Java .hashCode() Method: Representing Objects with Integers
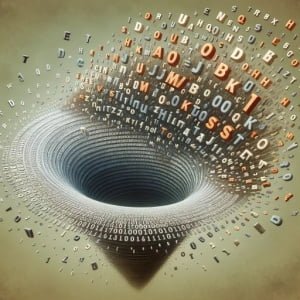
Ever found yourself puzzled over how Java’s hashCode method works? You’re not alone. Many developers find the concept of hashCode a bit challenging to grasp. Think of Java’s hashCode as a unique identifier – a tool that can be used to swiftly access data.
This guide will walk you through the ins and outs of Java’s hashCode method, from basic use to advanced techniques. We’ll cover everything from the basics of hashCode, how to implement it, to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Java’s hashCode!
TL;DR: What is Java’s hashCode method?
The
hashCode
method in Java is a built-in function used to return an integer hash code representing the value of the object, used with the syntax,int hash = targetString.hashCode();
. It plays a crucial role in data retrieval, especially when dealing with Java collections like HashMap and HashSet.
Here’s a simple example:
String str = 'Hello';
int hash = str.hashCode();
System.out.println(hash);
# Output:
# 69609650
In this example, we’ve created a string ‘Hello’ and used the hashCode method to generate a hash code for this string. The System.out.println(hash);
line then prints this hash code, which in this case is ‘69609650’.
This is just a basic way to use the hashCode method in Java, but there’s much more to learn about it. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Unraveling Java’s HashCode: A Beginner’s Guide
- Overriding Java’s HashCode: An Intermediate Guide
- Exploring Alternative HashCode Generators
- Troubleshooting Java’s HashCode: Common Pitfalls and Solutions
- Grasping the Fundamentals: Java’s Object Class and Hashing
- HashCode’s Role in Java Data Structures
- Exploring Related Concepts
- Wrapping Up: Mastering Java’s HashCode
Unraveling Java’s HashCode: A Beginner’s Guide
The hashCode method in Java is a built-in function from the Object class. Every class in Java inherits this method and can use or override it. The hashCode method is designed to return an integer that represents the value of the object. This integer is not unique, but it is generated in a way that helps minimize collisions (two different objects producing the same hash code).
Let’s take a look at a basic example:
String str1 = 'Hello';
String str2 = 'World';
int hash1 = str1.hashCode();
int hash2 = str2.hashCode();
System.out.println(hash1);
System.out.println(hash2);
# Output:
# 69609650
# 83766130
In this example, we have two different strings: ‘Hello’ and ‘World’. We use the hashCode method to generate two hash codes, which are then printed. As you can see, the two different strings produce two different hash codes.
The hashCode method is a great tool for quickly accessing data. For example, in a HashMap, the keys are stored as hash codes. When you want to retrieve a value, Java uses the key’s hash code to find it almost instantly, regardless of the size of the HashMap.
However, it’s important to note that the hashCode method is not without its pitfalls. For instance, because the generated hash code is not unique, different objects can potentially produce the same hash code, leading to collisions. This is a rare occurrence, but it’s something to be aware of when working with hash codes.
Overriding Java’s HashCode: An Intermediate Guide
As you delve deeper into Java, you’ll find scenarios where the default hashCode method doesn’t quite fit your needs. In such cases, you have the option to override the hashCode method in your own classes. Let’s look at an example:
class Student {
private int id;
private String name;
// constructor, getters, and setters omitted for brevity
@Override
public int hashCode() {
int result = 17;
result = 31 * result + id;
result = 31 * result + (name != null ? name.hashCode() : 0);
return result;
}
}
In this example, we’ve created a Student class with two fields: id and name. We then override the hashCode method to generate a hash code based on these fields. The prime numbers 17 and 31 are used to create a unique hash code, reducing the likelihood of collisions.
The Contract between Equals and HashCode
It’s important to understand the relationship between the equals() and hashCode() methods in Java. This relationship is often referred to as the ‘contract’ between equals and hashCode, and it consists of two rules:
- If two objects are equal according to the equals(Object) method, then calling the hashCode method on each of the two objects must produce the same integer result.
- If two objects are unequal according to the equals(Object) method, it is not required that calling the hashCode method on each of the two objects will produce distinct integer results. However, the programmer should be aware that producing distinct integer results for unequal objects may improve the performance of hash tables.
In simpler terms, if two objects are equal, their hash codes must also be equal. However, if two objects are not equal, their hash codes do not necessarily have to be different. This is why it’s important to override the hashCode method whenever you override the equals method, and vice versa, to maintain this contract.
Exploring Alternative HashCode Generators
While Java’s built-in hashCode method is widely used, there are alternative methods to generate hash codes, particularly useful in more complex scenarios. Two popular alternatives are Apache’s HashCodeBuilder and Google’s Guava library.
Apache’s HashCodeBuilder
Apache’s HashCodeBuilder is a part of the Apache Commons Lang library. It provides a simple and flexible way to generate hash codes. Here’s an example:
import org.apache.commons.lang3.builder.HashCodeBuilder;
class Student {
private int id;
private String name;
// constructor, getters, and setters omitted for brevity
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37)
.append(id)
.append(name)
.toHashCode();
}
}
In this example, we’re using HashCodeBuilder to generate the hash code for our Student class. We pass two numbers to the HashCodeBuilder constructor (these should be non-zero and odd for best results), and then append the fields we want to include in the hash code calculation.
Google’s Guava Library
Google’s Guava library also provides a utility for generating hash codes. Here’s how you can use it:
import com.google.common.base.Objects;
class Student {
private int id;
private String name;
// constructor, getters, and setters omitted for brevity
@Override
public int hashCode() {
return Objects.hashCode(id, name);
}
}
Guava’s Objects.hashCode method accepts any number of parameters and generates a hash code based on them.
Both Apache’s HashCodeBuilder and Google’s Guava library provide a flexible and efficient way to generate hash codes. They handle null values gracefully and allow you to easily include multiple fields in your hash code calculation. However, they do add an external dependency to your project, which is something to consider.
Troubleshooting Java’s HashCode: Common Pitfalls and Solutions
While Java’s hashCode method is a powerful tool, it’s not without its challenges. Let’s delve into some common issues you might encounter when working with hashCode, along with solutions and workarounds.
Collision Issues
One of the main issues with hashCode is the possibility of collisions. Since the hashCode method returns an integer, and there are a finite number of integer values, different objects can sometimes produce the same hash code. This is known as a collision.
Collisions can lead to performance issues, as Java has to then use the equals method to distinguish between the objects, which is slower than using the hash code.
Here’s an example of a collision:
String str1 = 'FB';
String str2 = 'Ea';
System.out.println(str1.hashCode());
System.out.println(str2.hashCode());
# Output:
# 64578
# 64578
In this example, the strings ‘FB’ and ‘Ea’ produce the same hash code, even though they are different strings. This is a collision.
One way to reduce the likelihood of collisions is to use a good hash function that takes into account all the data of the object. For example, if you’re generating a hash code for a class with several fields, make sure your hash function includes all the fields in its calculation.
Performance Issues
Another common issue with hashCode is performance. Generating a hash code can be a costly operation, especially for large objects. Moreover, if the hash code of an object changes after it’s been stored in a collection, it can lead to serious performance issues, as the object may not be found in its expected hash table bucket.
To avoid performance issues, it’s recommended to cache the hash code of an object, especially if calculating it is a complex operation. Also, make sure the hash code of an object doesn’t change once it’s been stored in a collection.
Here’s an example of caching a hash code:
class Student {
private int id;
private String name;
private int hashCode;
// constructor, getters, and setters omitted for brevity
@Override
public int hashCode() {
if (hashCode == 0) {
hashCode = new HashCodeBuilder(17, 37)
.append(id)
.append(name)
.toHashCode();
}
return hashCode;
}
}
In this example, we’re caching the hash code of a Student object. The first time hashCode is called, it calculates the hash code and stores it in the hashCode field. Subsequent calls to hashCode return the cached value, avoiding the cost of recalculating the hash code.
Grasping the Fundamentals: Java’s Object Class and Hashing
To truly understand the workings of the hashCode method, it’s essential to delve into two fundamental concepts: Java’s Object class and the concept of hashing.
Java’s Object Class
In Java, every class implicitly or explicitly extends the Object class. This means that every class in Java has the Object class as a superclass and can access its methods. The hashCode method is one of these methods. It’s a native method in the Object class, designed to return an integer representation of the memory address of the object.
When you create a class in Java and don’t override the hashCode method, your class will inherit the Object class’s hashCode method. This default hashCode method can serve many purposes, but it might not be the most efficient for your specific needs, which is why you might choose to override it.
The Concept of Hashing
Hashing is a concept used in many fields, including computer science, cryptography, and data retrieval. It involves taking input (in our case, an object), and returning a fixed-size string of bytes, typically in the form of an integer. The output is called a hash code.
The main goal of a hash function is to distribute the input across a range of potential outputs in such a way that similar inputs produce different outputs. This is important for data retrieval, as it allows us to access data quickly.
Here’s a simple example of a hash function:
int hashCode(String s) {
int hash = 0;
for (int i = 0; i < s.length(); i++) {
hash += s.charAt(i);
}
return hash;
}
String str = 'Hello';
System.out.println(hashCode(str));
# Output:
# 500
In this example, we’ve created a simple hash function that calculates the sum of the ASCII values of the characters in a string. The string ‘Hello’ produces a hash code of 500.
Understanding Java’s Object class and the concept of hashing is fundamental to understanding the hashCode method. With these concepts in mind, you can better appreciate the role and workings of the hashCode method.
HashCode’s Role in Java Data Structures
The hashCode method plays a crucial role in Java’s data structures, particularly in collections like HashMap and HashSet. These collections use the hashCode method to store and retrieve data quickly and efficiently.
For instance, in a HashMap, data is stored in key-value pairs. When storing data, the HashMap uses the key’s hashCode to determine where to store the value. When retrieving data, it uses the key’s hashCode again to find the value almost instantly. This makes operations like get and put very efficient in HashMap.
Similarly, HashSet, which is a collection of unique elements, uses the hashCode method to store elements. It uses the element’s hashCode to determine where to store the element in memory. This allows HashSet to quickly check if an element already exists in the set.
Exploring Related Concepts
Understanding the hashCode method opens the door to other related concepts in Java, such as the equals method and hash functions.
The equals method is often used in conjunction with the hashCode method. While the hashCode method is used for initial data retrieval, the equals method is used for an exact match. This is particularly important in cases where two different objects produce the same hash code.
Hash functions, on the other hand, are a broader concept used in many fields, including cryptography and data retrieval. In Java, the hashCode method is a type of hash function that takes an object and returns an integer.
Further Resources for Mastering Java’s hashCode
To deepen your understanding of Java’s hashCode method and related concepts, here are some resources that you might find helpful:
- Use Cases Explored: HashMap in Java – Discover how to serialize and deserialize HashMaps in Java for data storage.
Java LinkedHashMap Overview – Master using LinkedHashMap for maintaining insertion order in Java applications.
Rehashing in Java – Learn about rehashing, a process in Java HashMaps where the size of the underlying array is adjusted.
Java’s Official Documentation – A guide to Java’s classes and methods, including the hashCode method.
Oracle’s Java Tutorials covers various topics in Java, including collections, hash functions, and more.
Baeldung’s Guide to Java hashCode covers the hashCode method, including how to use it and how to override it.
Wrapping Up: Mastering Java’s HashCode
In this comprehensive guide, we’ve delved into the details of Java’s hashCode method, from understanding its basic use to implementing advanced techniques.
We began with the basics, shedding light on what Java’s hashCode method is and how it works. We then moved on to more advanced topics, such as how to override the hashCode method in your own classes and the relationship between equals and hashCode methods. We also touched upon the alternative methods to generate hash codes, such as using Apache’s HashCodeBuilder or Google’s Guava library.
Along the way, we tackled common issues you might encounter when working with hashCode, such as collisions and performance issues, and provided solutions and workarounds for each issue. We also explored the background and fundamentals of Java’s Object class and the concept of hashing to give you a better understanding of the underlying principles of the hashCode method.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Java’s hashCode | Built-in, easy to use | Possibility of collisions |
Apache’s HashCodeBuilder | Flexible, handles null values | External dependency |
Google’s Guava | Flexible, handles null values | External dependency |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of its hashCode method, we hope this guide has been a helpful resource. With the knowledge you’ve gained, you’re now well-equipped to use and implement Java’s hashCode method effectively and efficiently. Happy coding!