What is Pip? Guide to Python’s Package Installer
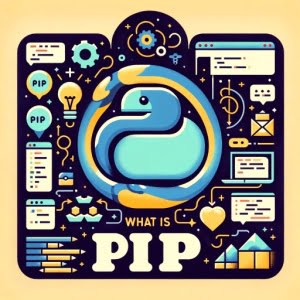
Ever wondered how Python developers manage their libraries? They use a powerful tool called pip. Pip is Python’s go-to package installer.
Pip allows you to install and manage additional libraries and dependencies that are not part of the Python standard library. This makes it a vital tool for Python developers, as it provides an easy way to add functionality to your projects.
This guide will help you understand what pip is, how to use it, and why it’s an essential tool for every Python programmer.
In the following sections, we’ll delve deeper into pip, exploring its basic and advanced uses, alternative approaches, and troubleshooting tips. Whether you’re a beginner or an experienced Python programmer, this guide will give you a comprehensive understanding of pip and its role in Python programming.
TL;DR: What is Pip in Python?
Pip is a package installer for Python. It allows you to install and manage additional libraries and dependencies that are not part of the Python standard library.
Here’s a simple example of how you might use pip to install a library:
pip install requests
# Output:
# Collecting requests
# Downloading requests-2.25.1-py2.py3-none-any.whl (61 kB)
# Installing collected packages: requests
# Successfully installed requests-2.25.1
In this example, we use the command pip install requests
to install the ‘requests’ library. This command tells pip to download and install the ‘requests’ library from the Python Package Index (PyPI).
This is just a basic introduction to pip. Keep reading for a more detailed guide on how to use pip, including advanced usage scenarios, alternative approaches, and troubleshooting tips.
Table of Contents
Basic Package Management with PIP
Pip is incredibly easy to use, even for beginners. Here’s a basic rundown of how you can use pip to manage your Python packages.
Installing Packages with Pip
To install a package, simply use the command pip install
followed by the name of the package. For example, if you want to install the popular ‘requests’ library, you would use the following command:
pip install requests
# Output:
# Collecting requests
# Downloading requests-2.25.1-py2.py3-none-any.whl (61 kB)
# Installing collected packages: requests
# Successfully installed requests-2.25.1
This command tells pip to download and install the ‘requests’ library from the Python Package Index (PyPI). The output shows that the ‘requests’ library was successfully installed.
Upgrading Packages with Pip
To upgrade a package, use the command pip install --upgrade
followed by the name of the package. Here’s an example:
pip install --upgrade requests
# Output:
# Requirement already up-to-date: requests in /usr/local/lib/python3.7/site-packages (2.25.1)
This command tells pip to check if there’s a newer version of the ‘requests’ library available on PyPI. If there is, pip will download and install it. The output shows that the ‘requests’ library is already up-to-date.
Uninstalling Packages with Pip
To uninstall a package, use the command pip uninstall
followed by the name of the package. Here’s an example:
pip uninstall requests
# Output:
# Uninstalling requests-2.25.1:
# Would remove:
# /usr/local/lib/python3.7/site-packages/requests-2.25.1.dist-info/*
# /usr/local/lib/python3.7/site-packages/requests/*
# Proceed (y/n)?
This command tells pip to remove the ‘requests’ library from your system. The output shows that the ‘requests’ library was successfully uninstalled after confirming the action.
These are the basics of using pip to manage your Python packages. In the next section, we’ll discuss some more advanced uses of pip.
Advanced Usage With Python’s Pip
Once you’ve mastered the basics of pip, there’s a lot more you can do with it. Let’s delve into some of the more advanced features of pip, including managing package versions, using pip with virtual environments, and installing packages from different sources.
Managing Package Versions with Pip
Pip allows you to install specific versions of a package. This can be useful when you need to ensure compatibility with other packages or specific versions of Python. To install a specific version of a package, use the command pip install
followed by the name of the package and the version number. Here’s an example:
pip install requests==2.23.0
# Output:
# Collecting requests==2.23.0
# Downloading requests-2.23.0-py2.py3-none-any.whl (58 kB)
# Installing collected packages: requests
# Successfully installed requests-2.23.0
In this example, pip installs version 2.23.0 of the ‘requests’ library. The output shows that the specified version of the ‘requests’ library was successfully installed.
Using Pip with Virtual Environments
Pip can be used in conjunction with virtual environments to manage package dependencies for specific projects. This prevents conflicts between package versions required by different projects. Here’s an example of how to create a virtual environment and use pip to install packages within it:
python -m venv myenv
source myenv/bin/activate
pip install requests
# Output:
# Collecting requests
# Downloading requests-2.25.1-py2.py3-none-any.whl (61 kB)
# Installing collected packages: requests
# Successfully installed requests-2.25.1
In this example, we first create a virtual environment called ‘myenv’ using the venv
module. We then activate the virtual environment using the source
command. Finally, we use pip to install the ‘requests’ library within the virtual environment. The output shows that the ‘requests’ library was successfully installed in the virtual environment.
Installing Packages from Different Sources
While pip usually installs packages from PyPI, it can also install packages from other sources, such as a local directory or a Git repository. Here’s an example of how to install a package from a local directory:
pip install ./my_package
# Output:
# Processing ./my_package
# Building wheels for collected packages: my-package
# Building wheel for my-package (setup.py) ... done
# Created wheel for my-package: filename=my_package-1.0.0-py3-none-any.whl size=4 kB
# Successfully built my-package
# Installing collected packages: my-package
# Successfully installed my-package-1.0.0
In this example, pip installs a package located in a directory called ‘my_package’ in the current directory. The output shows that the package was successfully built and installed.
These are just a few examples of the advanced features of pip. With its versatility and powerful functionality, pip is an essential tool for Python programmers of all skill levels.
Alternative Tools: Pipenv and Conda
While pip is a powerful tool for managing Python packages, it’s not the only option available. There are other tools like Pipenv and Conda that offer additional features and can be better suited to certain use cases.
Pipenv: Python Development Workflow for Humans
Pipenv is a tool that aims to bring the best of all packaging worlds to the Python world. It harnesses Pipfile, pip, and virtualenv into one single command. Here’s how you can use Pipenv to create a new project and install a package:
pip install pipenv
pipenv --python 3.7
pipenv install requests
# Output:
# Creating a virtualenv for this project...
# Pipfile: /home/user/my_project/Pipfile
# Using /usr/bin/python3.7 (3.7.7) to create virtualenv...
# Installing requests...
# Adding requests to Pipfile's [packages]...
# Installation Succeeded
In this example, we first install Pipenv using pip. We then create a new Pipenv project with Python 3.7. Finally, we use Pipenv to install the ‘requests’ library. The output shows that a new virtual environment was created for the project and the ‘requests’ library was successfully installed.
Conda: An Open Source Package Management System
Conda is a cross-platform package manager that can install packages for multiple languages, not just Python. It’s especially good for managing complex dependencies and large data science projects. Here’s how you can use Conda to create a new environment and install a package:
conda create --name myenv
conda activate myenv
conda install requests
# Output:
# Collecting package metadata (current_repodata.json): done
# Solving environment: done
# Installing packages: requests
# Preparing transaction: done
# Verifying transaction: done
# Executing transaction: done
In this example, we first create a new Conda environment called ‘myenv’. We then activate the environment. Finally, we use Conda to install the ‘requests’ library. The output shows that the ‘requests’ library was successfully installed in the Conda environment.
While pip is a powerful and versatile tool, Pipenv and Conda offer additional features that can be beneficial in certain scenarios. Depending on your specific needs, you might find one of these alternatives to be a better fit for your project.
Common Errors and Solutions of PIP
Like any tool, pip can sometimes throw up errors or behave unexpectedly. Here are some common issues you might encounter when using pip, along with their solutions.
Issue: Pip is not recognized as an internal or external command
This error usually means that Python and/or pip is not properly installed, or the system doesn’t know where to find it.
To fix this issue, first, check if Python and pip are correctly installed. Open a terminal and type the following commands:
python --version
pip --version
# Output:
# Python 3.8.5
# pip 20.2.3 from /usr/local/lib/python3.8/site-packages/pip (python 3.8)
If Python and pip are installed correctly, you should see their version numbers. If not, you’ll need to install Python and pip.
Issue: Could not find a version that satisfies the requirement
This error occurs when pip can’t find the package you’re trying to install. This could be because the package doesn’t exist, or because there’s a typo in the package name.
To fix this issue, check the spelling of the package name. If the spelling is correct, try searching for the package on PyPI to see if it exists.
Issue: Failed building wheel for [package]
This error can occur when pip can’t compile a package’s C extension. This could be because the necessary C libraries aren’t installed on your system.
To fix this issue, you might need to install the necessary C libraries. The error message should tell you which libraries are missing.
Best Practices and Optimization Tips
Here are some tips to optimize your usage of pip:
- Always use virtual environments to isolate your project’s dependencies.
- Keep your packages up-to-date by regularly running
pip install --upgrade [package]
. - Use
pip freeze > requirements.txt
to generate arequirements.txt
file, which makes it easier to install all of a project’s dependencies at once.
Pip is a powerful tool, but like any tool, it can sometimes be tricky to work with. However, with these troubleshooting tips and best practices, you should be able to overcome any issues you encounter.
Importance of Package Management
Python package management is a crucial aspect of Python programming, especially when working on complex projects that rely on external libraries. Managing packages effectively helps ensure that your project has access to the functionality it needs to operate correctly.
The Python Package Index (PyPI)
The Python Package Index, or PyPI, is a repository of software for the Python programming language. It’s a vast collection of third-party Python libraries that developers can include in their projects. When you use pip to install a package, it usually installs it from PyPI.
Here’s an example of how pip interacts with PyPI to install a package:
pip install requests
# Output:
# Collecting requests
# Downloading requests-2.25.1-py2.py3-none-any.whl (61 kB)
# Installing collected packages: requests
# Successfully installed requests-2.25.1
In this example, when we run pip install requests
, pip contacts PyPI and requests the ‘requests’ library. PyPI responds by sending the necessary files to our system. Pip then installs these files, making the ‘requests’ library available for our Python programs to use.
Importance of Pip in Package Management
Pip plays a vital role in Python package management. It’s responsible for interfacing with PyPI, downloading and installing packages, managing package versions, and more. Without pip, managing Python packages would be a much more complex and time-consuming task.
In summary, Python package management is a critical aspect of Python programming, and pip is a key tool in any Python programmer’s toolkit. Whether you’re installing a simple library for a small script or managing complex dependencies for a large project, pip makes the process straightforward and efficient.
The Role of Pip in Larger Projects
In larger Python projects, pip plays a key role in managing dependencies. Dependencies are external packages that your project needs to function properly. Pip allows you to easily install, update, and manage these dependencies.
Here’s an example of how pip can manage dependencies in a larger project:
pip install -r requirements.txt
# Output:
# Collecting package1
# Downloading package1-1.0.0-py2.py3-none-any.whl (1.2 MB)
# Collecting package2
# Downloading package2-2.0.0-py2.py3-none-any.whl (1.3 MB)
# Installing collected packages: package1, package2
# Successfully installed package1-1.0.0 package2-2.0.0
In this example, we use pip to install multiple dependencies from a requirements.txt
file. Pip reads the file, downloads the necessary packages from PyPI, and installs them on your system. This makes it easy to manage multiple dependencies in larger projects.
Virtual Environments and Package Versioning
When working on larger projects, it’s often necessary to use specific versions of packages. This can lead to conflicts if different projects on the same system require different versions of the same package. To avoid these conflicts, you can use virtual environments.
Virtual environments allow you to isolate your project’s dependencies from the system’s Python environment. This means you can install specific versions of packages for your project without affecting other projects on the same system.
Package versioning is another important aspect of managing dependencies in larger projects. Pip allows you to specify the version of a package that you want to install. This ensures that your project always uses the correct version of its dependencies.
Further Resources for Mastering Pip
To learn more about pip and Python package management, check out the following resources:
- Simplifying Python Environment Setup: pip install requirements.txt: Learn how to use “pip install requirements.txt” to streamline Python package management.
Python’s Official Documentation on Installing Packages: This guide covers the basics of installing packages in Python, including using pip and virtual environments.
Real Python’s Guide to Pip: This comprehensive guide covers everything you need to know about pip, from basic usage to advanced features.
Python Packaging User Guide: This guide provides an in-depth look at Python package management, including using pip, creating
requirements.txt
files, and more.
Recap: The Importance of Python’s Pip
In conclusion, pip
is a powerful package installer for Python. It serves as a bridge between your Python projects and the vast repository of Python packages available on the Python Package Index (PyPI). From installing a simple library to managing complex dependencies for larger projects, pip makes these tasks straightforward and efficient.
Learning how to use pip effectively is crucial for any Python programmer. With pip, you can easily install, upgrade, and manage Python packages. You can also use specific versions of packages, manage dependencies in larger projects, and troubleshoot common issues.
While pip is an excellent tool, alternatives like Pipenv and Conda offer additional features that can be beneficial in certain scenarios. Depending on your specific needs, you might find one of these alternatives to be a better fit for your project.
Here’s a quick comparison of pip and its alternatives:
Tool | Pros | Cons |
---|---|---|
Pip | Easy to use, versatile, comes pre-installed with Python | Can’t handle complex dependencies as well as some alternatives |
Pipenv | Manages packages and virtual environments in one place, creates lock files to ensure consistent environments | Slower than pip, more complex to use |
Conda | Can handle complex dependencies, can install packages for multiple languages | More complex to use, not as widely used as pip |
In the end, whether you choose to use pip, Pipenv, Conda, or another tool entirely, the important thing is to understand what these tools do and how they can help you manage your Python projects more effectively.