Python sum(): How to Get the Sum of Python List Elements
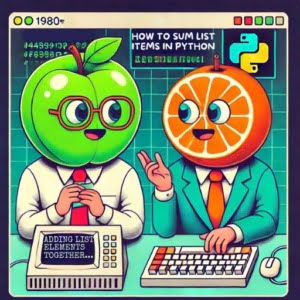
Aggregating and formatting Data has been on the forefront of our automation projects at IOFLOOD, and during this we have gotten familiar with the process of summing elements in Python lists. This simple process helps in calculating total values, for efficient data analysis. To aid our customers that are wanting to improve their data aggregation practices on their custom server configurations, we are providing our tips and processes in today’s article.
In this easy-to-follow guide, we’ll focus not only on the basics but also on advanced and practical applications of summing elements in a Python list, offering techniques that can take your Python programming to the next level.
So, let’s dive deeper and explore different techniques to calculate the sum of elements in a Python list.
TL;DR: How do I sum elements in a Python list?
The simplest way to sum elements in a Python list is by using the inbuilt
sum()
function with the syntax,sum(listOfNumbers)
. It takes an iterable, like a list, as its primary argument and returns the sum of its elements. For more advanced methods, read the rest of the article.
numbers = [1, 2, 3, 4, 5]
print(sum(numbers)) # Output: 15
Table of Contents
Using Python’s sum() Function
One of the simplest ways to calculate the sum of a Python list is by using the inbuilt sum()
function. This is akin to you manually counting each apple in each basket one by one. Python is known for its ‘batteries included’ philosophy, meaning it comes packed with pre-built functions like sum()
, designed to make your life as a programmer easier.
The sum()
function is straightforward to use. It takes an iterable (like our list) as its primary argument and returns the sum of its elements. The syntax is as follows:
sum(iterable, start)
Start Parameter
The start
parameter is optional and defaults to 0. It’s added to the sum of numbers in the iterable. Let’s see a quick example:
Code | Output |
---|---|
print(sum(numbers)) | 15 |
print(sum(numbers, 10)) | 25 |
numbers = [1, 2, 3, 4, 5]
print(sum(numbers)) # Output: 15
print(sum(numbers, 10)) # Output: 25
In the second print statement, we’ve used the start
parameter to add 10 to our sum.
sum() with other iterables
The sum()
function processes the iterable from left to right. It’s versatile and can be used with different data structures like dictionaries, sets, and tuples, not just lists. For instance, if you want to sum all the values in a dictionary, you can do it as follows:
dictionary = {'a': 5, 'b': 3, 'c': 2}
print(sum(dictionary.values())) # Output: 10
For more information on how to use Python sets, feel free to check out our free guide!
Computing Averages with the sum() Function
The sum()
function can also be used to easily compute the average of a list’s elements by dividing the sum by the length of the list:
numbers = [1, 2, 3, 4, 5]
average = sum(numbers) / len(numbers)
print(average) # Output: 3.0
This is just the tip of the iceberg when it comes to using the sum()
function. As we continue, we’ll explore more advanced techniques to calculate the sum of a Python list.
Handling Lists with Mixed Data Types
A more complex scenarios is handling lists with mixed data types. If you’ve ever tried to sum a list containing both string values and integers, you’ve likely run into a few challenges, just like you would if you tried to count apples and oranges together.
Let’s consider a simple example:
mixed_list = [1, '2', 3, '4', 5]
Attempting to use the sum()
function on this list as is, would result in a TypeError. Python doesn’t know how to add an integer and a string together, hence the error. So, how do we get around this problem?
Casting to int()
One solution is to use the int()
function inside a for loop to convert the string values to integers before summing them up. Here’s how you can do it:
mixed_list = [1, '2', 3, '4', 5]
total = 0
for i in mixed_list:
total += int(i)
print(total) # Output: 15
In the above code, we iterate over each element in the list. The int()
function is used to convert the elements to integers, whether they’re already integers or strings. Then we add each element to the total.
Code | Output |
---|---|
print(sum(mixed_list)) | TypeError |
print(total) | 15 |
Other data type problems
While this approach solves our immediate problem, it’s important to be aware of potential pitfalls.
For instance, if the list contains a string that cannot be converted to an integer (like ‘hello’), the int()
function will raise a ValueError. In such cases, you might need to use error handling techniques, such as try/except blocks, to ensure your program doesn’t crash.
Alternatives to sum()
While Python’s sum()
function is a powerful tool for summing lists, it’s not the only tool in our arsenal. Let’s explore 3 other options: for loop, add() method, and a while loop.
Method | Description | Pros | Cons |
---|---|---|---|
For Loop | Sum all numbers in [1,2,3,4,5] using a basic for loop and incremental variable. | Familiarity and ease of use for beginners. | Might require more lines of code for complex scenarios. |
While Loop | Takes the elements from [1,2,3,4,5] in a loop as long as the list is not empty, adding each element to a total sum. | High control over loop execution flow. | Risk of infinite loops if condition is not wisely stated. |
reduce() Method | Uses Python’s functools module’s reduce method to apply function of two arguments cumulatively to the elements of [1,2,3,4,5], from left to right, so as to reduce the list to a single output. | Excellent for applying a function for a sequence of arguments in an iterable. | Not a built-in function, it needs to be imported from functools module. |
Using a ‘For’ Loop for summation
A for
loop allows us to iterate over each element in the list, adding each one to a running total, much like manually counting each apple in a basket. Here’s a simple example:
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num
print(total) # Output: 15
In this code, we initialize a variable total
to 0. Then, for each number in our list, we add it to total
. The result is the sum of our list.
Using the add() Method
Beyond the for
loop and sum() method, Python also offers the add()
method from the operator module as another option for summing a list. The add()
method can be used with the reduce()
function from the functools module to sum a list as follows:
from operator import add
from functools import reduce
numbers = [1, 2, 3, 4, 5]
total = reduce(add, numbers)
print(total) # Output: 15
In this code, the reduce()
function applies the add()
method to the first two items in the list, then to the result and the next item, and so on, effectively ‘reducing’ the list to a single output.
Loops offer flexibility for more complex operations beyond summing. For instance, you could modify the for
loop to perform calculations based on the previous element in the list, something that would be tricky with the sum()
function. On the other hand, the sum() method allows for concise and efficient code, making your scripts cleaner and easier to read.
Using a While Loop
So far, we’ve discussed several ways to calculate the sum of a Python list, from using Python’s inbuilt sum()
function to leveraging for
loops and list comprehension. Now, let’s turn our attention to another essential tool in Python: the while
loop, which is like counting apples in a basket until the basket is empty.
The while
loop offers a different approach to iteration. Instead of iterating over a sequence of elements like a for
loop, a while
loop continues as long as a certain condition is true. This makes while
loops particularly useful in scenarios where the number of iterations is not known in advance.
Here’s how you can use a while
loop to calculate the sum of a list:
numbers = [1, 2, 3, 4, 5]
total = 0
i = 0
while i < len(numbers):
total += numbers[i]
i += 1
print(total) # Output: 15
In this code, we initialize a counter i
to 0 and a variable total
to hold our sum. The while
loop continues as long as i
is less than the length of the list. Inside the loop, we add the current element to total
and increment i
by 1.
When comparing the
while
loop method with thefor
loop andsum()
function, thewhile
loop can be more verbose and slightly more complex due to the need to manually manage the loop variable. However, it offers greater control over the looping process, which can be beneficial in more complex scenarios.
Further Resources for Python
If you’re interested in learning more ways to utilize the Python language, here are a few resources that you might find helpful:
- An In-Depth Look at Python List Comprehension: Learn the powerful concept of list comprehension in Python and unlock its potential.
Tutorial on Sorting a List in Python: IOFlood’s tutorial provides different methods for sorting a list in Python, including using the sorted() function, the sort() method, and custom sorting with lambda functions.
Guide on Reversing a List in Python: IOFlood’s guide demonstrates various approaches to reverse a list in Python, such as using the reversed() function, the reverse() method, and slicing with a negative step.
Extensive Guide on Python Syntax: IOFlood’s guide provides an extensive overview and cheat sheet of Python syntax, covering various topics such as variables, data types, control flow statements, loops, functions, classes, and more. It serves as a comprehensive reference for Python programmers of all levels.
Python sum() Function: A Comprehensive Guide: A comprehensive guide on GeeksforGeeks that explains how to use the sum() function in Python to calculate the sum of elements in a list or iterable.
How to Compute the Sum of a List in Python: An article on Educative that demonstrates different approaches to compute the sum of elements in a list in Python.
Sum of Elements in a List in Python: A tutorial on SparkByExamples that showcases different methods to compute the sum of elements in a list using Python.
Conclusion: Summing Python Iterables
We’ve journeyed through the various ways to calculate the sum of a Python list, each with its unique strengths and considerations. It’s like we’ve explored different methods to count apples in our baskets. Let’s take a moment to recap.
The sum()
function is Python’s inbuilt tool for summing an iterable. It’s simple to use, efficient, and works with different data structures, making it a solid first choice for many scenarios, just like counting apples one by one from each basket.
When dealing with lists that contain mixed data types, we learned that a for
loop can be a handy tool. By using a for
loop with the int()
function, we can convert all elements to integers and sum them up, even if the list contains strings. It’s like separating apples and oranges before counting them.
Additionally, we introduced the add()
method from the operator module as another option for summing a list. Finally, the while
loop provides a different approach to iteration, proving useful when the number of iterations is not known in advance. It’s like counting apples until the basket is empty.
By understanding these techniques and their practical applications, you can write more efficient, versatile code and take your Python skills to the next level. So keep exploring, keep learning, and most importantly, keep coding!