jsonwebtoken | npm Package Install Guide for Node.js
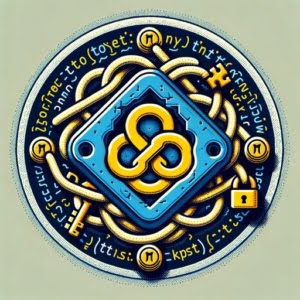
When it comes to secure data transmission and authentication in Node.js apps, utilizing JSON Web Tokens (JWT) has become a common practice. JWTs provide a compact way to securely transmit information between parties. As developers and server hosts, protecting the data we manage is paramount, and JWTs are a critical tool in achieving this goal.
While developing software at IOFLOOD, we have found that the ‘jsonwebtoken’ package can aid with JWT implementation in Node.js. To aid developers and customers utilizing our dedicated servers, we have put together this tutorial to enhance the integrity of your applications.
This guide dives into the ‘jsonwebtoken’ npm package, an essential tool for handling JWTs in Node.js applications. Whether you’re a beginner looking to understand the basics of JWT or an experienced developer seeking to implement more sophisticated security solutions, this guide will provide the insights and examples you need to utilize JWTs in your Node.js projects.
Let’s dive into JSON Web Tokens and discover how the ‘jsonwebtoken’ package can ensure reliable data transmission and user authentication!
TL;DR: How Do I Use the ‘jsonwebtoken’ Package for JWT in Node.js?
To use JWTs in Node.js, first install the ‘jsonwebtoken’ package with the command,
npm install jsonwebtoken
. Then configure the package, making sure to import it at the top of your application file with,const jwt = require('jsonwebtoken');
.
Here’s a quick example to generate a token:
const jwt = require('jsonwebtoken');
const token = jwt.sign({ user: 'John Doe' }, 'secretKey');
console.log(token);
# Output:
# eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyIjoiSm9obiBEb2UiLCJpYXQiOjE1MTYyMzkwMjJ9.s5P0_G8kU3CwWx-HZ6pmesfn9nU-6uh0p6p3LqgH0KU
In this quick example, we use the ‘jsonwebtoken’ package to sign a new token with a payload containing a user object. The jwt.sign
method takes two arguments: the payload and a secret key. The result is a compact, URL-safe string representing the claims to be transferred. This token can then be used for secure communication between the client and the server.
This is a basic way to use the ‘jsonwebtoken’ package in Node.js, but there’s much more to learn about creating and managing JWTs effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started: jsonwebtoken npm
Embarking on the journey of securing your Node.js application with JSON Web Tokens (JWT) begins with understanding the basics. The ‘jsonwebtoken’ npm package is a powerful tool that simplifies the process of creating and verifying JWTs. Let’s break down the steps to get you started.
Installing the jsonwebtoken Package
First things first, you need to install the ‘jsonwebtoken’ package. Open your terminal and run the following command in your project directory:
npm install jsonwebtoken
This command fetches the ‘jsonwebtoken’ package from npm and adds it to your project dependencies, ensuring you have the latest version ready to secure your application.
Generating Your First JWT
Once installed, generating a JWT is straightforward. Here’s an example of how to create a token using the ‘jsonwebtoken’ package:
const jwt = require('jsonwebtoken');
const payload = { username: 'exampleUser' };
const secret = 'yourSecretKey';
const token = jwt.sign(payload, secret, { expiresIn: '1h' });
console.log(token);
# Output:
# eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6ImV4YW1wbGVVc2VyIiwiaWF0IjoxNTE2MjM5MDIyLCJleHAiOjE1MTYyNDI2MjJ9.3Dw7prvuBQ-3VjPLkvr7l6nHcEi8Yp2lPAkXpZ5lHkU
In this code snippet, we create a simple payload with a username, specify a secret key, and set the token to expire in one hour. The jwt.sign
method then generates a JWT, which is output to the console. This token can be used in subsequent requests to authenticate the user.
Verifying Tokens
Verifying the authenticity of a JWT is crucial for secure application functionality. Here’s how you can verify a token using the ‘jsonwebtoken’ package:
const jwt = require('jsonwebtoken');
const token = 'yourJWT'; // The token you received
const secret = 'yourSecretKey';
try {
const decoded = jwt.verify(token, secret);
console.log('Token is valid:', decoded);
} catch (error) {
console.error('Token verification failed:', error.message);
}
# Output:
# Token is valid: { username: 'exampleUser', iat: 1516239022, exp: 1516242622 }
This example demonstrates how to verify a JWT by decoding it with the secret key used to sign the token. If the token is valid, the decoded payload is displayed; otherwise, an error is thrown, indicating the verification failed. This step is essential in ensuring that the tokens used in your application are authentic and have not been tampered with.
Advanced jsonwebtoken Techniques
As you become more comfortable with the ‘jsonwebtoken’ npm package, it’s time to explore its more advanced capabilities. These features allow for finer control over your JWTs, enhancing the security and flexibility of your Node.js applications.
Setting Token Expiration
One of the key aspects of JWT security is managing the token’s lifecycle. Here’s how you can set an expiration time for your tokens:
const jwt = require('jsonwebtoken');
const payload = { username: 'advancedUser' };
const secret = 'yourAdvancedSecretKey';
const token = jwt.sign(payload, secret, { expiresIn: '2h' });
console.log(token);
# Output:
# eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6ImFkdmFuY2VkVXNlciIsImlhdCI6MTUxNjIzOTAyMiwiZXhwIjoxNTE2MjQ2MjIyfQ.2kL3Vj4lrRk8IzmUc4bF5V_myasfasdfasdfa
In this example, the expiresIn
option is used to specify that the token should expire in two hours. This is a crucial security feature, ensuring that tokens are not valid indefinitely and reducing the risk of token misuse.
Handling Refresh Tokens
For applications requiring long-term authentication, refresh tokens are a vital feature. They allow users to obtain a new access token without re-authenticating, based on a longer-lived refresh token. Here’s a basic example of issuing a refresh token alongside an access token:
const jwt = require('jsonwebtoken');
const accessTokenPayload = { username: 'user' };
const refreshTokenPayload = { username: 'user', tokenType: 'refresh' };
const secret = 'yourSecretKey';
const refreshSecret = 'yourRefreshSecretKey';
const accessToken = jwt.sign(accessTokenPayload, secret, { expiresIn: '15m' });
const refreshToken = jwt.sign(refreshTokenPayload, refreshSecret, { expiresIn: '7d' });
console.log('Access Token:', accessToken);
console.log('Refresh Token:', refreshToken);
# Output:
# Access Token: eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6InVzZXIiLCJpYXQiOjE1MTYyMzkwMjIsImV4cCI6MTUxNjI0MDUyMn0.s3Dw7prvuBQ-3VjPLkvr7l6nHcEi8Yp2lPAkXpZ5lHkU
# Refresh Token: eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6InVzZXIiLCJ0b2tlblR5cGUiOiJyZWZyZXNoIiwiaWF0IjoxNTE2MjM5MDIyLCJleHAiOjE1MTY4NDM4MjJ9.Qs5P0_G8kU3CwWx-HZ6pmesfn9nU-6uh0p6p3LqgH0KU
This approach ensures that users can maintain their session without frequent re-authentication, improving the user experience while maintaining security.
Middleware for Protected Routes
Implementing middleware in Express.js applications to protect routes is another advanced feature of the ‘jsonwebtoken’ package. This middleware checks for a valid JWT before allowing access to certain endpoints. Here’s a simple example of such a middleware function:
const jwt = require('jsonwebtoken');
const express = require('express');
const app = express();
const secret = 'yourSecretKey';
app.use((req, res, next) => {
const token = req.headers['authorization'];
if (!token) return res.status(403).send('A token is required for authentication');
try {
const decoded = jwt.verify(token, secret);
req.user = decoded;
} catch (error) {
return res.status(401).send('Invalid Token');
}
return next();
});
app.get('/protected', (req, res) => {
res.send('This route is protected');
});
app.listen(3000, () => console.log('Server running on port 3000'));
In this code, the middleware function checks for a token in the ‘authorization’ header of incoming requests. If the token is not present or is invalid, the request is denied, effectively protecting the route. This demonstrates the ‘jsonwebtoken’ package’s capability to integrate seamlessly with Express.js for secure route handling.
Exploring JWT Alternatives in Node.js
While the ‘jsonwebtoken’ npm package is a popular choice for handling JSON Web Tokens in Node.js applications, it’s not the only player in the game. Understanding alternative libraries and approaches can empower you to make informed decisions, especially when working on more complex applications that may require different performance, flexibility, or ease of use characteristics.
node-jsonwebtoken vs. jws
One notable alternative is the jws
library, which focuses solely on the signing and verification of JSON Web Signatures (JWS). Unlike ‘jsonwebtoken’, which provides a higher-level API for dealing with JWTs, jws
offers more granular control over the JWS creation and verification process.
const jws = require('jws');
const signature = jws.sign({
header: { alg: 'HS256' },
payload: 'hello world',
secret: 'shhhhh',
});
console.log(signature);
# Output:
# eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.aGVsbG8gd29ybGQ.4yqP4qB4fJaFUxv6PbQMIgC6T0g4DVp5pshEW5SJLzM
In this code block, we use jws
to sign a simple payload of ‘hello world’ with the HS256 algorithm. The output is a compact, URL-safe string that represents the JWS. This example demonstrates jws
‘s straightforward approach to generating signatures without the additional JWT-specific functionality found in ‘jsonwebtoken’.
Performance and Flexibility
When comparing ‘jsonwebtoken’ with alternatives like jws
, it’s important to consider your application’s specific needs. ‘jsonwebtoken’ is highly regarded for its ease of use and comprehensive feature set tailored to JWT handling. On the other hand, jws
and similar libraries may offer better performance and flexibility in scenarios where lower-level operations are preferred or when working with non-JWT tokens.
Making the Right Choice
Choosing between ‘jsonwebtoken’ and its alternatives boils down to your application’s requirements and your comfort level with the library’s API. If you need a straightforward, high-level API for JWTs with good community support, ‘jsonwebtoken’ is a great choice. However, for applications that require custom token handling or prioritize performance, exploring alternatives like jws
could provide the flexibility needed to achieve your goals.
In conclusion, while ‘jsonwebtoken’ npm remains a solid choice for many Node.js developers, being aware of and understanding alternative libraries enriches your toolkit. This knowledge allows you to tailor your security implementation to the unique demands of your application, ensuring the best possible outcome.
Optimizing JWT for Node.js
When integrating JSON Web Tokens (JWT) into your Node.js applications using the ‘jsonwebtoken’ npm package, it’s crucial to be aware of common pitfalls and best practices. This ensures not only the security of your implementation but also its performance. Let’s dive into some of these considerations and how to address them effectively.
Handling Token Expiration Gracefully
Token expiration is a fundamental aspect of JWT security, reducing the window of opportunity for token misuse. However, managing token expiration requires careful planning to avoid disrupting the user experience. Here’s an example of how you can handle expired tokens on the server side:
const jwt = require('jsonwebtoken');
app.use((req, res, next) => {
const token = req.headers['authorization'];
if (!token) return res.status(403).send('Token required');
jwt.verify(token, 'yourSecretKey', (err, decoded) => {
if (err) {
if (err.name === 'TokenExpiredError') {
return res.status(401).send('Token expired');
}
return res.status(401).send('Invalid token');
}
req.user = decoded;
next();
});
});
# Output:
# 'Token expired' or 'Invalid token'
This code snippet demonstrates a middleware function that verifies the JWT and checks for expiration. If the token is expired, it sends a ‘Token expired’ response. This approach allows you to prompt users to refresh their token, maintaining security while minimizing disruption.
Securing Secret Keys
The security of your JWT implementation heavily relies on the secrecy of the signing key. Exposing your secret keys can lead to token forgery and significant security vulnerabilities. Therefore, it’s crucial to store your secret keys securely. Environment variables, encrypted using tools like dotenv
and cryptex
, offer a safe way to handle secrets in your application.
Addressing Security Vulnerabilities
Staying updated on security vulnerabilities within the ‘jsonwebtoken’ package and its dependencies is essential. Regularly updating the package to the latest version can mitigate risks associated with known vulnerabilities. Moreover, implementing additional security measures, such as HTTPS and using secure cookies for token storage in web applications, further enhances your JWT security posture.
Best Practices for Performance
Performance optimization for JWT handling involves minimizing the payload size and efficiently managing the token lifecycle. Smaller payloads result in faster transmission times and reduced processing overhead. Efficient token lifecycle management, including timely revocation of tokens and minimizing database lookups for token verification, ensures a responsive and scalable application.
In conclusion, while JWTs offer a robust method for securing your Node.js applications, understanding and addressing these considerations is key to maximizing both the security and performance of your JWT implementation. By adopting these best practices, you can build a secure, efficient, and user-friendly authentication system.
JWTs: The Security Backbone
Before diving into the practical use of the ‘jsonwebtoken’ npm package, it’s essential to grasp the fundamentals of JSON Web Tokens (JWTs) and their pivotal role in modern web applications. JWTs are a compact, URL-safe means of representing claims to be transferred between two parties. The information can be verified and trusted because it is digitally signed.
JWTs consist of three parts: the Header, the Payload, and the Signature. Let’s break down each part:
The Header
The Header typically consists of two parts: the type of the token, which is JWT, and the signing algorithm being used, such as HMAC SHA256 or RSA.
{
"alg": "HS256",
"typ": "JWT"
}
The Payload
The Payload contains the claims. These claims are statements about an entity (typically, the user) and additional data. There are three types of claims: registered, public, and private claims.
{
"sub": "1234567890",
"name": "John Doe",
"admin": true
}
The Signature
The Signature is used to secure the token and verify that the sender of the JWT is who it says it is and to ensure that the message wasn’t changed along the way.
const encodedHeader = 'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9';
const encodedPayload = 'eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiYWRtaW4iOnRydWV9';
const signature = 'HMACSHA256(encodedHeader + '.' + encodedPayload, 'yourSecretKey');
Why JWTs Are Secure
JWTs are secure because of their structure. The signature ensures that the token hasn’t been altered after it was issued. It also confirms the identity of the sender. The process of creating the signature involves a secret key that only the issuer knows, making it difficult for attackers to forge tokens.
Their Role in Web Applications
JWTs are widely used in web applications for authentication and information exchange. After a user logs in, the server creates a JWT with user information and sends it back to the client. The client can then use this token to make requests to the server without having to authenticate again. This is particularly useful in Single Page Applications (SPAs) and for creating stateless session management in APIs.
In conclusion, understanding the structure and security mechanisms of JWTs provides a solid foundation for effectively implementing them in your Node.js applications using the ‘jsonwebtoken’ npm package. Their ability to securely transmit information between parties makes them an indispensable tool in the developer’s toolkit.
JWTs in Complex Architectures
As your Node.js applications grow in complexity and scale, the role of JSON Web Tokens (JWTs) expands. JWTs become not just a method for authentication but a cornerstone for secure communication in distributed systems, such as microservices architectures, and for integrating with various frontend frameworks.
JWTs and Microservices
In a microservices architecture, services need to communicate securely and efficiently. JWTs facilitate this by allowing each service to verify the authenticity of requests independently. Here’s an example of how a service might verify a JWT:
const jwt = require('jsonwebtoken');
const token = 'yourJWT';
const secret = 'yourSecretKey';
try {
const decoded = jwt.verify(token, secret);
console.log('Service access granted for:', decoded.user);
} catch (error) {
console.error('Access denied:', error.message);
}
# Output:
# Service access granted for: John Doe
This code block demonstrates a service extracting and verifying a JWT. Upon successful verification, the service grants access to the requested resource. This independent verification is crucial in a microservices architecture, ensuring that each service can protect itself from unauthorized access.
JWTs and Frontend Integration
Integrating JWTs with frontend frameworks like React or Angular enhances application security. By storing the JWT in the browser’s local storage or in a cookie, the frontend application can send the token with each request, maintaining the user’s session. Here’s a conceptual example of sending a JWT with an HTTP request from a frontend application:
fetch('api/protected', {
method: 'GET',
headers: {
'Authorization': 'Bearer yourJWT'
}
})
.then(response => response.json())
.then(data => console.log(data));
This example illustrates how a frontend application might include a JWT in the Authorization header of an HTTP request. This token is then verified by the backend to ensure that the request is authenticated.
Further Resources for Mastering JWTs
To deepen your understanding of JWTs and their application in Node.js, consider exploring the following resources:
- JWT.io – A comprehensive resource for learning about JWTs, including a debugger to inspect tokens.
Auth0 Blog – Offers in-depth articles on JWTs and authentication best practices.
Node.js Security Best Practices – Provides insights into securing Node.js applications, including the use of JWTs.
These resources offer valuable information for developers looking to enhance their security knowledge and effectively implement JWTs in their Node.js applications.
Recap: jsonwebtoken npm Integration
In this comprehensive guide, we’ve navigated the intricacies of securing Node.js applications using JSON Web Tokens (JWTs) with the ‘jsonwebtoken’ npm package. JWTs serve as a robust method for securely transmitting information between parties, ensuring that data can be trusted and verified.
We began with the basics, introducing how to install the ‘jsonwebtoken’ package and generate your first JWT. We then explored verifying tokens, a critical step in authenticating requests and maintaining secure communication between the client and server.
Moving to more advanced topics, we delved into setting token expiration, handling refresh tokens, and creating middleware for protected routes. These practices are essential for enhancing the security and efficiency of your Node.js applications.
We also examined alternative libraries and approaches for handling JWTs, providing you with a broader perspective on the tools available for implementing JWTs in your projects. This exploration helps in making informed decisions when selecting the right tool for your application’s needs.
Feature | ‘jsonwebtoken’ npm | Alternatives |
---|---|---|
Ease of Use | High | Varies |
Flexibility | Moderate | High |
Community Support | Strong | Varies |
As we wrap up, it’s clear that understanding the fundamentals of JWTs and how to implement them using the ‘jsonwebtoken’ npm package is crucial for securing your Node.js applications. Whether you’re handling basic authentication or building complex, distributed systems, JWTs offer a flexible and powerful solution.
With the knowledge and examples provided in this guide, you’re now equipped to implement JWTs in your Node.js projects confidently. Remember, the key to effective security is ongoing learning and adaptation to new challenges. Happy coding!