Incrementing Bash Variables | Shell Scripting Syntax Guide
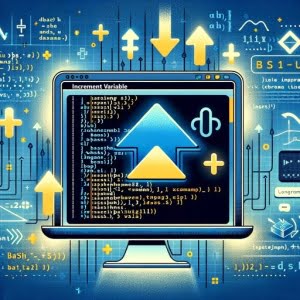
Are you finding it challenging to increment variables in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling variable incrementation in Bash, but we’re here to help.
Think of Bash as a calculator – a tool that allows you to easily increment variables with a simple command. This command is a powerful way to manipulate data in your Bash scripts, making it an essential skill for any Bash user.
In this guide, we’ll walk you through the process of incrementing variables in Bash, from the basics to more advanced techniques. We’ll cover everything from using the ((var++))
syntax, handling different types of incrementation methods, to troubleshooting common issues.
So, let’s dive in and start mastering variable incrementation in Bash!
TL;DR: How Do I Increment a Variable in Bash?
Incrementing a variable in Bash can be achieved using the
((var++))
syntax. This command increases the value of the variable by one.
Here’s a simple example:
var=1
((var++))
echo $var
# Output:
# 2
In this example, we start with a variable var
set to 1. We then use the ((var++))
syntax to increment the value of var
by one. The echo $var
command is used to print the value of var
after incrementation, which outputs ‘2’.
This is a basic way to increment a variable in Bash, but there’s much more to learn about variable manipulation in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the ((var++)) Syntax: A Beginner’s Guide
- Diving Deeper: let Command and Arithmetic Expansion
- Exploring Alternatives: expr and bc Commands
- Troubleshooting Common Errors in Bash Variable Incrementation
- Bash Variables and Arithmetic Operations: The Basics
- Exploring Variable Incrementation in Larger Bash Projects
- Wrapping Up: Mastering Bash Variable Incrementation
Understanding the ((var++))
Syntax: A Beginner’s Guide
The ((var++))
syntax is the most basic and commonly used method to increment a variable in Bash. Let’s break it down:
(( ))
: This denotes an arithmetic expression in Bash. It tells Bash that the content inside the brackets should be evaluated as an arithmetic operation.var++
: This is a post-increment operation. It means that the value ofvar
is used in the expression and then incremented by one.
To illustrate, let’s consider a simple example:
var=5
((var++))
echo $var
# Output:
# 6
In this example, we start with a variable var
set to 5. We then use the ((var++))
syntax to increment the value of var
by one. The echo $var
command is used to print the value of var
after incrementation, which outputs ‘6’.
This method of incrementing a variable is straightforward and easy to use. However, it’s important to note that it only works with integer values. If you try to increment a variable that holds a non-integer value, you’ll encounter an error. Furthermore, the ((var++))
syntax is specific to Bash and may not work in other shells.
Despite these potential pitfalls, the ((var++))
syntax is a powerful tool for incrementing variables in Bash and is a great starting point for beginners learning to manipulate variables in Bash.
Diving Deeper: let
Command and Arithmetic Expansion
As you become more comfortable with Bash, you’ll find that there are more complex ways to increment variables. Two such methods are using the let
command and arithmetic expansion.
The let
Command
The let
command in Bash allows for arithmetic operations to be performed on variables. It’s similar to the ((var++))
syntax, but it offers more flexibility. Here’s an example:
var=5
let "var=var+2"
echo $var
# Output:
# 7
In this example, we’re using the let
command to increment the variable var
by 2. The let
command allows for more complex incrementation, as you can specify the increment value, which is not possible with the ((var++))
syntax.
Arithmetic Expansion
Arithmetic expansion in Bash allows for the evaluation of an arithmetic expression and its replacement with the resulting value. It’s denoted by the $(( ))
syntax. Here’s how you can use it to increment a variable:
var=5
var=$((var+2))
echo $var
# Output:
# 7
In this example, the expression var+2
is evaluated, and the result is assigned back to var
. This method is similar to the let
command but uses a different syntax.
Both the let
command and arithmetic expansion offer more flexibility than the ((var++))
syntax when incrementing variables in Bash. However, they can be slightly more complex to use, especially for beginners. It’s important to practice and understand these methods as they can be incredibly useful in more complex Bash scripts.
Exploring Alternatives: expr
and bc
Commands
As you dive deeper into Bash, you’ll encounter situations that require more advanced methods for incrementing variables. Two such methods involve using the expr
command and the bc
command.
The expr
Command
The expr
command in Bash is used for expression evaluation. It can handle integers and strings, but not floating-point numbers. Here’s an example of how you can use it to increment a variable:
var=5
var=$(expr $var + 2)
echo $var
# Output:
# 7
In this code block, we’re using the expr
command to increment the variable var
by 2. The expression $var + 2
is evaluated, and the result is assigned back to var
. This method is slightly more complex but can be useful in certain situations.
The bc
Command
The bc
command is a language that supports arbitrary precision numbers with interactive execution of statements. It’s especially useful when you need to increment floating-point numbers in Bash. Here’s an example:
var=5.5
var=$(echo "$var + 0.5" | bc)
echo $var
# Output:
# 6.0
In this example, we’re using the bc
command to increment the floating-point number var
by 0.5. The expression $var + 0.5
is piped into bc
, which evaluates it and returns the result. The result is then assigned back to var
.
While these methods are more advanced, they provide additional flexibility when incrementing variables in Bash. However, they also come with their own drawbacks. The expr
command doesn’t support floating-point numbers, and the bc
command requires piping and can be more complex to use. It’s important to consider these factors when deciding which method to use in your Bash scripts.
Troubleshooting Common Errors in Bash Variable Incrementation
As with any programming language, you’ll likely encounter some errors when working with Bash. Here, we’ll discuss some common issues you might face when incrementing variables in Bash and how to solve them.
Non-Integer Incrementation Error
The ((var++))
syntax and let
command can only handle integer values. If you try to increment a variable containing a non-integer value, you’ll encounter an error. Let’s see an example:
var=5.5
((var++))
echo $var
# Output:
# bash: ((: var++: syntax error: operand expected (error token is ".5++")
In this example, we tried to increment a floating-point number using the ((var++))
syntax, which resulted in a syntax error. To solve this, you can use the bc
command, which can handle floating-point numbers:
var=5.5
var=$(echo "$var + 0.5" | bc)
echo $var
# Output:
# 6.0
Uninitialized Variable Error
If you try to increment a variable that hasn’t been initialized, Bash will treat it as a zero. This might not be an error per se, but it can lead to unexpected results. Here’s an example:
((var++))
echo $var
# Output:
# 1
In this example, we didn’t initialize the variable var
before incrementing it. Bash treated var
as a zero, incremented it, and the output was ‘1’. To avoid this, always initialize your variables before using them.
Understanding these common errors and their solutions can help you write more robust Bash scripts and avoid potential pitfalls when incrementing variables.
Bash Variables and Arithmetic Operations: The Basics
To fully grasp the concept of incrementing variables in Bash, it’s important to understand what variables are and how arithmetic operations work in Bash.
Understanding Variables in Bash
A variable in Bash is a symbol or name that stands for a value. Variables are used to store data, like text strings, numbers, or file data, which can then be used in scripts and commands. Here’s an example of how to declare and use a variable in Bash:
name='Alice'
echo "Hello, $name"
# Output:
# Hello, Alice
In this example, we declare a variable name
and assign it the value ‘Alice’. We then use the echo
command to print a greeting, using the variable name
to represent ‘Alice’.
Arithmetic Operations in Bash
Arithmetic operations in Bash are operations that allow you to perform mathematical calculations. This includes operations like addition, subtraction, multiplication, and division. Bash supports integer arithmetic, and with the help of external commands like bc
, it can also handle floating-point arithmetic.
Here’s an example of an arithmetic operation in Bash:
var1=5
var2=2
result=$((var1 + var2))
echo $result
# Output:
# 7
In this example, we declare two variables var1
and var2
and assign them the values 5 and 2, respectively. We then use arithmetic expansion $(( ))
to add the values of var1
and var2
together. The result is stored in the variable result
, which is then printed, outputting ‘7’.
Understanding variables and arithmetic operations in Bash is fundamental to mastering the process of incrementing variables. With this knowledge, you can create more complex scripts and better understand how data manipulation works in Bash.
Exploring Variable Incrementation in Larger Bash Projects
Incrementing variables in Bash isn’t just about adding one to a number. It’s a crucial tool that you’ll often use when writing larger Bash scripts or projects. Understanding how to increment variables can help you manipulate data, control flow, and write more efficient code.
Loop Control Structures
One of the most common use cases for variable incrementation is within loop control structures. For instance, you might have a for
loop where you need to increment a counter each time the loop runs. Here’s an example:
for ((i=0; i<5; i++)); do
echo $i
done
# Output:
# 0
# 1
# 2
# 3
# 4
In this script, we initialize a variable i
to 0 and then increment i
by one in each iteration of the loop. The loop continues until i
is no longer less than 5.
Related Commands and Concepts
There are several related commands and concepts that often accompany variable incrementation in Bash. For example, you might use the read
command to get input from a user, store it in a variable, and then increment it. Or, you might use the if
command to check the value of a variable, and then increment it based on certain conditions.
Understanding how to increment variables in Bash is a fundamental skill for any Bash user. It’s a tool that you’ll use often, and mastering it can help you write more efficient and effective scripts.
Further Resources for Bash Mastery
If you’re interested in learning more about Bash and how to use it effectively, here are some resources that might help:
- GNU Bash Manual: This is the official manual for Bash. It’s comprehensive and detailed, making it a great resource for any Bash user.
Bash Increment Decrement Variable: This guide by Linuxize offers a comprehensive guide on how to increment and decrement variables in Bash.
Bash Scripting Guide: This guide offers a detailed introduction to Bash scripting, covering everything from basic to advanced concepts.
Wrapping Up: Mastering Bash Variable Incrementation
In this comprehensive guide, we’ve journeyed through the world of Bash, focusing on the essential skill of incrementing variables. We’ve explored various methods, from the basic ((var++))
syntax to more advanced techniques involving commands like let
, expr
, and bc
.
We started with the basics, learning how to increment a variable using the ((var++))
syntax. We then delved into more advanced territory, exploring the let
command and arithmetic expansion. We also discussed alternative methods for incrementing variables, such as using the expr
and bc
commands.
Along the way, we tackled common challenges you might face when incrementing variables in Bash, providing you with solutions and workarounds for each issue. We also discussed the fundamentals of Bash variables and arithmetic operations, providing a solid foundation for understanding variable incrementation.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
((var++)) syntax | Simple, easy to use | Only works with integer values, specific to Bash |
let command | Allows for more complex incrementation | Slightly more complex to use |
Arithmetic expansion | Allows for more complex incrementation, different syntax | Slightly more complex to use |
expr command | Can handle integers and strings | Doesn’t support floating-point numbers |
bc command | Can handle floating-point numbers | Requires piping, more complex to use |
Whether you’re just starting out with Bash or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to increment variables in Bash. With these skills in your toolkit, you’re well-equipped to write more efficient and effective Bash scripts. Happy coding!