Selection Sort Java: Guide to Sorting Array Elements
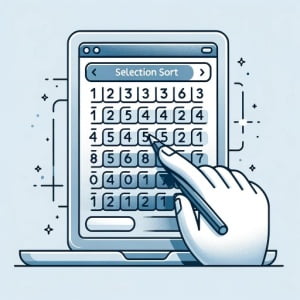
Ever found yourself grappling with implementing selection sort in Java? You’re not alone. Many developers find the selection sort algorithm a bit tricky to implement. Think of selection sort as a meticulous organizer, efficiently sorting your data in a systematic manner.
This guide will walk you through the process of implementing selection sort in Java, from the basics to more advanced techniques. We’ll cover everything from understanding the algorithm, writing the code, to optimizing it for better performance.
So, let’s dive in and start mastering selection sort in Java!
TL;DR: How Do I Implement Selection Sort in Java?
To implement
selection sort
in Java, you create a method that iterates over an array,public void selectionSort(int[] arr){for (int i = 0; i < arr.length - 1; i++)}
, identifies the smallest element,int minIndex = i;
, and swaps it with the first unsorted element,if (arr[j] < arr[minIndex]) {minIndex = j;}
. Here’s the full example:
public void selectionSort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
}
In this example, we define a method selectionSort
that sorts an array arr
. The outer loop iterates over each element in the array, while the inner loop finds the smallest element in the unsorted part of the array. Once the smallest element is found, it is swapped with the first unsorted element. This process continues until the entire array is sorted.
This is a basic way to implement selection sort in Java, but there’s much more to learn about optimizing this algorithm and understanding its time and space complexities. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding Selection Sort in Java
- Time and Space Complexity of Selection Sort
- Optimizing Selection Sort in Java
- Best Practices for Implementing Selection Sort
- Exploring Other Sorting Algorithms in Java
- Common Errors and Solutions in Selection Sort
- Unpacking Sorting Algorithms and their Significance
- Applying Selection Sort in Larger Projects
- Expanding Your Knowledge: Data Structures, Recursion, and Dynamic Programming
- Wrapping Up: Selection Sort in Java
Understanding Selection Sort in Java
Selection Sort is a simple sorting algorithm with a straightforward implementation. The basic idea behind the selection sort algorithm is to divide the input list into two parts: the sorted part and the unsorted part. Initially, the sorted part is empty and the unsorted part is the entire input list. The algorithm repeatedly selects the smallest (or largest, depending on the sorting order) element from the unsorted part and moves it to the end of the sorted part. This operation is performed repeatedly until the unsorted part becomes empty and the sorted part contains all elements in the desired sorted order.
Let’s look at a simple implementation of the selection sort algorithm in Java:
public void selectionSortDescending(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
int maxIndex = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] > arr[maxIndex]) {
maxIndex = j;
}
}
int temp = arr[maxIndex];
arr[maxIndex] = arr[i];
arr[i] = temp;
}
}
In this code snippet, we start by iterating over the array using the outer for
loop. For each iteration, we assume the first unsorted element (the i
th element) is the largest. We then use another for
loop to iterate over the remaining unsorted elements. If we find an element larger than the current largest, we update our maxIndex
.
Once we’ve checked all unsorted elements, we swap the largest found with the first unsorted element. This places the next largest element in its correct sorted position, and we repeat this process for all elements in the array.
Time and Space Complexity of Selection Sort
In terms of time complexity, the selection sort algorithm performs poorly on large lists. Regardless of the initial state of the data, it has a time complexity of O(n^2) because of the nested loops. This makes it inefficient to use for large data sets.
On the other hand, the selection sort algorithm does not require extra space to sort the list. The space complexity is O(1), which means it’s a good choice when memory is a concern. However, the trade-off between time complexity and space complexity is an important consideration when choosing a sorting algorithm.
Optimizing Selection Sort in Java
While the basic implementation of the selection sort algorithm is straightforward, there are ways to optimize it for better performance. One such technique involves reducing the number of swaps in the algorithm.
In the basic implementation, a swap operation is performed in every iteration of the outer loop. However, we can optimize this by performing the swap operation only once per iteration of the outer loop. We do this by finding the minimum element in the unsorted part of the array and swapping it with the first element of the unsorted part only once per iteration.
Here’s how you can implement this optimization in Java:
public void optimizedSelectionSort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
}
}
In this optimized version, we add a condition to check if the minimum element was found in the unsorted part of the array. If it was, we perform the swap operation; otherwise, we move to the next iteration. This reduces the number of swap operations from n to the number of inversions in the array, which can significantly improve performance for nearly sorted arrays.
Best Practices for Implementing Selection Sort
When implementing selection sort in Java, keep the following best practices in mind:
- Understand the trade-offs: While selection sort has a simple implementation and low space complexity, its time complexity is high for large data sets. Use it when memory is a concern and the data set is not too large.
Optimize when possible: As shown above, you can optimize the algorithm to reduce the number of swap operations. This can improve performance, especially for nearly sorted arrays.
Test your implementation: Always test your sorting algorithm with different types of data sets to ensure it works correctly. This includes sorted, reversed, and random data sets of different sizes.
Exploring Other Sorting Algorithms in Java
While selection sort has its advantages, it’s important to be aware of other sorting algorithms. This allows you to choose the best algorithm for your specific use case. Let’s explore three other popular sorting algorithms in Java: quick sort, merge sort, and bubble sort.
Quick Sort
Quick sort is a divide-and-conquer algorithm that works by selecting a ‘pivot’ element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted.
Here’s a simple implementation of quick sort in Java:
public void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi-1);
quickSort(arr, pi+1, high);
}
}
Quick sort has a worst-case time complexity of O(n^2), but in the average case, it’s much faster with a time complexity of O(n log n). Its space complexity is O(log n) due to the recursive stack.
Merge Sort
Merge sort is another divide-and-conquer algorithm. It works by dividing the unsorted list into n sublists, each containing one element, and then repeatedly merging sublists to produce new sorted sublists until there is only one sublist remaining.
Here’s a basic implementation of merge sort in Java:
public void mergeSort(int[] arr, int left, int right) {
if (left < right) {
int mid = (left + right) / 2;
mergeSort(arr, left, mid);
mergeSort(arr , mid + 1, right);
merge(arr, left, mid, right);
}
}
Merge sort performs well even on large data sets, with a time complexity of O(n log n). However, it requires additional space, leading to a space complexity of O(n).
Bubble Sort
Bubble sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted.
Here’s how you can implement bubble sort in Java:
public void bubbleSort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
Bubble sort has a worst-case and average time complexity of O(n^2), making it inefficient on large lists. Its main advantage is its simplicity and the fact that it performs well on nearly sorted lists.
When compared to selection sort, these sorting algorithms present different trade-offs in terms of time and space complexity, as well as their performance on different types of data sets. It’s crucial to understand these differences to choose the best sorting algorithm for your specific use case.
Common Errors and Solutions in Selection Sort
While implementing selection sort in Java, developers often encounter some common issues. Let’s discuss these problems and how to resolve them.
Incorrect Swap
One common mistake is incorrectly swapping elements. Remember, the goal is to swap the smallest unsorted element with the first unsorted element. If you swap incorrectly, your array won’t be sorted correctly. Here’s an incorrect swap operation:
public void incorrectSwap(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
int temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
In this example, the temp
variable should store arr[minIndex]
, not arr[i]
. The correct swap operation is as follows:
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
Array Index Out of Bounds
Another common error is going out of the array bounds. This can happen if you’re not careful with the indices in your loops. Always ensure that your loop variables are within the array bounds.
Testing and Debugging
While implementing selection sort, it’s crucial to test your code with different types of data sets. This includes sorted, reversed, and random data sets of different sizes. If your code doesn’t sort these data sets correctly, it’s likely there’s an issue with your implementation.
Use debugging techniques, such as printing out the array at each step, to understand what your code is doing. This can help you identify where things are going wrong.
Remember, coding is an iterative process. Don’t be discouraged if your first implementation isn’t perfect. With practice and troubleshooting, you’ll be able to implement selection sort successfully.
Unpacking Sorting Algorithms and their Significance
Sorting algorithms are fundamental to computer science. They arrange elements in a particular order, typically numerical or lexicographical. Sorting can be in ascending or descending order, and the efficiency of a sorting algorithm is determined by its ability to arrange records in minimum time and using minimum space.
Java offers a host of sorting algorithms, and selection sort is one of them. Understanding how these algorithms work and their time and space complexities can help you choose the right one for your specific use case.
Time Complexity: The Speed of Sorting
Time complexity is a measure of the amount of time an algorithm takes to run. It’s usually expressed in big O notation, which describes the worst-case scenario of an algorithm in terms of time. For instance, the time complexity of the basic selection sort algorithm is O(n^2), meaning the time it takes to run increases quadratically with the number of elements in the input list.
However, time complexity doesn’t tell the whole story. Some algorithms with a worse time complexity might perform better for small data sets or certain types of data. Therefore, understanding time complexity can help you make informed decisions about which sorting algorithm to use.
Space Complexity: The Cost of Sorting
Space complexity is a measure of the amount of memory an algorithm uses to execute. Just like time complexity, it’s usually expressed in big O notation. The space complexity of the basic selection sort algorithm is O(1), meaning it uses a constant amount of memory regardless of the input size.
While selection sort is efficient in terms of space, it’s not as efficient in terms of time. Therefore, it’s important to consider both time and space complexity when choosing a sorting algorithm. Depending on your use case, you might prioritize one over the other.
In conclusion, understanding sorting algorithms and their time and space complexities is crucial for efficient programming. By understanding these concepts, you can choose the best sorting algorithm for your specific needs and optimize your code for performance.
Applying Selection Sort in Larger Projects
While selection sort can be a useful tool in your programming arsenal, its application goes beyond sorting small arrays. It’s a fundamental algorithm that forms the basis for understanding more complex sorting algorithms. Understanding selection sort can also help you grasp other important computer science concepts, such as recursion and dynamic programming.
In larger projects, you might use selection sort as a subroutine in a more complex algorithm. For instance, you might use it to sort small sections of a data set in a divide-and-conquer algorithm. Or, you might use it to sort the data before applying a search algorithm, to make the search process faster.
However, remember that selection sort has a time complexity of O(n^2), which makes it inefficient for sorting large data sets. In such cases, other sorting algorithms, such as quicksort or mergesort, might be more appropriate.
Expanding Your Knowledge: Data Structures, Recursion, and Dynamic Programming
To take your Java programming skills to the next level, consider learning more about data structures, recursion, and dynamic programming.
Data structures are a way of organizing and storing data so that they can be accessed and worked with efficiently. They are fundamental to programming and are used in nearly every aspect of software development.
Recursion is a method where the solution to a problem depends on solutions to smaller instances of the same problem. It’s a common technique for solving problems in computer science and can often make code more readable and easier to understand.
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s used in many areas of computer science, including artificial intelligence, computer graphics, and bioinformatics.
Further Resources for Java Sorting Algorithms
To help you dive deeper into these topics, here are some resources that you might find useful:
- Java Stream Efficiency Techniques – Understand how Java streams improve code readability and maintainability.
Exploring Bubble Sort in Java – Master Java bubble sort implementation for small-scale sorting tasks.
Filtering Streams in Java – Learn Java stream filtering techniques for processing collections and arrays.
GeeksforGeeks’ Data Structures in Java is a guide to selection sort in Java.
Baeldung’s Guide to Recursion in Java covers the basics of recursion in Java, including how to write recursive methods
Selection Sort in Java – This javatpoint tutorial explains the selection sort algorithm in Java.
Wrapping Up: Selection Sort in Java
In this comprehensive guide, we’ve delved into the intricacies of implementing the selection sort algorithm in Java. We’ve explored its basic use, optimization techniques, and even touched on common errors and their solutions.
We started with the basics, learning how to implement selection sort in Java. We then moved on to an optimized version of the algorithm, reducing the number of swap operations for better efficiency. We also tackled common issues that you might encounter when implementing selection sort, and provided solutions to help you overcome these challenges.
We didn’t stop at selection sort. We ventured into alternative sorting algorithms, such as quick sort, merge sort, and bubble sort, comparing their time and space complexities with selection sort. This gave us a broader perspective on the landscape of sorting algorithms in Java. Here’s a quick comparison of these algorithms:
Algorithm | Time Complexity | Space Complexity |
---|---|---|
Selection Sort | O(n^2) | O(1) |
Quick Sort | O(n log n) | O(log n) |
Merge Sort | O(n log n) | O(n) |
Bubble Sort | O(n^2) | O(1) |
Whether you were struggling with implementing selection sort in Java or just needed a refresher, we hope this guide has given you a deeper understanding of the algorithm, its optimization techniques, and alternatives. With this knowledge, you’re well-equipped to tackle sorting challenges in your future Java projects. Happy coding!