Learn Python: How To Sort a List with sort() and sorted()
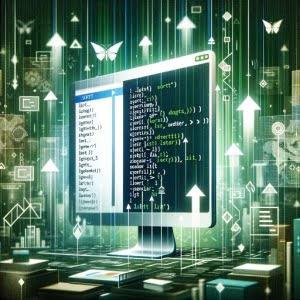
Properly sorting a list in Python is a basic task, yet it is essential when organizing data for use in our scripts at IOFLOOD. In our experience, sorting lists makes data more accessible and easier to analyze. Today’s article aims to provide valuable insights and examples to assist our customers that are having questions on managing data in scripts on their dedicated cloud servers.
This comprehensive guide is designed to take you on a journey through the various techniques of sorting lists in Python. Whether you’re just starting out or already have some experience under your belt, there’s something here for everyone. We’ll start with the basics and gradually delve into more advanced methods.
So, let’s dive in and tame those unruly lists!
TL;DR: How Do I Sort a List in Python?
You can quickly sort a list in Python using the built-in function
sorted()
or thesort()
function with the syntax,my_list.sort()
.
Let’s take a look at a simple example using the sort()
function:
my_list = [3, 1, 4, 1, 5, 9]
my_list.sort()
print(my_list)
# Output:
# [1, 1, 3, 4, 5, 9]
In this example, we’ve used sort()
to arrange the elements of my_list
in ascending order. By invoking sort()
on my_list
, Python automatically sorts the list in-place, meaning the original list is rearranged without creating a new list. The print()
function then outputs the sorted list.
Intrigued? Stick around as we unravel more detailed explanations and advanced usage scenarios to help you become a pro at sorting lists in Python!
Table of Contents
The Basics: sort()
and sorted()
Python offers two built-in functions for sorting lists: sort()
and sorted()
. Both functions serve the same purpose, but they do have some key differences.
The sort()
Function
The sort()
function is a method that you can call on a list object to sort the items in place. This means it modifies the original list. Here’s a simple example:
my_list = [3, 1, 4, 1, 5, 9]
my_list.sort()
print(my_list)
# Output:
# [1, 1, 3, 4, 5, 9]
In this example, sort()
rearranges the elements of my_list
in ascending order. The original list is modified without creating a new list.
The sorted()
Function
On the other hand, the sorted()
function returns a new sorted list, leaving the original list unaffected. Here’s how you can use it:
my_list = [3, 1, 4, 1, 5, 9]
sorted_list = sorted(my_list)
print(sorted_list)
print(my_list)
# Output:
# [1, 1, 3, 4, 5, 9]
# [3, 1, 4, 1, 5, 9]
As you can see, sorted()
creates a new sorted list, sorted_list
, while my_list
remains unchanged.
Choosing Between sort()
and sorted()
So, when should you use sort()
and when should you use sorted()
? If you want to keep the original list intact and create a sorted version of it, go for sorted()
. However, if you don’t need the original list and want to save memory, sort()
is your best bet as it sorts the list in-place.
Remember, while these functions make sorting lists in Python a breeze, they work best with lists that contain the same type of items. Mixing different data types in a list can lead to unexpected results or errors. But don’t worry, we’ll tackle that in the ‘Troubleshooting and Considerations’ section.
If you have questions on the available data types in Python, you can refer to this cheat sheet.
Advanced Techniques: Python Sorting
After mastering the basics, it’s time to explore some more advanced sorting techniques in Python. These techniques allow you to control the sorting process more precisely and handle more complex situations.
Sorting with a Key Function
Both sort()
and sorted()
functions accept a key
parameter that you can use to specify a function of one argument that is used to extract a comparison key from each list element. Let’s say you have a list of strings that you want to sort by length:
my_list = ['apple', 'fig', 'kiwi', 'cherry', 'banana']
sorted_list = sorted(my_list, key=len)
print(sorted_list)
# Output:
# ['fig', 'kiwi', 'apple', 'banana', 'cherry']
In this example, len
is used as the key function, which means the list is sorted based on the lengths of its elements.
Reverse Sorting
Both sort()
and sorted()
also accept a reverse
parameter. If reverse=True
is passed, the list elements are sorted as if each comparison were reversed:
my_list = [3, 1, 4, 1, 5, 9]
sorted_list = sorted(my_list, reverse=True)
print(sorted_list)
# Output:
# [9, 5, 4, 3, 1, 1]
In this example, sorted()
sorts the elements in descending order.
Sorting Complex Objects
You can also sort lists of complex objects, like tuples or objects, by using the key
parameter. For example, if you have a list of tuples representing points in a 2D space, you can sort them by their distance from the origin:
import math
points = [(1, 2), (3, 2), (1, 1), (2, 2)]
def distance(point):
return math.sqrt(point[0]**2 + point[1]**2)
sorted_points = sorted(points, key=distance)
print(sorted_points)
# Output:
# [(1, 1), (1, 2), (2, 2), (3, 2)]
In this example, distance
is a function that calculates the Euclidean distance from a point to the origin. It’s used as the key function to sort the list of points.
Exploring Alternative Sorting Methods
Python’s built-in sorting functions are wonderfully versatile, but there are other powerful tools in Python’s arsenal for more specialized sorting tasks. Let’s take a look at a couple of them.
The heapq
Module for Large Lists
The heapq
module provides functions for using lists as heaps, which are binary trees for which every parent node is less than or equal to its child node. This is especially useful for large lists where efficiency is a concern. The smallest element gets pushed to the index position 0. But the rest of the data is not necessarily sorted.
import heapq
my_list = [3, 1, 4, 1, 5, 9]
heapq.heapify(my_list)
print(my_list)
# Output:
# [1, 1, 3, 4, 5, 9]
In this example, we use heapify()
to transform the list into a heap, in-place, in linear time. While the resulting list is not fully sorted, it guarantees that the first element is the smallest.
The bisect
Module for Maintaining Sorted Lists
The bisect
module offers tools for maintaining a list in sorted order without having to sort the list after each insertion. For example, if you’re repeatedly adding elements to a list and looking up elements in it, bisect
can be a more efficient approach.
import bisect
my_list = [1, 3, 3, 4, 4, 6, 7]
bisect.insort(my_list, 5)
print(my_list)
# Output:
# [1, 3, 3, 4, 4, 5, 6, 7]
In this example, insort()
inserts 5 into its correct position in my_list
, maintaining the list’s sorted order.
These alternative methods each have their advantages and disadvantages. The heapq
module is excellent for dealing with large data sets efficiently, but it doesn’t fully sort the list. On the other hand, the bisect
module is fantastic for maintaining sorted lists, but it’s not designed for sorting unsorted lists. Choose the tool that best fits your use case.
Handling Issues in Python List Sorting
While sorting lists in Python is straightforward most of the time, you might occasionally run into some common issues. Let’s discuss how to handle these situations.
Sorting Mixed Data Types
Python’s sorting functions expect list elements of the same type. If you try to sort a list with mixed data types, you’ll encounter a TypeError
:
my_list = [3, 'two', 1]
try:
my_list.sort()
except TypeError as e:
print(e)
# Output:
# '<' not supported between instances of 'str' and 'int'
In this example, the sort()
function raises a TypeError
because it doesn’t know how to compare an integer (int
) and a string (str
). One way to handle this is to use a key function that can handle all the types in your list.
Handling None
Values
The None
keyword, used to represent the absence of values, can also cause issues when sorting lists. Python’s sorting functions handle None
values in a specific way: they consider None
to be less than any number. This means that None
values will always be at the start of the sorted list:
my_list = [3, None, 1, 2]
my_list.sort()
print(my_list)
# Output:
# [None, 1, 2, 3]
In this example, sort()
places the None
value at the start of the sorted list. If you want to change this behavior, you can use a key function that assigns a high value to None
.
Sorting lists in Python can occasionally throw a curveball at you, but with the right knowledge, you can handle any situation. Always consider the types of the elements in your list and how Python’s sorting functions will handle them.
Unveiling Python’s Sorting Mechanism
To fully harness the power of Python’s sorting functions, it’s helpful to understand the underlying principles. Let’s delve into the workings of sorting algorithms in Python, the concept of stable sorting, and the time complexity of different sorting methods.
Inside Python’s Sorting Algorithm
Python uses a sorting algorithm known as Timsort. It’s a hybrid sorting algorithm, derived from merge sort and insertion sort, designed to perform well on many kinds of real-world data. Timsort takes advantage of the fact that many data sets have order that can be used to increase efficiency.
# Python's built-in sorting algorithm at work
my_list = [3, 1, 4, 1, 5, 9]
my_list.sort()
print(my_list)
# Output:
# [1, 1, 3, 4, 5, 9]
In this example, Python’s Timsort algorithm organizes the elements of my_list
in ascending order.
Stable Sorting in Python
Python’s sorting algorithm is stable, which means that when sorting a list with equal elements (according to the comparison used for sorting), their original order is preserved. This can be useful in scenarios where secondary ordering is important.
# Demonstrating stable sorting
my_list = ['apple', 'banana', 'APPLE', 'BANANA']
my_list.sort(key=str.lower)
print(my_list)
# Output:
# ['apple', 'APPLE', 'banana', 'BANANA']
In this example, the list is sorted in alphabetical order, but the original order of ‘apple’ and ‘APPLE’, ‘banana’ and ‘BANANA’ is preserved.
Time Complexity of Sorting
The time complexity of a sorting algorithm quantifies the amount of time taken by an algorithm to run, as a function of the length of the input. Python’s Timsort has a worst-case and average time complexity of O(n log n), making it efficient for large-scale data.
Understanding these fundamentals gives you a deeper insight into Python’s sorting capabilities and helps you make better decisions when writing Python code.
Practical Use Cases of List Sorting
List sorting, while seemingly basic, plays a significant role in many advanced fields such as data analysis and machine learning. In data analysis, sorting is often the first step in understanding a new dataset. It allows us to identify patterns, detect outliers, and make data-driven decisions.
# Example of data analysis using sorting
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 45, 35]}
df = pd.DataFrame(data)
sorted_df = df.sort_values(by='Age')
print(sorted_df)
# Output:
# Name Age
# 0 Alice 25
# 2 Charlie 35
# 1 Bob 45
In this example, we use the sort_values()
function from pandas, a popular data analysis library in Python, to sort a DataFrame by age. This allows us to quickly see the age distribution of the dataset.
In machine learning, sorting is used in various algorithms, such as k-nearest neighbors for classification and regression, and in model evaluation techniques like ROC AUC.
Python’s sorting capabilities can be further extended when combined with other powerful features of the language like list comprehensions and lambda functions. For example, you can use a list comprehension to generate a list of numbers and a lambda function as a key to sort the list in a custom way.
# Example of using list comprehension and lambda function
my_list = [i for i in range(10)] # List comprehension
sorted_list = sorted(my_list, key=lambda x: -x) # Lambda function
print(sorted_list)
# Output:
# [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
In this example, we use a list comprehension to generate a list of numbers from 0 to 9, and a lambda function as a key to sort the list in descending order.
Further Resources for Python
If you’re interested in learning more about Python’s sorting capabilities and related topics, here are a few resources that you might find helpful:
- Essential Python List Manipulation Techniques: Discover essential techniques for manipulating and transforming Python lists to meet your project needs.
Tutorial on Removing an Item from a List in Python IOFlood’s tutorial explains various methods to remove an item from a list in Python, including using the remove() function, list comprehension, and slicing.
Calculating the Sum of Elements in a List using Python: This guide demonstrates different approaches to calculate the sum of elements in a list using Python, such as using a for loop, the sum() function, and the reduce() function from the functools module
Python documentation on sorting: The official Python documentation provides detailed information on how to sort data in Pythons.
pandas documentation on sort_values() function: The pandas documentation explains the sort_values() function.
scikit-learn documentation on KNeighborsClassifier: The scikit-learn documentation provides an overview of the KNeighborsClassifier class, which is used for classification tasks in Python.
Recap: Python List Sorting
We’ve taken a comprehensive journey through the world of sorting lists in Python. Let’s recap what we’ve learned.
We began with Python’s built-in sort()
and sorted()
functions. sort()
sorts a list in-place, modifying the original list, while sorted()
returns a new sorted list and leaves the original list untouched. Both functions are versatile and can be customized with the key
and reverse
parameters.
Next, we explored advanced sorting techniques like sorting with a key function, reverse sorting, and sorting complex objects. These techniques allow for more control and flexibility when sorting lists in Python.
For more specialized tasks, we discussed alternative sorting methods provided by the heapq
and bisect
modules. The heapq
module is useful for efficiently handling large lists, while the bisect
module is great for maintaining sorted lists.
We also covered how to handle common issues when sorting lists, such as sorting mixed data types and handling None
values. Remember, Python’s sorting functions expect list elements of the same type, and they consider None
to be less than any number.
Finally, we delved into the underlying mechanics of Python’s sorting algorithm, Timsort, the concept of stable sorting, and the time complexity of sorting. Understanding these fundamentals can help you write more efficient Python code.
In conclusion, sorting lists in Python is a versatile and powerful tool in your Python toolkit. Whether you’re just starting out or are a seasoned Pythonista, understanding how to sort lists effectively can help you in a wide array of tasks, from data analysis to machine learning. So keep practicing, keep experimenting, and happy sorting!