Python Set difference() | Usage Guide with Examples
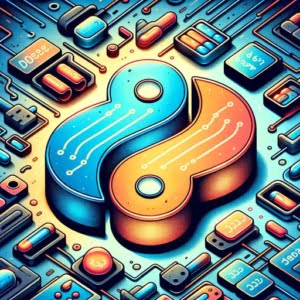
The Python set difference function, often overlooked, can significantly boost your data handling abilities. However, like any potent tool, it comes with its complexities and common pitfalls.
In this guide, we’ll delve into the Python set difference function, exploring its usage, syntax, and common errors. By the end of this read, you’ll have a thorough understanding of this function and be prepared to apply it in your Python projects.
So, are you ready to harness the power of the Python set difference function and enhance your Python programming skills? Let’s get started!
TL;DR: What is the Python set difference function?
The Python set difference function is a built-in function that returns a new set containing elements present in the first set but not in the second set. It’s a powerful tool for comparing sets and finding unique elements. Here’s a simple example:
# Define two sets
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
# Use the difference function
result = set1.difference(set2)
# Print the result
print(result) # Output: {1, 2}
For more advanced methods, background, tips, and tricks, continue reading the article.
Table of Contents
Understanding Set Difference
Python, a highly versatile language, offers a rich set of built-in functions. Among these, the set difference function stands out. As the name implies, it’s used to find the difference between two sets. But what exactly does that entail? Let’s dissect it.
Definition and Usage
The Python set difference function returns a new set containing elements found in the first set but absent in the second. It proves invaluable when you need to compare two sets and identify elements exclusive to the first one. Here’s an example of how to use it:
# Define two sets
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
# Use the difference function
result = set1.difference(set2)
# Print the result
print(result) # Output: {1, 2}
In this example, the result is {1, 2}
because these elements exist in set1
but not in set2
.
Syntax
The syntax for the set difference function is fairly simple. You call the difference()
method on a set and pass the other set as an argument, like so: result = set1.difference(set2)
.
Let’s say we have two sets, set1
and set2
. Here is how you can use the difference()
method.
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
result = set1.difference(set2)
print(result) # Outputs: {1, 2, 3}
In this example, result
will be a set containing all the elements that are in set1
but not in set2
, which are 1, 2, and 3.
Return Values
The difference function yields a new set containing the difference between two sets. If there’s no difference, it returns an empty set. Crucially, it doesn’t alter the original sets.
Here are two examples demonstrating how the difference()
method works with sets.
# Example 1: The sets have some differences
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
result = set1.difference(set2)
print(result) # Outputs: {1, 2, 3}
print(set1) # Outputs: {1, 2, 3, 4, 5}, set1 remains unchanged
# Example 2: The sets have no differences
set3 = {1, 2, 3}
set4 = {1, 2, 3}
result = set3.difference(set4)
print(result) # Outputs: set(), an empty set
print(set3) # Outputs: {1, 2, 3}, set3 remains unchanged
In examples above, the difference method returns a new set without modifying the original sets.
Set Difference Method vs ‘-‘ Operator
Python also offers the ‘-‘ operator to find the set difference. However, there are notable differences in usage and operator precedence between the difference()
method and the ‘-‘ operator.
The ‘-‘ operator can only handle two sets at a time and has higher operator precedence, while the difference()
method can handle multiple sets simultaneously and has lower operator precedence.
Here is an example that illustrates the differences in operator precedence between the difference()
method and the ‘-‘ operator.
# Set difference via difference() method
set1 = {1, 2, 3, 4, 5}
set2 = {3, 4, 5, 6, 7}
set3 = {1, 2, 8, 9, 10}
result = set1.difference(set2, set3)
print(result) # Outputs: {}, as everything in set1 is also present in either set2 or set3
# Set difference via '-' operator
result_operator = set1 - set2 - set3
print(result_operator) # Outputs: {} as well, as it first diffs set 1 from set 2, and then takes that output and diffs it with set3
In the above case, the end results are the same, but consider what happens in the following example:
# Operator precedence with '-' operator
result_operator_precedence_changed = set1 - (set2 - set3) # This operates differently due to the parentheses
print(result_operator_precedence_changed) # Outputs: {1, 2}
This example outputs {1, 2}
because it first finds the difference between set2 and set3, and then finds the difference between that output and set1
So, {1, 2}
are elements present in set1
but not in the difference between set2
and set3
.
Processing Multiple Sets
A significant strength of Python’s set.difference()
method is its ability to process multiple sets, enhancing its utility in data comparison tasks.
For example, if you have three sets—set1
, set2
, and set3
—you can find the difference between set1
and the other two sets like this: result = set1.difference(set2, set3)
.
Example:
set1 = set([1, 2, 3, 4, 5])
set2 = set([4, 5, 6, 7, 8])
set3 = set([7, 8, 9, 10, 11])
result = set1.difference(set2, set3)
print(result)
When this code is executed, the output you should see will be {1, 2, 3}
. These are the numbers that are in set1
, but not in set2
or set3
.
The difference_update()
method
While difference()
returns a new set with the differences and leaves the original sets unchanged, the difference_update()
method modifies the original set with the result of the difference.
difference_update()
takes as its arguments the other sets you want to use for the comparison, and removes any elements from the original set that are also found in the other sets. It works much the same as the difference()
method, but it doesn’t return anything – it modifies the original set in-place.
Remember that difference_update()
works only on the set that it’s called on. It doesn’t affect other sets.
Here is an example of difference_update()
in action:
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
set3 = {7, 8, 9, 10, 11}
# This will remove all elements from set1 that are also in set2 and set3
set1.difference_update(set2, set3)
print(set1)
When you run this code, you’ll get the following output:
{1, 2, 3}
Here, set1
is updated to remove elements found in set2
and set3
.
difference_update()
can be advantageous when dealing with large datasets because it can be more memory-efficient. Rather than creating a copy of the data, it alters the original set, preventing the redundancy of keeping two sets in memory when only one is needed in the end.
Practical Application
Having established a solid understanding of the Python set difference function, it’s time to put it into action. We’ll walk through an example, explain the output, and discuss key points related to set copying, the use of the minus operator, and the versatility of the difference()
method.
Step-by-Step Example
Consider three sets of integers, and we aim to find the elements unique to the first set. Here’s how we can achieve this:
# Define three sets
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
set3 = {7, 8, 9, 10, 11}
# Use the difference function
result = set1.difference(set2, set3)
# Print the result
print(result) # Output: {1, 2, 3}
In this example, the difference()
method returns {1, 2, 3}
. These are the elements present in set1
but not in set2
or set3
.
Set Copying
It’s crucial to note that the difference()
method does not modify the original sets. Instead, it returns a new set. This is because Python creates a copy of the sets for the operation, which impacts memory allocation.
Consider the following example:
set1 = set([1, 2, 3, 4, 5])
set2 = set([4, 5, 6, 7, 8])
result = set1.difference(set2)
print("set1: ", set1)
print("set2: ", set2)
print("Result: ", result)
After you run this code, you’ll get the following output:
set1: {1, 2, 3, 4, 5}
set2: {4, 5, 6, 7, 8}
Result: {1, 2, 3}
As you can see, even after the difference()
operation, the original sets (set1
and set2
) remain unchanged. That’s because the difference()
method doesn’t directly modify the original sets. Instead, it makes a copy of the sets and performs the operation on these copies.
This behavior is crucial when you’re making temporary modifications using methods like difference()
but still want to preserve the original data.
This operation of making a copy can have implications for memory usage, particularly when working with very large sets. This is because each copy means the data is stored twice in memory.
Therefore, if memory management is a concern, you might need to consider alternate approaches or use in-place methods like difference_update()
, which do modify the original set.
Using the Minus Operator
As previously mentioned, Python also provides the ‘-‘ operator to find the set difference. However, it can only handle two sets at a time. Here’s how you can use it:
# Use the minus operator
result = set1 - set2
# Print the result
print(result) # Output: {1, 2, 3}
Versatility of the Difference() Method
One of the reasons Python’s difference()
method is so powerful is its versatility. It can accept multiple iterables as arguments for set difference computations. This means you can pass lists, tuples, or other sets, and Python will automatically convert them to sets for the operation. For example:
# Define a set and a list
set1 = {1, 2, 3, 4, 5}
list1 = [4, 5, 6, 7, 8]
# Use the difference function
result = set1.difference(list1)
# Print the result
print(result) # Output: {1, 2, 3}
In this example, Python automatically converts list1
to a set before performing the operation, and the result is {1, 2, 3}
.
Common Errors
While Python is a flexible and powerful programming language, it has its share of quirks and common errors. The set difference function is no exception.
You might encounter some errors related to data types and operand types. Let’s delve into these errors and discuss how to debug them.
‘AttributeError: ‘list’ object has no attribute ‘difference”
This error pops up when you attempt to use the difference()
method on a list. Remember, the difference()
method belongs to the set class, hence it’s not applicable to lists. Here’s an error-causing example:
# Define a list
list1 = [1, 2, 3, 4, 5]
# Try to use the difference method
result = list1.difference([4, 5])
To rectify this error, ensure you convert your lists to sets before using the difference()
method.
‘TypeError: unsupported operand type(s) for -: ‘set’ and ‘list”
Error | Cause | Solution |
---|---|---|
‘AttributeError: ‘list’ object has no attribute ‘difference” | Attempting to use the difference() method on a list | Convert lists to sets before using the difference() method |
‘TypeError: unsupported operand type(s) for -: ‘set’ and ‘list” | Attempting to use the ‘-‘ operator between a set and a list | Convert lists to sets before performing the operation |
This error is akin to the previous one. It arises when you try to use the ‘-‘ operator between a set and a list. The ‘-‘ operator is only applicable to two sets. Here’s an example that triggers this error:
# Define a set and a list
set1 = {1, 2, 3, 4, 5}
list1 = [4, 5]
# Try to use the minus operator
result = set1 - list1
The solution is the same as before: convert your lists to sets before performing the operation.
Data Type Validation
One effective way to prevent these errors is through data type validation. Before performing an operation, verify if the variable is of the correct data type. If it’s not, you can convert it to the right data type or handle the error appropriately.
This practice can save you significant debugging time and enhance the robustness and reliability of your Python programs.
Consider the following example:
def difference_of_sets(set1, set2):
# Ensure the parameters are sets
if not isinstance(set1, set):
raise TypeError('set1 must be a set')
if not isinstance(set2, set):
raise TypeError('set2 must be a set')
return set1.difference(set2)
try:
set1 = {1, 2, 3, 4, 5}
set2 = [4, 5, 6] # This is a list, not a set
print(difference_of_sets(set1, set2))
except TypeError as error:
print(error)
In this code block, we have defined a function difference_of_sets
which takes two sets as arguments and returns the difference of them. Inside the function, we validate the data type of the parameters using Python’s built-in method isinstance()
. If the parameters are not sets, the function raises a TypeError
.
In the try
block, we intentionally pass a list as the second parameter to the difference_of_sets
function. The exception is caught in the except
block, and the error message is printed out. If we didn’t validate the data type in the function, Python would raise an error later when trying to use the difference
method on a non-set object.
Further Resources and Related Topics
To extend your prowess on Data Type usage in Python, these resources may prove to be quite valuable:
- Python Data Types Fundamentals Covered – Understand how Python handles boolean data type for logical operations.
len() in Python: Getting Length of Sequences explains the usage of the len() function in Python for length of various data structures.
Set Intersection in Python – Master Python set intersection techniques for data manipulation and filtering.
Guide on Queue in Python – Exploring queue data structures in Python guide by Real Python.
Reference on Python Set – Comprehensive reference for Python’s set functions by W3Schools.
Python 2 Data Structures Official Documentation – In-depth guide on data structures in Python 2 from official resources.
By deepening your understanding of Python related facets, you can become even more adept and efficient as a developer.
Wrapping Up
We’ve taken a comprehensive journey through the Python set difference function in this guide. We began by breaking down the function, explaining what it does and how to use it. We learned that it returns a new set with elements present in the first set but not in the second one, and it’s capable of handling multiple sets, which enhances its utility in data comparison tasks.
We then examined a practical example of the Python set difference function, discussing key points related to set copying and the use of the minus operator. We also highlighted the versatility of Python’s difference()
method that can accept multiple iterables as arguments for set difference computations.
Next, we tackled common errors associated with the Python set difference function and offered debugging tips. We emphasized the importance of using the correct data types in Python and the role of data type validation in preventing errors.
As we wrap up, consider broadening your Python knowledge with this handy reference guide.
By understanding these key points about the Python set difference function, you’re now well-equipped to use this function effectively in your Python programming tasks. So go ahead, give it a try, and start writing cleaner and more efficient Python code!