Java’s String IndexOf Method: A Detailed Usage Guide
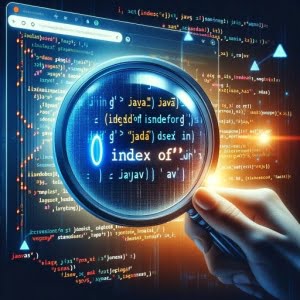
Are you finding it challenging to locate the index of a character or substring in Java? You’re not alone. Many developers find themselves in a similar situation, but there’s a method in Java that can make this task straightforward.
Think of Java’s indexOf() method as a detective – it can help you pinpoint the exact position of your target in a string. This method is a powerful tool, providing a versatile and handy solution for various tasks.
In this guide, we’ll walk you through the process of using the indexOf() method in Java, from its basic usage to more advanced techniques. We’ll cover everything from the fundamentals of string indexing to handling common issues and their solutions.
Let’s dive in and start mastering indexOf() in Java!
TL;DR: How Do I Use the indexOf() Method in Java?
The indexOf() method in Java is used to find the index of a character or substring in a string, used with the syntax:
int index = targetString.indexOf('characterToFind')
It returns the position of the first occurrence of the specified character or substring. If the character or substring is not found, it returns -1.
Here’s a simple example:
String str = 'Hello, World!';
int index = str.indexOf('World');
System.out.println(index);
// Output:
// 7
In this example, we have a string ‘Hello, World!’. We use the indexOf() method to find the index of the substring ‘World’. The method returns 7, which is the position of the first character of ‘World’ in the string.
This is a basic way to use the indexOf() method in Java, but there’s much more to learn about it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use of indexOf() in Java
The indexOf() method in Java is a part of the String class and is used to find the position of a character or substring in a string. The method returns the index within this string of the first occurrence of the specified character or substring. If it does not occur as a substring, -1 is returned.
Let’s take a look at a basic example of how to use the indexOf() method:
String str = 'Hello, World!';
int index = str.indexOf('Hello');
System.out.println(index);
// Output:
// 0
In this example, we have a string ‘Hello, World!’. We use the indexOf() method to find the index of the substring ‘Hello’. Since ‘Hello’ starts from the start of the string, the returned index is 0.
Advantages of Using indexOf()
Using indexOf() method has several advantages:
- Simplicity: The indexOf() method is straightforward and easy to use. It only requires the character or substring you’re looking for as an argument.
Versatility: This method can be used to find the position of a character or a substring, making it a versatile tool in string manipulation tasks.
Efficiency: It provides an efficient way to find the index of a character or substring without having to loop through the string manually.
Pitfalls to Be Aware Of
While the indexOf() method is handy, there are a few things to keep in mind:
- Case Sensitivity: The indexOf() method is case-sensitive. It treats uppercase and lowercase letters as different characters. So, ‘A’ and ‘a’ will have different indexes.
No Match Scenario: If the character or substring is not found in the string, the method will return -1. It’s important to handle this scenario in your code to prevent any unexpected behavior.
First Occurrence Only: The method only returns the index of the first occurrence of the character or substring. If you need to find subsequent occurrences, you’ll have to use different techniques, which we will cover in the advanced use section.
Advanced Usage of indexOf() in Java
The indexOf() method also supports more advanced usage. Specifically, it allows you to specify a starting index to begin the search. This can be extremely useful when you want to find subsequent occurrences of a character or substring in a string.
Let’s look at an example:
String str = 'Hello, World! Hello, Universe!';
int firstIndex = str.indexOf('Hello');
int secondIndex = str.indexOf('Hello', firstIndex + 1);
System.out.println(firstIndex);
System.out.println(secondIndex);
// Output:
// 0
// 14
In this example, we first find the index of the first occurrence of ‘Hello’. Then, we use that index to find the second occurrence of ‘Hello’. We add 1 to the first index to start the search after the first occurrence.
This illustrates how you can use the indexOf() method to find multiple occurrences of a character or substring in a string. The ability to specify a starting index gives you more control over your search and enables more complex string manipulation tasks.
Exploring Alternative Approaches
While the indexOf() method is a powerful tool for finding the index of a character or substring in a string, Java provides other methods that you can use for similar tasks. Let’s take a look at one such alternative: the lastIndexOf() method.
Using lastIndexOf()
The lastIndexOf() method works similarly to indexOf(), but instead of returning the index of the first occurrence of a character or substring, it returns the index of the last occurrence. This can be particularly useful when you’re dealing with strings that contain multiple occurrences of the same character or substring.
Here’s an example:
String str = 'Hello, World! Hello, Universe!';
int lastIndex = str.lastIndexOf('Hello');
System.out.println(lastIndex);
// Output:
// 14
In this example, the lastIndexOf() method returns 14, which is the index of the first character of the last occurrence of ‘Hello’ in the string.
Comparing indexOf() and lastIndexOf()
Both indexOf() and lastIndexOf() are useful methods for finding the index of a character or substring in a string. The key difference is that indexOf() returns the first occurrence, while lastIndexOf() returns the last occurrence.
Method | Advantage | Disadvantage |
---|---|---|
indexOf() | Finds first occurrence | Cannot directly find last occurrence |
lastIndexOf() | Finds last occurrence | Cannot directly find first occurrence |
In the end, the choice between indexOf() and lastIndexOf() depends on your specific needs. If you need to find the first occurrence of a character or substring, use indexOf(). If you need to find the last occurrence, use lastIndexOf().
Troubleshooting Common indexOf() Issues
While using the indexOf() method in Java, you might encounter some common issues. Let’s discuss these problems and their solutions to ensure a smooth coding experience.
Dealing with ‘StringIndexOutOfBoundsException’
One of the common issues you might encounter is the ‘StringIndexOutOfBoundsException’. This exception is thrown by methods in the String class when the index argument is not within the bounds of the string.
For instance, if you specify a starting index that is greater than the length of the string while using the indexOf() method, you’ll encounter this exception.
Here’s an example:
String str = 'Hello, World!';
try {
int index = str.indexOf('World', 20);
System.out.println(index);
} catch (StringIndexOutOfBoundsException e) {
e.printStackTrace();
}
// Output:
// java.lang.StringIndexOutOfBoundsException: String index out of range: 20
In this example, we tried to find the index of ‘World’ starting from the 20th position. But the length of the string is less than 20, so a ‘StringIndexOutOfBoundsException’ is thrown.
To avoid this issue, always ensure that the starting index is within the bounds of the string. You can use the length() method of the String class to check the length of the string.
Here’s how to do it:
String str = 'Hello, World!';
int startIndex = 20;
if (startIndex < str.length()) {
int index = str.indexOf('World', startIndex);
System.out.println(index);
} else {
System.out.println('Start index is out of bounds');
}
// Output:
// Start index is out of bounds
In this code, we first check if the starting index is less than the length of the string. If it is, we proceed with the indexOf() method. If not, we print a message indicating that the starting index is out of bounds. This way, we can prevent the ‘StringIndexOutOfBoundsException’.
Understanding Java’s String Class and Indexing
Before diving deeper into the indexOf() method, it’s crucial to understand the fundamentals of Java’s String class and the concept of string indexing.
Java’s String Class
In Java, strings are objects that are instances of the String class. The String class offers numerous methods to manipulate strings, indexOf() being one of them. These methods provide functionality for various operations like comparing strings, concatenating strings, converting cases, finding substrings, and more.
String Indexing in Java
String indexing is a way of accessing individual characters in a string. In Java, strings are indexed from 0. That means the first character of a string is at index 0, the second character is at index 1, and so on.
Here’s a visual representation of string indexing in Java:
String str = 'Hello';
// Indexes: 01234
System.out.println(str.charAt(0)); // Output: H
System.out.println(str.charAt(1)); // Output: e
In this example, we have a string ‘Hello’. The character ‘H’ is at index 0, ‘e’ is at index 1, and so on. The method charAt() is used to get the character at a specific index.
Understanding string indexing is vital for using the indexOf() method effectively, as this method returns the index of a character or substring in a string.
With a strong grasp of these fundamentals, you’ll find it easier to understand and use the indexOf() method in Java.
Going Beyond indexOf() in Java
The indexOf() method is a fundamental tool for string manipulation in Java. However, it’s just one of the many methods provided by the String class for handling strings. To truly master string manipulation in Java, it’s worth exploring related concepts and methods.
Exploring String Comparison
Java’s String class provides several methods for comparing strings, such as equals(), compareTo(), and startsWith(). These methods allow you to compare two strings for equality, determine their lexicographical order, and check if a string starts with a specific substring, respectively.
Unraveling String Splitting
The split() method in the String class is a powerful tool for dividing a string into an array of substrings based on a specified delimiter. It’s particularly useful when you need to parse a string into individual words or components.
Further Resources for Mastering String Manipulation in Java
To deepen your understanding of string manipulation in Java, here are some resources you might find useful:
- Understanding Java String Operations – Discover how to create, manipulate, and compare strings in Java.
Java Regex Basics – Learn about the java.util.regex package and its classes for working with regular expressions.
Accessing Characters in Java Strings – Master manipulating strings at the character level using charAt() in Java.
The Official Java String Documentation provides comprehensive details about the String class.
Java String Tutorial by TutorialsPoint covers all the essential topics related to strings in Java.
Java String indexOf() by W3Schools gives an easy-to-understand explanation of the
indexOf()
method in Java’s String class.
Remember, mastering a programming language involves continuous learning and practice. So, don’t stop exploring and experimenting!
Wrapping Up: Mastering indexOf() in Java
In this comprehensive guide, we’ve delved into the depths of the indexOf() method in Java, a key tool for finding the index of a character or substring within a string.
We began with the basics, learning how to use the indexOf() method in its simplest form. We then explored more advanced usage, such as specifying a starting index to find subsequent occurrences of a character or substring.
We also addressed common issues you might encounter when using the indexOf() method, such as the ‘StringIndexOutOfBoundsException’, and provided solutions to help you navigate these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantage | Disadvantage |
---|---|---|
indexOf() | Finds first occurrence | Cannot directly find last occurrence |
lastIndexOf() | Finds last occurrence | Cannot directly find first occurrence |
We also looked at alternative approaches to finding the index of a character or substring, introducing you to the lastIndexOf() method. We compared these two methods, helping you understand when to use each one.
Whether you’re just starting out with Java or you’re looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of the indexOf() method and its capabilities.
With its simplicity and versatility, the indexOf() method is a powerful tool for string manipulation in Java. Happy coding!