Python’s len() Function: A Comprehensive Guide
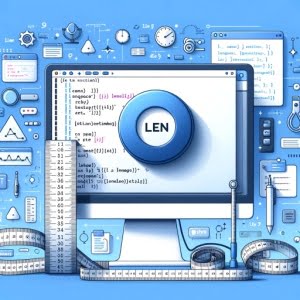
Are you grappling with the len() function in Python? Just like a ruler measures length, Python’s len() function helps you measure the length of various data types. It’s a simple yet powerful tool that can be a bit tricky to understand at first.
This comprehensive guide is designed to take you on a journey from the basics of the len() function to its advanced usage. Whether you’re a beginner just starting out or an intermediate looking to sharpen your skills, this guide has something for you. So, let’s dive in and unravel the mysteries of the len() function in Python.
TL;DR: How Do I Use the len() Function in Python?
The len() function in Python is used to determine the length of an object, whether that’s a string, list, tuple, or other iterable types. Here’s a straightforward example:
my_list = [1, 2, 3, 4, 5]
print(len(my_list))
# Output:
# 5
In this example, we have a list named ‘my_list’ with five elements in it. When we use the len() function on ‘my_list’, it returns 5, which is the number of elements in the list.
This is just the tip of the iceberg when it comes to the len() function in Python. Stick around for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Getting Started with len() in Python
- Using len() with Dictionaries and Sets
- Exploring Alternative Methods for Measuring Length in Python
- Navigating Common Issues with Python’s len() Function
- Diving Deeper: Python’s Built-in Functions and Length Concept
- The Power of len() in Data Analysis and Machine Learning
- Exploring Related Concepts
- Wrapping Up: The len() Function in Python
Getting Started with len() in Python
The len() function in Python is a built-in function that returns the number of items in an object. It’s quite straightforward when used with basic data types such as lists, tuples, and strings.
Let’s start with a simple string:
my_string = 'Hello, World!'
print(len(my_string))
# Output:
# 13
In this case, len() counts the number of characters in the string, including spaces and punctuation.
Working with Lists and Tuples
The len() function also works with lists and tuples, returning the number of elements in them:
my_list = [1, 2, 3, 4, 5]
print(len(my_list))
# Output:
# 5
my_tuple = ('apple', 'banana', 'cherry')
print(len(my_tuple))
# Output:
# 3
Here, len() returns the number of elements in the list and the tuple, respectively.
While len() is a powerful and useful function, it’s important to remember that it only works with iterable objects. Attempting to use len() on an integer or a float, for example, will result in a TypeError.
Using len() with Dictionaries and Sets
As we delve deeper into the len() function, we discover its versatility. It’s not just limited to strings, lists, and tuples – it can also handle more complex data types such as dictionaries and sets.
Measuring Dictionary Length
A dictionary in Python is an unordered collection of items. Each item is stored as a key-value pair. Let’s see how len() works with dictionaries:
dictionary = {'apple': 1, 'banana': 2, 'cherry': 3}
print(len(dictionary))
# Output:
# 3
In this case, the len() function returns the number of key-value pairs in the dictionary.
Counting Elements in Sets
A set is another data type in Python that can be measured with len(). A set is an unordered collection of unique elements. Here’s how you can use len() with sets:
fruits = {'apple', 'banana', 'cherry', 'apple'}
print(len(fruits))
# Output:
# 3
Even though ‘apple’ appears twice in our set, len() returns 3. This is because sets only consider unique elements.
While len() is a versatile function, it’s important to remember its limitations. It works with iterable objects, but it doesn’t always behave the same way with different data types. Understanding these differences is key to using len() effectively.
Exploring Alternative Methods for Measuring Length in Python
While len() is the most common way to measure the length of an object in Python, it’s not the only way. Python offers other methods that can be used to get the length of an object, such as the __len__()
method.
Using the len() Method
If you’ve ever wondered how the len() function works behind the scenes, the answer lies in the __len__()
method. Python objects that support len() usually have a __len__()
method defined. When you call len() on an object, Python internally calls the object’s __len__()
method.
Here’s an example:
my_list = [1, 2, 3, 4, 5]
print(my_list.__len__())
# Output:
# 5
As you can see, calling __len__()
on our list gives us the same result as calling len().
While you can use __len__()
directly, it’s generally recommended to use the len() function instead. This is because len() checks if the object has a __len__()
method and calls it, providing a level of abstraction that makes your code more flexible and easier to read.
It’s also worth noting that some objects may not have a __len__()
method. In these cases, len() will raise a TypeError, while calling __len__()
directly will raise an AttributeError.
Understanding these alternative approaches not only gives you more tools to work with, but also provides insight into the inner workings of Python and its design philosophy.
As with any tool, using the len() function in Python can sometimes lead to unexpected issues. The most common issue you might encounter is a TypeError when trying to measure the length of an object that doesn’t have a defined length. This is typically seen with data types like integers and floats.
Understanding the TypeError
Let’s look at an example of this issue:
number = 12345
print(len(number))
# Output:
# TypeError: object of type 'int' has no len()
In this case, we’re trying to use len() on an integer, which doesn’t have a defined length. As a result, Python raises a TypeError.
How to Avoid TypeErrors with len()
One way to avoid this issue is to always ensure that the object you’re passing to len() is iterable. Strings, lists, tuples, dictionaries, and sets are all iterable types that can be measured with len().
If you’re unsure whether an object is iterable, you can check its type using the type() function:
number = 12345
print(type(number))
# Output:
# <class 'int'>
In this example, type() tells us that ‘number’ is an integer. Since integers aren’t iterable, we know that we can’t use len() on ‘number’.
By understanding these common issues and how to navigate them, you can use the len() function more effectively and avoid potential pitfalls.
Diving Deeper: Python’s Built-in Functions and Length Concept
To fully grasp the power of the len() function in Python, it’s crucial to understand the concept of Python’s built-in functions and how the idea of ‘length’ applies to different data types.
Python’s Built-in Functions
Python comes equipped with a variety of built-in functions, len() being one of them. These functions are always available for use, providing functionality that would otherwise require multiple lines of code. For instance, the len() function conveniently measures the length of various data types, from strings to dictionaries.
# len() with a string
print(len('Hello, World!')) # Output: 13
# len() with a list
print(len([1, 2, 3, 4, 5])) # Output: 5
# len() with a dictionary
print(len({'apple': 1, 'banana': 2, 'cherry': 3})) # Output: 3
In each of these examples, len() quickly and accurately provides the length of the object.
Understanding ‘Length’ in Different Data Types
The concept of ‘length’ can vary depending on the data type. For strings, ‘length’ refers to the number of characters. For lists, tuples, and dictionaries, ‘length’ refers to the number of elements or items.
# 'Length' in a string
print(len('abc')) # Output: 3
# 'Length' in a list
print(len([1, 2, 3])) # Output: 3
# 'Length' in a dictionary
print(len({'a': 1, 'b': 2, 'c': 3})) # Output: 3
Even though the objects in these examples are different, len() returns the same result because each object contains three items or characters.
By understanding Python’s built-in functions and the concept of ‘length’, you’ll be better equipped to use the len() function effectively.
The Power of len() in Data Analysis and Machine Learning
The len() function isn’t just for counting characters in a string or elements in a list. It plays a significant role in more advanced fields such as data analysis and machine learning.
len() in Data Analysis
In data analysis, understanding the size of your data is crucial. Whether you’re dealing with a list of sales figures or a dictionary of customer data, len() helps you understand the scope of your data.
data = [120, 150, 90, 200, 95]
print(len(data))
# Output:
# 5
In this example, len() tells us that we have data for five sales figures.
len() in Machine Learning
In machine learning, len() can be used to measure the size of datasets, among other things. This can help when splitting data into training and test sets, for instance.
# Let's say we have a dataset 'X' with 1000 samples
X = list(range(1000))
print(len(X))
# Output:
# 1000
In this case, len() tells us that we have 1000 samples in our dataset.
Exploring Related Concepts
While len() is a powerful tool, Python offers many other functions and concepts worth exploring. Here are some resources to get you started:
- Python Data Types: Quick Dive – Explore set manipulation techniques to work with collections of unique elements.
Exploring Set Differences in Python – Master set difference calculations in Python for data filtering and set algebra.
Python Named Tuple: Simplifying Data Structures – Dive into named tuples in Python for creating lightweight data structures with named fields.
Python Lambda, Filter, Map, Reduce – Detailed guide on Python’s functions and capabilities by GeeksforGeeks.
Python’s Official Documentation on Array – Understanding arrays in Python from the official documentation.
Queue in Python Explained – Expert guide on implementing queues in Python by Real Python.
There’s always more to learn in Python. So get started here and have fun!
Wrapping Up: The len() Function in Python
The len() function is a powerful and versatile tool in Python, capable of measuring the length of various data types, from strings and lists to dictionaries and sets. It’s a built-in function that provides a quick and easy way to understand the size or count of an object.
Throughout this guide, we’ve explored the basic and advanced usage of len(), delving into its application with different data types. We’ve also encountered potential pitfalls, such as TypeErrors, and offered solutions to navigate these issues.
Beyond the len() function, we’ve discussed alternative approaches like the __len__()
method. While these alternatives offer additional tools for measuring length, they come with their own considerations and are generally best used in specific circumstances.
Both the len() function and __len__()
method return the same result, but len() is generally preferred for its readability and flexibility.
Whether you’re just starting your Python journey or looking to deepen your understanding, the len() function is a fundamental tool in your Python toolkit. It’s a simple function with a wide range of applications, from basic programming tasks to data analysis and machine learning.