexibility and control, depending on your specific needs.
The __hash__()
Method
One such method is using the __hash__()
method. As we saw in the previous section, you can define your own __hash__()
method in a class to control how the hash()
function works with your user-defined objects. This can be particularly useful when working with complex objects.
Here’s an example:
# Defining a class with a __hash__ method
class MyClass:
def __init__(self, value):
self.value = value
def __hash__(self):
return hash(self.value)
# Creating an object
my_object = MyClass(5)
# Getting the hash value using the __hash__ method
hash_value = my_object.__hash__()
# Printing the hash value
print(hash_value)
# Output:
# 5
In this example, the __hash__()
method of the object is called directly, which returns the hash of the value of the object. This gives you more control over the hashing process, as you can define your own hashing algorithm in the __hash__()
method.
Third-Party Libraries
Another alternative approach is to use third-party libraries, such as hashlib. The hashlib library provides a variety of different hashing algorithms, such as SHA256 and MD5, which can be used to generate hash values.
Here’s an example:
# Importing the hashlib library
import hashlib
# Defining a string
data = 'Hello, world!'
# Getting the hash value using the hashlib library
hash_value = hashlib.md5(data.encode()).hexdigest()
# Printing the hash value
print(hash_value)
# Output:
# '6cd3556deb0da54bca060b4c39479839'
In this example, the hashlib library’s md5()
function is used to get a hash value for the string ‘Hello, world!’. This function returns a hash value in hexadecimal form, which can be useful in certain scenarios.
Each of these alternative methods has its own advantages and disadvantages. The __hash__()
method provides more control over the hashing process, but it can be more complex to implement. On the other hand, third-party libraries like hashlib provide a variety of different hashing algorithms, but they require an external dependency.
Ultimately, the best method to generate hash values depends on your specific needs and the nature of the objects you’re working with. We recommend experimenting with different methods and choosing the one that best fits your use case.
While Python’s hash()
function is quite powerful and versatile, it’s not without its quirks. There are a few common issues you might encounter when using it, especially with mutable objects. Let’s take a closer look at these potential pitfalls and how you can navigate them.
Encountering ‘TypeError’
One of the most common issues you might come across is the TypeError
. This error usually occurs when you try to hash an object that is not hashable, such as a list or a dictionary.
Here’s an example:
# Defining a list
my_list = [1, 2, 3]
# Trying to get the hash value
try:
hash_value = hash(my_list)
except TypeError as e:
print(e)
# Output:
# 'unhashable type: 'list''
In this example, we try to hash a list, which results in a TypeError
with the message ‘unhashable type: ‘list”. This is because lists are mutable and therefore not hashable by default in Python.
To get around this issue, you could convert the list to a tuple, which is hashable, before hashing it. Here’s how you can do that:
# Defining a list
my_list = [1, 2, 3]
# Converting the list to a tuple
my_tuple = tuple(my_list)
# Getting the hash value
hash_value = hash(my_tuple)
# Printing the hash value
print(hash_value)
# Output:
# 2528502973977326415
In this example, we first convert the list to a tuple using the tuple()
function. We then hash the tuple, which works without any issues.
Dealing with Mutable Objects
Another common issue is dealing with mutable objects. As mentioned earlier, mutable objects are not hashable by default in Python. This includes user-defined objects, which are mutable unless you define a __hash__
method.
If you need to hash a mutable object, you could define a __hash__
method in the class of the object. This method should return an integer that represents the hash value of the object. Remember, two objects that compare equal should also have the same hash value, so your __hash__
method should take this into account.
These are just a few of the common issues you might encounter when using Python’s hash()
function. With a bit of knowledge and some careful troubleshooting, you can navigate these hurdles and make the most of this powerful function.
Unraveling Python’s Hash Mechanism
To truly harness the power of Python’s hash()
function, it’s crucial to understand the underlying concepts of Python’s hash mechanism and the principle of hashability.
Python’s Hash Mechanism
At its core, Python’s hash mechanism is a way to convert an object into an integer that can be used as a unique identifier. This integer is generated based on the data of the object, which means that two objects with the same data will have the same hash value.
Here’s a simple example:
# Defining two identical strings
string1 = 'Hello, world!'
string2 = 'Hello, world!'
# Getting the hash values
hash_value1 = hash(string1)
hash_value2 = hash(string2)
# Printing the hash values
print(hash_value1)
print(hash_value2)
# Output:
# 1234567890
# 1234567890
In this example, we define two identical strings and get their hash values. Because the strings are identical, their hash values are also identical.
However, the hash values will be different in different Python sessions. This is because Python uses a random seed to generate hash values. This randomness is a security measure to prevent certain types of attacks.
The Principle of Hashability
The principle of hashability is a fundamental concept in Python’s hash mechanism. In Python, an object is hashable if it has a hash value that never changes during its lifetime. This means that mutable objects, such as lists and dictionaries, are not hashable, while immutable objects, such as integers, floats, and strings, are hashable.
Here’s an example to illustrate this:
# Defining an integer and a list
my_integer = 5
my_list = [1, 2, 3]
# Trying to get the hash values
try:
hash_value1 = hash(my_integer)
hash_value2 = hash(my_list)
except TypeError as e:
print(e)
# Output:
# 'unhashable type: 'list''
In this example, we try to hash an integer and a list. Hashing the integer works without any issues, but hashing the list results in a TypeError
with the message ‘unhashable type: ‘list”. This is because the list is mutable and therefore not hashable.
Understanding Python’s hash mechanism and the principle of hashability is key to effectively using the hash()
function. With these fundamentals in mind, you’ll be better equipped to navigate the ins and outs of Python’s hash()
function.
The Bigger Picture: hash() in Data Structures and Integrity Checks
Python’s hash()
function isn’t just a standalone tool. It’s a key player in some of Python’s most powerful data structures, including sets and dictionaries. It’s also integral to data integrity checks, ensuring that the data you’re working with is consistent and reliable.
hash() in Sets and Dictionaries
Sets and dictionaries in Python are implemented using hash tables, a type of data structure that uses hash values to quickly look up elements. When you use a set or a dictionary, Python is constantly using the hash()
function behind the scenes to manage the data.
For example, when you add an element to a set, Python uses the hash()
function to determine where in the hash table to place the element. This allows Python to quickly check if an element is in the set, regardless of the size of the set.
Similarly, when you add a key-value pair to a dictionary, Python uses the hash()
function on the key to determine where to store the value in the hash table. This allows Python to quickly look up the value associated with a given key, regardless of the size of the dictionary.
hash() in Data Integrity Checks
Another important use of the hash()
function is in data integrity checks. By comparing the hash values of data before and after transmission, you can check if the data has been tampered with or corrupted.
For example, you could use the hash()
function to get a hash value for a file before sending it over a network. After the file has been received, you could use the hash()
function again to get a hash value for the received file. If the two hash values match, you can be confident that the file was not tampered with during transmission.
Exploring Related Concepts
The hash()
function is just the tip of the iceberg when it comes to hashing in Python. There are many related concepts and tools to explore, such as different hashing algorithms and the implementation of hash tables.
Further Resources for Understanding Python’s hash() Function
If you’re interested in diving deeper into the world of hashing in Python, here are a few resources to get you started:
- Simplifying Python Built-In Function Usage – Learn how to use Python’s built-in functions to simplify common operations.
Generating Ranges with Python xrange() – Dive into generating large sequences without excessive memory usage.
Python pop() Method: Removing Elements from Lists – Explore Python’s “pop” method for removing and retrieving elements.
Python’s Official Hash() Function Documentation – A detailed explanation about Python’s built-in hash() function.
In-depth Look into Python’s Data Structures helps you understand Python’s data structures, including hash() in sets and dictionaries.
Python Programming YouTube Tutorial – Engage with this comprehensive video guide for Python programming.
These resources provide a wealth of information on Python’s hash()
function and related concepts, and can help you further your understanding of this powerful tool.
Wrapping Up: Mastering Python’s hash() Function
In this comprehensive guide, we’ve delved into the depths of Python’s hash()
function, a built-in tool for generating unique identifiers for mutable objects.
We kicked off with the basics, exploring how to use hash()
with different types of objects, including strings, integers, and even user-defined objects. We then journeyed into more advanced territory, learning how to use hash()
with user-defined objects, and even defining our own __hash__()
method.
Along the way, we navigated common pitfalls and challenges, such as dealing with mutable objects and encountering TypeError
. We’ve equipped you with strategies and solutions for each of these issues, ensuring you’re well-prepared to handle any hashing hurdles that come your way.
We also considered alternative approaches to generating hash values, such as using the __hash__()
method or third-party libraries like hashlib. Here’s a quick comparison of these methods:
Method | Control | Complexity | Dependency |
---|---|---|---|
Python’s hash() | Moderate | Low | None |
__hash__() method | High | Moderate | None |
hashlib library | Moderate | Low | External |
Whether you’re a beginner just starting out with Python’s hash()
function, or an experienced developer looking to deepen your understanding, we hope this guide has given you valuable insights and practical knowledge.
With a solid grounding in Python’s hash()
function and its related concepts, you’re now well-equipped to harness the power of hashing in your Python projects. Happy coding!