Pipenv Guide: Simple Python Package Management
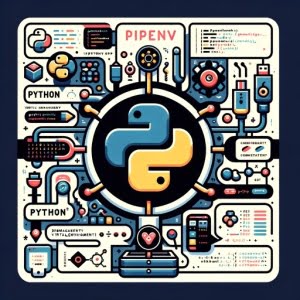
Are you finding it challenging to manage your Python packages? You’re not alone. Many developers find themselves puzzled when it comes to managing Python packages. But, think of Pipenv as a master organizer – it simplifies Python package management.
In this guide, we’ll walk you through the process of using Pipenv, from the basics to more advanced techniques. We’ll cover everything from installing Pipenv, creating a new project, installing packages, to managing different environments, and handling package conflicts.
Let’s get started mastering Pipenv!
TL;DR: What is Pipenv and Why Should I Use It?
Pipenv is a Python packaging tool that combines the best features of pip and virtualenv into one tool. It simplifies package management and environment setup, making it easier for you to manage your Python projects.
Here’s a simple example of how to install a package using Pipenv:
pipenv install requests
In this example, we’re using the pipenv install
command to install the requests
package. This command tells Pipenv to create a new virtual environment (if one doesn’t already exist), and then install the requests
package into that environment.
But that’s just the tip of the iceberg. Pipenv has many more features and capabilities that can help you manage your Python projects more effectively. Continue reading to learn more about Pipenv’s features and usage.
Table of Contents
- Getting Started with Pipenv
- Benefits and Drawbacks of Pipenv
- Advanced Pipenv Techniques
- Alternative Python Packaging Tools
- Solving Common Pipenv Errors
- Best Practices and Optimization Tips
- Understanding Package Management
- Solving Packaging Issues with Pipenv
- Use Cases of PipEnv in Programming
- Pipenv in Larger Python Projects
- Recap: Pipenv Package Management
Getting Started with Pipenv
Installing Pipenv
The first step in using Pipenv is, of course, installing it. This can be done using pip, the Python package installer. Here is how you can install Pipenv:
pip install pipenv
The command above tells pip to install the Pipenv package. Once the installation is complete, you can verify the installation by running:
pipenv --version
This command will display the installed version of Pipenv.
Creating a New Project with Pipenv
Once Pipenv is installed, you can create a new project. Navigate to your project directory and run the following command:
pipenv shell
This command creates a new virtual environment for your project. It isolates your project and its dependencies from other Python projects, preventing potential conflicts.
Installing Packages with Pipenv
Pipenv simplifies the process of installing Python packages. For instance, to install the requests
package, you would run:
pipenv install requests
This command tells Pipenv to install the requests
package into your project’s virtual environment.
Benefits and Drawbacks of Pipenv
Pipenv brings several benefits. It simplifies Python package management, combines the best features of pip and virtualenv, and isolates project environments. However, it might be overkill for simple projects, and its additional features could be confusing for beginners.
Advanced Pipenv Techniques
Managing Different Environments
Pipenv allows you to manage different environments for your project. This is useful when you want to have separate environments for development and production. To create a new environment, you use the pipenv --python
command followed by the version of Python you want to use. Here’s an example:
pipenv --python 3.8
This command creates a new environment using Python 3.8.
Understanding Pipfile and Pipfile.lock
When you use Pipenv, it generates two files: Pipfile
and Pipfile.lock
. The Pipfile
contains the names of the packages your project depends on, and Pipfile.lock
contains the exact version of the packages.
You can view the contents of Pipfile
by using the cat
command:
cat Pipfile
# Output:
# [[source]]
# url = "https://pypi.org/simple"
# verify_ssl = true
# name = "pypi"
# [packages]
# requests = "*"
# [dev-packages]
# [requires]
# python_version = "3.8"
This file shows that our project uses the requests
package and Python 3.8.
Handling Package Conflicts
Sometimes, different packages may depend on different versions of the same package. Pipenv handles these conflicts gracefully. If a conflict arises, Pipenv will inform you about it and suggest a solution.
For example, if you try to install a package that conflicts with an existing one, you would see something like this:
pipenv install conflicting-package
# Output:
# Warning: Your dependencies could not be resolved. You likely have a mismatch in your sub-dependencies.
# You can use $ pipenv graph to see a dependency graph which will help identify the dependencies that are causing the conflict.
In this case, you can use the pipenv graph
command to view a graph of your dependencies and identify the conflict.
Alternative Python Packaging Tools
While Pipenv is a powerful tool, it’s not the only one available for managing Python packages. Other tools like pip, virtualenv, and poetry can also be used, each with its own strengths and weaknesses.
The Classic: pip
pip
is the standard package manager for Python. It’s used to install and manage Python packages. Here’s an example of installing a package using pip:
pip install requests
# Output:
# Collecting requests
# Installing collected packages: requests
# Successfully installed requests
However, pip doesn’t isolate environments, which means packages installed in one project can interfere with another. This is where virtualenv comes in.
Isolating Environments: virtualenv
virtualenv
is a tool to create isolated Python environments. It allows you to create an environment that has its own installation directories and doesn’t share libraries with other virtualenv environments.
Here’s how to create a new environment and activate it using virtualenv:
virtualenv myenv
source myenv/bin/activate
# Output:
# New python executable in /myenv/bin/python
# Installing setuptools, pip, wheel...
# done.
While virtualenv solves the environment isolation problem, it doesn’t manage packages as effectively as Pipenv.
The Newcomer: poetry
poetry
is a relatively new tool that aims to manage Python package creation and distribution. It helps you declare the libraries your project depends on and it will manage (install/update) them for you.
Here’s how to create a new project with poetry:
poetry new myproject
# Output:
# Created package myproject in myproject
While poetry is powerful, it has a steeper learning curve compared to Pipenv and is more suited for package creation rather than application development.
In conclusion, while Pipenv is a great tool, depending on your specific needs and project requirements, other tools like pip, virtualenv, or poetry might be more suitable.
Solving Common Pipenv Errors
Dealing with Dependency Conflicts
One common issue when using Pipenv (or any package manager) is dealing with dependency conflicts. This happens when two packages depend on the same package but require different versions. Pipenv will notify you when such conflicts occur. Let’s simulate a conflict scenario:
pipenv install requests==2.18.0
pipenv install "requests==2.24.0"
# Output:
# Warning: Your dependencies could not be resolved. You likely have a mismatch in your sub-dependencies.
# You can use $ pipenv graph to see a dependency graph which will help identify the dependencies that are causing the conflict.
In this case, we’re trying to install two different versions of the requests
package, which causes a conflict. To resolve this, you can use the pipenv graph
command to identify the conflicting dependencies and choose the correct version.
Handling Missing Packages
Another common issue is missing packages. This usually happens when you’re trying to run your project on a new system or after deleting your Pipfile.lock
file. To resolve this, you can use the pipenv install
command without specifying a package:
pipenv install
# Output:
# Installing dependencies from Pipfile.lock...
This command tells Pipenv to install all the packages listed in your Pipfile.lock
, ensuring your environment has all the necessary packages.
Best Practices and Optimization Tips
- Always specify the version of the packages you’re installing. This ensures that your project remains consistent and works as expected on all systems.
- Regularly update your packages using the
pipenv update
command. This keeps your project secure and efficient. - Use the
pipenv check
command to check for security vulnerabilities in your project. This helps keep your project secure.
Understanding Package Management
Python, a versatile and powerful programming language, offers a vast ecosystem of packages. These packages, or libraries, extend Python’s functionality, allowing developers to avoid ‘reinventing the wheel’ by reusing code written by others.
However, managing these packages can be a daunting task. Different projects may require different versions of the same package, leading to conflicts. Moreover, installing a package might inadvertently upgrade or downgrade other packages, causing your project to break.
This is where Pipenv comes in.
Solving Packaging Issues with Pipenv
Pipenv solves these problems by combining the best features of pip (Python’s package installer) and virtualenv (a tool for creating isolated environments).
With Pipenv, each project gets its own isolated environment. This means each project has its own set of packages, separate from other projects. This solves the problem of conflicting package versions.
Here’s an example of creating a new project with its own environment:
pipenv --python 3.8
This command creates a new project with its own environment, using Python 3.8.
Use Cases of PipEnv in Programming
A virtual environment is like a sandbox for your project. It’s a self-contained area where your project and its dependencies live, isolated from other projects.
Dependency management, on the other hand, is about managing the packages your project depends on. Pipenv does this using the Pipfile
and Pipfile.lock
files. The Pipfile
lists the packages your project needs, and the Pipfile.lock
specifies the exact versions of these packages.
When you install a package, Pipenv updates these files accordingly. For example:
pipenv install requests
# Output:
# Installing requests...
# Adding requests to Pipfile's [packages]...
# Locking [dev-packages] dependencies...
# Locking [packages] dependencies...
# Updated Pipfile.lock (a89f7c)!
In this example, we’re installing the requests
package. Pipenv installs the package, adds it to the Pipfile
, and then updates the Pipfile.lock
.
In conclusion, Pipenv is a powerful tool that simplifies Python package management, making it easier to manage and maintain your Python projects.
Pipenv in Larger Python Projects
When it comes to larger Python projects, Pipenv shows its true strength. Its ability to create isolated environments and manage dependencies efficiently makes it an ideal tool for large-scale applications. For instance, if you have a project with multiple developers working on different parts, Pipenv ensures that everyone is using the same package versions, avoiding potential conflicts.
Pipenv and Docker: A Powerful Combination
Pipenv can also work together with Docker, a platform that automates deploying applications inside lightweight containers. You can use Pipenv to manage your Python packages and Docker to create a container for your application. Here’s an example of a Dockerfile for a Python application using Pipenv:
# Use an official Python runtime as a parent image
FROM python:3.7-slim
# Set the working directory in the container to /app
WORKDIR /app
# Add Pipfile and Pipfile.lock to the container
ADD Pipfile Pipfile.lock /app/
# Install pipenv and install python packages
RUN pip install pipenv && pipenv install --system
# Add the rest of the code
ADD . /app/
# Make port 80 available to the world outside this container
EXPOSE 80
# Run the application when the container launches
CMD ["python", "app.py"]
In this Dockerfile, we’re creating a new Python application, adding our Pipfile and Pipfile.lock, installing our packages using Pipenv, and then running our application.
Pipenv and CI/CD Pipelines
Pipenv can also be integrated into Continuous Integration/Continuous Deployment (CI/CD) pipelines. In a CI/CD pipeline, Pipenv can be used to manage dependencies during the build stage. This ensures that the application is built with the correct package versions.
Further Resources for Pipenv Proficiency
To learn more about Pipenv and how to use it effectively, check out the following resources:
- All About Pip – Python’s Package Manager: Discover how “pip” serves as a package installer and dependency resolver in Python.
Upgrading Python Packages with pip – Best Practices: Learn how to use “pip upgrade” to update all Python packages in one go.
Jumpstarting Python Development – How to Install pip: Master the art of setting up “pip” for Python, enabling you to install and manage packages effortlessly.
Pipenv – A Guide to the New Python Packaging Tool: A comprehensive guide to Pipenv, covering everything from installation to advanced usage.
Python Dev Workflow for Humans: The official Pipenv documentation. It provides an in-depth look at Pipenv and its features.
Python Application Dependency Management in 2018: A YouTube video that provides a comparison of different Python dependency management tools, including Pipenv.
Recap: Pipenv Package Management
In this comprehensive guide, we’ve delved deep into the world of Pipenv, exploring its many features and benefits for managing Python packages.
We began with the basics, learning how to install Pipenv and use it to create new projects and install packages. We then ventured into more complex uses of Pipenv, such as managing different environments, understanding the Pipfile and Pipfile.lock, and handling package conflicts. We also tackled common issues you might encounter when using Pipenv, providing solutions and tips for each challenge.
In our journey, we didn’t neglect alternative tools. We compared Pipenv with other Python package management tools like pip, virtualenv, and poetry, giving you a broader view of the landscape. Here’s a quick comparison of these tools:
Tool | Isolation | Dependency Management | Ease of Use |
---|---|---|---|
Pipenv | High | High | Moderate |
pip | Low | Moderate | High |
virtualenv | High | Low | Moderate |
poetry | High | High | Moderate |
Whether you’re a beginner just starting out with Pipenv or an experienced Python developer looking to level up your package management skills, we hope this guide has given you a deeper understanding of Pipenv and its capabilities.
With its balance of environment isolation, dependency management, and ease of use, Pipenv is a powerful tool for Python package management. Happy coding!