Python Set intersection() Guide (With Examples)
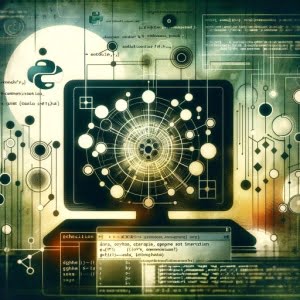
Have you ever faced the challenge of finding common elements from two separate lists in Python? If you have, then you’ve essentially been looking for the ‘intersection’ of two sets.
Much like finding common friends in two separate friend lists, the concept of set intersection can be a powerful tool in your Python programming arsenal.
In this guide, we will demystify the concept of set intersection in Python, explain how it works, and illustrate when and how to use it. So, let’s get started!
TL;DR: How Do I Find the Intersection of Sets in Python?
You can leverage the
intersection()
method or the&
operator to find the intersection of sets in Python. Let’s look at a simple example:
set1 = {1, 2, 3}
set2 = {2, 3, 4}
intersection = set1.intersection(set2)
print(intersection)
# Output:
# {2, 3}
In this example, we have two sets: set1
and set2
. We use the intersection()
method to find the common elements between these two sets, which are 2
and 3
. This result is then printed out.
Intrigued by how this works? Stick around for more detailed examples and advanced usage scenarios that will help you master the concept of Python set intersection.
Table of Contents
Understanding Python Set Intersection
Python provides two main ways to find the intersection of sets – the intersection()
method and the &
operator. Both of these methods return a new set that contains the common elements from the sets being compared.
The intersection()
Method
The intersection()
method is a built-in Python function that finds the common elements from the sets it is applied to. Here’s an example of how it works:
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
common_elements = set1.intersection(set2)
print(common_elements)
# Output:
# {3, 4}
In this example, set1
and set2
are two sets with some common elements. The intersection()
method is applied to set1
and set2
to find the common elements, which are 3
and 4
. These common elements are stored in the common_elements
set and then printed out.
One advantage of the intersection()
method is its readability. It clearly communicates the intention of finding common elements. However, it’s a bit longer than the alternative method.
The &
Operator
The &
operator is another way to find the intersection of sets in Python. It works in the same way as the intersection()
method but is more concise. Here’s an example of how it works:
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
common_elements = set1 & set2
print(common_elements)
# Output:
# {3, 4}
In this example, we use the &
operator to find the common elements between set1
and set2
. The result is the same as the previous example, but the code is shorter.
While the &
operator is more concise, it might be less readable for beginners or those unfamiliar with set operations in Python. However, once you get the hang of it, it’s a quick and efficient way to find the intersection of sets.
Advanced Set Intersection in Python
As you become more comfortable with Python set intersections, you may find yourself wanting to find the intersection of more than two sets. Python makes this easy with both the intersection()
method and the &
operator.
Intersection of Multiple Sets
Let’s look at an example where we find the intersection of three sets:
set1 = {1, 2, 3, 4}
set2 = {2, 3, 4, 5}
set3 = {3, 4, 5, 6}
common_elements = set1.intersection(set2, set3)
# OR, use &
common_elements = set1 & set2 & set3
print(common_elements)
# Output:
# {3, 4}
In this example, we use the intersection()
method to find the common elements among set1
, set2
, and set3
. The intersection()
method can take any number of sets, and it finds the common elements among all of them.
Dealing with Empty Sets
What if one of the sets is empty? Let’s see what happens:
set1 = {1, 2, 3, 4}
set2 = {}
common_elements = set1.intersection(set2)
print(common_elements)
# Output:
# set()
In this case, the intersection()
method returns an empty set, because an empty set has no common elements with any other set. This is a useful feature to remember when working with set intersections in Python, as it handles this edge case gracefully without throwing an error.
Exploring Other Set Operations in Python
While finding the intersection of sets is a common task in Python, there are other set operations that you might find useful. These include the union, difference, and symmetric difference operations. Understanding these operations can help you manipulate and analyze sets more effectively.
Union of Sets
The union of two sets is a set of all elements from both sets. You can find the union of sets in Python using the union()
method or the |
operator. Here’s an example:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union = set1.union(set2)
print(union)
# Output:
# {1, 2, 3, 4, 5}
In this example, the union()
method combines all unique elements from set1
and set2
into a new set.
Difference of Sets
The difference of two sets is a set of elements that are in the first set but not in the second set. You can find the difference of sets in Python using the difference()
method or the -
operator. Here’s an example:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
difference = set1.difference(set2)
print(difference)
# Output:
# {1, 2}
In this example, the difference()
method finds elements that are in set1
but not in set2
.
Symmetric Difference of Sets
The symmetric difference of two sets is a set of elements that are in either of the sets but not in their intersection. You can find the symmetric difference of sets in Python using the symmetric_difference()
method or the ^
operator. Here’s an example:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
symmetric_difference = set1.symmetric_difference(set2)
print(symmetric_difference)
# Output:
# {1, 2, 4, 5}
In this example, the symmetric_difference()
method finds elements that are in set1
or set2
but not in both.
These alternative set operations can be powerful tools in your Python programming toolkit. By understanding and using these operations, you can manipulate sets in Python to solve complex problems.
Troubleshooting Python Set Intersection Issues
While Python’s set operations can be powerful tools, you might encounter some common issues during their use. Let’s discuss these issues and provide some solutions and workarounds.
Dealing with Unhashable Items
In Python, sets only support hashable, or immutable, items. If you try to create a set with unhashable items, such as lists or dictionaries, you’ll encounter a TypeError
. Here’s an example:
set1 = {[1, 2, 3]}
# Output:
# TypeError: unhashable type: 'list'
To solve this issue, you can convert unhashable items into hashable ones. For example, you can convert a list into a tuple, which is hashable:
set1 = {(1, 2, 3)}
print(set1)
# Output:
# {(1, 2, 3)}
Handling Mutable Sets
Another common issue is trying to use mutable sets, or set
objects, as items in another set.
Because
set
objects are mutable, they are unhashable and cannot be included in other sets.
However, Python provides the frozenset
data type, which is a hashable, or immutable, version of a set. Here’s how to use it:
set1 = {frozenset({1, 2, 3})}
print(set1)
# Output:
# {frozenset({1, 2, 3})}
In this example, we create a frozenset
from a set and then include it in another set. The frozenset
data type allows us to include sets as items in other sets.
By understanding these common issues and their solutions, you can avoid potential pitfalls during your Python set operations.
Understanding Python’s Set Data Type and Mathematical Sets
To fully grasp Python set intersection, it’s crucial to understand the underlying concept of sets in both Python and mathematics.
Python’s Set Data Type
In Python, a set is an unordered collection of unique elements. It’s mutable, meaning you can add and remove elements after its creation. Here’s how to create a set in Python:
my_set = {1, 2, 3, 4, 5}
print(my_set)
# Output:
# {1, 2, 3, 4, 5}
In this example, we create a set my_set
with five elements. Notice that the elements are enclosed in curly braces {}
and separated by commas.
Mathematical Concept of Sets
In mathematics, a set is a collection of distinct objects, considered as an object in its own right. Sets are usually symbolized by uppercase, italicized, boldface letters such as A, B, C. The objects in the set are called elements or members.
The concept of intersection comes from the field of set theory in mathematics. The intersection of two sets A and B is a new set that contains all elements that are in both A and B. It’s denoted as A ∩ B.
Connecting the Dots
The Python set data type is an implementation of the mathematical concept of a set. The intersection operation in Python is a direct application of the mathematical intersection operation. By understanding these connections, you can better understand how and why Python set intersection works the way it does.
Expanding Your Python Skills
Python set operations, including intersection, are not just theoretical concepts. They have practical relevance in many areas of computing and data analysis.
Set Operations in Data Analysis
In data analysis, you often deal with large datasets where you need to find common elements. For example, you might want to find common customers in two different sales databases. Python set intersection provides an efficient way to do this.
database1 = {'John', 'Alice', 'Bob'}
database2 = {'Bob', 'Charlie', 'David'}
common_customers = database1 & database2
print(common_customers)
# Output:
# {'Bob'}
In this example, database1
and database2
are two sets of customer names. We use the &
operator to find the common customers, who are ‘Bob’.
Further Learning and Resources
While set operations are powerful tools, Python offers many other features that can help you manipulate and analyze data. For example, list comprehension allows you to create lists based on existing lists. Dictionary operations let you store data in key-value pairs for efficient retrieval.
If you’re interested in learning more about these topics, consider the following online resources.
- Python Data Types Use Cases Explored – Master Python boolean values and operators for conditional expressions and control flow.
Understanding Set Difference in Python – Learn Python set difference methods for comparing and analyzing sets efficiently.
Tuple Usage in Python – Explore Python tuple operations and methods for efficient data handling and processing.
Python 2 Data Structures Official Documentation – Comprehensive guide on data structures in Python 2.
W3Schools’ Python Lists Comprehension Tutorial – Learn Python’s list comprehension from W3Schools.
GeeksforGeeks’ Python Dictionary Methods Guide – Detailed guide on Python dictionary methods by GeeksforGeeks.
Websites like Stack Overflow, Python’s official documentation, and various Python forums can provide more in-depth information and examples.
Wrapping Up Python Set Intersection
We’ve covered a lot of ground in our exploration of Python set intersection. Let’s recap what we’ve learned.
Python Set Intersection: A Quick Recap
Python set intersection allows us to find common elements between two or more sets. We can perform this operation using the intersection()
method or the &
operator. Both methods return a new set with the common elements.
Here’s a quick example of Python set intersection:
set1 = {1, 2, 3}
set2 = {2, 3, 4}
common_elements = set1 & set2
print(common_elements)
# Output:
# {2, 3}
In this example, we use the &
operator to find the common elements between set1
and set2
, which are 2
and 3
.
Common Issues and Solutions
While Python set operations are generally straightforward, we discussed some common issues you might encounter, such as dealing with unhashable items or mutable sets. Remember that sets only support hashable items, and you can use the frozenset
data type to include sets as items in other sets.
Beyond Intersection: Other Set Operations
We also explored other set operations in Python, including union, difference, and symmetric difference. These operations can be performed using their respective methods or operators, and they can help you manipulate and analyze sets in different ways.
Taking Your Python Skills Further
Finally, we discussed the practical relevance of Python set operations in areas like data analysis and highlighted other Python features worth exploring, such as list comprehension and dictionary operations.
By mastering Python set intersection and other set operations, you can enhance your Python programming skills and tackle more complex problems. Keep practicing and exploring, and you’ll continue to grow as a Python programmer.