Mastering Java Regex: Guide to Pattern Matching and More
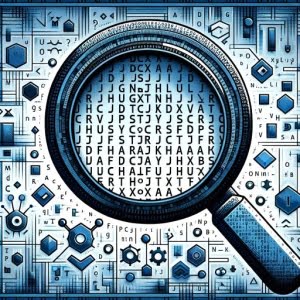
Are you finding it difficult to match patterns in Java? You’re not alone. Many developers find Java’s regex (short for regular expressions) a bit of a puzzle, but we’re here to help.
Think of Java’s regex as a detective – it can help you find patterns in your data, making it an invaluable tool for various tasks.
In this guide, we’ll walk you through the basics of Java regex and even delve into advanced usage scenarios. We’ll cover everything from using the Pattern
and Matcher
classes, to handling complex regex patterns and alternative approaches.
So, let’s get started and master Java regex!
TL;DR: How Do I Use Regex in Java?
To utilize Regex you must first import the
java.util.regex
package with,import java.util.regex.*;
. Then you will need to define thePattern
andMatcher
classes with the syntax,Pattern pattern = Pattern.compile('initialPattern')
andMatcher matcher = pattern.matcher('patternto');
.
Here’s a simple example:
import java.util.regex.*;
Pattern pattern = Pattern.compile('ab');
Matcher matcher = pattern.matcher('abc');
boolean matches = matcher.matches();
// Output:
// true
In this example, we import the java.util.regex
package and create a Pattern
object by compiling a simple regex pattern ‘ab’. We then create a Matcher
object by applying the pattern to a string ‘abc’. The matches()
method checks if the string matches the pattern, and in this case, it returns true because ‘abc’ starts with ‘ab’.
This is just a basic way to use regex in Java, but there’s much more to learn about pattern matching and handling complex regex patterns. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
Basic Use of Java Regex
Java Regex, or Regular Expressions, is a powerful tool that allows you to match, locate, and manage text. The java.util.regex
package provides the necessary classes for pattern matching using regex in Java. The two main classes we’ll focus on are Pattern
and Matcher
.
The Pattern
Class
The Pattern
class, as the name suggests, represents a compiled representation of a regular expression. You create a Pattern
object by invoking the Pattern.compile()
method and passing the regex pattern as a string.
The Matcher
Class
The Matcher
class, on the other hand, is the engine that interprets the pattern and performs match operations against an input string. You create a Matcher
object by invoking the matcher()
method on a Pattern
object.
Let’s see these classes in action:
import java.util.regex.*;
// Create a Pattern object
Pattern pattern = Pattern.compile('ab');
// Create a Matcher object
Matcher matcher = pattern.matcher('abcde');
// Check if the pattern matches the input string
boolean matches = matcher.matches();
System.out.println(matches);
// Output:
// false
The above code does not return a match because the matches()
method attempts to match the entire input string against the pattern. Our pattern ‘ab’ does not match the entire string ‘abcde’, hence, it returns false.
If you want to check if the input string contains the pattern, you can use the find()
method of the Matcher
class:
boolean found = matcher.find();
System.out.println(found);
// Output:
// true
The find()
method scans the input string for the pattern ‘ab’ and returns true because ‘ab’ is found in ‘abcde’.
Advanced Java Regex Patterns
As you become more comfortable with Java regex, you can start exploring more complex patterns. These include quantifiers, character classes, and boundary matchers.
Harnessing Quantifiers
Quantifiers determine how many instances of a character, group, or character class must be present in the input for a match to be found. Here are some common quantifiers:
*
Zero or more times+
One or more times?
Zero or one time{n}
Exactly n times{n,}
At least n times{n,m}
At least n but not more than m times
Here’s an example of how to use quantifiers:
Pattern pattern = Pattern.compile('a*');
Matcher matcher = pattern.matcher('aaaaab');
boolean matches = matcher.matches();
System.out.println(matches);
// Output:
// false
In the above code, the pattern ‘a*’ matches zero or more ‘a’ characters. However, the matches()
method returns false because it tries to match the entire string, and our string ends with ‘b’.
Exploring Character Classes
Character classes represent a set of characters, and any character from the set can match. For instance, [abc]
matches ‘a’, ‘b’, or ‘c’.
Pattern pattern = Pattern.compile('[abc]*');
Matcher matcher = pattern.matcher('abc');
boolean matches = matcher.matches();
System.out.println(matches);
// Output:
// true
In this example, the pattern ‘[abc]*’ matches any sequence (including an empty one) of ‘a’, ‘b’, or ‘c’ characters. Therefore, the string ‘abc’ matches the pattern.
Utilizing Boundary Matchers
Boundary matchers help you find a particular word or a pattern at the start or end of a line. Some common boundary matchers are ^
(start of a line) and $
(end of a line).
Pattern pattern = Pattern.compile('^a.*b$');
Matcher matcher = pattern.matcher('abc');
boolean matches = matcher.matches();
System.out.println(matches);
// Output:
// true
In this example, the pattern ‘^a.*b$’ matches any string that starts with ‘a’ and ends with ‘b’. Therefore, the string ‘abc’ matches the pattern.
Alternative Pattern Matching Methods in Java
Java offers more ways to perform pattern matching beyond the Pattern
and Matcher
classes. Let’s explore two such alternatives: the String.matches()
method and third-party libraries like Apache Commons Lang.
Java’s String.matches()
Method
Java’s String class has a matches()
method that takes a regex pattern as a parameter and checks if the string matches the pattern. It’s a quick and easy way to perform pattern matching, especially for simple patterns.
String str = 'abc';
boolean matches = str.matches('abc');
System.out.println(matches);
// Output:
// true
In the example above, we use the matches()
method of the String class to check if the string ‘abc’ matches the pattern ‘abc’. The method returns true, indicating a match.
Apache Commons Lang Library
Apache Commons Lang is a third-party library that provides helper utilities for the java.lang API, including pattern matching. The StringUtils
class, for example, has several methods for string manipulation and pattern matching.
import org.apache.commons.lang3.StringUtils;
String str = 'abc';
boolean contains = StringUtils.contains(str, 'a');
System.out.println(contains);
// Output:
// true
In this example, we use the contains()
method of the StringUtils
class to check if the string ‘abc’ contains the character ‘a’. The method returns true, indicating the character is present.
Here’s a comparison of the three methods we’ve discussed:
Method | Complexity | Use Case |
---|---|---|
Pattern and Matcher classes | High | Powerful and flexible, suitable for complex pattern matching |
String.matches() method | Medium | Quick and easy, suitable for simple pattern matching |
Apache Commons Lang | Low | Provides many helper methods, suitable for string manipulation and pattern matching |
These alternative methods provide additional flexibility when working with Java regex, allowing you to choose the method that best suits your use case.
Troubleshooting Java Regex
While Java regex is a powerful tool, it does come with its own set of challenges. Let’s discuss some common issues you might encounter when using regex in Java, and how to solve them.
Pattern Syntax Exceptions
A PatternSyntaxException
is a type of unchecked exception that Java throws when the syntax of the regex pattern is incorrect.
try {
Pattern pattern = Pattern.compile('*ab');
} catch (PatternSyntaxException e) {
System.out.println('Invalid regex pattern');
}
// Output:
// Invalid regex pattern
In this example, the pattern ‘ab’ is invalid because the ‘‘ quantifier doesn’t have a valid preceding expression. The Pattern.compile()
method throws a PatternSyntaxException
, which we catch and handle by printing a custom error message.
Performance Considerations
Regex can be slow, especially for complex patterns or long strings. This can impact your application’s performance. One way to mitigate this is by using the Pattern
class’s compile()
method to create a precompiled pattern. This pattern can then be reused, saving the cost of compiling the pattern each time it’s used.
Pattern pattern = Pattern.compile('ab');
for (int i = 0; i < 1000; i++) {
Matcher matcher = pattern.matcher('abc' + i);
boolean matches = matcher.matches();
}
In the above example, we compile the pattern ‘ab’ once and reuse it in a loop. This is more efficient than compiling the pattern inside the loop.
Remember, while Java regex is powerful, it’s not always the best tool for the job. If you’re dealing with simple patterns or string manipulation tasks, consider using String methods or third-party libraries like Apache Commons Lang.
Understanding Regular Expressions
Regular Expressions, commonly known as regex, are a powerful tool in programming that allow for complex pattern matching and manipulation of text. They are essentially a sequence of characters that form a search pattern.
The Syntax of Regex
Regex has its own unique syntax that can be broken down into several elements:
- Literal Characters: These are standard characters that match themselves exactly.
Metacharacters: These characters have special meanings, like
{}
,()
,.
,^
,$
,|
,*
,+
,?
, and[]
.Quantifiers: These define how many times a character, group, or character class must be present for a match. Examples include
{}
,*
,+
, and?
.Character Classes: These represent a set of characters. For instance,
[abc]
matches any character that is either ‘a’, ‘b’, or ‘c’.Escape Sequences: These are denoted by a backslash (
\
) followed by the character you want to match. For instance,\d
matches any digit.
Here’s a simple example of a regex pattern:
Pattern pattern = Pattern.compile('[a-z]+');
Matcher matcher = pattern.matcher('abc');
boolean matches = matcher.matches();
System.out.println(matches);
// Output:
// true
In this example, the pattern [a-z]+
matches any sequence of one or more lowercase letters. The string ‘abc’ matches this pattern, so the matches()
method returns true.
Structure of Regex Patterns
Regex patterns can be simple, matching specific sequences of characters, or complex, using metacharacters and quantifiers to match a wide range of strings. The structure of your regex pattern will depend on what you’re trying to achieve.
Understanding the fundamentals of regex is crucial when working with Java regex. It provides the foundation upon which you can build more complex pattern matching scenarios and effectively manipulate text in your Java programs.
The Power of Java Regex Beyond Pattern Matching
Java regex is not just about pattern matching. It’s a powerful tool that can be used in various other aspects of programming, such as data validation, search and replace operations, and text processing.
Data Validation with Java Regex
Data validation is a common use case for regex. You can use regex to check if a string matches a specific format, such as an email address or phone number.
Pattern pattern = Pattern.compile('^\w+@[a-zA-Z_]+?\.[a-zA-Z]{2,3}$');
Matcher matcher = pattern.matcher('[email protected]');
boolean matches = matcher.matches();
System.out.println(matches);
// Output:
// true
In this example, we use regex to validate an email address. The pattern ^\w+@[a-zA-Z_]+?\.[a-zA-Z]{2,3}$
checks if the string is a valid email address, and in this case, ‘[email protected]’ is valid, so the matches()
method returns true.
Search and Replace Operations
Regex can also be used to perform search and replace operations in a string. For instance, you can use the replaceAll()
method of the String
class to replace all occurrences of a pattern in a string.
String str = 'The quick brown fox jumps over the lazy dog.';
String replacedStr = str.replaceAll('fox', 'cat');
System.out.println(replacedStr);
// Output:
// 'The quick brown cat jumps over the lazy dog.'
In this example, we use regex to replace ‘fox’ with ‘cat’ in the string.
Text Processing with Java Regex
Regex is also useful for text processing tasks, such as splitting a string into tokens. You can use the split()
method of the String
class to split a string around matches of a regex pattern.
String str = 'apple,banana,cherry';
String[] fruits = str.split(',');
for (String fruit : fruits) {
System.out.println(fruit);
}
// Output:
// 'apple'
// 'banana'
// 'cherry'
In this example, we use regex to split a string into an array of substrings.
Further Resources for Mastering Java Regex
If you’re interested in learning more about Java regex, here are some resources you might find helpful:
- Exploring Java String Manipulation: Essential Techniques – Learn essential methods for working with strings in Java.
Finding Substrings in Java – Master using indexOf() for string manipulation, searching, and pattern matching in Java
Patterns in Java – Learn about the Pattern class in Java for compiling and working with regular expressions.
Java’s Official Regular Expressions Tutorial – Gain official insights into handling regular expressions in Java.
Java Regex Tutorial by Tutorialspoint covers handling Java regular expressions.
Java Regular Expressions – Explore regular expressions in Java with Baeldung’s expert guide.
These resources provide in-depth tutorials and examples that can help you master Java regex.
Wrapping Up: Regex in Java
In this comprehensive guide, we’ve delved deep into the world of Java Regex, a powerful tool for pattern matching and text manipulation in Java.
We began with the basics, learning how to use the Pattern
and Matcher
classes for simple pattern matching. We then ventured into more advanced territory, exploring complex regex patterns using quantifiers, character classes, and boundary matchers. We also discussed alternative approaches, such as Java’s String.matches()
method and third-party libraries like Apache Commons Lang.
Along the way, we tackled common challenges you might face when using Java regex, such as pattern syntax exceptions and performance considerations, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Complexity | Use Case |
---|---|---|
Pattern and Matcher classes | High | Powerful and flexible, suitable for complex pattern matching |
String.matches() method | Medium | Quick and easy, suitable for simple pattern matching |
Apache Commons Lang | Low | Provides many helper methods, suitable for string manipulation and pattern matching |
Whether you’re just starting out with Java regex or you’re looking to level up your pattern matching skills, we hope this guide has given you a deeper understanding of Java regex and its capabilities.
With its balance of power, flexibility, and ease of use, Java regex is an invaluable tool for any Java developer. Happy coding!