Java HashMap Explained: Your Guide to Effective Coding
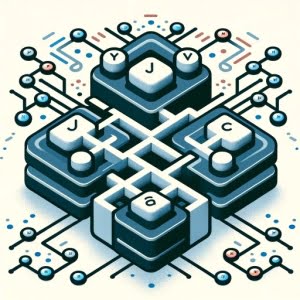
Are you finding it challenging to work with Java’s HashMap? You’re not alone. Many developers find themselves puzzled when it comes to using HashMap in Java, but we’re here to help.
Think of Java’s HashMap as a well-organized library – it allows you to store and retrieve data efficiently, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using HashMap in Java, from basic usage to advanced techniques. We’ll cover everything from creating a HashMap, adding and retrieving elements, to more advanced methods such as iteration, sorting, and handling null values, as well as alternative approaches.
Let’s dive in and start mastering Java’s HashMap!
TL;DR: What is Java HashMap and How Do I Use It?
Java HashMap is a part of the Java Collections Framework that allows you to store key-value pairs, created with the syntax,
HashMap<String, Integer> map = new HashMap<>();
. It’s a powerful tool that can significantly streamline your data management process in Java.
Here’s a simple example of how to use it:
HashMap<String, Integer> map = new HashMap<>();
map.put('apple', 1);
int value = map.get('apple');
// Output:
// 1
In this example, we create a new HashMap named ‘map’. We then use the ‘put’ method to store the integer 1 with the key ‘apple’. Finally, we retrieve the value associated with the key ‘apple’ using the ‘get’ method, which in this case, returns 1.
This is just a basic way to use HashMap in Java, but there’s much more to learn about this versatile tool. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Getting Started with Java HashMap
- Advanced Methods of Java HashMap
- Exploring Alternative Map Implementations in Java
- Troubleshooting Common Issues with Java HashMap
- Understanding Hashing and the Map Interface
- Expanding the Use of HashMap in Larger Projects
- Exploring Related Topics
- Wrapping Up: Java HashMap
Getting Started with Java HashMap
HashMap in Java allows you to store data as ‘key-value’ pairs, similar to a real-life map where you can find a location (value) using an address (key).
Creating a HashMap
The first step is to create a HashMap. Here’s how you do it:
HashMap<String, Integer> map = new HashMap<>();
This line of code creates a new HashMap named ‘map’ that can store keys of type String and values of type Integer.
Adding Elements
Next, let’s add some elements to our HashMap:
map.put('apple', 1);
map.put('banana', 2);
map.put('cherry', 3);
In this example, we are adding three elements to our HashMap. The ‘put’ method is used to add new key-value pairs to the HashMap.
Retrieving Values
To retrieve a value from the HashMap, you use the ‘get’ method and provide the key:
int value = map.get('apple');
// Output:
// 1
This line retrieves the value associated with the key ‘apple’, which is 1.
Removing Elements
To remove an element from the HashMap, you use the ‘remove’ method:
map.remove('apple');
This line of code removes the key-value pair with the key ‘apple’ from the HashMap.
And there you have it! This is the basic usage of HashMap in Java. But there’s still more to learn, so let’s move on to some more advanced topics.
Advanced Methods of Java HashMap
As you become more comfortable with the basic operations of Java’s HashMap, you can start exploring some of its more advanced features. Let’s delve into iteration, sorting, and handling null values.
Iterating Over a HashMap
To go through each key-value pair in the HashMap, you can use a for-each loop along with the entrySet
method:
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println('Key: ' + entry.getKey() + ', Value: ' + entry.getValue());
}
// Output:
// Key: banana, Value: 2
// Key: cherry, Value: 3
In this example, we’re iterating over the HashMap and printing out each key-value pair. The entrySet
method returns a set of all the key-value pairs contained in the HashMap.
Sorting a HashMap
Sorting a HashMap can be done based on either keys or values. Here’s how you can sort a HashMap by keys:
Map<String, Integer> sortedMap = new TreeMap<>(map);
In this line, we’re creating a new TreeMap from our HashMap. A TreeMap in Java is automatically sorted based on keys.
Handling Null Values
A HashMap allows one null key and multiple null values. Here’s how you can add a null key and a null value:
map.put(null, 4);
map.put('durian', null);
In the first line, we’re adding a key-value pair with a null key. In the second line, we’re adding a key-value pair with a null value. When retrieving data, the HashMap will return null
if the key doesn’t exist or if the value is null
.
These are just a few of the many advanced methods of Java’s HashMap. As you delve deeper, you’ll find that HashMap is a powerful tool that can greatly enhance your data handling capabilities in Java.
Exploring Alternative Map Implementations in Java
While HashMap is a powerful tool, Java offers other Map implementations, such as TreeMap and LinkedHashMap, which can be more suitable for specific scenarios.
TreeMap: Sorted Key-Value Pairs
TreeMap is a Map implementation that keeps its entries sorted according to the natural ordering of its keys or a custom comparator provided at map creation time. Here’s how you create a TreeMap:
Map<String, Integer> treeMap = new TreeMap<>();
And here’s how you can add elements and print a TreeMap:
treeMap.put('banana', 2);
treeMap.put('apple', 1);
treeMap.put('cherry', 3);
System.out.println(treeMap);
// Output:
// {apple=1, banana=2, cherry=3}
As you can see, the output is sorted by keys. TreeMap can be useful when you need to maintain an order in your key-value pairs.
LinkedHashMap: Maintaining Insertion Order
LinkedHashMap is another Map implementation that maintains the insertion order of its entries. Here’s how you create a LinkedHashMap:
Map<String, Integer> linkedHashMap = new LinkedHashMap<>();
And here’s how you can add elements and print a LinkedHashMap:
linkedHashMap.put('banana', 2);
linkedHashMap.put('apple', 1);
linkedHashMap.put('cherry', 3);
System.out.println(linkedHashMap);
// Output:
// {banana=2, apple=1, cherry=3}
As you can see, the output maintains the insertion order. LinkedHashMap is useful when you need to maintain the order of elements as they were put into the Map.
Remember, while HashMap is a great general-purpose Map implementation, TreeMap and LinkedHashMap offer unique features that can be more suitable in certain scenarios. Understanding when to use each can significantly improve your Java programming skills.
Troubleshooting Common Issues with Java HashMap
While working with HashMap in Java, you might encounter some common issues. Let’s discuss these problems, their solutions, and some best practices.
Handling Collisions
A collision happens when two keys have the same hashcode. HashMap handles collisions using a linked list to store collided elements and maintains a separate list for each bucket.
HashMap<String, Integer> map = new HashMap<>();
map.put('apple', 1);
map.put('bPple', 2);
// Output:
// The keys 'apple' and 'bPple' have the same hashcode, causing a collision.
In this example, both ‘apple’ and ‘bPple’ have the same hashcode, causing a collision. But HashMap handles this gracefully by adding ‘bPple’ to the linked list of ‘apple’.
Understanding Initial Capacity and Load Factor
The initial capacity is the number of buckets in the hash table at the time of its creation, and the load factor is a measure of how full the hash table is allowed to get before its capacity is automatically increased.
HashMap<String, Integer> map = new HashMap<>(16, 0.75f);
In this example, we’re creating a HashMap with an initial capacity of 16 and a load factor of 0.75. This means the HashMap will be resized when it’s 75% full.
It’s important to set an appropriate initial capacity and load factor to prevent unnecessary resizing operations, which can impact performance.
Remember, while HashMap is a versatile tool, it’s not without its challenges. Understanding these common issues and how to handle them can help you use HashMap more effectively in your Java programming.
Understanding Hashing and the Map Interface
To fully grasp how HashMap works, it’s essential to understand the concept of hashing and the Map interface in Java.
The Concept of Hashing
Hashing is a technique that converts a large String to a small String that represents the same String. A ‘hash code’ is generated by a ‘hash function’. Here’s a simple example:
String name = 'apple';
int hashCode = name.hashCode();
System.out.println(hashCode);
// Output:
// 99162322
In this example, we’re generating the hash code of the string ‘apple’. As you can see, the hash code is an integer that represents the string.
HashMap in Java uses hashing to store and retrieve elements. When storing an element, HashMap uses the hash code of the key to determine where to store the element. When retrieving the element, it uses the hash code again to find it.
The Map Interface
HashMap is an implementation of the Map interface in Java. The Map interface provides methods for storing key-value pairs and retrieving values based on keys.
Map<String, Integer> map = new HashMap<>();
map.put('apple', 1);
int value = map.get('apple');
// Output:
// 1
In this example, we’re using the ‘put’ method of the Map interface to store a key-value pair and the ‘get’ method to retrieve the value based on the key.
Understanding these fundamental concepts is crucial for mastering Java’s HashMap. With this knowledge, you can better understand how HashMap works under the hood and use it more effectively in your Java programming.
Expanding the Use of HashMap in Larger Projects
As you become more proficient with HashMap, you can start to apply it in larger, more complex projects. Two common use cases are creating a frequency map and caching data.
Frequency Map with HashMap
A frequency map is a data structure that maintains a count of all elements in an input collection. Here’s a simple example of how to use HashMap to create a frequency map:
String text = 'apple banana apple cherry banana banana';
String[] words = text.split(' ');
HashMap<String, Integer> frequencyMap = new HashMap<>();
for (String word : words) {
frequencyMap.put(word, frequencyMap.getOrDefault(word, 0) + 1);
}
System.out.println(frequencyMap);
// Output:
// {apple=2, banana=3, cherry=1}
In this example, we split a text into words and count the frequency of each word using a HashMap.
Caching Data with HashMap
HashMap can also be used to cache data, which can significantly improve the performance of your application. Here’s a simple example:
HashMap<String, Integer> cache = new HashMap<>();
cache.put('apple', 1);
// Later in the code
if (cache.containsKey('apple')) {
int value = cache.get('apple');
}
// Output:
// value = 1
In this example, we store a value in a HashMap and retrieve it later. This can be much faster than retrieving the value from a database or a file, especially in a large application.
Exploring Related Topics
As you continue to explore Java’s HashMap, you might also want to look into related topics, such as the Java Collections Framework, which includes other useful data structures, and concurrency issues with HashMap, which can be a critical consideration in multi-threaded applications.
Further Resources for Mastering Java’s HashMap
Here are some additional resources to help you delve deeper into the world of HashMap and related topics:
- Java Data Structures Overview – Explore fundamental data structures like arrays, lists, sets, and maps in Java.
Java HashCode Explained – Understand how to use hashCode() method in Java to generate hash values of objects.
Initializing HashMap in Java – Learn how to initialize and populate HashMaps in Java for key-value pair storage.
Oracle’s Official Documentation for HashMap is filled with detailed explanations of all methods provided by HashMap.
Baeldung’s Guide on Java HashMap contains numerous examples covering basic and advanced topics.
Java Code Geeks’ Tutorial on Java Collections covers the Java Collections Framework, including HashMap.
Wrapping Up: Java HashMap
In this comprehensive guide, we’ve unlocked the power of Java’s HashMap, a key part of the Java Collections Framework that allows you to store key-value pairs.
We began with the basics, exploring how to create a HashMap, add elements, retrieve values, and remove elements. We then delved into more advanced usage, such as iterating over a HashMap, sorting, and handling null values. We also introduced alternative Map implementations in Java, such as TreeMap and LinkedHashMap, and discussed when to use these alternatives.
Along the way, we tackled common issues you might encounter when using HashMap, such as handling collisions and understanding the impact of initial capacity and load factor. We provided solutions and best practices to help you overcome these challenges.
Here’s a quick comparison of the Map implementations we’ve discussed:
Map Implementation | Order Maintained | Null Keys/Values | Sorted Entries |
---|---|---|---|
HashMap | No | Yes | No |
TreeMap | Sorted by Key | No | Yes |
LinkedHashMap | Insertion Order | Yes | No |
Whether you’re just starting out with Java’s HashMap or you’re looking to level up your Java programming skills, we hope this guide has given you a deeper understanding of HashMap and its capabilities.
With its balance of flexibility, efficiency, and ease of use, HashMap is a powerful tool for data management in Java. Keep exploring, keep learning, and happy coding!