Rehashing in Java: Guide to Load Factor Manipulation
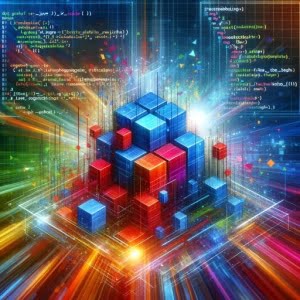
Ever wondered how Java’s HashMap class manages to store and retrieve data so efficiently? The secret lies in a process called rehashing. Like a city expanding its roads to accommodate more traffic, HashMap expands its capacity to store more entries.
Rehashing in Java is not just a technical process, but a vital strategy that ensures your data is always accessible and manageable, even as your application scales. It’s a key aspect of Java that every developer should understand.
This guide will walk you through the concept of rehashing in Java, from the basics to advanced usage. We’ll explore how rehashing works, delve into its practical applications, and even discuss common issues and their solutions. So, let’s dive in and start mastering rehashing in Java!
TL;DR: What is Rehashing in Java?
Rehashing
in Java is a process where theHashMap class
creates a new array of greater capacity when the number of entries in the map reaches a certain threshold. This is done to maintain the efficiency of data storage and retrieval. For instance, if you have a HashMapmyMap
and you add an entry to it like so:myMap.put(key, value)
, rehashing may occur if the number of entries exceeds a certain limit.
Here’s a simple example:
import java.util.HashMap;
HashMap<String, Integer> myMap = new HashMap<>();
for (int i = 0; i < 100; i++) {
myMap.put("Key" + i, i);
}
System.out.println(myMap.size());
// Output:
// 100
In this example, we create a HashMap myMap
and add 100 entries to it. The put()
method is used to add entries to the map. If the number of entries exceeds the threshold, rehashing will occur to expand the map’s capacity.
This is a basic explanation of rehashing in Java, but there’s much more to learn about how it works, when and why it occurs, and how to optimize it. Continue reading for a more detailed explanation and examples.
Table of Contents
- Unraveling Rehashing: The Beginner’s Guide
- Customizing Load Factor: Control Your Rehashing
- Exploring Alternatives: LinkedHashMap and Hashtable
- Navigating Rehashing Pitfalls: Performance and Memory
- The Theory Behind Rehashing: Hash Functions Unveiled
- Beyond Rehashing: Exploring Data Structures in Java
- Wrapping Up: Rehashing in Java
Unraveling Rehashing: The Beginner’s Guide
Before we delve into rehashing, let’s first understand what a HashMap is. A HashMap in Java is a part of the Java collections framework, which stores key-value pairs. It uses a technique called ‘Hashing’ to store and retrieve elements efficiently.
Now, what happens when our HashMap starts to fill up? This is where rehashing comes into play. Rehashing in Java is a process where the HashMap class creates a new array of greater capacity when the number of entries in the map reaches a certain threshold. This is done to maintain the efficiency of data storage and retrieval.
Let’s look at a simple example of using a HashMap and see when and why rehashing occurs:
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<Integer, String> map = new HashMap<Integer, String>();
// Adding elements to the map
for (int i = 1; i <= 12; i++) {
map.put(i, "Element" + i);
}
// Displaying the size of the map
System.out.println("Size of map is:- " + map.size());
}
}
// Output:
// Size of map is:- 12
In this example, we first create a HashMap map
and then add 12 elements to it using a for loop. The put()
method is used to add entries to the map. After adding the entries, we display the size of the map, which is 12.
In the background, however, something else is happening. When the number of entries in the HashMap exceeds a certain threshold (the default load factor is 0.75), rehashing occurs to create a new array with greater capacity. This ensures that the HashMap continues to store and retrieve data efficiently, even as more entries are added.
Customizing Load Factor: Control Your Rehashing
As we’ve learned, rehashing in Java occurs when the number of entries in the HashMap reaches a certain threshold. This threshold is a product of the HashMap’s current capacity and a value known as the ‘load factor’. The default load factor in Java’s HashMap is 0.75, but did you know you can customize this?
By manipulating the load factor, we can control when rehashing occurs. A lower load factor increases the threshold, which reduces the chance of rehashing but increases the space complexity. Conversely, a higher load factor decreases the threshold, increasing the chance of rehashing but saving memory.
Let’s see how we can customize the load factor in a HashMap:
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
float loadFactor = 0.5f;
HashMap<Integer, String> map = new HashMap<Integer, String>(16, loadFactor);
// Adding elements to the map
for (int i = 1; i <= 12; i++) {
map.put(i, "Element" + i);
}
// Displaying the size of the map
System.out.println("Size of map is:- " + map.size());
}
}
// Output:
// Size of map is:- 12
In this example, we’ve set the load factor to 0.5, which is lower than the default 0.75. This means rehashing will occur earlier (when the map is 50% full rather than 75% full), leading to more memory usage but potentially more efficient data retrieval.
Understanding and tweaking the load factor can help you balance between memory usage and performance in your Java applications, making your use of HashMaps and rehashing more effective.
Exploring Alternatives: LinkedHashMap and Hashtable
While HashMap is the most commonly used class for implementing maps in Java, there are other classes that also use rehashing, such as LinkedHashMap and Hashtable. These classes offer alternative ways to manage your data and can be more suitable depending on your specific needs.
LinkedHashMap
LinkedHashMap is a subclass of HashMap. It maintains a doubly-linked list running through all of its entries. This linked list defines the iteration ordering, which is normally the order in which keys were inserted into the map (insertion-order).
import java.util.LinkedHashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
LinkedHashMap<Integer, String> map = new LinkedHashMap<Integer, String>();
// Adding elements to the map
for (int i = 1; i <= 5; i++) {
map.put(i, "Element" + i);
}
// Displaying the elements of the map
for (Map.Entry<Integer, String> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
// Output:
// 1: Element1
// 2: Element2
// 3: Element3
// 4: Element4
// 5: Element5
In this example, we create a LinkedHashMap and add five elements to it. When we print the elements, they are displayed in the order they were inserted, which is a characteristic of LinkedHashMap.
Hashtable
Hashtable, on the other hand, is synchronized, unlike HashMap and LinkedHashMap. This means Hashtable is thread-safe and can be shared between multiple threads.
import java.util.Hashtable;
public class Main {
public static void main(String[] args) {
Hashtable<Integer, String> table = new Hashtable<Integer, String>();
// Adding elements to the table
for (int i = 1; i <= 5; i++) {
table.put(i, "Element" + i);
}
// Displaying the size of the table
System.out.println("Size of table is:- " + table.size());
}
}
// Output:
// Size of table is:- 5
In this example, we create a Hashtable and add five elements to it. The size of the Hashtable is then printed, showing that it contains the five elements we added.
Choosing between HashMap, LinkedHashMap, and Hashtable depends on your specific use case. LinkedHashMap is useful when you need to maintain the insertion order, while Hashtable is a good choice when you need to share the map between multiple threads.
Rehashing, while essential for efficient data storage and retrieval, does come with its own set of challenges. Two common issues related to rehashing are performance implications and memory usage.
Performance Implications
While rehashing can improve the efficiency of data retrieval, it can also impact performance. The process of rehashing involves creating a new, larger array and reinserting all the existing entries into this new array. This can be a time-consuming operation, especially for large maps.
Memory Usage
Another consideration is memory usage. As the load factor decreases and the frequency of rehashing increases, the memory usage of the HashMap also increases. This is because the HashMap maintains a larger array to store the entries, even if many of the array positions are unused.
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
float loadFactor = 0.1f; // Decreasing load factor
HashMap<Integer, String> map = new HashMap<Integer, String>(16, loadFactor);
// Adding elements to the map
for (int i = 1; i <= 12; i++) {
map.put(i, "Element" + i);
}
// Displaying the size of the map
System.out.println("Size of map is:- " + map.size());
}
}
// Output:
// Size of map is:- 12
In this example, we’ve set the load factor to 0.1, which is much lower than the default 0.75. This means rehashing will occur more frequently, leading to higher memory usage.
Optimizing HashMap Usage
To optimize the use of HashMap and other classes that use rehashing, you should consider the following tips:
- Choose an appropriate load factor. A higher load factor reduces memory usage but increases the chance of rehashing. A lower load factor does the opposite.
If the number of entries in the map is known in advance, specify an initial capacity that is large enough to hold the entries without rehashing.
If the map size grows or shrinks significantly over time, consider using the
trimToSize()
method of the HashMap class to minimize the memory footprint.
Understanding these considerations can help you use rehashing more effectively in your Java applications.
The Theory Behind Rehashing: Hash Functions Unveiled
To fully grasp the concept of rehashing in Java, it’s crucial to understand the theory behind it, particularly the concept of a hash function.
A hash function is a special function used in the hashing technique that maps data of arbitrary size to fixed-size values. In the context of a HashMap, the keys are hashed using a hash function, and the resulting hash code is used to determine the index where the corresponding value should be stored in the array.
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
// Generate hash code for a key
int hashCode = "Two".hashCode();
System.out.println("Hash code for 'Two': " + hashCode);
}
}
// Output:
// Hash code for 'Two': 842345
In this example, we create a HashMap and add three entries to it. We then generate the hash code for the key ‘Two’ using the hashCode()
method. This hash code would be used by the HashMap to determine the index where the value 2 should be stored.
Rehashing comes into play when the number of entries in the HashMap reaches a certain threshold, which is a product of the HashMap’s capacity and its load factor. When this happens, a new array with a greater capacity is created, and all the existing entries are rehashed and put into the new array. This process ensures that the HashMap can continue to store and retrieve data efficiently as more entries are added.
Understanding the concept of a hash function and its role in rehashing is key to mastering the use of HashMaps and similar data structures in Java.
Beyond Rehashing: Exploring Data Structures in Java
Rehashing, as we’ve learned, is a crucial aspect of data management in Java. However, it’s just one part of a larger picture. Java offers a rich collection of data structures, each with its unique characteristics and uses. From ArrayLists and LinkedLists to Stacks and Queues, each data structure in Java has its own way of managing data.
While HashMaps use rehashing to ensure efficient data storage and retrieval, other data structures might use different techniques. For example, an ArrayList dynamically resizes itself by creating a new array and copying the old elements to the new array, similar to rehashing. LinkedLists, on the other hand, manage data by linking nodes together in a chain.
Understanding these different data structures and how they manage data is key to becoming a proficient Java developer. It allows you to choose the right tool for the job, optimizing your code for efficiency, performance, and readability.
Further Resources for Mastering Java Data Structures
If you’re interested in diving deeper into the world of Java data structures, here are some resources to get you started:
- IOFlood’s Article on Java HashMap – Learn about HashMap iteration and traversal techniques in Java programming.
Understanding Java HashSet – Master using HashSet to store and manipulate unique elements in Java collections.
Using HashCode in Java – Learn about implementing custom hashCode() methods for hashing in user-defined classes.
Coursera’s Java Data Structures and Algorithms offers a comprehensive look at data structures and algorithms in Java.
Java Collections Framework Tutorial covers the Java Collections Framework and many of the data structures used in Java.
Udemy’s Data Structures and Algorithms in Java course covers data structures, as well as algorithms, in Java.
These resources offer a wealth of information on Java data structures, helping you deepen your understanding and improve your coding skills.
Wrapping Up: Rehashing in Java
We’ve embarked on a comprehensive journey exploring the concept of rehashing in Java, the secret behind the efficient data storage and retrieval in Java’s HashMap class.
We began with the basics, understanding what rehashing is and how it works in Java. We then delved into a simple example of using a HashMap and saw when and why rehashing occurs. From there, we ventured into more advanced territory, learning how to customize the load factor in a HashMap to control when rehashing occurs and how it impacts performance and memory usage.
Along the way, we explored alternative classes in Java that use rehashing, such as LinkedHashMap and Hashtable, and compared their performance and use cases. We also tackled common issues related to rehashing and provided tips on how to optimize the use of HashMap and other classes that use rehashing.
Here’s a quick comparison of the Java classes we’ve discussed:
Class | Use Case | Thread-Safe | Order Preservation |
---|---|---|---|
HashMap | General purpose | No | No |
LinkedHashMap | When insertion order matters | No | Yes |
Hashtable | When thread safety is required | Yes | No |
Whether you’re just starting out with rehashing in Java or you’re looking to deepen your understanding, we hope this guide has equipped you with the knowledge to use and optimize rehashing effectively in your Java applications.
Understanding rehashing is key to mastering data management in Java. With this knowledge, you’re well on your way to becoming a more proficient Java developer. Happy coding!