Python Named Tuple Usage Guide (With Examples)
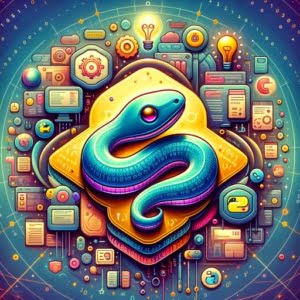
This article will guide you through the power of named tuples in Python, a feature that takes the versatility of tuples a notch higher.
Regular tuples are a type of data structure in Python the while they are incredibly versatile, come with a limitation: the need to remember the index of each element. Named tuples, however, are similar to regular tuples, but with the advantage of accessing elements by name instead of index.
By the end of this guide, you’ll have a solid understanding of how to create, use, and manipulate named tuples effectively in Python. So, let’s dive in and explore how named tuples can transform your Python coding experience!
TL;DR: What are named tuples in Python?
Named tuples in Python are an extension of regular tuples that allow for accessing elements by name instead of index. This feature enhances code readability and efficiency.
For a simple example:
from collections import namedtuple
Person = namedtuple('Person', 'name age')
john = Person(name='John', age=30)
print(john.name) # Output: John
print(john.age) # Output: 30
This guide covers more advanced methods, background, tips, and tricks about named tuples. So keep reading!
Table of Contents
Basics of Named Tuples in Python
Named tuples are a Python feature that augments the functionality of regular tuples. While tuples are handy for grouping data, they can pose challenges when dealing with numerous elements, especially remembering the index of each element.
Named tuples provide a solution to this by allowing you to assign names to each tuple element, enhancing your code’s readability and self-documentation.
Named tuples make your code more self-explanatory, eliminating the need to remember the index of each element. You can access them by their names, making your code easier to understand for both you and others who might read your code.
Creating a Named Tuple
Creating a named tuple in Python is a straightforward process. Python’s collections
module provides the namedtuple()
function for this purpose.
Let’s illustrate this with an example:
from collections import namedtuple
# Define a named tuple
Person = namedtuple('Person', 'name age')
# Create an instance of the named tuple
john = Person(name='John', age=30)
In this example, we’ve defined a named tuple, Person
, with two fields, name
and age
. We then create an instance of Person
named john
.
Accessing Elements in a Named Tuple
Accessing elements in a named tuple is as simple as accessing an attribute in a class. You can do this using their names. Here’s how:
# Access elements in a named tuple
print(john.name) # Output: John
print(john.age) # Output: 30
As demonstrated, we can access the elements name
and age
in the john
instance using dot notation.
Immutability of Named Tuples
Named tuples, like regular tuples, are immutable. Once a named tuple is created, it cannot be altered.
Although this can be desirable, it also necessitates caution when creating named tuples. Any changes would require the creation of a new named tuple. This can have performance consequences when working with very large datasets.
Example of immutability of named tuples:
# Attempt to change a value in a named tuple
john.age = 31 # This will raise an AttributeError
Advanced Usage of Named Tuples
Having grasped the basics of named tuples, it’s time to delve into their more advanced usage.
Built-in Methods
Named tuples come equipped with several built-in methods that enhance their functionality.
Here are some notable ones:
Method | Description | Example |
---|---|---|
_make(iterable) | Creates a named tuple from an iterable | Person._make(['John', 30]) |
_asdict() | Transforms the named tuple into an OrderedDict | john._asdict() |
_replace(**kwargs) | Generates a new instance of the named tuple, replacing specified fields with new values | john._replace(name='Jane') |
Use in Functions and Data Structures
Named tuples can be embedded in functions and data structures just like regular tuples.
Named tuples are particularly beneficial when dealing with complex data structures, as they enhance code readability and debuggability.
Here’s a practical example of a named tuple used in a function:
from collections import namedtuple
# Creating the named tuple
Person=namedtuple('Person', ['name'])
def greet(person):
print('Hello, ' + person.name)
# Creating a person named John
john = Person(name='John')
# Using the function greet
greet(john) # Output: Hello, John
In this example, we’re using a named tuple called Person
with the field name
. We then create a Person
named john, and pass it to the greet
function. The function uses the name
field of the named tuple to generate a greeting.
Potential Pitfalls
Despite their power, named tuples come with potential pitfalls. We’ll cover immutability, case sensitivity, and reserved keywords as being three of the main ones that cause problems.
Immutability
If you attempt to modify a named tuple after its creation, you will encounter an error. To circumvent this, always use the _replace
method when you need to alter the value of a field.
from collections import namedtuple
Person = namedtuple('Person', 'name age')
bob = Person(name='Bob', age=30)
# Attempting to change an attribute
bob.age = 31 # This raises an AttributeError because namedtuples are immutable
# Instead use _replace
bob = bob._replace(age=31)
print(bob) # Output: Person(name='Bob', age=31)
In this example, we see how attempting to change the value of an attribute directly raises an AttributeError
. Instead, we use the _replace()
method, which creates a new namedtuple
with the specified modifications.
Case Sensitivity
Another potential pitfall is the case sensitivity of field names in a named tuple. This means that person.name
and person.NAME
would refer to two different fields. To avoid confusion, it’s advisable to consistently use lower case for field names.
from collections import namedtuple
Person = namedtuple('Person', 'name age')
bob = Person(name='Bob', age=30)
# Case sensitivity in field names, Bob's name is represented in lowercase
try:
print(bob.NAME)
except AttributeError:
print('Field name is case sensitive') # Output: Field name is case sensitive
In this example, we see that attempting to access bob.NAME
raises an AttributeError
because namedtuples’ field names are case sensitive. Therefore, it’s recommended to use lowercase for field names to maintain consistency.
Reserved Keywords
Another common error is using reserved keywords as field names. For instance, class
or def
are reserved words in Python. If you try to use them as field names in your named tuple, Python will raise a ValueError
. To avoid this, ensure your field names are not reserved words.
Example of a common error when using named tuples:
# Attempt to use a reserved keyword as a field name
Person = namedtuple('Person', 'name class') # This will raise a ValueError
When to Use Named Tuples
Named tuples are an ideal choice when dealing with a small, fixed number of fields that are closely related.
They offer more efficiency than classes, and they enhance your code’s readability. However, if you need to add methods or change the fields dynamically, a class would be a more suitable choice.
Data Structure | When to Use | Efficiency | Flexibility |
---|---|---|---|
Named Tuple | When dealing with a small, fixed number of fields that are closely related | More efficient than classes | Fields cannot be changed dynamically |
Class | When you need to add methods or change the fields dynamically | Less efficient than named tuples | Fields can be added or changed dynamically |
In essence, named tuples are a valuable tool in your Python toolkit, and knowing when to utilize them can make your code cleaner and more efficient.
Named tuples are more memory-efficient than classes or dictionaries because they do not need to store attribute names for each instance. However, creating a named tuple is slightly slower than creating a regular tuple or a list due to the extra functionality. In practice, the difference is negligible unless you’re creating millions of named tuples.
Use with Other Python Features
Named tuples can interact seamlessly with other Python features. For instance, you can use named tuples with inheritance. Here’s an illustration:
class Employee(Person):
'Employee is a subclass of Person'
def __init__(self, name, age, job):
super().__init__(name, age)
self.job = job
In this example, Employee
is a subclass of Person
, and it inherits the fields name
and age
from Person
. It also adds a new field job
.
Python Data Structures
While the focus of this article is on named tuples, it may be helpful to review some other data structures in Python. Let’s explore some of these data structures and how they compare to named tuples.
Before we talk about each one, here’s a table summarizing the key differences:
Data Structure | Description | Example |
---|---|---|
List | Ordered collection of items, mutable | [1, 2, 3] |
Dictionary | Unordered collection of key-value pairs, mutable | {'name': 'John', 'age': 30} |
Set | Unordered collection of unique elements | {1, 2, 3} |
Regular Tuple | Ordered collection of items, immutable | (1, 2, 3) |
Named Tuple | Ordered collection of items, immutable, elements can be accessed by name | Person(name='John', age=30) |
Lists
Lists are one of Python’s most frequently used data structures. They are ordered collections of items, which can be of different types.
Unlike named tuples, lists are mutable, meaning you can alter their content without creating a new list. However, lists are less memory-efficient than named tuples, particularly for large data collections.
# Example of List
fruits = ['apple', 'banana', 'cherry']
fruits.append('orange') # We can add to a list
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
Dictionaries
Dictionaries in Python are unordered collections of key-value pairs. Like lists, dictionaries are mutable. They are incredibly efficient for looking up values by their keys.
Unlike named tuples, dictionaries do not maintain the order of their elements. Moreover, dictionaries consume more memory than named tuples.
# Example of Dictionary
fruit_colors = {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'}
fruit_colors['orange'] = 'orange' # We can add to a dictionary
print(fruit_colors) # Output: {'apple': 'red', 'banana': 'yellow', 'cherry': 'red', 'orange': 'orange'}
Sets
Sets are unordered collections of unique elements. They are highly efficient for membership tests, meaning they can quickly determine if an element is in the set or not.
Sets do not support indexing or slicing like named tuples.
# Example of Set
unique_fruits = set(['apple', 'banana', 'cherry', 'apple'])
unique_fruits.add('orange') # We can add to a set, duplicates are not added
print(unique_fruits) # Output: {'cherry', 'banana', 'orange', 'apple'} - Note: order can vary
Regular Tuples
Regular tuples are similar to named tuples. They are ordered, immutable collections of items.
The primary distinction between regular tuples and named tuples is how you access elements. In a regular tuple, elements are accessed by their index, while in a named tuple, you can also use the field name.
# Example of Regular Tuple
person = ('John', 30)
# person[1] = 31 # This would raise an error because tuples are immutable
print(person) # Output: ('John', 30)
Further Resources for Python Data Types
To fortify your mastery of Python’s Data Types, we encourage delving into the following resources that will be extremely beneficial:
- Python Data Types Best Practices – Learn how to use Python sets for efficient membership testing and set operations.
Exploring len() Function in Python – Master Python len() function usage for efficient data analysis and manipulation.
Getting Length of Array in Python: Guide and Examples – Learn how to get the length of an array in Python using built-in functions and methods.
Python’s Official Standard Types Documentation – Comprehensive overview of Python’s standard types from the official docs.
Python’s Official Data Types Documentation – Deep dive into Python’s data types from the official documentation.
W3Schools’ Python Data Types Tutorial – Beginner-friendly tutorial on Python data types by W3Schools.
By deepening your understanding of Python’s Data Types and exploring corresponding topics, you can elevate your prowess and efficiency as a Python developer.
Wrapping Up
We’ve journeyed through a comprehensive guide to named tuples in Python, starting from understanding what named tuples are and how they offer a distinct advantage over regular tuples and lists by enhancing readability and efficiency in your code.
We delved into creating and accessing elements in a named tuple, and we explored their immutability and the positive impact they have on code readability and maintenance.
We also ventured into the advanced usage of named tuples, including their associated methods, their usage in functions and data structures, and their seamless interaction with other Python features. We discussed potential pitfalls of using named tuples and provided tips on how to avoid them.
In our exploration, we also looked at how named tuples fit into the broader ecosystem of Python data structures, comparing them with lists, dictionaries, sets, and regular tuples.
In conclusion, named tuples are a powerful, efficient, and versatile tool in Python. Gaining an understanding and effectively using them can significantly enhance your Python coding experience. So, next time you find yourself reaching for a regular tuple or a list, consider whether a named tuple could be a better option. Happy coding!