NPM Create-React-App | Quick Start Guide
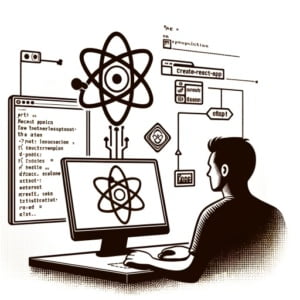
At IOFlood, we often need to kickstart new React projects en masse. However, setting up a new React app from scratch can be time-consuming and error-prone, which is why we created this guide on npm create-react-app
. Learning to use the create-react-app package, can allow you to create a new React app and get started on your projects as quickly as possible.
This guide aims to be your compass in the vast landscape of React development. By leveraging npm create-react-app
, you’re not just starting another project; you’re embracing an efficient and optimized beginning. With this command, the complexities of setting up a new React project are abstracted away, leaving you with a solid foundation to build upon.
Ready to jump into React development? Let’s kickstart your next project effortlessly with npm create-react-app!
TL;DR: How Do I Create a New React App Using NPM?
To start a new React project with zero configuration, use the commands
npx create-react-app my-awesome-app
andnpm start
while in the apps directory.
Here’s a quick example:
npx create-react-app my-awesome-app
cd my-awesome-app
npm start
# Output:
# Starts the development server for 'my-awesome-app', opening a new browser window showing your React app.
In this example, npx
runs the create-react-app
command to generate a new React application named ‘my-awesome-app’. After navigating into your new app’s directory and starting the development server with npm start
, you’ll see your new React app live in your browser. This process encapsulates the beauty of create-react-app
: simplicity and efficiency in launching new projects.
Ready to dive deeper into the world of React with
npm create-react-app
? Keep reading for a comprehensive guide on leveraging this powerful tool for your next project.
Table of Contents
Kickstart Your React App with NPM
The Magic of create-react-app
When you’re ready to dive into React development, npm create-react-app
is the first command that comes to the rescue. It’s designed to set up your project quickly, without the hassle of configuring the development environment manually. Let’s walk through the basic use of this command to understand its simplicity and power.
npm init react-app your-first-react-app
# Output:
# Success! Created your-first-react-app at /your-path/your-first-react-app
# Inside that directory, you can run several commands:
# npm start
# npm run build
# npm test
# npm run eject
# We suggest that you begin by typing:
# cd your-first-react-app
# npm start
In this example, npm init react-app your-first-react-app
is an alternative syntax to npx create-react-app
, specifically tailored for npm 5.2+ users. This command creates a new folder named your-first-react-app
and sets up a React project inside it. The output messages guide you through the next steps: moving into your new project’s directory and starting the development server with npm start
.
Why is this important? The create-react-app
command abstracts away the complexity of configuring a React development environment. It automatically sets up the project structure, installs necessary dependencies, and configures the development server. This means you can focus on writing code rather than setting up the environment. The downside, however, is the limited customization options in its default setup. For beginners, this trade-off is often worth the simplicity and speed it offers for getting started.
Elevate Your React Projects
Custom Templates with Create-React-App
Diving deeper into npm create-react-app
, you’ll discover its ability to initialize projects with custom templates. This feature is a game-changer for developers looking to start with a configuration that’s a step beyond the default.
npx create-react-app my-app --template typescript
# Output:
# Success! Created my-app at /your-path/my-app
# Inside that directory, you can run several commands:
# npm start
# npm run build
# npm test
# npm run eject
#
# We suggest that you begin by typing:
# cd my-app
# npm start
# Initialized a TypeScript React app.
By appending --template typescript
to the command, create-react-app
initializes a new React project pre-configured with TypeScript. This eliminates the need for manual TypeScript setup, streamlining the development process for projects where type safety is a priority.
Integrating TypeScript
The integration of TypeScript with React projects enhances development by providing static type checking, which can catch errors early in the development cycle. The example above showcases how effortlessly one can start a TypeScript-based React project, combining the robustness of TypeScript with the simplicity of create-react-app
.
Custom Webpack Configurations via Ejecting
For projects requiring more customization, create-react-app
offers an ‘eject’ feature. Ejecting allows you to modify the build configuration directly.
npm run eject
# Output:
# Ejected successfully. Now you have full control over your webpack configuration.
Ejecting is a one-way operation that removes the single build dependency from your project. After ejecting, you gain full control over the webpack configuration, enabling you to tweak your project’s build process as needed. While powerful, it’s important to consider this step carefully, as it exposes you to the complexity create-react-app
was designed to abstract away.
Why Go Advanced? Utilizing these advanced features of npm create-react-app
empowers developers to tailor their React projects more precisely to their needs. Whether it’s starting with a TypeScript template for better type safety or customizing the webpack configuration post-ejection, these capabilities ensure your project’s foundation is as strong and flexible as possible.
Beyond Create-React-App
Exploring React Project Alternatives
While npm create-react-app
offers a streamlined approach to setting up React applications, the ecosystem provides several alternative tools and methods each tailored to different needs and preferences. Let’s delve into a few notable alternatives: Next.js, Gatsby, and manual configuration, comparing them with create-react-app
in terms of flexibility, performance, and specific use cases.
Next.js: The SSR Champion
npx create-next-app my-next-app
# Output:
# Success! Created my-next-app at /your-path/my-next-app
# Inside that directory, you can run several commands:
# npm run dev
# npm run build
# npm start
#
# Ready to go!
Next.js is renowned for its server-side rendering capabilities, offering improved SEO and performance for projects where initial load time is critical. The command npx create-next-app
initializes a Next.js project, similar to how create-react-app
works for React. This simplicity, combined with powerful features like automatic code splitting and static site generation, makes Next.js a compelling alternative for more complex applications.
Gatsby: The Static Site Generator
Gatsby leverages React to produce static websites, optimizing for speed and security. It’s particularly well-suited for blogs, portfolios, and corporate websites where content is king but dynamic server-side processing is not required.
npm init gatsby
# Output:
# Welcome to Gatsby!
Gatsby projects start with npm init gatsby
, initiating a setup process that’s both quick and customizable. With its vast plugin ecosystem, Gatsby excels in pulling data from various sources, transforming it, and compiling the most performant static sites possible.
Manual Configuration: Total Control
For developers seeking the utmost flexibility, manually configuring a React project from scratch is the ultimate path. This approach involves setting up webpack, Babel, and other dependencies by hand, providing complete control over the project’s structure and build process.
mkdir my-custom-react-app && cd my-custom-react-app
npm init -y
npm install react react-dom webpack webpack-cli babel-loader @babel/core @babel/preset-react --save-dev
This sequence of commands sets the foundation for a custom React project. While it offers unparalleled flexibility, it requires a deep understanding of the build tools and their configurations, making it more suitable for experienced developers.
Choosing the Right Path: Each alternative to npm create-react-app
caters to different project needs and developer preferences. Whether prioritizing SEO and performance with Next.js, aiming for the speed and security of Gatsby, or seeking the total control of manual configuration, the React ecosystem has options to suit various development scenarios. Understanding these alternatives allows you to make informed decisions, ensuring your project is built on the best possible foundation.
Troubleshooting Create-React-App
Overcoming Common Hurdles
Even with the streamlined process of npm create-react-app
, developers can encounter issues ranging from dependency conflicts to challenges with npm versus npx usage. Understanding these common pitfalls and how to navigate them can significantly enhance your development experience.
Dependency Conflicts
One frequent challenge is dealing with dependency conflicts. This can occur when the versions of packages required by create-react-app
clash with those already in your project or globally installed on your machine.
npm list react
# Output:
# [email protected]
# └── [email protected]
The npm list
command, followed by the package name, helps you identify the installed version of a dependency. Knowing the exact version can guide you in resolving conflicts, either by updating the conflicting packages or adjusting your project’s dependencies to compatible versions.
NPM vs. NPX Usage
Another common source of confusion is the distinction between npm and npx. While both are part of the Node.js package ecosystem, they serve different purposes. npm
is used to install dependencies, whereas npx
is designed to execute Node.js packages.
npx create-react-app my-app
Using npx
as shown above ensures you’re using the latest version of create-react-app
without needing to install it globally. This approach avoids version conflicts and keeps your global package space clean.
Build Process Issues
Encountering problems during the build process is not uncommon. Issues can range from missing environment variables to incorrect file paths.
npm run build
# Output:
# Failed to compile.
# ./path/to/file.js
# Cannot find file './components/MyComponent' in './path/to'.
The error output from npm run build
provides clues about what went wrong. In this example, the issue is a missing file or incorrect path. Carefully reviewing the error messages can lead you to a quick resolution, whether it’s correcting a file path or installing a missing dependency.
Best Practices for a Smooth Development Experience:
- Regularly update your local npm and npx to their latest versions to minimize conflicts.
- Use
npx
to runcreate-react-app
to ensure you’re always using the most current version. - Before running
npm run build
, ensure all dependencies are correctly installed and that there are no path errors in your imports.
By being aware of these common issues and adopting best practices, you can navigate the challenges of using npm create-react-app
more effectively, leading to a smoother and more productive development process.
NPM and Modern Web Development
The Backbone of JavaScript Projects
npm, or Node Package Manager, is a fundamental tool in the world of JavaScript, serving as the largest software registry in existence. It facilitates the management of packages, allowing developers to share and reuse code across projects efficiently.
npm -v
# Output:
# 6.14.8
The command npm -v
quickly tells you the version of npm installed on your system. This is crucial for ensuring compatibility with various packages and tools, including create-react-app
. Keeping npm up-to-date is essential for accessing the latest features and security updates.
React: A Pillar in Web Development
React has emerged as a leading library for building user interfaces, renowned for its efficiency and flexibility. It enables developers to create large web applications that can change data without reloading the page, significantly improving the user experience.
Simplifying React Setup with Create-React-App
create-react-app
plays a pivotal role in modern web development by providing a seamless setup process for React applications. It eliminates the need for manual configuration, which can be both time-consuming and prone to errors.
npx create-react-app --info
# Output:
# Running this command will list the default configuration options and scripts provided by create-react-app.
The npx create-react-app --info
command can be used to display information about the default setup provided by create-react-app
. This includes the configurations and scripts that are preconfigured, highlighting the tool’s effort to simplify the initial setup process for React projects.
The Evolution of Web Development Tools
The landscape of web development tools has evolved dramatically, with a shift towards frameworks and libraries that offer more robust and efficient ways to build applications. create-react-app
stands out by providing a standardized setup that caters to both beginners and experienced developers, streamlining the development process and allowing more focus on building the application itself.
The Significance of Boilerplate Projects
Boilerplate projects, like those generated by create-react-app
, are crucial in accelerating development cycles. They offer a ready-made structure and set of conventions to follow, significantly reducing the time and effort involved in setting up new projects from scratch.
In summary, npm’s role as a package manager, the ascendancy of React in web development, and the simplification provided by create-react-app
are all key components in the modern web development toolkit. These tools and conventions have not only made development more accessible but also more efficient and standardized across the board.
Advancing Your React Development
Adding Routing with React Router
Once you’ve set up your React application using npm create-react-app
, a natural next step is to introduce routing to manage navigation between different components. React Router is the standard for achieving this.
npm install react-router-dom
# Output:
# + [email protected]
# added 1 package in 1.237s
This command installs React Router, enabling you to define routes in your application. By integrating React Router, you can create a single-page application (SPA) with seamless navigation between your app’s components.
State Management with Redux or Context API
For complex applications requiring global state management, Redux or the Context API offer robust solutions. Here’s how you can start with Redux:
npm install redux react-redux
# Output:
# + [email protected]
# + [email protected]
# added 2 packages in 2.645s
The installation of Redux and React-Redux sets the stage for centralized state management, allowing for more predictable state updates across your React application.
Best Practices for Component Structure
Adopting best practices for structuring your components is crucial for maintainability and scalability. This involves organizing components into clear, logical directories and leveraging reusable components.
Further Resources for React Development
To deepen your understanding and mastery of React and its ecosystem, here are three invaluable resources:
- React Official Documentation – A comprehensive guide to everything React, from basic concepts to advanced techniques.
Redux Official Documentation – The official guide to Redux, perfect for understanding state management in React applications.
React Router: Web Guide – A detailed walkthrough on using React Router for adding navigation to your React apps.
These resources offer a wealth of information and are excellent starting points for developers looking to expand their React knowledge and skills. Whether you’re exploring state management options, routing solutions, or best practices for component structure, these guides provide the insights needed to elevate your React projects.
Recap: NPM Create-React-App Guide
In this comprehensive guide, we’ve navigated the essentials of kickstarting your React development journey with npm create-react-app
. This powerful command streamlines the process of setting up a new React application, allowing you to focus on what truly matters – building your project.
We began with the basics, demonstrating how to create a new React app with a simple command. We explored the command’s ability to set up a development environment swiftly, without the need for manual configuration, making it an ideal starting point for beginners.
Moving onto more advanced territory, we delved into custom templates and the integration of TypeScript, showcasing how create-react-app
can be tailored to fit more specific development needs. We also covered the process of ‘ejecting’ for custom webpack configurations, providing you with the knowledge to take full control of your project’s build process when necessary.
For those seeking alternatives, we compared create-react-app
with other tools like Next.js and Gatsby, and discussed manual configuration approaches. This comparison highlighted the flexibility and performance benefits offered by each method, helping you make an informed decision based on your project requirements.
Approach | Flexibility | Performance | Use Case |
---|---|---|---|
Create-React-App | Moderate | High | Beginners/Quick Startups |
Next.js | High | Very High | SEO-Intensive Projects |
Gatsby | High | Very High | Static Sites |
Manual Configuration | Very High | Depends on Setup | Custom Projects |
Whether you’re just starting out with npm create-react-app
or looking to deepen your understanding of React and its ecosystem, we hope this guide has provided you with valuable insights and resources. The simplicity and efficiency of create-react-app
make it an excellent tool for both beginners and experienced developers alike.
With the knowledge you’ve gained, you’re now better equipped to embark on your React development projects, leveraging npm create-react-app
to its fullest potential. Continue exploring the vast landscape of React development and happy coding!