Python 2’s xrange() Function: Historical Guide
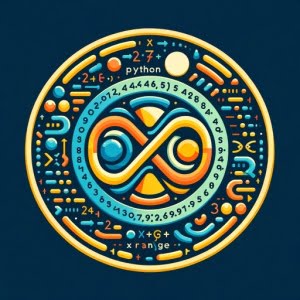
Are you finding it challenging to understand Python’s xrange function? You’re not alone. Many developers find themselves puzzled when it comes to handling large ranges in Python 2, but we’re here to help.
Think of Python’s xrange as a marathon runner pacing themselves – it’s a tool that helps control memory usage when dealing with large ranges. It’s a handy utility that can make your Python 2 code more efficient and memory-friendly.
This guide will walk you through using xrange in Python 2, from its basic usage to more advanced techniques. We’ll cover everything from the basics of xrange to its role in memory-efficient programming and large-scale data processing.
So, let’s dive in and start mastering xrange!
TL;DR: What is xrange in Python?
In Python 2, xrange() is a built-in function that generates an object producing numbers within a specified range. It’s similar to the range function, but more memory efficient when dealing with large ranges.
Here’s a simple example:
for i in xrange(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, we’re using the xrange function to generate numbers from 0 up to, but not including, 5. The function prints each number in turn, resulting in the output shown.
This is just a basic usage of xrange in Python 2. For a deeper understanding and more advanced usage scenarios, continue reading.
Table of Contents
Understanding Python’s xrange: Basic Usage
The xrange function in Python 2 is a built-in function used to generate a sequence of numbers within a specified range. Unlike the range function, which creates a list containing the entire sequence and stores it in memory, xrange creates an object that generates the numbers on the fly. This makes xrange more memory-efficient, especially when dealing with large ranges.
Here’s a simple example of how the xrange function works:
for i in xrange(10):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
# 5
# 6
# 7
# 8
# 9
In this example, we’re using the xrange function to generate numbers from 0 up to, but not including, 10. The function prints each number in turn, resulting in the output shown.
While xrange and range might seem similar, there’s a crucial difference when it comes to memory usage. When you use range to generate a large sequence of numbers, Python has to create a list containing all those numbers, which can consume a significant amount of memory. On the other hand, xrange generates the numbers one at a time, only when you need them. This makes xrange a better choice when dealing with large sequences.
However, there are potential pitfalls to be aware of when using xrange. For one, because xrange generates numbers on the fly, it can be slower than range when iterating over the sequence multiple times. Additionally, xrange is specific to Python 2, and has been replaced by the range function in Python 3.
Python’s xrange: Advanced Usage
Beyond the basic usage of xrange, you can also customize the sequence it generates by specifying different parameters. The xrange function in Python 2 can take one, two, or three parameters:
xrange(stop)
: Generates numbers from 0 up to, but not including,stop
.xrange(start, stop)
: Generates numbers fromstart
up to, but not including,stop
.xrange(start, stop, step)
: Generates numbers fromstart
up to, but not including,stop
, incrementing bystep
.
Here’s an example illustrating the use of xrange with different parameters:
# using xrange with one parameter
for i in xrange(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
# using xrange with two parameters
for i in xrange(2, 5):
print(i)
# Output:
# 2
# 3
# 4
# using xrange with three parameters
for i in xrange(0, 10, 2):
print(i)
# Output:
# 0
# 2
# 4
# 6
# 8
In the first example, we’re using xrange with one parameter. This generates numbers from 0 up to, but not including, 5. The second example uses xrange with two parameters, generating numbers from 2 up to, but not including, 5. Finally, the third example uses xrange with three parameters to generate even numbers from 0 up to, but not including, 10.
Understanding how to use xrange with different parameters allows you to customize the sequence it generates, giving you more control over your Python 2 code.
Exploring Alternatives to Python’s xrange
While xrange is a useful function for generating sequences in Python 2, there are alternative methods available, particularly in Python 3 and through third-party libraries.
Python 3’s range Function
In Python 3, the xrange function has been replaced by the range function, which now behaves like xrange in Python 2. It generates numbers on the fly, making it more memory-efficient for large sequences. Here’s an example:
# Python 3
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this Python 3 code, the range function generates numbers from 0 up to, but not including, 5, just like xrange in Python 2.
Third-Party Libraries
There are also third-party libraries available that offer additional ways to generate sequences in Python. For example, the NumPy library provides the arange function, which can generate sequences of numbers with decimal increments:
# Using NumPy's arange function
import numpy as np
for i in np.arange(0, 1, 0.1):
print(i)
# Output:
# 0.0
# 0.1
# 0.2
# 0.3
# 0.4
# 0.5
# 0.6
# 0.7
# 0.8
# 0.9
In this example, np.arange generates numbers from 0 up to, but not including, 1, incrementing by 0.1 each time.
Both of these alternatives have their own advantages. Python 3’s range function is built-in and doesn’t require any additional libraries, while NumPy’s arange function offers more flexibility with decimal increments. However, they might not be suitable for all use cases. For instance, if you’re working with Python 2 code, you might not be able to use Python 3’s range function without making additional changes to your code. Similarly, using NumPy’s arange function requires installing the NumPy library, which might not be desirable in all situations.
In summary, while xrange is a powerful tool for generating sequences in Python 2, it’s not the only option available. Depending on your specific needs and circumstances, you might find Python 3’s range function or third-party libraries like NumPy to be more suitable.
Troubleshooting Common Issues with Python’s xrange
Despite its usefulness, you may encounter some common issues when using the xrange function. One of the most common errors is NameError: name 'xrange' is not defined
, which occurs when you try to use xrange in Python 3.
‘NameError: name ‘xrange’ is not defined’ in Python 3
In Python 3, the xrange function has been replaced by the range function, which behaves like xrange in Python 2. So, if you try to use xrange in Python 3, you’ll get a NameError
:
# Python 3
for i in xrange(5):
print(i)
# Output:
# NameError: name 'xrange' is not defined
To fix this error, you can replace xrange with range in your Python 3 code:
# Python 3
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this corrected Python 3 code, the range function generates numbers from 0 up to, but not including, 5, just like xrange in Python 2.
Remember, understanding the differences between Python 2 and Python 3 is crucial for troubleshooting issues like this. If you’re working with Python 2 code that uses xrange, you’ll need to replace xrange with range if you want to run the code in Python 3.
Python Iteration and Sequence Generation
To fully understand the xrange function, it’s essential to grasp Python’s concepts of iteration and sequence generation. In Python, a sequence is a collection of items, and iteration is the process of looping over each item in the sequence.
Python’s Iteration Concept
In Python, you can iterate over sequences using the for
loop. For example, here’s how you can print each item in a list:
# Python 2
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, fruits
is a list (a type of sequence), and the for
loop iterates over each item in the list, printing it to the console.
Python’s Sequence Generation
Python provides several built-in functions for generating sequences of numbers, including range
and xrange
. These functions generate a sequence of numbers within a specified range, which can then be iterated over using a for
loop.
For example, here’s how you can use the range
function to print the numbers 0 through 4:
# Python 2
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, range(5)
generates a sequence of numbers from 0 up to, but not including, 5. The for
loop then iterates over each number in the sequence, printing it to the console.
Understanding these fundamental concepts of iteration and sequence generation is key to understanding the xrange function. As we’ve seen, xrange is a function that generates a sequence of numbers on the fly, making it more memory-efficient for large sequences. This makes it a powerful tool for controlling memory usage in Python 2.
Beyond xrange: Memory-Efficient Programming in Python
The xrange function is just one of many tools Python provides for memory-efficient programming. Understanding xrange and its role in handling large sequences is a stepping stone to mastering more complex concepts in Python, such as list comprehension, generators, and memory management.
List Comprehension in Python
List comprehension is a concise way to create lists in Python. It can make your code more readable and efficient. Here’s an example using xrange:
# Python 2
squares = [i**2 for i in xrange(5)]
print(squares)
# Output:
# [0, 1, 4, 9, 16]
In this example, we’re using list comprehension with xrange to generate a list of square numbers.
Python Generators
Generators are a type of iterable in Python. Like xrange, they generate items on the fly, which makes them more memory-efficient for large sequences. Here’s an example of a generator that behaves like xrange:
# Python 2
def my_xrange(stop):
i = 0
while i < stop:
yield i
i += 1
for number in my_xrange(5):
print(number)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, my_xrange
is a generator that produces numbers from 0 up to, but not including, stop
. The yield
keyword is used to produce items from a generator.
Python Memory Management
Understanding how Python manages memory can help you write more efficient code. For instance, knowing that xrange generates numbers on the fly (and therefore uses less memory for large sequences) can inform your choice between xrange and range.
Further Resources for Mastering Python’s xrange
If you’re interested in learning more about xrange and related concepts in Python, the following resources are excellent starting points:
- Exploring Essential Python Built-In Functions – Dive into Python’s built-in functions to streamline your coding experience.
Dynamic Code Execution in Python with eval() – Learn how to leverage “eval” for custom scripting and program flexibility.
Python hash() Function: Hashing Data in Python – Learn about the Python “hash()” function for generating hash values.
Python 2 Documentation – The official Python 2 documentation is a resource on all built-in functions, including xrange.
Python for Data Analysis – This book by offers in-depth coverage of data analysis techniques in Python.
Python Module of the Week series covers a variety of Python modules and functions, with examples and explanations.
Wrapping Up: Mastering Python’s xrange
In this comprehensive guide, we’ve delved deep into the world of Python’s xrange function, a built-in function in Python 2 that’s designed to generate an object that produces numbers within a specified range.
We began with the basics, learning how to use xrange in its simplest form. We then explored more advanced usage, understanding how to customize the sequence generated by xrange by specifying different parameters. Along the way, we tackled common issues you might encounter when using xrange, such as the ‘NameError: name ‘xrange’ is not defined’ error in Python 3, providing you with solutions for each issue.
We also ventured into alternative approaches to generating sequences in Python, comparing xrange with Python 3’s range function and third-party libraries like NumPy. Here’s a quick comparison of these methods:
Method | Memory Efficiency | Flexibility | Ease of Use |
---|---|---|---|
xrange (Python 2) | High | Moderate | High |
range (Python 3) | High | Moderate | High |
NumPy’s arange | Moderate | High | Moderate |
Whether you’re just starting out with Python 2 or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of xrange and its role in memory-efficient programming.
Understanding xrange and its alternatives is a crucial step in mastering Python, especially when dealing with large sequences. Now, you’re well equipped to handle large ranges in Python 2 with ease. Happy coding!