Java Collections: From Basics to Advanced Usage
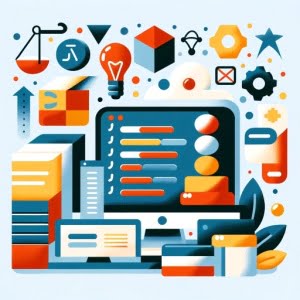
Are you finding it challenging to manage groups of objects in Java? You’re not alone. Many developers find themselves in a maze when it comes to handling groups of objects in Java, but there’s a solution at hand.
Think of Java Collections as a well-organized library. This framework provides a set of classes and interfaces that can help you manage and manipulate your data efficiently, just like a librarian managing books.
This guide will walk you through the ins and outs of the Java Collections Framework, from basic usage to advanced techniques. We’ll cover everything from the basics of using different types of collections like List, Set, and Map, to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Java Collections!
TL;DR: What is Java Collections?
Java Collections are frameworks that provide architectures for storing and manipulating groups of data, such as,
List, ArrayList, Queue, Set, Map,
and more. For instance, you can use the ArrayList class to create a dynamic array. Here’s a simple example:
ArrayList<String> list = new ArrayList<String>();
In this example, we’ve created an instance of the ArrayList class. This instance, list
, is a dynamic array that can hold strings. It’s dynamic because it can grow or shrink at runtime, unlike standard arrays.
This is just a basic way to use Java Collections, but there’s much more to learn about managing and manipulating groups of data in Java. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Understanding Basic Java Collections
- Advanced Techniques with Java Collections
- Exploring Alternative Approaches to Java Collections
- Troubleshooting Java Collections: Common Issues and Solutions
- Decoding the Fundamentals of Java Collections
- The Relevance of Java Collections in Large-Scale Projects
- Wrapping Up
Understanding Basic Java Collections
Java Collections include several types, each with its unique characteristics and use cases. Let’s dive into the basics of List, Set, and Map.
Exploring List in Java
A List in Java is an ordered collection (also known as a sequence). Lists can contain duplicate elements. Here’s an example of how to use a List in Java:
List<String> list = new ArrayList<String>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
System.out.println(list);
// Output:
// [Apple, Banana, Cherry]
In the code above, we’ve created a List and added three elements to it. When we print the list, it maintains the order in which we added the elements.
Delving into Set in Java
A Set in Java is a collection that cannot contain duplicate elements. It models the mathematical set abstraction. Here’s a simple Set example:
Set<String> set = new HashSet<String>();
set.add("Apple");
set.add("Banana");
set.add("Apple");
System.out.println(set);
// Output:
// [Apple, Banana]
In this example, even though we tried to add the string “Apple” twice, the resulting Set only contains one instance of “Apple”. This is because a Set cannot contain duplicate elements.
Understanding Map in Java
A Map in Java is an object that maps keys to values. No two keys can be the same, ensuring that every key maps to a single value. Here’s how you can use a Map in Java:
Map<String, Integer> map = new HashMap<String, Integer>();
map.put("Alice", 25);
map.put("Bob", 30);
map.put("Charlie", 35);
System.out.println(map);
// Output:
// {Alice=25, Charlie=35, Bob=30}
In the code above, we’ve created a Map that maps names to ages. When we print the map, it displays the key-value pairs.
Each of these collection types has its advantages and potential pitfalls. For instance, a List preserves order but can contain duplicates, while a Set can’t contain duplicates but doesn’t preserve order. A Map, on the other hand, associates keys to values but doesn’t maintain order. It’s essential to understand these characteristics to choose the right type of collection for your specific needs.
Advanced Techniques with Java Collections
Now that you’re familiar with the basics of Java Collections, let’s explore some more complex uses, such as sorting and searching with the Collections class.
Sorting Collections in Java
Java Collections Framework provides a Collections
class that includes various methods for manipulating collections. One of these methods is sort()
, which sorts the elements of a List into ascending order. Here’s an example:
List<Integer> list = new ArrayList<Integer>();
list.add(3);
list.add(1);
list.add(2);
Collections.sort(list);
System.out.println(list);
// Output:
// [1, 2, 3]
In the code above, we’ve created a List of integers and added three elements to it in a random order. When we use Collections.sort(list)
, it sorts the elements into ascending order.
Searching in Collections
The Collections
class also provides methods for searching elements in collections, such as binarySearch()
. This method uses the binary search algorithm to find the specified element in a List. However, the List must be sorted for binarySearch()
to work correctly. Here’s how you can use it:
List<Integer> list = new ArrayList<Integer>();
list.add(1);
list.add(2);
list.add(3);
int index = Collections.binarySearch(list, 2);
System.out.println(index);
// Output:
// 1
In this example, we’ve created a sorted List of integers and used Collections.binarySearch(list, 2)
to find the index of the number 2. The output is 1 because the index in a List starts at 0.
These are just a few examples of the more complex uses of Java Collections. By understanding these techniques, you can effectively manage and manipulate your data in Java.
Exploring Alternative Approaches to Java Collections
While Java Collections are powerful, they are not the only way to manage groups of objects in Java. Let’s dive into some alternative methods, such as using arrays or third-party libraries.
Harnessing the Power of Arrays
Arrays are the simplest form of data structure in Java and can be used to store multiple values of the same type. Here’s an example of how to use an array in Java:
int[] array = new int[3];
array[0] = 1;
array[1] = 2;
array[2] = 3;
System.out.println(Arrays.toString(array));
// Output:
// [1, 2, 3]
In this example, we’ve created an array and added three elements to it. When we print the array using Arrays.toString(array)
, it displays the elements in the order we added them.
Arrays can be a straightforward and efficient way to manage groups of objects. However, they have a fixed size once created, which can limit their flexibility compared to collections.
Utilizing Third-Party Libraries
Third-party libraries, such as Google’s Guava, provide additional collection types and utilities that can enhance your data manipulation capabilities. For instance, Guava’s Multiset
is a type of collection that can hold multiple instances of each element, and it counts the number of times an element is added.
Here’s an example of how to use a Multiset
:
Multiset<String> multiset = HashMultiset.create();
multiset.add("Apple");
multiset.add("Apple");
multiset.add("Banana");
System.out.println(multiset.count("Apple"));
// Output:
// 2
In this example, we’ve created a Multiset
and added “Apple” twice and “Banana” once. When we use multiset.count("Apple")
, it returns 2 because “Apple” was added twice.
Third-party libraries can offer powerful and flexible tools for managing groups of objects. However, they also add external dependencies to your project, which can increase its complexity and potential for issues.
Choosing the right method to manage groups of objects in Java depends on your specific needs and constraints. While Java Collections are versatile and built into Java, arrays and third-party libraries can provide alternative approaches that may be more suitable in certain scenarios.
Troubleshooting Java Collections: Common Issues and Solutions
While Java Collections are powerful tools, developers can sometimes encounter issues that can be tricky to debug. Let’s discuss a common issue and how to solve it.
Understanding ConcurrentModificationException
A ConcurrentModificationException
is thrown when a collection is modified while it’s being iterated. This is a common issue when working with Java Collections. Here’s an example of how this might happen:
List<String> list = new ArrayList<String>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
for (String fruit : list) {
if (fruit.equals("Banana")) {
list.remove(fruit);
}
}
// Output:
// Exception in thread "main" java.util.ConcurrentModificationException
In this example, we’re trying to remove an element from the list while iterating over it, which causes a ConcurrentModificationException
.
Solving ConcurrentModificationException
One way to avoid this issue is to use an Iterator
to modify the collection while iterating. Here’s how you can do it:
List<String> list = new ArrayList<String>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String fruit = iterator.next();
if (fruit.equals("Banana")) {
iterator.remove();
}
}
System.out.println(list);
// Output:
// [Apple, Cherry]
In this revised example, we’re using an Iterator
to iterate over the list. When we find the element we want to remove, we call iterator.remove()
. This avoids the ConcurrentModificationException
and successfully removes “Banana” from the list.
Understanding common issues like ConcurrentModificationException
and their solutions can help you use Java Collections more effectively and avoid potential pitfalls.
Decoding the Fundamentals of Java Collections
To fully grasp the power of Java Collections, it’s essential to understand the underlying concepts, such as interfaces, generics, and iterators.
Understanding Interfaces in Java Collections
The Java Collections Framework is designed around several core interfaces, namely Collection, List, Set, Queue, and Map. These interfaces form the foundation of the framework and define the methods that collections can use.
For instance, the List interface provides methods for accessing elements based on their position in the list, while the Set interface provides methods for adding elements, checking if an element is in the set, and more.
List<String> list = new ArrayList<String>();
list.add("Apple");
String firstElement = list.get(0);
System.out.println(firstElement);
// Output:
// Apple
In the example above, we’re using the get(int index)
method from the List interface to retrieve the first element of the list.
Grasping Generics in Java Collections
Generics add stability to your code by checking the type of objects you’re using at compile time. This means you can catch potential class cast exceptions before your code even runs.
List<String> list = new ArrayList<String>();
list.add("Apple");
String firstElement = list.get(0);
System.out.println(firstElement);
// Output:
// Apple
In this example, we’re using generics to specify that our List will only hold Strings. This way, we can be sure that when we retrieve an element from the list, it will be a String.
Diving into Iterators
Iterators are an integral part of the Java Collections Framework. They provide a way to cycle through a collection, allowing you to access each element in turn.
List<String> list = new ArrayList<String>();
list.add("Apple");
list.add("Banana");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String fruit = iterator.next();
System.out.println(fruit);
}
// Output:
// Apple
// Banana
In this example, we’re using an Iterator to loop over a List and print each element. The hasNext()
method checks if there’s another element in the collection, and the next()
method retrieves the next element.
By understanding these fundamental concepts, you can harness the power of Java Collections to efficiently manage and manipulate your data.
The Relevance of Java Collections in Large-Scale Projects
Java Collections are not just useful for small programs or scripts, but are also vital in larger projects and real-world applications. They provide the tools necessary to manage data efficiently, which is critical in applications dealing with large amounts of data or complex data structures.
Java Streams: A Related Topic
Java Streams, introduced in Java 8, are a powerful complement to Java Collections. They provide a simple, declarative approach to data manipulation, allowing you to perform complex data processing tasks in a readable and concise way.
List<String> list = Arrays.asList("Apple", "Banana", "Cherry");
list.stream()
.filter(fruit -> fruit.startsWith("A"))
.forEach(System.out::println);
// Output:
// Apple
In this example, we’re using a Java Stream to filter and print elements from a List. The filter()
method removes elements that don’t match the given predicate, and forEach()
performs an action for each remaining element.
Multithreading: Another Related Topic
Multithreading is another topic that often goes hand-in-hand with Java Collections. When dealing with large amounts of data, you may need to process data in parallel to improve performance. Java provides built-in support for multithreading, which can be combined with collections for efficient data processing.
List<String> list = Arrays.asList("Apple", "Banana", "Cherry");
list.parallelStream()
.forEach(fruit -> System.out.println(fruit + " " + Thread.currentThread().getName()));
// Output (may vary):
// Apple main
// Banana ForkJoinPool.commonPool-worker-1
// Cherry ForkJoinPool.commonPool-worker-2
In this example, we’re using a parallel stream, a feature introduced in Java 8, to process a List in parallel. The output shows that each element is processed by a different thread.
Further Resources for Mastering Java Collections
To deepen your understanding of Java Collections, consider exploring these resources:
- Understanding Java: Its Purpose and Applications – Learn the practical applications of Java programming.
Java Syntax Explained – Master writing clear and concise code using proper Java syntax.
Understanding Java Classes – Learn how to define classes, fields, constructors, and methods in Java.
Oracle’s Java Collections Tutorial provides an in-depth tutorial on Java Collections.
Baeldung’s Guide to Java Collections offers a variety of guides and articles on Java Collections.
Java Code Geeks’ Java Collections Examplesis a series of examples and tutorials on Java Collections.
Wrapping Up
In this comprehensive guide, we’ve delved deep into the world of Java Collections, a powerful framework for managing and manipulating groups of objects in Java.
We began with the basics, learning how to use different types of collections such as List, Set, and Map. We then ventured into more advanced territory, exploring complex uses of Java Collections, such as sorting and searching with the Collections class.
Along the way, we tackled common challenges you might face when using Java Collections, such as ConcurrentModificationException, providing you with solutions for each issue. We also looked at alternative approaches to managing groups of objects, comparing Java Collections with arrays and third-party libraries. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | External Dependencies |
---|---|---|---|
Java Collections | High | Moderate | None |
Arrays | Low | Low | None |
Third-Party Libraries | High | High | Yes |
Whether you’re just starting out with Java Collections or you’re looking to level up your data manipulation skills, we hope this guide has given you a deeper understanding of Java Collections and its capabilities.
With its balance of flexibility, efficiency, and built-in support, Java Collections is a powerful tool for managing groups of objects in Java. Now, you’re well equipped to handle any data manipulation tasks that come your way. Happy coding!