LinkedHashMap | Guide to Java’s Hybrid Data Structure
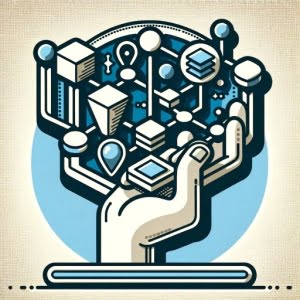
Are you finding it challenging to understand and use LinkedHashMap in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling this hybrid data structure, but we’re here to help.
Think of LinkedHashMap as a bridge – a bridge that combines the best of HashMap and LinkedList. It’s a powerful tool that can help you handle data in a more efficient and predictable way.
In this guide, we’ll walk you through the process of understanding and using LinkedHashMap in Java, from the basics to more advanced techniques. We’ll cover everything from creating and manipulating LinkedHashMaps, to discussing alternative approaches and troubleshooting common issues.
Let’s dive in and start mastering LinkedHashMap in Java!
TL;DR: What is a LinkedHashMap in Java?
A
LinkedHashMap
in Java is a hybrid data structure that implements the Map interface using a hash table and a linked list, providing predictable iteration order. It is instantiated with the syntax,LinkedHashMap<Integer, String> map = new LinkedHashMap<>();
, and elements are mainted with the.put()
method. This means you can access and manipulate key-value pairs in the order they were inserted, unlike a regular HashMap.
Here’s a simple example of how to use it:
LinkedHashMap<Integer, String> map = new LinkedHashMap<>();
map.put(1, 'one');
map.put(2, 'two');
System.out.println(map);
# Output:
# {1=one, 2=two}
In this example, we create a LinkedHashMap map
and insert two key-value pairs into it. The keys are integers and the values are strings. When we print the map, we see the key-value pairs in the order they were inserted.
This is just a basic way to use LinkedHashMap in Java, but there’s much more to learn about this versatile data structure. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with LinkedHashMap
- Leveraging LinkedHashMap’s Unique Methods
- Exploring Alternatives to LinkedHashMap
- Navigating LinkedHashMap Pitfalls and Performance Issues
- Unpacking Java’s Map Interface and LinkedHashMap
- The Power of LinkedHashMap in Real-World Applications
- Wrapping Up: LinkedHashMap in Java
Getting Started with LinkedHashMap
Let’s start with the basics. A LinkedHashMap is a Map, meaning it stores key-value pairs. The keys are unique, and each key maps to a specific value. However, unlike a regular HashMap, a LinkedHashMap also maintains the order in which keys were inserted. This is where it gets its name – it’s a HashMap that is also a LinkedList.
Here’s a simple example of how to create and use a LinkedHashMap:
LinkedHashMap<Integer, String> map = new LinkedHashMap<>();
map.put(1, 'one');
map.put(2, 'two');
map.put(3, 'three');
System.out.println(map);
# Output:
# {1=one, 2=two, 3=three}
In this example, we create a LinkedHashMap map
and insert three key-value pairs into it. The keys are integers and the values are strings. When we print the map, we see the key-value pairs in the order they were inserted.
This is one of the main advantages of a LinkedHashMap over a regular HashMap. With a HashMap, the order of the keys is not guaranteed, which can lead to unpredictable results. But with a LinkedHashMap, you can always rely on the keys being in the order in which they were inserted.
However, there’s a potential pitfall to be aware of. Because a LinkedHashMap maintains this order, it uses slightly more memory than a regular HashMap. This is usually not a problem, but it’s something to keep in mind if you’re working with a very large amount of data.
Leveraging LinkedHashMap’s Unique Methods
Once you’ve mastered the basics of LinkedHashMap, you can start to explore its more advanced features. LinkedHashMap provides several specific methods that can make your code more efficient and easier to read.
The getOrDefault Method
The getOrDefault
method is a powerful tool that can simplify your code. It retrieves the value for a given key, but if that key doesn’t exist, it returns a default value.
Here’s an example:
LinkedHashMap<Integer, String> map = new LinkedHashMap<>();
map.put(1, 'one');
map.put(2, 'two');
System.out.println(map.getOrDefault(3, 'unknown'));
# Output:
# unknown
In this example, we try to retrieve the value for the key 3
. But since we haven’t inserted a key-value pair with the key 3
, the getOrDefault
method returns the default value 'unknown'
.
The forEach Method
The forEach
method is another useful tool. It allows you to iterate over the LinkedHashMap and perform an action for each key-value pair.
Here’s an example:
LinkedHashMap<Integer, String> map = new LinkedHashMap<>();
map.put(1, 'one');
map.put(2, 'two');
map.forEach((key, value) -> System.out.println(key + "=" + value));
# Output:
# 1=one
# 2=two
In this example, we use the forEach
method to print each key-value pair in the LinkedHashMap. The forEach
method takes a lambda expression, which is a short block of code that is executed for each element in the LinkedHashMap.
The replaceAll Method
The replaceAll
method is a powerful tool that allows you to update all values in the LinkedHashMap based on the old values.
Here’s an example:
LinkedHashMap<Integer, String> map = new LinkedHashMap<>();
map.put(1, 'one');
map.put(2, 'two');
map.replaceAll((key, value) -> value.toUpperCase());
System.out.println(map);
# Output:
# {1=ONE, 2=TWO}
In this example, we use the replaceAll
method to change all the values in the LinkedHashMap to uppercase. The replaceAll
method takes a lambda expression, which is a short block of code that is executed for each value in the LinkedHashMap.
These are just a few examples of the powerful methods provided by LinkedHashMap. By leveraging these methods, you can write more efficient and readable code.
Exploring Alternatives to LinkedHashMap
While LinkedHashMap is a powerful and versatile data structure, it’s not always the best tool for the job. Depending on your needs, other data structures such as TreeMap or HashMap might be more suitable.
HashMap: The Lightweight Alternative
HashMap is the most basic implementation of the Map interface. It doesn’t maintain any order of its keys, which makes it slightly more efficient in terms of memory usage.
Here’s an example of how to use a HashMap:
HashMap<Integer, String> map = new HashMap<>();
map.put(1, 'one');
map.put(2, 'two');
System.out.println(map);
# Output:
# {1=one, 2=two}
In this example, we create a HashMap and insert two key-value pairs. Notice that the output doesn’t guarantee any specific order of the keys.
TreeMap: Order by Key
TreeMap is another implementation of the Map interface. Unlike HashMap and LinkedHashMap, TreeMap sorts its keys based on their natural ordering, or by a custom Comparator provided at map creation time.
Here’s an example of how to use a TreeMap:
TreeMap<Integer, String> map = new TreeMap<>();
map.put(2, 'two');
map.put(1, 'one');
System.out.println(map);
# Output:
# {1=one, 2=two}
In this example, we create a TreeMap and insert two key-value pairs. Notice that the output is sorted by the keys.
Both HashMap and TreeMap have their uses, and choosing between them, LinkedHashMap, or another data structure entirely, depends on the specific requirements of your project. If you need to maintain insertion order, LinkedHashMap is the way to go. If you don’t care about order and want to save some memory, consider HashMap. If you need to keep your keys sorted, TreeMap might be your best bet.
While LinkedHashMap is a powerful tool, it’s not without its quirks and potential issues. Let’s discuss some of the common problems you might encounter when using LinkedHashMap, along with their solutions and some handy tips.
Memory Usage Considerations
One of the primary considerations when using LinkedHashMap is its memory usage. LinkedHashMap maintains an order of keys, which requires additional memory compared to a regular HashMap. This is usually not a concern for small to medium-sized datasets, but it can become a problem when dealing with large amounts of data.
Consider the following example:
LinkedHashMap<Integer, String> map = new LinkedHashMap<>(1000000);
for (int i = 0; i < 1000000; i++) {
map.put(i, 'value' + i);
}
System.out.println('Map size: ' + map.size());
# Output:
# Map size: 1000000
In this example, we create a LinkedHashMap with one million entries. This map will use significantly more memory than a regular HashMap with the same number of entries. If memory usage is a concern, consider using a HashMap or another data structure that doesn’t maintain an order of keys.
Performance Issues
LinkedHashMap provides constant-time performance for the basic operations (get and put), just like HashMap. However, performance can degrade to O(n) for operations that need to iterate over the map, such as toString
or clear
, because these operations need to traverse the linked list of entries.
Here’s an example that illustrates this:
LinkedHashMap<Integer, String> map = new LinkedHashMap<>(1000000);
for (int i = 0; i < 1000000; i++) {
map.put(i, 'value' + i);
}
long start = System.currentTimeMillis();
map.clear();
long end = System.currentTimeMillis();
System.out.println('Time taken to clear the map: ' + (end - start) + ' ms');
# Output:
# Time taken to clear the map: X ms
In this example, we measure the time it takes to clear a LinkedHashMap with one million entries. Depending on your system, this operation can take a noticeable amount of time. If performance is a concern, consider using a data structure that provides better performance for these operations, such as HashMap.
Unpacking Java’s Map Interface and LinkedHashMap
To truly understand LinkedHashMap, we need to delve into the fundamentals of Java’s Map interface and the underlying data structures that LinkedHashMap utilizes: the hash table and linked list.
The Map Interface in Java
In Java, Map is an interface that belongs to the Java Collections Framework. It maps keys to values, meaning each key is associated with one value. The keys are unique, but the values can be duplicated.
Here’s a simple example of a Map in Java:
Map<Integer, String> map = new HashMap<>();
map.put(1, 'one');
map.put(2, 'two');
System.out.println(map);
# Output:
# {1=one, 2=two}
In this example, we create a Map and insert two key-value pairs. The keys are integers and the values are strings. When we print the map, we see the key-value pairs, but the order in which they were inserted is not maintained.
The LinkedHashMap Implementation
LinkedHashMap is a class in Java that implements the Map interface. It uses a hash table and a linked list to store the key-value pairs, which allows it to maintain the order of the keys.
The hash table provides constant-time performance for the basic operations (get and put), while the linked list maintains the insertion order of the keys. This combination of features makes LinkedHashMap a versatile and efficient data structure.
Here’s an example of a LinkedHashMap in Java:
LinkedHashMap<Integer, String> map = new LinkedHashMap<>();
map.put(1, 'one');
map.put(2, 'two');
System.out.println(map);
# Output:
# {1=one, 2=two}
In this example, we create a LinkedHashMap and insert two key-value pairs. When we print the map, we see the key-value pairs in the order they were inserted, unlike the previous example with the Map.
By understanding the Map interface and the underlying data structures of LinkedHashMap, you can leverage its features to write more efficient and predictable code.
The Power of LinkedHashMap in Real-World Applications
LinkedHashMap is more than just a theoretical concept. It’s a practical tool that’s used in a variety of real-world applications. Let’s take a closer look at how LinkedHashMap can be used in practice, and what related concepts you might want to explore next.
LinkedHashMap for Caching
One common use of LinkedHashMap is in caching. Because LinkedHashMap maintains the order of its keys, it’s easy to implement a Least Recently Used (LRU) cache with it. In an LRU cache, the least recently used items are removed when the cache is full. This is a common strategy in memory management.
Here’s an example of how you might implement an LRU cache with LinkedHashMap:
int cacheSize = 5;
LinkedHashMap<Integer, String> cache = new LinkedHashMap<>(cacheSize, 0.75f, true) {
protected boolean removeEldestEntry(Map.Entry<Integer, String> eldest) {
return size() > cacheSize;
}
};
for (int i = 1; i <= 10; i++) {
cache.put(i, 'value' + i);
}
System.out.println(cache);
# Output:
# {6=value6, 7=value7, 8=value8, 9=value9, 10=value10}
In this example, we create a cache with a maximum size of 5. When we insert 10 items, the first 5 items are automatically removed to maintain the size limit. This is because we override the removeEldestEntry
method to remove the oldest entry when the size exceeds the cache size.
LinkedHashMap for Maintaining Insertion Order
Another use of LinkedHashMap is when you need to maintain the insertion order of your keys. This can be useful in a variety of scenarios, such as when you’re processing records in a specific order.
Further Resources for LinkedHashMaps
To further your understanding of LinkedHashMap and related concepts, check out the following resources:
- Getting Started with Java HashMap Tutorial – Dive into HashMap implementation details and performance in Java.
Exploring Java HashCode – Master generating reliable hash codes to optimize performance and integrity in Java.
Hash Tables in Java – Dive into the concept of hash tables in Java for fast data retrieval based on keys.
Java Collections Tutorial covers all aspects of the Java Collections Framework, including LinkedHashMap.
Java Generics Tutorial provides a deep dive into Java generics, which are often used with collections like LinkedHashMap.
Java LinkedHashMap Documentation provides a detailed overview of all the methods and features of LinkedHashMap.
Wrapping Up: LinkedHashMap in Java
In this comprehensive guide, we’ve journeyed through the intricacies of LinkedHashMap in Java, a hybrid data structure that combines the best of HashMap and LinkedList.
We began with the basics, introducing LinkedHashMap and demonstrating how to use it at a beginner level. We then delved into more advanced usage, exploring the unique methods that LinkedHashMap provides, such as getOrDefault
, forEach
, and replaceAll
.
We also tackled common challenges you might encounter when using LinkedHashMap, such as memory usage considerations and performance issues, providing you with solutions and workarounds for each problem. Additionally, we discussed alternative approaches to data manipulation in Java, comparing LinkedHashMap with other data structures like HashMap and TreeMap.
Here’s a quick comparison of these data structures:
Data Structure | Order Maintained | Memory Usage | Performance |
---|---|---|---|
LinkedHashMap | Insertion Order | High | High |
HashMap | No Order | Moderate | High |
TreeMap | Sorted Order | High | Moderate |
Whether you’re just starting out with LinkedHashMap or looking to deepen your understanding, we hope this guide has equipped you with the knowledge and skills to use LinkedHashMap effectively.
With its balance of order maintenance, memory usage, and performance, LinkedHashMap is a powerful tool for data manipulation in Java. Now, you’re well prepared to harness its capabilities in your own projects. Happy coding!