Java Stream .filter() method: A Usage Guide
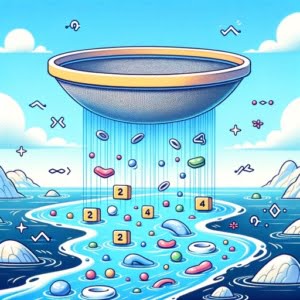
Are you finding it challenging to filter elements in Java Streams? You’re not alone. Many developers find themselves puzzled when it comes to handling filtering in Java Streams, but we’re here to help.
Think of the Stream filter method as your personal sieve – a tool that helps you sort through data with ease. It’s a versatile and handy tool for various tasks, especially when dealing with large data sets.
In this guide, we’ll walk you through the process of using the Java Stream filter method, from basic usage to advanced techniques. We’ll cover everything from the basics of the filter method, how to use it effectively, to more advanced techniques, as well as alternative approaches.
Let’s get started and master the Java Stream filter!
TL;DR: How Do I Use the Filter Method in Java Streams?
The filter method in Java Streams is used to filter elements based on a predicate, with the syntax
List<dataType> output = unfilteredList.stream().filter(unfilteredList -> unfilteredList.filterMethods());
. It’s a powerful tool that allows you to sift through data and extract what you need.
Here’s a simple example:
List<String> names = Arrays.asList('John', 'Susan', 'Kim', 'George');
List<String> result = names.stream().filter(name -> name.startsWith('J')).collect(Collectors.toList());
// Output:
// [John]
In this example, we have a list of names and we want to filter out those that start with ‘J’. We use the filter
method with a lambda expression name -> name.startsWith('J')
as the predicate. This expression returns true for names that start with ‘J’, and these are the ones that get included in the result.
This is just a basic usage of the Java Stream filter method. But there’s so much more you can do with it, including chaining multiple filters and using complex predicates. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Getting Started with Java Stream Filter
- Advanced Techniques with Java Stream Filter
- Exploring Alternative Approaches to Filtering in Java
- Troubleshooting Common Issues with Java Stream Filter
- Understanding Java Streams and Functional Programming
- The Real-World Application of Java Stream Filter
- Further Resources for Mastering Java Stream Filter
- Wrapping Up: Java Stream filter() Method
Getting Started with Java Stream Filter
Java Stream filter is a powerful tool in the hands of a programmer. It provides a simple yet effective way to filter elements from a stream based on a given condition or predicate.
How Does the Filter Method Work?
The filter method works by applying a given predicate to each element in the stream. If the predicate returns true, the element is included in the resulting stream. If it returns false, the element is discarded.
Here’s a basic example:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream().filter(n -> n % 2 == 0).collect(Collectors.toList());
// Output:
// [2, 4, 6, 8, 10]
In this example, we have a list of numbers from 1 to 10. We want to filter out the even numbers. To do this, we use the filter
method with a lambda expression n -> n % 2 == 0
as the predicate. This expression returns true for even numbers, and these are the ones that get included in the result.
Advantages and Potential Pitfalls
One of the main advantages of using the filter method is its simplicity and readability. It allows you to express complex filtering logic in a concise and readable manner.
However, there are a few potential pitfalls to be aware of. One is performance. The filter method processes each element in the stream individually, which can be slow for large streams. Another potential pitfall is null values. If your stream contains null values, you need to handle them carefully to avoid NullPointerExceptions.
Advanced Techniques with Java Stream Filter
As you become more comfortable with the filter method in Java Streams, you can start to explore more complex uses. This includes chaining multiple filters and using complex predicates.
Chaining Multiple Filters
One of the powerful features of Java Streams is the ability to chain multiple operations. This means you can apply more than one filter to a stream. Here’s an example:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> result = numbers.stream()
.filter(n -> n % 2 == 0)
.filter(n -> n > 5)
.collect(Collectors.toList());
// Output:
// [6, 8, 10]
In this example, we first filter out the even numbers, and then we filter out the numbers that are greater than 5. The result is a list of even numbers greater than 5.
Using Complex Predicates
You can also use complex predicates in the filter method. A predicate is a function that takes an input and returns a boolean value. Here’s an example of using a complex predicate:
List<String> names = Arrays.asList('John', 'Susan', 'Kim', 'George');
List<String> result = names.stream()
.filter(name -> name.startsWith('J') && name.length() > 3)
.collect(Collectors.toList());
// Output:
// [John]
In this example, we’re filtering names that start with ‘J’ and have more than 3 characters. The result is a list containing just ‘John’.
Best Practices
When using the filter method, it’s important to remember a few best practices. First, try to keep your predicates simple and readable. If your predicate is too complex, it can be difficult to understand what it’s doing. Second, be aware of the performance implications. Filtering a large stream can be slow, so consider other options if performance is a concern. Finally, handle null values carefully to avoid NullPointerExceptions.
Exploring Alternative Approaches to Filtering in Java
While the Stream filter method provides a straightforward and functional approach to filtering data, it’s not the only tool available in Java. Let’s explore some alternative methods for filtering data, such as using loops or third-party libraries.
Using Loops for Filtering
Before the introduction of Streams in Java 8, loops were commonly used for filtering data. Here’s an example of how you can filter a list of numbers using a for loop:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = new ArrayList<>();
for (Integer number : numbers) {
if (number % 2 == 0) {
evenNumbers.add(number);
}
}
// Output:
// evenNumbers: [2, 4, 6, 8, 10]
In this example, we’re using a for-each loop to iterate over the list of numbers. If a number is even, we add it to the evenNumbers
list. This approach gives you more control over the filtering process, but it’s more verbose and less expressive than using a Stream filter.
Leveraging Third-Party Libraries
There are also third-party libraries like Apache Commons Collections and Google’s Guava that provide additional utilities for filtering data. For example, Guava’s Collections2.filter
method allows you to filter a collection based on a Predicate
:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
Collection<Integer> evenNumbers = Collections2.filter(numbers, new Predicate<Integer>() {
public boolean apply(Integer number) {
return number % 2 == 0;
}
});
// Output:
// evenNumbers: [2, 4, 6, 8, 10]
These libraries can provide more flexibility and functionality than Java’s built-in tools, but they also add external dependencies to your project, which may not always be desirable.
Making the Right Choice
When deciding which approach to use for filtering data in Java, consider the size and complexity of your data, the performance implications, and the readability and maintainability of your code. While the Stream filter method is a powerful and expressive tool, alternative approaches may be more suitable in certain situations.
Troubleshooting Common Issues with Java Stream Filter
While the Stream filter method in Java is a powerful tool, like any other feature, you might encounter a few issues while using it. Let’s discuss some common problems and how to solve them.
Handling Performance Issues
When dealing with large streams, the filter method can slow down your application. This is because it processes each element individually. If performance is a concern, consider using parallel streams or other data structures like sets or maps that have faster lookup times.
Dealing with Null Values
If your stream contains null values, using the filter method without a null check can lead to a NullPointerException. To prevent this, you can add a null check in your predicate:
List<String> names = Arrays.asList('John', null, 'Kim', 'George');
List<String> result = names.stream()
.filter(name -> name != null && name.startsWith('J'))
.collect(Collectors.toList());
// Output:
// [John]
In this example, we add name != null
to our predicate to ensure that we don’t call startsWith
on a null value.
Tips for Using the Filter Method Effectively
Here are a few tips to keep in mind when using the filter method:
- Keep your predicates simple and readable. If a predicate is too complex, consider breaking it down into smaller, more manageable parts.
- Be aware of the performance implications. If you’re dealing with large streams, consider using parallel streams or other data structures with faster lookup times.
- Handle null values carefully to avoid NullPointerExceptions.
Understanding Java Streams and Functional Programming
To fully appreciate the power of the filter
method in Java Streams, it’s essential to grasp the fundamentals of Java Streams and the concept of functional programming.
Java Streams: A Brief Overview
Introduced in Java 8, Streams represent a sequence of elements and support various operations to perform computations on those elements. These operations can be either intermediate (transforming a Stream into another Stream, such as filter
and map
) or terminal (producing a result or a side-effect, like collect
and forEach
).
Here’s a simple example of a Stream operation:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squares = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
// Output:
// squares: [1, 4, 9, 16, 25]
In this example, we’re transforming a list of numbers into a list of their squares using a Stream.
Embracing Functional Programming in Java
Java 8 introduced functional programming features into its object-oriented environment, which includes lambda expressions and functional interfaces. This paradigm encourages writing programs that have less mutable data and use functions or methods as first-class entities.
The filter
method in Java Streams is a prime example of functional programming. It takes a predicate (a function that returns a boolean) as an argument and applies this predicate to each element in the Stream.
Understanding these fundamentals will help you better comprehend the workings of the filter
method and how it can be effectively used in various scenarios.
The Real-World Application of Java Stream Filter
Java Stream filter is not just a theoretical concept; it has practical applications in real-world scenarios. Let’s explore a few of these applications and how the filter
method can be a game-changer.
Java Stream Filter in Data Processing
Data processing is one area where Java Stream filter shines. Whether you’re dealing with large datasets or small, the filter
method can help you sift through and extract the data you need. It’s a much more efficient and readable alternative to traditional loops.
Leveraging Java Stream Filter for File I/O
File I/O operations often involve reading data, processing it, and writing the results back to a file. The filter
method can be used to process the data read from the file, such as filtering out unwanted lines or extracting specific information.
Other Stream Operations and Parallel Streams
The filter
method is just one of many operations available in Java Streams. Other operations like map
, reduce
, and collect
can be combined with filter
to perform complex data processing tasks. Moreover, Java Streams support parallel processing, which can significantly improve performance for large datasets.
Further Resources for Mastering Java Stream Filter
To deepen your understanding of Java Stream filter and its applications, check out the following resources:
- Java Stream Tutorial: Getting Started – Discover the power of Java streams for parallel and sequential data processing.
Java Selection Sort Algorithm – Learn Java selection sort techniques for sorting small to medium
Java Binary Search Algorithm – Explore Java binary search techniques for finding elements quickly in large datasets.
Oracle’s Java Tutorials: Streams – These official tutorials from Oracle provide a comprehensive introduction to Java Streams, including the
filter
method.Baeldung’s Guide to Java 8’s Streams offers a deep dive into Java 8’s Streams, with numerous examples and explanations.
Java Stream Filter Examples by GeeksforGeeks gives a detailed explanation about Stream Filter in Java.
Wrapping Up: Java Stream filter() Method
In this comprehensive guide, we’ve delved into the ins and outs of the Java Stream filter method. From basic usage to advanced techniques, we’ve explored how this powerful tool can be used to sift through and process data in Java.
We began with the basics, understanding how the filter method works with Java Streams and how to use it effectively. We then advanced to more complex uses of the filter method, such as chaining multiple filters and using complex predicates. We also tackled common issues you might encounter when using the filter method, such as performance issues and handling null values, providing you with solutions and tips to overcome these challenges.
We also ventured beyond the filter method, exploring alternative approaches for filtering data in Java, such as using loops or third-party libraries. These alternatives, while not always as expressive or convenient as the filter method, can provide more control or flexibility depending on your specific needs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Performance | Complexity |
---|---|---|---|
Java Stream filter | High | Moderate | Low |
Loops | Moderate | High | High |
Third-Party Libraries | High | Varies | Moderate |
Whether you’re a beginner just starting out with Java Streams or an experienced developer looking to deepen your understanding of the filter method, we hope this guide has been a valuable resource.
The power of the Java Stream filter method lies in its ability to process large data sets in a readable and expressive way. With this guide, you’re now well-equipped to leverage this power in your Java programming. Happy coding!